Mastering Array to String Conversion in Java
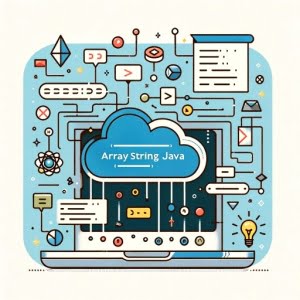
Finding it difficult to convert an array to a string in Java? You’re not alone. Many developers find this task a bit tricky, but Java, like a skilled craftsman, is fully capable of molding arrays into strings.
This guide will walk you through the process of converting arrays to strings in Java, from the basics to more advanced techniques. We’ll cover everything from using the Arrays.toString()
method, handling different types of arrays, to exploring alternative approaches for this conversion.
So, let’s dive in and start mastering the conversion of arrays to strings in Java!
TL;DR: How Do I Convert an Array to a String in Java?
In Java, you can convert an array to a string using the
Arrays.toString()
method. This method is part of thejava.util.Arrays
class and it’s designed to return a string representation of the contents of the specified array.
Here’s a simple example:
String str = Arrays.toString(array);
System.out.println(str);
// Output:
// '[1, 2, 3]'
In this example, we have an array of integers, called array
. We use the Arrays.toString()
method to convert this array into a string, which we then print to the console. The output is a string representation of our array.
This is a basic way to convert an array to a string in Java, but there’s much more to learn about handling different types of arrays and alternative approaches. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Converting Arrays to Strings: The Basics
- Handling Different Array Types in Java
- Exploring Alternative Array-to-String Conversion Methods
- Troubleshooting Array to String Conversion Issues
- Understanding Java Arrays and Strings
- Exploring the Applications of Array to String Conversion
- Wrapping Up: Mastering Array to String Conversion in Java
Converting Arrays to Strings: The Basics
Java provides a built-in method called Arrays.toString()
for converting arrays to strings. This method is part of the java.util.Arrays
class and is designed to return a string representation of the contents of the specified array.
Let’s examine how this method works with a simple example:
int[] array = {1, 2, 3};
String str = Arrays.toString(array);
System.out.println(str);
// Output:
// '[1, 2, 3]'
In this code block, we have an array of integers named array
. We then use the Arrays.toString()
method to convert this array into a string, which is then stored in the str
variable. Finally, we print the str
variable to the console. The output is a string representation of our array, enclosed in square brackets and separated by commas.
Advantages and Potential Pitfalls
The Arrays.toString()
method is straightforward and easy to use, making it an excellent tool for beginners. It works with different types of arrays, including arrays of objects, where it uses the toString()
method of the object to get the string representation.
However, there are a few things to keep in mind when using Arrays.toString()
:
- It doesn’t work as expected with multi-dimensional arrays. For such arrays, you’ll need to use
Arrays.deepToString()
instead. - It returns a string representation of the array, not a human-readable string. For example, if your array contains objects, the method may return the object’s hash code instead of its actual content.
Let’s explore these points in more detail in the next section.
Handling Different Array Types in Java
As you advance in your journey with Java, you’ll encounter different types of arrays. Here, we’ll focus on how to convert multi-dimensional arrays to strings and analyze the output.
Dealing with Multi-Dimensional Arrays
In Java, multi-dimensional arrays are essentially ‘arrays of arrays’. The Arrays.toString()
method doesn’t work quite as expected with them. Instead, Java offers Arrays.deepToString()
, designed specifically for handling multi-dimensional arrays.
Let’s see it in action:
int[][] multiArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
String str = Arrays.deepToString(multiArray);
System.out.println(str);
// Output:
// '[[1, 2, 3], [4, 5, 6], [7, 8, 9]]'
In this example, multiArray
is a two-dimensional array. When we use Arrays.deepToString()
, it correctly converts the multi-dimensional array to a string, with each sub-array enclosed in its own set of square brackets.
Best Practices When Converting Arrays to Strings
When converting arrays to strings in Java, it’s important to:
- Understand the type of array you’re dealing with. Is it a single-dimensional array or a multi-dimensional one? Choose the appropriate method accordingly.
Be aware that
Arrays.toString()
andArrays.deepToString()
return a string representation of the array, not a human-readable string. If your array contains complex objects, consider using a custom method to convert these objects to a more human-readable string.
Exploring Alternative Array-to-String Conversion Methods
While Arrays.toString()
and Arrays.deepToString()
are the standard methods for converting arrays to strings in Java, there are alternative approaches that offer more flexibility and control. Two such methods involve using the StringBuilder
class and Java 8 Streams.
Using StringBuilder
for Conversion
StringBuilder
is a mutable sequence of characters that can be used to create strings. It’s especially useful when you need to modify strings or construct strings from other data types:
int[] array = {1, 2, 3};
StringBuilder builder = new StringBuilder();
for(int i : array) {
builder.append(i).append(" ");
}
String str = builder.toString();
System.out.println(str.trim());
// Output:
// '1 2 3'
In this example, we create a StringBuilder
object and use a for-each loop to append each element of the array, followed by a space, to the StringBuilder
. The resulting string is then trimmed to remove the trailing space.
Leveraging Java 8 Streams
Java 8 introduced Streams, which provide a more declarative approach to working with data. Here’s how you can use a Stream to convert an array to a string:
int[] array = {1, 2, 3};
String str = Arrays.stream(array)
.mapToObj(Integer::toString)
.collect(Collectors.joining(" "));
System.out.println(str);
// Output:
// '1 2 3'
In this example, we create a Stream from the array, map each integer to its string representation, and then collect the results into a single string, with each element separated by a space.
Comparing the Methods
Method | Advantages | Disadvantages |
---|---|---|
Arrays.toString() | Simple, easy to use | Not suitable for multi-dimensional arrays, not very flexible |
Arrays.deepToString() | Handles multi-dimensional arrays | Not very flexible |
StringBuilder | Highly flexible, efficient for large arrays | More verbose, requires manual loop |
Java 8 Streams | Declarative, flexible | More complex, may be slower for large arrays |
In conclusion, while Arrays.toString()
and Arrays.deepToString()
are great for simple use cases, StringBuilder
and Java 8 Streams offer more flexibility and control. Choose the method that best fits your specific needs.
Troubleshooting Array to String Conversion Issues
While converting arrays to strings in Java, you may encounter some common issues. Let’s discuss these problems and provide solutions and workarounds.
Dealing with ‘NullPointerException’
A ‘NullPointerException’ can occur if you try to convert a null array to a string. Here’s an example:
int[] array = null;
String str = Arrays.toString(array);
// Output:
// 'java.lang.NullPointerException'
In this case, before converting an array, it’s good practice to check if it’s null:
int[] array = null;
if (array != null) {
String str = Arrays.toString(array);
System.out.println(str);
} else {
System.out.println("Array is null");
}
// Output:
// 'Array is null'
Handling Different Array Types
As we discussed earlier, using Arrays.toString()
with multi-dimensional arrays doesn’t provide the expected output. Instead, you should use Arrays.deepToString()
.
Final Thoughts on Troubleshooting
When dealing with array to string conversion in Java, it’s essential to understand the type of array you’re working with and use the appropriate method. Also, always check if your array is null before trying to convert it to avoid ‘NullPointerException’. These simple considerations can help you avoid common issues during the conversion process.
Understanding Java Arrays and Strings
To fully grasp the process of converting arrays to strings in Java, it’s crucial to understand the fundamental concepts of arrays and strings in Java.
What is an Array in Java?
An array in Java is a static data structure that holds a fixed number of values of a single type. The elements in an array are stored in contiguous memory locations and can be accessed by their indices.
Here’s how you declare and initialize an array in Java:
int[] array = new int[5];
// Or with values
int[] array = {1, 2, 3, 4, 5};
What is a String in Java?
A string in Java is an object that represents a sequence of characters. Unlike arrays, strings in Java are immutable, which means their values cannot be changed once created.
Here’s how you create a string in Java:
String str = "Hello, World!";
Array to String Conversion: The Fundamentals
When converting an array to a string in Java, what we’re essentially doing is creating a string representation of the array. This involves iterating over the array’s elements and adding each element to the resulting string. As we’ve seen earlier, Java provides several methods to do this, including Arrays.toString()
, Arrays.deepToString()
, using StringBuilder
, and Java 8 Streams.
In the next section, we’ll explore how array to string conversion plays a role in data processing and file handling, and suggest further topics for exploration.
Exploring the Applications of Array to String Conversion
The conversion of arrays to strings in Java is more than just a programming exercise. It has practical applications in various areas of software development, including data processing, file handling, and more.
Array to String Conversion in Data Processing
In data processing, you might need to convert arrays to strings to prepare data for output or to format data for storage. For instance, if you’re working with a list of numerical data points that you want to save to a text file, you might convert that list (array) to a string before writing it to the file.
File Handling and Array to String Conversion
In file handling, array to string conversion can be useful when you’re reading data from a file into an array, processing that data, and then need to write the processed data back to a file. The processed data might need to be in string format to be properly written to the file.
Delving Deeper: Java Collections and Java 8 Streams
If you’re interested in going beyond array to string conversion, you might want to explore related concepts such as Java Collections and Java 8 Streams. Java Collections provide a powerful set of data structures for organizing and managing data, while Java 8 Streams offer a functional approach to data processing.
Further Resources for Mastering Java Conversions
To deepen your understanding of array to string conversion and related topics, here are some resources you might find helpful:
- Java Arrays Tutorial: Getting Started – Dive into one-dimensional and multi-dimensional arrays in Java for versatile data structures.
Sort Array in Java – Explore various sorting algorithms and techniques for arrays in Java.
Exploring String Arrays in Java – Learn about initializing, accessing, and modifying string arrays.
Oracle’s Java Documentation covers all aspects of Java, including Collections and Streams.
GeeksforGeeks’ Java Library includes a vast collection of Java tutorials and articles on array and string manipulations.
Baeldung’s Guide to Java covers many Java topics, including Java 8 Streams and Collections.
Wrapping Up: Mastering Array to String Conversion in Java
In this comprehensive guide, we’ve delved into the process of converting arrays to strings in Java, a common task that holds significant value in various programming scenarios.
We began with the basics, exploring how to use the Arrays.toString()
method for simple array to string conversion. We then delved deeper, examining how to handle different types of arrays, such as multi-dimensional arrays, and the best practices when converting these arrays to strings.
Our journey took us further into alternative approaches for this conversion. We explored the use of StringBuilder
for more flexible conversion and the power of Java 8 Streams for a more declarative approach. Each method was illustrated with practical code examples, shedding light on their usage and effectiveness.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
Arrays.toString() | Simple, easy to use | Not suitable for multi-dimensional arrays, not very flexible |
Arrays.deepToString() | Handles multi-dimensional arrays | Not very flexible |
StringBuilder | Highly flexible, efficient for large arrays | More verbose, requires manual loop |
Java 8 Streams | Declarative, flexible | More complex, may be slower for large arrays |
We also tackled common issues you might encounter during the conversion process, such as ‘NullPointerException’, and provided solutions and workarounds for each issue. This should equip you with the necessary tools to troubleshoot and resolve any challenges you might face.
Whether you’re just starting out with Java or looking to deepen your understanding of array to string conversion, we hope this guide has been a valuable resource. The ability to efficiently convert arrays to strings is a vital skill in your Java toolkit. Happy coding!