Compare Numbers in Bash | Scripting Command Guide
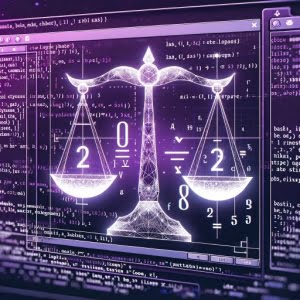
Ever found yourself puzzled when trying to compare numbers in Bash? You’re not alone. Many developers find themselves in a maze when it comes to handling number comparisons in Bash, but we’re here to help.
Think of Bash’s number comparison as a referee in a race – it can help you determine which number is greater, lesser, or if they are equal. It’s a powerful tool that can make your scripts more dynamic and responsive.
This guide will walk you through the process of comparing numbers in Bash, from basic to advanced techniques. We’ll cover everything from the basics of using relational operators to more complex comparisons and even alternative approaches.
So, let’s dive in and start mastering number comparison in Bash!
TL;DR: How Do I Compare Numbers in Bash?
In Bash, you can compare numbers using relational operators such as
-eq
,-ne
,-gt
,-lt
,-ge
,-le
. These operators allow you to evaluate the relationship between two numbers.
Here’s a simple example:
num1=10
num2=20
if [ "$num1" -gt "$num2" ]; then
echo "$num1 is greater than $num2"
else
echo "$num1 is not greater than $num2"
fi
# Output:
# '10 is not greater than 20'
In this example, we’re comparing two numbers, num1
and num2
, using the -gt
(greater than) operator. The if
statement checks if num1
is greater than num2
. If the condition is true, it prints ’10 is greater than 20′. If not, it prints ’10 is not greater than 20′. In this case, since 10 is not greater than 20, the latter message is printed.
This is just the tip of the iceberg when it comes to comparing numbers in Bash. Continue reading for a more in-depth explanation and advanced usage scenarios.
Table of Contents
- Getting Started with Bash Number Comparison
- Complex Comparisons in Bash
- Exploring Alternative Methods for Number Comparison
- Avoiding Pitfalls in Bash Number Comparison
- Understanding Bash Scripting and Relational Operators
- The Bigger Picture: Number Comparison in Larger Scripts
- Wrapping Up: Mastering Number Comparison in Bash
Getting Started with Bash Number Comparison
Before we dive into the deep end, let’s start with the basics of comparing numbers in Bash. We’ll begin by introducing you to relational operators, which are the heart of number comparison in Bash.
In Bash, there are six relational operators for number comparison:
-eq
: Checks if two numbers are equal-ne
: Checks if two numbers are not equal-gt
: Checks if the first number is greater than the second number-lt
: Checks if the first number is less than the second number-ge
: Checks if the first number is greater than or equal to the second number-le
: Checks if the first number is less than or equal to the second number
Let’s see these relational operators in action with a simple example:
num1=7
num2=12
if [ "$num1" -eq "$num2" ]; then
echo "$num1 and $num2 are equal"
else
echo "$num1 and $num2 are not equal"
fi
# Output:
# '7 and 12 are not equal'
In this example, we’re using the -eq
operator to check if num1
is equal to num2
. The if
statement evaluates the condition and, based on its truthfulness, executes the appropriate echo
command. Here, since 7 is not equal to 12, the message ‘7 and 12 are not equal’ is printed.
Using these operators, you can build conditions in your Bash scripts to perform different actions based on the relationship between two numbers. But, be aware of potential pitfalls. For instance, these operators only work with integers. If you try to use them with floating-point numbers or non-numeric values, you’ll encounter errors.
In the next section, we’ll explore more complex comparisons and how to handle them in Bash.
Complex Comparisons in Bash
Now that you’re comfortable with the basics, let’s dive into more complex scenarios. Bash scripting allows for comparisons beyond just two numbers. You can compare multiple numbers and even handle floating-point numbers, albeit with a bit more finesse.
Comparing Multiple Numbers
Comparing more than two numbers in Bash involves nested if
statements or using logical operators like &&
(AND) and ||
(OR). Let’s look at an example where we compare three numbers to find the largest:
num1=7
num2=12
num3=9
if [ "$num1" -ge "$num2" ] && [ "$num1" -ge "$num3" ]; then
echo "$num1 is the largest number"
elif [ "$num2" -ge "$num1" ] && [ "$num2" -ge "$num3" ]; then
echo "$num2 is the largest number"
else
echo "$num3 is the largest number"
fi
# Output:
# '12 is the largest number'
In this code, we’re using the -ge
operator in conjunction with the &&
logical operator to check if num1
or num2
is greater than or equal to the other two numbers. If neither condition is true, we conclude that num3
is the largest.
Handling Floating Point Numbers
As mentioned earlier, Bash’s built-in comparison operators only work with integers. To compare floating-point numbers, we need to call upon external utilities like bc
:
num1=7.5
num2=7.2
comparison=$(echo "$num1 > $num2" | bc)
if [ "$comparison" -eq 1 ]; then
echo "$num1 is greater than $num2"
else
echo "$num1 is not greater than $num2"
fi
# Output:
# '7.5 is greater than 7.2'
In this example, we use bc
to perform the comparison and then evaluate its result (1
for true, 0
for false) using the -eq
operator.
These advanced techniques open up new possibilities for your Bash scripts, allowing them to handle more complex conditions and data types. In the next section, we’ll explore alternative approaches for comparing numbers in Bash.
Exploring Alternative Methods for Number Comparison
Bash provides a variety of ways to compare numbers, each with its unique advantages. Let’s explore some of the alternative approaches you can use to compare numbers in Bash.
Using the ‘expr’ Command
The ‘expr’ command is a versatile utility in Bash that can also be used for number comparison. It supports relational operators like ‘=’, ‘!=’, ‘>’, ‘=’, and ‘<=’.
Here’s how you can use ‘expr’ to compare numbers:
num1=10
num2=20
result=$(expr $num1 \<= $num2)
if [ $result -eq 1 ]; then
echo "$num1 is less than or equal to $num2"
else
echo "$num1 is not less than or equal to $num2"
fi
# Output:
# '10 is less than or equal to 20'
In this example, we’re using ‘expr’ to compare num1
and num2
. The expr
command returns 1
if the condition is true and 0
if it’s false. We then evaluate this result using the -eq
operator.
Comparing Numbers with ‘awk’
‘awk’ is a powerful text-processing command in Unix, and it can also be used to compare numbers in Bash. Unlike Bash’s built-in operators and ‘expr’, ‘awk’ can handle floating-point numbers without an issue.
Let’s see ‘awk’ in action:
num1=7.2
num2=7.5
awk -v n1=$num1 -v n2=$num2 'BEGIN {if (n1>n2) print n1" is greater than "n2; else print n1" is not greater than "n2}'
# Output:
# '7.2 is not greater than 7.5'
In this example, we’re passing num1
and num2
to ‘awk’ as variables n1
and n2
using the -v
option. We then use ‘awk’s built-in comparison operators to compare the numbers.
Both ‘expr’ and ‘awk’ offer more flexibility than Bash’s built-in operators, allowing you to handle more complex scenarios and data types. Depending on your needs, you might find them more suitable for your scripts.
Avoiding Pitfalls in Bash Number Comparison
As with any programming language, Bash scripting comes with its share of potential issues and pitfalls. When comparing numbers in Bash, you might encounter a few common problems. Let’s discuss these issues and how to tackle them.
Dealing with Non-Numeric Input
One of the most common issues when comparing numbers in Bash is dealing with non-numeric input. Bash’s built-in operators, ‘expr’, and ‘awk’ all expect numeric input. If they receive a non-numeric value, they’ll throw an error.
To handle this, you can use Bash’s built-in pattern matching to check if a variable contains a valid number before performing the comparison:
num="abc"
if [[ $num =~ ^[0-9]+$ ]]; then
echo "$num is a valid number"
else
echo "$num is not a valid number"
fi
# Output:
# 'abc is not a valid number'
In this example, we’re using the =~
operator to check if num
matches the regular expression ^[0-9]+$
, which matches any string consisting solely of digits. If num
is a valid number, the script prints ‘abc is a valid number’. Otherwise, it prints ‘abc is not a valid number’.
Unexpected Results Due to Incorrect Operator Use
Another common issue is getting unexpected results due to incorrect use of comparison operators. For example, using the -gt
operator when you meant to use -ge
can lead to different outcomes.
Always ensure you’re using the correct operator for your intended comparison. If you’re unsure, refer back to the operator definitions or use test cases to verify your script’s behavior.
By being aware of these potential issues and knowing how to handle them, you can write more robust Bash scripts that handle number comparisons accurately and gracefully.
Understanding Bash Scripting and Relational Operators
Before we delve further into number comparison in Bash, it’s crucial to understand the basics of Bash scripting and the concept of relational operators.
Bash Scripting Basics
Bash (Bourne Again Shell) is a popular command-line interface (CLI) used in Unix-based systems, including Linux and macOS. It allows users to interact with the system by typing commands into the terminal.
Bash scripting takes this a step further, allowing you to write a series of commands in a file (known as a script) and execute them all at once. This is incredibly useful for automating tasks, running scheduled jobs, and much more.
The Role of Relational Operators
Relational operators are an essential part of Bash scripting. They allow you to compare values and make decisions based on that comparison. As we’ve seen, Bash includes several relational operators for number comparison, like -eq
, -ne
, -gt
, -lt
, -ge
, and -le
.
Here’s a quick refresher example:
num1=10
num2=20
if [ "$num1" -lt "$num2" ]; then
echo "$num1 is less than $num2"
else
echo "$num1 is not less than $num2"
fi
# Output:
# '10 is less than 20'
In this script, we’re using the -lt
operator to check if num1
is less than num2
. The if
statement evaluates the condition and prints the appropriate message based on the result.
Why Number Comparison Matters
Number comparison is a fundamental concept in scripting and programming. It’s crucial for conditional execution, where different code blocks are executed based on the outcome of a comparison. It’s also essential for sorting and arranging data, among other tasks.
Mastering number comparison in Bash will make your scripts more dynamic and versatile, allowing them to handle a wider range of tasks and scenarios.
The Bigger Picture: Number Comparison in Larger Scripts
Number comparison in Bash is not just a standalone concept. It plays a significant role in larger scripts and projects, often forming the backbone of decision-making processes.
Number Comparison in Larger Scripts
In a larger Bash script, number comparison can be used to control the flow of execution. For example, you might use number comparison to decide which parts of your script to run, or to repeat certain actions a specific number of times.
for i in {1..5}
do
if [ "$i" -eq 3 ]; then
echo "Halfway there!"
fi
echo "Processing item $i"
done
# Output:
# Processing item 1
# Processing item 2
# Halfway there!
# Processing item 3
# Processing item 4
# Processing item 5
In this script, we’re using a for
loop to process a series of items. We use a number comparison to check when we’re halfway through the loop and print a special message.
Expanding Your Knowledge: Related Concepts
Mastering number comparison in Bash opens the door to understanding more complex scripting concepts. You might find it useful to explore related concepts like arithmetic operations in Bash, conditional statements, loops, and more. Each of these topics ties in closely with number comparison, and understanding them will make your Bash scripts more powerful and flexible.
Further Resources for Bash Scripting Mastery
Ready to take your Bash scripting skills to the next level? Here are some resources that can help you deepen your understanding:
- Advanced Bash-Scripting Guide: An in-depth exploration of Bash scripting, including number comparison and much more.
- Bash Academy: An interactive platform to learn Bash scripting from scratch.
- Bash Scripting Tutorial: A comprehensive tutorial covering all aspects of Bash scripting, including number comparison.
Wrapping Up: Mastering Number Comparison in Bash
In this comprehensive guide, we’ve navigated through the process of comparing numbers in Bash, shedding light on the usage of relational operators, common issues, and their solutions. We’ve also explored alternative approaches to handle number comparison, broadening our understanding of this essential aspect of Bash scripting.
We began with the basics, learning how to use relational operators like -eq
, -ne
, -gt
, -lt
, -ge
, and -le
to compare numbers in Bash. We then delved into more complex comparisons, exploring how to compare multiple numbers and handle floating-point numbers. Along the way, we tackled common challenges like dealing with non-numeric input and unexpected results due to incorrect operator use, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to number comparison, introducing you to the ‘expr’ command and ‘awk’ for comparing numbers in Bash. These methods offer more flexibility than Bash’s built-in operators, allowing you to handle more complex scenarios and data types.
Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Data Type Support |
---|---|---|---|
Bash’s built-in operators | Moderate | Low | Integer |
‘expr’ command | High | Moderate | Integer |
‘awk’ | High | High | Floating-point |
Whether you’re just starting out with Bash scripting or looking to level up your skills, we hope this guide has given you a deeper understanding of number comparison in Bash and its importance in scripting.
With its balance of simplicity and power, Bash scripting is a versatile tool for automating tasks, processing data, and much more. Now, you’re well-equipped to handle number comparison in Bash, a key skill that will undoubtedly come in handy in your scripting journey. Happy scripting!