Python NaN: Guide To “Not a Number” / Undefined Values
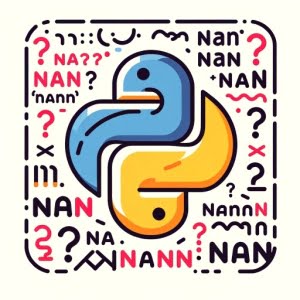
Are you wrestling with NaN values in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling these elusive values in Python. Think of Python’s NaN values as ghosts – they’re there, but not quite tangible or visible.
Like a skilled detective, Python provides us with the tools to detect and handle these NaN values. These tools are essential for data analysis and machine learning tasks, where NaN values can often appear and cause issues if not properly handled.
In this guide, we’ll walk you through the process of detecting and handling NaN values in Python, from the basics to more advanced techniques. We’ll cover everything from checking for NaN values using the math.isnan()
function and pandas isnull()
function, to handling NaN values using pandas fillna()
function and dropna()
function, and even more advanced techniques.
Let’s dive in and start mastering Python NaN!
TL;DR: What is NaN in Python and How to Handle It?
NaN, standing for ‘Not a Number’, is a special floating-point value that represents missing or undefined values in Python. You can detect NaN values using the
math.isnan()
function or pandasisnull()
function. To handle NaN values, you can use pandasfillna()
function ordropna()
function.
Here’s a simple example:
import math
import pandas as pd
# Using math.isnan()
print(math.isnan(float('nan'))) # Returns: True
# Using pandas isnull()
df = pd.DataFrame({'A': [1, 2, float('nan')]})
print(df['A'].isnull())
# Returns:
# 0 False
# 1 False
# 2 True
# Name: A, dtype: bool
# Handling NaN using fillna()
df['A'].fillna(0, inplace=True)
print(df)
# Returns:
# A
# 0 1.0
# 1 2.0
# 2 0.0
# Handling NaN using dropna()
df = pd.DataFrame({'A': [1, 2, float('nan')]})
df.dropna(inplace=True)
print(df)
# Returns:
# A
# 0 1.0
# 1 2.0
In this example, we first check for NaN values using math.isnan()
and pandas’ isnull()
function. Then, we handle the NaN values using pandas’ fillna()
function, which replaces NaN values with a specified value (in this case, 0), and dropna()
function, which removes rows with NaN values.
But there’s much more to handling NaN values in Python. Continue reading for more detailed information and advanced techniques.
Table of Contents
- Understanding and Detecting NaN in Python
- Handling NaN Values with Pandas
- Advanced Techniques for Handling Python NaN
- Troubleshooting Common Issues with Python NaN
- Unraveling Python NaN: What, Why, and How?
- The Bigger Picture: Python NaN in Data Analysis and Machine Learning
- Wrapping Up: Mastering Python NaN for Effective Data Handling
Understanding and Detecting NaN in Python
In Python, NaN stands for ‘Not a Number’. It’s a special floating-point value that signifies undefined or unrepresentable values, especially in the field of data analysis and machine learning. NaN values in Python are represented as float('nan')
.
Checking for NaN using math.isnan()
The math.isnan()
function is a handy tool in Python’s math module for checking if a value is NaN. It returns True
if the value is NaN and False
otherwise.
Here’s how you can use math.isnan()
:
import math
print(math.isnan(float('nan'))) # Returns: True
print(math.isnan(10)) # Returns: False
In this example, math.isnan(float('nan'))
returns True
because float('nan')
is a NaN value, while math.isnan(10)
returns False
because 10 is not a NaN value.
Checking for NaN using pandas’ isnull()
When dealing with pandas DataFrames or Series, you can use the isnull()
function to check for NaN values. This function returns a Boolean mask of the same shape as the DataFrame or Series, where True
indicates NaN values.
Here’s an example:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, float('nan')]})
print(df['A'].isnull())
# Output:
# 0 False
# 1 False
# 2 True
# Name: A, dtype: bool
In this DataFrame, the isnull()
function returns False
for the first two values because they are not NaN, and True
for the third value because it is NaN.
Handling NaN Values with Pandas
Once you’ve detected NaN values in your data, the next step is to handle them. Two commonly used methods in pandas for handling NaN values are the fillna()
function and the dropna()
function.
Using fillna()
to Replace NaN Values
The fillna()
function allows you to replace NaN values with a specified value. This is especially useful when you want to fill in missing data with a default value or an average value.
Here’s an example of how to use fillna()
:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, float('nan')]})
df['A'].fillna(0, inplace=True)
print(df)
# Output:
# A
# 0 1.0
# 1 2.0
# 2 0.0
In this example, the fillna(0)
function replaces the NaN value in the DataFrame with 0.
Using dropna()
to Remove NaN Values
The dropna()
function allows you to remove rows or columns with NaN values from your DataFrame. This is useful when you want to exclude missing data from your analysis.
Here’s how you can use dropna()
:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, float('nan')]})
df.dropna(inplace=True)
print(df)
# Output:
# A
# 0 1.0
# 1 2.0
In this example, the dropna()
function removes the row with the NaN value from the DataFrame.
Pros and Cons
Both fillna()
and dropna()
have their pros and cons. The fillna()
function allows you to maintain the size of your DataFrame, but the replacement value might skew your data. On the other hand, dropna()
ensures that you’re only working with valid data, but it reduces the size of your DataFrame.
Advanced Techniques for Handling Python NaN
Beyond pandas’ fillna()
and dropna()
, there are more advanced techniques for handling NaN values in Python, such as using scikit-learn’s Imputer
class or machine learning algorithms that can handle NaN values.
Using Scikit-learn’s Imputer
Class
Scikit-learn’s Imputer
class provides a more sophisticated way to fill in missing values. It allows you to replace missing values using the mean, median, or most frequent value along each column.
Here’s an example:
from sklearn.impute import SimpleImputer
import numpy as np
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan]})
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
df['A'] = imputer.fit_transform(df[['A']]).ravel()
print(df)
# Output:
# A
# 0 1.0
# 1 2.0
# 2 1.5
In this example, the SimpleImputer
replaces the NaN value with the mean of the other values in the column.
Using Machine Learning Algorithms that Handle NaN
Some machine learning algorithms, like XGBoost or LightGBM, can handle NaN values right out of the box. They treat NaN values as a separate category and find the best way to handle them during the model training process.
Pros and Cons
These advanced techniques provide more flexibility in handling NaN values. However, they may also introduce additional complexity and computational cost. For instance, scikit-learn’s Imputer
class requires extra computation to calculate the mean, median, or most frequent value. Machine learning algorithms that handle NaN values may require more computational resources and time to train.
Troubleshooting Common Issues with Python NaN
Handling NaN values in Python can sometimes lead to unexpected results or performance issues. Here, we’ll discuss some common issues and their solutions.
Unexpected Results
One common issue is that operations involving NaN values often result in NaN. This is because NaN is a ‘viral’ value — any operation involving NaN will result in NaN.
import numpy as np
print(np.nan + 1) # Returns: nan
print(np.nan * 0) # Returns: nan
In these examples, any arithmetic operation involving NaN returns NaN. To avoid this, you need to handle NaN values before performing operations.
Performance Issues
Another issue is that handling NaN values, especially in large datasets, can lead to performance issues. For instance, using pandas’ fillna()
function to fill a large DataFrame can be computationally expensive.
To mitigate this, you can use the inplace=True
parameter to modify the existing DataFrame instead of creating a new one. Alternatively, you can use NumPy’s numpy.nan_to_num()
function, which is faster than pandas’ fillna()
.
import numpy as np
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan]})
df['A'] = np.nan_to_num(df['A'])
print(df)
# Output:
# A
# 0 1.0
# 1 2.0
# 2 0.0
In this example, numpy.nan_to_num()
replaces the NaN value with 0, and it’s faster than fillna()
for large datasets.
Unraveling Python NaN: What, Why, and How?
In the realm of programming and data science, NaN is a concept that often puzzles beginners. So, what exactly is NaN? In Python, NaN stands for ‘Not a Number’. It’s a special floating-point value that represents undefined or unrepresentable values.
The Existence of NaN
NaN values exist for a variety of reasons. In data analysis and machine learning, NaN often signifies missing data. For example, if you’re analyzing a dataset of survey responses, and some respondents didn’t answer certain questions, those missing responses might be represented as NaN values.
The Importance of Handling NaN
So, why is it crucial to handle NaN values? NaN values can distort your data analysis and machine learning models. For instance, if you’re calculating the average of a list of numbers, and one of those numbers is NaN, the result will be NaN, irrespective of the other numbers. This can lead to misleading results.
Here’s an example:
import numpy as np
numbers = [1, 2, 3, np.nan]
print(np.mean(numbers)) # Returns: nan
In this example, even though the list contains three valid numbers, the presence of a single NaN value causes the mean to be NaN. This underlines the importance of handling NaN values correctly.
NaN in Data Analysis and Machine Learning
In data analysis and machine learning, handling NaN values is a crucial part of the data cleaning process. Depending on the nature of the data and the specific analysis or model, NaN values can be replaced with a specific value (such as 0 or the mean of the data), or the rows or columns containing NaN values can be removed entirely.
In a nutshell, understanding and correctly handling NaN values is fundamental to effective data analysis and machine learning in Python.
The Bigger Picture: Python NaN in Data Analysis and Machine Learning
Understanding and handling Python’s NaN values is not just a matter of mastering a specific function or technique. It’s a crucial part of the broader fields of data analysis and machine learning. In these fields, NaN values often signify missing data, and how you choose to handle these NaN values can significantly influence your analysis or model’s results.
Exploring Related Concepts
Once you’ve mastered handling NaN values, you might want to explore related concepts like data cleaning and handling missing data. Data cleaning involves more than just handling NaN values. It also includes tasks like removing duplicate data, handling outliers, and normalizing data. Similarly, handling missing data involves strategies like data imputation, where missing values are replaced with substituted values.
Further Resources for Mastering Python NaN
To deepen your understanding of Python NaN and related concepts, here are some resources you might find helpful:
- IOFlood’s Python Math Article can help with leveraging the mathematical capabilities of the math module.
Python Increment by 1: Quick Examples – Discover quick and easy examples of incrementing in Python for iterative tasks
Exploring Exponentiation in Python – Master Python exponentiation techniques for performing mathematical operations.
Pandas Documentation – A comprehensive resource for all things pandas, including examples of functions for handling NaN values.
Scikit-Learn Documentation is great for machine learning in Python, including techniques for dealing with missing values.
Python for Data Analysis provides a comprehensive introduction to data analysis in Python, including handling NaN values.
Remember, mastering Python NaN is a stepping stone to becoming proficient in data analysis and machine learning. So keep exploring, keep learning, and keep coding!
Wrapping Up: Mastering Python NaN for Effective Data Handling
In this comprehensive guide, we’ve delved deep into the world of Python NaN, a floating-point value representing undefined or unrepresentable values in Python.
We began with the basics, understanding what NaN values are and how to detect them using the math.isnan()
function and pandas’ isnull()
function. We then explored advanced techniques for handling NaN values, including the use of pandas’ fillna()
and dropna()
functions, scikit-learn’s Imputer
class, and machine learning algorithms that can handle NaN values right out of the box.
We also discussed common issues you might encounter when handling NaN values, such as unexpected results and performance issues, and provided solutions to these challenges. Furthermore, we touched on the importance of handling NaN values in the broader context of data analysis and machine learning, and suggested further resources for mastering Python NaN.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
math.isnan() /isnull() | Simple, easy to use | Only for detecting NaN |
fillna() | Maintains DataFrame size | Replacement value might skew data |
dropna() | Ensures valid data | Reduces DataFrame size |
Scikit-learn’s Imputer | Flexible, sophisticated | Computationally expensive |
ML algorithms | Handles NaN during training | Requires more resources, time |
Whether you’re just starting out with Python NaN or looking to refine your skills, we hope this guide has provided you with a comprehensive understanding of Python NaN and its significance in data analysis and machine learning.
Mastering Python NaN is a fundamental step towards effective data handling in Python. With these techniques in your arsenal, you’re well-equipped to handle NaN values and clean your data for analysis or modeling. Happy coding!