Java ‘Protected’ Keyword | Balanced Encapsulation Guide
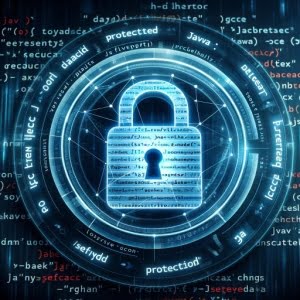
Ever found yourself puzzled by the ‘protected’ keyword in Java? You’re not alone. Many developers find themselves in a maze when it comes to using ‘protected’ in Java. Think of the ‘protected’ keyword as a security guard – it’s a tool that helps control access to your code, ensuring only authorized parts can interact with it.
The ‘protected’ keyword is a powerful way to manage access in your Java programs, making it an essential tool for any Java developer’s toolkit.
In this guide, we’ll walk you through the process of using the ‘protected’ keyword in Java, from the basics to more advanced techniques. We’ll cover everything from defining and using ‘protected’ variables and methods, to understanding how ‘protected’ interacts with Java’s inheritance model, and even troubleshooting common issues.
So, let’s dive in and start mastering the ‘protected’ keyword in Java!
TL;DR: What is the ‘protected’ keyword in Java?
In Java, ‘protected’ is an access modifier called before data type and variable:
protected int myVariable
that allows visibility to subclasses and classes within the same package. It’s a powerful tool that helps manage access in your Java programs. Here’s a simple example:
protected int myVariable;
In this example, myVariable
is a protected integer variable. It can be accessed within its own class, by any class in the same package, and by subclasses of its class in any package.
This is just a basic use of the ‘protected’ keyword in Java. There’s much more to learn about managing access control in Java. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Unveiling the ‘Protected’ Keyword in Java
- Delving Deeper: ‘Protected’ and Inheritance
- Exploring Other Access Modifiers in Java
- Overcoming Challenges with ‘Protected’ in Java
- Java Access Control: The Basics
- The ‘Protected’ Keyword in Large-Scale Java Projects
- Wrapping Up: Mastering the ‘Protected’ Keyword in Java
Unveiling the ‘Protected’ Keyword in Java
At its core, ‘protected’ is an access modifier in Java. Access modifiers dictate which other classes in your Java program can access a particular variable or method. ‘Protected’ is one of four access modifiers provided by Java, and it strikes a balance between the restrictive ‘private’ and the open ‘public’.
Understanding the ‘Protected’ Keyword
When you declare a variable or method as ‘protected’ in a class, it becomes accessible within the same class, any class within the same package, and any subclass, irrespective of the package it belongs to. This is what sets ‘protected’ apart from the other access modifiers.
Let’s look at a basic example:
// Defining a class named Vehicle
public class Vehicle {
// Declaring a protected variable
protected String brand;
}
// Defining a subclass named Car
public class Car extends Vehicle {
// Accessing the protected variable from the superclass
void display() {
brand = "Tesla";
System.out.println("Brand: " + brand);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.display();
}
}
/* Output:
Brand: Tesla
*/
In this example, brand
is a protected string variable declared in the Vehicle
class. This variable is then accessed directly within the Car
class, which is a subclass of Vehicle
. The display()
method in the Car
class assigns the value ‘Tesla’ to the brand
variable and prints it. The output of this program is ‘Brand: Tesla’.
Advantages and Potential Pitfalls
The ‘protected’ keyword offers a higher degree of encapsulation compared to the ‘public’ keyword, as it restricts access to the class itself, its package, and its subclasses. This can be beneficial in maintaining the integrity of your code.
However, it’s essential to note that overusing ‘protected’ can lead to a higher coupling between classes, which could make your code harder to maintain in the long run. Therefore, it’s crucial to use ‘protected’ judiciously and only when necessary.
Delving Deeper: ‘Protected’ and Inheritance
The ‘protected’ keyword shines when used with Java’s inheritance concept. Let’s dive deeper into this scenario.
‘Protected’ in Inheritance
In Java, a subclass can access the ‘protected’ members of its superclass, even if it’s in a different package. This is a unique feature of the ‘protected’ keyword, as neither ‘private’ nor ‘package-private’ (default) access modifiers allow this cross-package access.
Here’s an example to illustrate this point:
// In file Vehicle.java
package com.example.vehicle;
public class Vehicle {
protected String brand = "Default Brand";
}
// In file Car.java
package com.example.car;
import com.example.vehicle.Vehicle;
public class Car extends Vehicle {
void displayBrand() {
System.out.println("Brand: " + brand);
}
}
// In file Main.java
package com.example.main;
import com.example.car.Car;
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.displayBrand();
}
}
/* Output:
Brand: Default Brand
*/
In this example, we have three classes – Vehicle
, Car
, and Main
– each in a different package. The Vehicle
class has a protected member brand
. The Car
class, which is a subclass of Vehicle
, can access this protected member directly, even though it’s in a different package. The Main
class creates an object of Car
and calls the displayBrand()
method, which prints the value of brand
.
‘Protected’ and Interfaces
It’s important to note that you cannot use the ‘protected’ keyword with interface methods in Java. Interface methods are implicitly ‘public’, and trying to declare them as ‘protected’ will result in a compilation error.
As you can see, the ‘protected’ keyword in Java is a powerful tool for managing access control, especially in more complex scenarios involving inheritance across different packages. However, it’s essential to use it judiciously to maintain the readability and maintainability of your code.
Exploring Other Access Modifiers in Java
While ‘protected’ is a powerful access modifier, Java offers other access modifiers that can be more suitable depending on your specific needs. These include ‘public’, ‘private’, and ‘default’ (package-private).
Embracing ‘Public’ Access
The ‘public’ access modifier is the most permissive. When a variable or method is declared as ‘public’, it can be accessed from any other class in the program, regardless of the package the class belongs to.
Here’s an example:
public class Vehicle {
public String brand = "Default Brand";
}
In this case, the brand
variable can be accessed from any class in any package.
Going ‘Private’
On the other end of the spectrum, the ‘private’ access modifier is the most restrictive. A ‘private’ variable or method can only be accessed within the class it’s declared in.
Here’s an example:
public class Vehicle {
private String brand = "Default Brand";
}
In this case, the brand
variable can only be accessed within the Vehicle
class.
Understanding ‘Default’ (Package-Private) Access
If no access modifier is specified, the variable or method is given ‘default’ (also known as package-private) access. This means it can be accessed from any class within the same package.
Here’s an example:
public class Vehicle {
String brand = "Default Brand";
}
In this case, the brand
variable can be accessed from any class within the same package as the Vehicle
class.
Making the Right Choice
Choosing the right access modifier depends on the specific needs of your program. If you want to restrict access to a variable or method as much as possible, ‘private’ is the way to go. If you want to allow access from subclasses or classes within the same package, ‘protected’ is a good choice. If you want to allow access from any class in the same package, consider using ‘default’. And if you want to allow unrestricted access, ‘public’ is the best choice.
Remember, the key to choosing the right access modifier is understanding the needs of your program and the relationships between your classes.
Overcoming Challenges with ‘Protected’ in Java
While the ‘protected’ keyword can be a useful tool, it can also lead to some common pitfalls and errors if not used correctly. Let’s explore some of these challenges and how to overcome them.
Accessing ‘Protected’ from Non-Subclasses
One common mistake is trying to access a ‘protected’ member from a non-subclass in a different package. This will result in a compile-time error.
Here’s an example that demonstrates this error:
// In file Vehicle.java
package com.example.vehicle;
public class Vehicle {
protected String brand = "Default Brand";
}
// In file Main.java
package com.example.main;
import com.example.vehicle.Vehicle;
public class Main {
public static void main(String[] args) {
Vehicle myVehicle = new Vehicle();
System.out.println(myVehicle.brand); // Error: brand has protected access in Vehicle
}
}
In this case, the Main
class is trying to access the brand
variable of the Vehicle
class directly. However, since brand
is ‘protected’ and Main
is not a subclass of Vehicle
, this results in a compile-time error.
‘Protected’ and Interface Methods
As mentioned earlier, ‘protected’ cannot be used with interface methods. Attempting to do so will result in a compile-time error.
Here’s an example that demonstrates this error:
public interface MyInterface {
protected void myMethod(); // Error: Modifier 'protected' not allowed here
}
In this case, we’re trying to declare the myMethod
method in the MyInterface
interface as ‘protected’. However, this is not allowed and results in a compile-time error.
Best Practices and Optimization
When using ‘protected’, it’s important to follow best practices to maintain the readability and maintainability of your code. Here are some tips:
- Use ‘protected’ sparingly: Overuse of ‘protected’ can lead to high coupling between classes, making your code harder to maintain. Use it only when necessary.
Favor composition over inheritance: If you find yourself using ‘protected’ frequently, it might be a sign that you’re relying too heavily on inheritance. Consider using composition instead, as it can lead to more flexible and maintainable code.
Understand your access needs: Before deciding to use ‘protected’, make sure to understand the access needs of your program. If you only need to allow access within the same class, use ‘private’. If you need to allow access within the same package, use ‘default’. And if you need to allow unrestricted access, use ‘public’.
Java Access Control: The Basics
Java’s access control mechanism is a fundamental part of its object-oriented design. It allows developers to control the visibility and accessibility of classes, variables, and methods, thereby promoting encapsulation and maintaining the integrity of the code.
Understanding Packages, Classes, and Subclasses
In Java, a package is a namespace that organizes a set of related classes and interfaces. It’s a way to group related classes together, which can be useful in large applications with hundreds or thousands of classes.
A class, on the other hand, is a blueprint for creating objects. It defines a set of variables and methods that the objects created from that class will have.
A subclass is a class that inherits from another class, known as the superclass. The subclass can inherit the variables and methods of the superclass, and it can also define its own.
The Role of the ‘Protected’ Keyword
In this context, the ‘protected’ keyword plays a crucial role in managing access control. When a variable or method is declared as ‘protected’, it can be accessed within its own class, any class within the same package, and any subclass, regardless of the package it belongs to.
This is demonstrated in the following example:
// In file Vehicle.java
package com.example.vehicle;
public class Vehicle {
protected String brand = "Default Brand";
}
// In file Car.java
package com.example.car;
import com.example.vehicle.Vehicle;
public class Car extends Vehicle {
void displayBrand() {
System.out.println("Brand: " + brand);
}
}
// In file Main.java
package com.example.main;
import com.example.car.Car;
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.displayBrand();
}
}
/* Output:
Brand: Default Brand
*/
In this example, the Car
class, which is a subclass of Vehicle
, can access the brand
variable of the Vehicle
class, even though they’re in different packages. This is the power of the ‘protected’ keyword in Java.
The ‘Protected’ Keyword in Large-Scale Java Projects
As your Java projects grow in size and complexity, the ‘protected’ keyword becomes increasingly important. It provides a way to manage access control at a granular level, ensuring that your code’s integrity is maintained even as your codebase expands.
Encapsulating with ‘Protected’
In large-scale projects, encapsulation – the principle of hiding the internal details of how an object works – becomes crucial. The ‘protected’ keyword plays a key role in this. It allows you to hide certain details of a class from the outside world, while still making them accessible to subclasses and classes within the same package.
Abstracting with ‘Protected’
‘Protected’ also plays a role in abstraction, another key object-oriented programming concept. By allowing subclasses to access certain variables and methods, ‘protected’ enables you to define more abstract classes that can be extended to create more specific subclasses.
Further Resources for Mastering ‘Protected’ in Java
To deepen your understanding of the ‘protected’ keyword and related concepts, consider exploring these resources:
- Java Variables Demystified: Comprehensive Guide – Dive into variables in Java for effective coding.
Mastering Access Control in Java – Learn how to use access modifiers like public, private, and protected in Java
Variable Declaration with var in Java – Explore the versatility of the var keyword in Java for enhancing code readability.
Oracle’s Java Tutorials offer a wealth of information on all aspects of Java access control and the ‘protected’ keyword.
GeeksforGeeks Java Programming Language covers many aspects of Java access modifiers, and the’protected’ keyword.
Java Code Geeks offers a wide range of tutorials and articles on the Java’protected’ keyword.
Remember, mastering the ‘protected’ keyword in Java is a journey. Keep learning, keep practicing, and don’t be afraid to dive deep!
Wrapping Up: Mastering the ‘Protected’ Keyword in Java
In this comprehensive guide, we’ve delved deep into the world of the ‘protected’ keyword in Java, a powerful tool for managing access control in your programs.
We started with the basics, explaining what the ‘protected’ keyword is and how it works. We then moved on to more advanced topics, such as using ‘protected’ in inheritance and the limitations of ‘protected’ with interface methods. Along the way, we’ve provided numerous code examples to help you understand these concepts better.
We’ve also discussed common pitfalls and errors when using ‘protected’, and provided solutions to help you overcome these challenges. And we’ve explored alternative approaches to handle access control in Java, comparing the ‘protected’ keyword with other access modifiers like ‘public’, ‘private’, and ‘default’.
Here’s a quick comparison of these access modifiers:
Access Modifier | Accessibility | Use Case |
---|---|---|
Protected | Within the same class, any class within the same package, and any subclass | When you want to restrict access but still allow access to subclasses and classes within the same package |
Public | Any class, regardless of the package | When you want to allow unrestricted access |
Private | Within the same class | When you want to restrict access as much as possible |
Default (Package-Private) | Any class within the same package | When you want to allow access within the same package but restrict access from outside the package |
Whether you’re a beginner just starting out with Java or an experienced developer looking to deepen your understanding of access control, we hope this guide has helped you master the ‘protected’ keyword in Java.
With its balance of accessibility and restriction, ‘protected’ is a powerful tool for managing access control in Java. Happy coding!