Python Copy File Guide: 8 Ways To Copy a File in Python
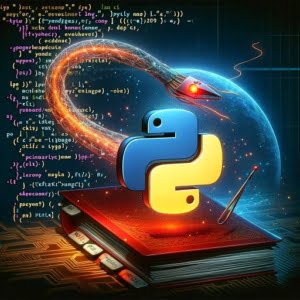
Ever wondered about the secret powers of Python’s built-in modules? Buckle up! We’re about to dive into the fascinating world of copying files in Python.
Python, with its powerful and versatile nature, is packed with a rich library of modules that simplify our coding lives. Among these, the os
, subprocess
, and shutil
modules shine, providing strong support for File Input/Output (I/O) operations. Think of these modules as the unsung heroes of Python, quietly handling the heavy lifting of file operations.
In this blog post, we’re going to dissect the realm of Python file duplication. We’ll uncover various techniques to copy files, offering you a comprehensive understanding of this crucial task. So, whether you’re a novice programmer or a seasoned Pythonista seeking to refresh your skills, this guide is tailor-made for you. Let’s dive in!
TL;DR: How do I copy a file in Python?
Python offers multiple ways to copy a file using built-in modules like
os
,subprocess
, andshutil
. For instance, theshutil.copy()
function can be used to copy a file.
Here’s a simple example:
import shutil
shutil.copy('source.txt', 'destination.txt')
For more advanced methods, background, tips and tricks, continue reading the article.
Table of Contents
- 8 Methods to Copy a File in Python
- 1. The shutil.copyfile() Method
- 2. The shutil.copy() Method
- 3. The shutil.copyfileobj() Method
- 4. The shutil.copy2() Method
- 5. The os.popen() Method
- 6. The os.system() Method
- 7. Asynchronous File Copying with the threading Library
- 8. The subprocess Module
- Further Resources for Python File Mangement
- Wrapping Up: Mastering File Duplication in Python
8 Methods to Copy a File in Python
Python offers a plethora of ways to copy a file, each boasting its unique features and use-cases. Let’s delve into these methods, illuminating their unique characteristics and practical applications.
1. The shutil.copyfile()
Method
Starting with the shutil.copyfile()
method, this function is straightforward and efficient. It takes two parameters: the source file name and the destination file name. It reads the source file and writes its contents to the destination file, creating it if it doesn’t exist, and overwriting it if it does. Here’s an example:
import shutil
shutil.copyfile('source.txt', 'destination.txt')
This method is ideal for simple file copying tasks where you just need to duplicate the content.
2. The shutil.copy()
Method
Here’s an example of how to use shutil.copy()
:
import shutil
shutil.copy('source.txt', 'destination.txt')
Next, we have the shutil.copy()
method. At first glance, it might seem similar to the shutil.copyfile()
method. However, there’s a key difference: while copyfile()
only copies the file’s contents, copy()
also copies the permission bits of the source file to the destination file.
This method is particularly useful when you need to maintain the same file permissions in the copied file.
3. The shutil.copyfileobj()
Method
Here’s an example of how to use shutil.copyfileobj()
:
import shutil
with open('source.txt', 'r') as src, open('destination.txt', 'w') as dst:
shutil.copyfileobj(src, dst)
The shutil.copyfileobj()
method is another useful function for copying files. It takes two file objects as parameters, and copies the contents of the first file object to the second. This is particularly useful when you want to copy files that are already open.
4. The shutil.copy2()
Method
Here’s an example of how to use shutil.copy2()
:
import shutil
shutil.copy2('source.txt', 'destination.txt')
For those who need to copy more than just the file contents and permission bits, there’s shutil.copy2()
. This function works like copy()
, but with an added bonus: it also copies the source file’s metadata, including its timestamps. This can be useful when you need to preserve the file’s last access and modification times.
5. The os.popen()
Method
Last but not least, we have the os.popen()
method. This function opens a pipe to or from the command line. You can use it to execute a shell command that copies a file, like so:
import os
os.popen('cp source.txt destination.txt')
This will copy the file source.txt
to destination.txt
using the shell command cp
.
import os
os.popen('cp source.txt destination.txt')
This method gives you the power and flexibility of the command line, right from your Python script. However, it’s worth noting that this method is not portable across different platforms, so use it with caution.
6. The os.system()
Method
The os.system()
method is a versatile function that can be employed for file copying. This method allows you to execute a command in a subshell, enabling you to run the cp
command, just as you would in a terminal. Here’s an example:
import os
os.system('cp source.txt destination.txt')
While os.system()
is potent, it does come with its limitations. The primary one being its lack of portability as it relies on shell commands that might differ from one operating system to another.
7. Asynchronous File Copying with the threading
Library
Another advanced technique involves using the threading
library to copy files asynchronously. This can be particularly useful when you need to copy multiple files simultaneously.
By copying files in separate threads, you can accelerate the process and make your application more responsive.
Multithreading can lead to potential issues, such as race conditions, where two threads try to access or modify the same data at the same time. It’s crucial to manage your threads carefully to avoid these issues.
8. The subprocess
Module
Here’s an example of how to use subprocess.call()
:
import subprocess
subprocess.call(['cp', 'source.txt', 'destination.txt'])
The subprocess
module offers another advanced method for file copying. The call()
and check_output()
methods allow you to execute external commands and capture their output. This can be handy when you need to copy files using a command-line tool or another external program.
Further Resources for Python File Mangement
To deepen your understanding of Python file handling, here are some resources that you might find insightful:
- Python OS Module Usage – Learn how to navigate and manipulate the file system effortlessly in Python.
Verifying File Existence with Python – Discover the practical applications of file existence checks in Python programming.
Opening Files in Python: A Practical Guide – Learn how to open and access files in Python with the “open” function.
Python’s official documentation on input and output outlines the ways to display outputs and to read inputs.
W3Schools’ explanation of the
fileno
method in Python gives a detailed insight into the built-infileno
file handling method.GeeksforGeeks’ Python file objects guide provides insights into file objects and manipulation in Python.
Wrapping Up: Mastering File Duplication in Python
And that’s a wrap! We’ve traversed the expansive terrain of file duplication in Python, delving into the depths of various methods, each with its unique use-cases.
We kicked off with the basics, unraveling the shutil.copyfile()
, shutil.copy()
, and shutil.copyfileobj()
methods. These functions offer a simple and efficient way to copy files, making them an excellent starting point for Python novices.
We then stepped into advanced territory, exploring the shutil.copy2()
and os.popen()
methods. These functions bring additional features to the table, such as copying file metadata and executing shell commands, offering more flexibility for complex tasks.
Finally, we delved into some advanced techniques, including asynchronous file copying with the threading
library and executing external commands with the subprocess
module. These methods provide potent capabilities for advanced users, but they also come with their own complexities and considerations.
In the end, remember that the best method for copying files in Python hinges on your specific needs and the requirements of your application. By understanding the different methods and their use-cases, you can select the one that best aligns with your situation.
Master the art of Python coding with our exhaustive syntax primer.
So, the next time you find yourself needing to duplicate a file in Python, remember: you have a plethora of options at your disposal. Choose wisely, and happy coding!