Bash Arrays: A Syntax and Use Cases Guide
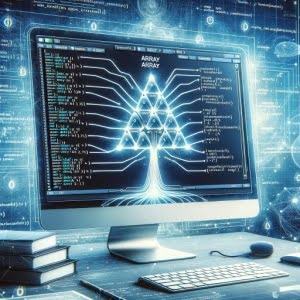
Are you finding it challenging to work with arrays in Bash scripting? You’re not alone. Many developers find themselves puzzled when it comes to handling arrays in Bash, but we’re here to help.
Think of Bash arrays as a well-organized bookshelf – allowing you to store and manage your data with ease. These arrays can help you manipulate data effectively, making Bash arrays a powerful tool for scripting tasks.
In this guide, we’ll walk you through the process of working with Bash arrays, from their creation, manipulation, and usage. We’ll cover everything from the basics of arrays to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use Arrays in Bash?
To create an array in Bash, you can use the following syntax:
array=(element1 element2 element3)
. This command initializes an array with three elements.
Here’s a simple example:
array=("Apple" "Banana" "Cherry")
echo ${array[1]}
# Output:
# Banana
In this example, we create an array named ‘array’ with three elements: ‘Apple’, ‘Banana’, and ‘Cherry’. Then, we print the second element of the array (remember, Bash arrays are zero-indexed, so index 1 corresponds to the second element). The output of this command is ‘Banana’.
This is a basic way to use arrays in Bash, but there’s much more to learn about creating and manipulating arrays in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Bash Array Basics: Creation, Access, and Modification
In Bash, creating an array is as simple as assigning values. You can create an array by assigning elements separated by spaces to a variable. Here’s how you do it:
fruits=('Apple' 'Banana' 'Cherry')
echo ${fruits[*]}
# Output:
# Apple Banana Cherry
In this example, we’ve created an array named ‘fruits’ with three elements: ‘Apple’, ‘Banana’, and ‘Cherry’. The echo ${fruits[*]}
command prints all elements of the array.
Accessing Array Elements
To access an element from the array, you use the index of that element. Remember, Bash arrays are zero-indexed, meaning the first element is at index 0. Here’s how you can access an element:
fruits=('Apple' 'Banana' 'Cherry')
echo ${fruits[0]}
# Output:
# Apple
In this example, ${fruits[0]}
accesses the first element of the ‘fruits’ array, which is ‘Apple’.
Modifying Array Elements
Modifying an element in an array is as straightforward as accessing it. Here’s how you can change the value of an array element:
fruits=('Apple' 'Banana' 'Cherry')
fruits[1]='Blueberry'
echo ${fruits[*]}
# Output:
# Apple Blueberry Cherry
In this example, we replaced the second element ‘Banana’ with ‘Blueberry’. The echo ${fruits[*]}
command then prints the updated array.
Understanding these basics of Bash arrays is crucial for managing and manipulating your data effectively in Bash scripting.
Advanced Bash Array Operations
As you become more comfortable with Bash arrays, you can start to explore more complex operations. These include adding elements, deleting elements, and iterating over an array. Let’s dive in.
Adding Elements to an Array
Adding an element to a Bash array is simple. You can add an element to the end of the array using the following syntax:
fruits=('Apple' 'Banana' 'Cherry')
fruits+=('Dragonfruit')
echo ${fruits[*]}
# Output:
# Apple Banana Cherry Dragonfruit
In this example, we’ve added ‘Dragonfruit’ to the end of the ‘fruits’ array. The echo ${fruits[*]}
command then prints the updated array.
Deleting Elements from an Array
Deleting an element from a Bash array is as simple as adding one. Here’s how you can delete an element from an array:
fruits=('Apple' 'Banana' 'Cherry')
unset fruits[1]
echo ${fruits[*]}
# Output:
# Apple Cherry
In this example, we used the unset
command to remove the second element ‘Banana’ from the ‘fruits’ array. The echo ${fruits[*]}
command then prints the updated array.
Iterating Over an Array
Finally, let’s discuss how to iterate over a Bash array. This is useful when you want to perform the same operation on each element of the array. Here’s an example:
fruits=('Apple' 'Banana' 'Cherry')
for fruit in "${fruits[@]}"
do
echo "I love $fruit"
done
# Output:
# I love Apple
# I love Banana
# I love Cherry
In this example, we used a for loop to iterate over each element of the ‘fruits’ array. For each element, we printed a statement saying ‘I love [fruit]’. This is a powerful technique for processing arrays in Bash.
By mastering these advanced operations, you can manipulate Bash arrays to suit your needs and make your Bash scripts more powerful and flexible.
Expert Techniques: Bash Array Slicing and Indirect References
As you delve deeper into Bash scripting, you’ll find there are more advanced techniques for manipulating arrays. Let’s explore two such techniques: array slicing and indirect references.
Array Slicing in Bash
Array slicing is a method to retrieve a range of elements from an array. Here’s how you can slice an array in Bash:
fruits=('Apple' 'Banana' 'Cherry' 'Dragonfruit' 'Elderberry')
slice=${fruits[@]:1:3}
echo ${slice[*]}
# Output:
# Banana Cherry Dragonfruit
In this example, we’ve created an array ‘fruits’ with five elements. The slice ${fruits[@]:1:3}
retrieves three elements starting from index 1 (second element). The echo ${slice[*]}
command then prints the sliced array. Array slicing is a powerful tool when you need to work with a subset of an array.
Indirect References in Bash
Indirect references allow you to use the value of a variable as the name of another variable. Here’s an example of how you can use indirect references with arrays in Bash:
fruits=('Apple' 'Banana' 'Cherry')
index=1
ref='fruits[index]'
echo ${!ref}
# Output:
# Banana
In this example, we’ve created an array ‘fruits’ with three elements. We then set ‘index’ to 1 and ‘ref’ to ‘fruits[index]’. The ${!ref}
syntax is an indirect reference that gets the value of the variable named by ‘ref’, which in this case is the second element of the ‘fruits’ array. The echo ${!ref}
command then prints this element. Indirect references can be useful when you need to dynamically access array elements.
By leveraging these advanced techniques, you can manipulate Bash arrays in more sophisticated ways, making your Bash scripts even more flexible and powerful.
Troubleshooting Common Bash Array Issues
As with any programming concept, working with Bash arrays can sometimes be tricky. Let’s discuss a couple of common issues you may encounter, such as dealing with spaces in array elements and handling associative arrays.
Dealing with Spaces in Array Elements
Spaces can be a source of confusion when working with Bash arrays. If an element in your array contains a space, Bash may interpret it as a separate element. Here’s an example demonstrating this issue, and how to resolve it:
fruits=('Red Apple' 'Yellow Banana' 'Black Cherry')
echo ${fruits[1]}
# Output:
# Yellow
In this example, we intended ‘Yellow Banana’ to be a single element. However, Bash interpreted ‘Yellow’ and ‘Banana’ as separate elements because of the space. To avoid this, you should enclose elements with spaces in quotes:
fruits=("Red Apple" "Yellow Banana" "Black Cherry")
echo ${fruits[1]}
# Output:
# Yellow Banana
Handling Associative Arrays
Bash also supports associative arrays, where you can use keys instead of indexes to access elements. Here’s an example of how to declare an associative array, and a common error you might encounter:
declare -A fruits
fruits=(['color']='Red' ['name']='Apple')
echo ${fruits['name']}
# Output:
# Apple
In this example, we declared an associative array ‘fruits’ with two elements: ‘color’ with a value of ‘Red’, and ‘name’ with a value of ‘Apple’. We then accessed the ‘name’ element using its key. Remember, when using associative arrays, you must declare them with declare -A
before using them.
By being aware of these common issues and their solutions, you can avoid potential pitfalls and make your Bash scripting more effective.
Understanding Arrays in Bash
Before we delve deeper into the usage of arrays in Bash, let’s take a step back and understand what an array is and why it’s so crucial in scripting and programming in general.
An array is a data structure that stores a collection of elements. These elements, also known as values, can be accessed by an index that points to a specific location in the array. Arrays can be visualized as a series of boxes, each holding a value, and each having a unique address (the index).
# An example of an array in Bash
fruits=('Apple' 'Banana' 'Cherry')
# Output:
# No output as we're just declaring an array
In the above code block, we’ve created an array named ‘fruits’ with three elements. Each element can be accessed using its index, which in Bash, starts at 0.
Arrays play a vital role in scripting and programming due to their versatility and efficiency. They allow programmers to store multiple values in a single variable, which can then be easily manipulated. This is particularly useful in situations where a script needs to handle a variable amount of data.
In Bash, arrays are not as powerful or as flexible as in other programming languages. However, they are still a valuable tool for handling lists of strings and can be used in a variety of ways to enhance your Bash scripts.
Bash Arrays in Larger Scripts and Projects
Bash arrays are not just useful for small scripts; they play a significant role in larger scripts and projects as well. The ability to store and manipulate sets of data is a fundamental requirement in many scripting scenarios, making Bash arrays an essential tool in a developer’s arsenal.
Exploring Related Concepts
As you become more comfortable with Bash arrays, you may want to explore related concepts. For instance, Bash associative arrays allow you to use arbitrary strings as array indices instead of just integers. This can be particularly useful when you need to map keys to values.
declare -A fruits
fruits=(['color']='Red' ['name']='Apple')
echo ${fruits['name']}
# Output:
# Apple
In this example, we’ve created an associative array ‘fruits’ with two elements. Each element is accessed using a string key instead of an integer index.
Bash doesn’t support multi-dimensional arrays directly, but there are ways to emulate them. This can be useful when you need to work with more complex data structures.
Lastly, Bash offers other data structures, such as strings and integers, which can be combined with arrays to create more complex and versatile scripts.
Further Resources for Mastering Bash Arrays
For those looking to delve deeper into Bash arrays and related topics, here are some valuable resources:
- Advanced Bash-Scripting Guide: This guide provides an in-depth look at Bash scripting, including a detailed section on arrays.
- GeeksforGeeks – Bash Scripting Array: This guide explores the topic of Bash scripting arrays, discussing various aspects and providing examples.
- GNU Bash Manual: The official manual for Bash includes comprehensive information on arrays and other Bash features.
By exploring these resources and practicing with Bash arrays, you can enhance your Bash scripting skills and tackle more complex scripting challenges.
Wrapping Up: Mastering Bash Arrays
In this comprehensive guide, we’ve dissected the world of Bash arrays, a fundamental construct in Bash scripting. From basic creation and manipulation to advanced operations and alternative approaches, we’ve delved deep into the intricacies of Bash arrays.
We began with the basics, understanding how to create, access, and modify elements in Bash arrays. We then ventured into more advanced territory, exploring complex operations such as adding and deleting elements, and iterating over an array. We also uncovered expert techniques, including array slicing and indirect references, and how to leverage these techniques to manipulate Bash arrays in sophisticated ways.
Along the way, we tackled common challenges you might encounter when dealing with Bash arrays, such as handling spaces in array elements and working with associative arrays. We provided solutions to these issues, equipping you with the knowledge to overcome potential pitfalls.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Use | Simple and straightforward | Limited functionality |
Advanced Use | More powerful operations | Slightly more complex |
Alternative Approaches | Offers sophisticated techniques | Requires deeper understanding |
Whether you’re just starting out with Bash arrays, or you’re looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash arrays and their capabilities.
With the ability to effectively manage and manipulate data, Bash arrays are a powerful tool in any developer’s toolkit. Now, you’re well equipped to harness the power of Bash arrays in your scripts. Happy scripting!