Java Access Modifiers: A Concise Guide
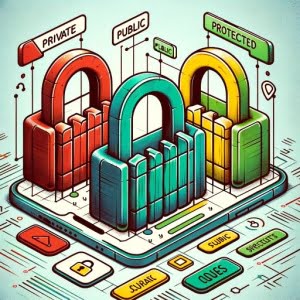
Are you finding it difficult to understand access modifiers in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling access modifiers in Java, but we’re here to help.
Think of access modifiers as the gatekeepers of your code – they control who can access what in your Java classes, methods, and variables. Access modifiers are a fundamental part of Java, and understanding them can make your coding journey smoother and more efficient.
In this guide, we’ll walk you through the different types of access modifiers in Java, their usage, and importance. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
Let’s get started and master access modifiers in Java!
TL;DR: What Are Access Modifiers in Java?
Access modifiers in Java are keywords that set the accessibility of classes, methods, and variables. The four types of access modifiers are:
public, private, protected, and default
. They determine the visibility and accessibility of these elements in your code.
Here’s a simple example:
public class MyClass { }
In this example, MyClass
is a public class, which means it’s accessible from any other class in your application.
This is just the tip of the iceberg when it comes to understanding and using access modifiers in Java. Continue reading for a more detailed explanation, practical examples, and advanced usage scenarios.
Table of Contents
- Understanding Basic Use of Access Modifiers in Java
- Advanced Usage of Java Access Modifiers
- Exploring Alternative Approaches to Access Control
- Troubleshooting Common Issues with Java Access Modifiers
- Access Control in Object-Oriented Programming
- Access Modifiers in Larger Java Projects
- Access Modifiers and Design Principles
- Wrapping Up: Mastering Access Modifiers in Java
Understanding Basic Use of Access Modifiers in Java
Access modifiers in Java are used to set the visibility and accessibility of classes, methods, and variables. There are four types of access modifiers:
- Public
- Private
- Protected
- Default (no keyword)
Let’s delve into each of these access modifiers.
Public Access Modifier
The public
keyword allows a class, method, or variable to be accessible from any other class in your application. Here’s an example:
public class PublicClass {
public int publicVariable = 1;
public void publicMethod() {
System.out.println('This is a public method.');
}
}
# Output:
# This is a public method.
In this example, PublicClass
, publicVariable
, and publicMethod
are all accessible from any other class in your application.
Private Access Modifier
The private
keyword restricts the accessibility of a class, method, or variable to its own class. Here’s an example:
public class PrivateClass {
private int privateVariable = 1;
private void privateMethod() {
System.out.println('This is a private method.');
}
}
# Output:
# This is a private method.
In this example, privateVariable
and privateMethod
are only accessible within PrivateClass
.
Protected Access Modifier
The protected
keyword allows a class, method, or variable to be accessible within its own package and by subclasses in other packages. Here’s an example:
public class ProtectedClass {
protected int protectedVariable = 1;
protected void protectedMethod() {
System.out.println('This is a protected method.');
}
}
# Output:
# This is a protected method.
In this example, protectedVariable
and protectedMethod
are accessible within ProtectedClass
and any subclasses.
Default Access Modifier
In Java, if you do not specify an access modifier, it defaults to package-private, which allows a class, method, or variable to be accessible within its own package. Here’s an example:
class DefaultClass {
int defaultVariable = 1;
void defaultMethod() {
System.out.println('This is a default method.');
}
}
# Output:
# This is a default method.
In this example, defaultVariable
and defaultMethod
are accessible within DefaultClass
and any other classes in the same package.
Understanding these access modifiers and their scope is crucial in Java programming as it controls the visibility and accessibility of your code components, ensuring data integrity and security.
Advanced Usage of Java Access Modifiers
Once you’ve grasped the basic use of access modifiers in Java, it’s time to explore their advanced usage. This involves understanding how access modifiers interact with classes, methods, and variables in different scenarios, and the best practices for using them.
Access Modifiers with Classes
In Java, when it comes to classes, only two access modifiers are applicable: public and default (no keyword). A public
class is accessible to all other classes, while a default class is only accessible to classes within the same package.
public class PublicClass {
// This class is accessible everywhere
}
class DefaultClass {
// This class is accessible only within the same package
}
Access Modifiers with Methods and Variables
All four access modifiers can be used with methods and variables. The choice of modifier depends on the level of access you want to provide. Here’s an example illustrating different scenarios:
public class TestClass {
public int publicVar = 1;
private int privateVar = 2;
protected int protectedVar = 3;
int defaultVar = 4; //default access modifier
public void publicMethod() {
System.out.println('Public Method');
}
private void privateMethod() {
System.out.println('Private Method');
}
protected void protectedMethod() {
System.out.println('Protected Method');
}
void defaultMethod() { //default access modifier
System.out.println('Default Method');
}
}
# Output:
# Public Method
# Private Method
# Protected Method
# Default Method
In this example, publicVar
and publicMethod
are accessible everywhere, privateVar
and privateMethod
are only accessible within TestClass
, protectedVar
and protectedMethod
are accessible within TestClass
and any subclasses, and defaultVar
and defaultMethod
are accessible within TestClass
and any other classes in the same package.
Best Practices for Using Access Modifiers
When using access modifiers, it’s important to adhere to the principle of least privilege. This means you should only provide the minimum level of access necessary for a class, method, or variable to function. This helps maintain data integrity and security in your code.
For instance, if a variable or method should not be directly accessible outside its class, make it private
. If it needs to be accessible to subclasses, make it protected
. If it needs to be accessible everywhere, make it public
. If it only needs to be accessible within the same package, leave it as default.
By understanding and applying these advanced uses and best practices, you’ll be able to use access modifiers effectively in your Java programming.
Exploring Alternative Approaches to Access Control
While access modifiers are the primary way to control access in Java, there are other techniques that you can use to achieve similar outcomes. These include using interfaces and encapsulation.
Using Interfaces for Access Control
Interfaces in Java can be used as a means of access control. By defining an interface, you can control what methods a class must implement. This ensures that certain methods are always accessible for classes implementing that interface.
Here’s an example of using an interface for access control:
public interface Accessible {
void accessibleMethod();
}
public class TestClass implements Accessible {
public void accessibleMethod() {
System.out.println('This method is accessible because of the interface.');
}
}
# Output:
# This method is accessible because of the interface.
In this example, any class that implements the Accessible
interface must implement the accessibleMethod()
. This ensures that accessibleMethod()
is always accessible for TestClass
.
Encapsulation for Access Control
Encapsulation is another technique for access control in Java. It involves wrapping data (variables) and code (methods) together as a single unit and controlling access to the data by using access modifiers.
Here’s an example of using encapsulation for access control:
public class EncapsulatedClass {
private int privateVar = 1;
public int getPrivateVar() {
return privateVar;
}
public void setPrivateVar(int privateVar) {
this.privateVar = privateVar;
}
}
# Output:
# No output
In this example, the privateVar
variable is private and can only be accessed and modified through the getPrivateVar()
and setPrivateVar()
methods. This is a form of access control as it restricts direct access to the privateVar
variable.
While access modifiers are the standard way to control access in Java, using interfaces and encapsulation can offer more flexibility and control in certain scenarios. Understanding these alternative approaches and when to use them can enhance your Java programming skills.
Troubleshooting Common Issues with Java Access Modifiers
While access modifiers in Java are powerful tools for controlling access to classes, methods, and variables, they can sometimes lead to issues, particularly in terms of visibility and inheritance. Let’s discuss some of these common problems and their solutions.
Visibility Issues
One of the most common issues related to access modifiers in Java is visibility issues. This typically happens when you’re trying to access a private or default member from outside its class or package.
Here’s an example of a visibility issue:
public class TestClass {
private int privateVar = 1;
}
public class AnotherClass {
TestClass tc = new TestClass();
int testVar = tc.privateVar; // This will cause a compile error
}
# Output:
# Error: privateVar has private access in TestClass
In this example, trying to access privateVar
from AnotherClass
causes a compile error because privateVar
is private and can only be accessed within TestClass
.
The solution to this issue is to use the appropriate access modifier based on the level of access you want to provide. If privateVar
needs to be accessed from AnotherClass
, it should be declared as public
or protected
, or you should provide a public getter method in TestClass
to access it.
Inheritance Problems
Another common issue related to access modifiers in Java is inheritance problems. This happens when a subclass tries to access a superclass’s private members.
Here’s an example of an inheritance problem:
public class SuperClass {
private int privateVar = 1;
}
public class SubClass extends SuperClass {
int testVar = privateVar; // This will cause a compile error
}
# Output:
# Error: privateVar has private access in SuperClass
In this example, trying to access privateVar
from SubClass
causes a compile error because privateVar
is private and can only be accessed within SuperClass
.
The solution to this issue is similar to the visibility issue. If privateVar
needs to be accessed from SubClass
, it should be declared as protected
in SuperClass
, or you should provide a public or protected getter method in SuperClass
to access it.
Understanding these common issues and their solutions can help you avoid potential pitfalls when using access modifiers in Java, making your coding process smoother and more efficient.
Access Control in Object-Oriented Programming
Access control is a fundamental concept in object-oriented programming (OOP). It’s the mechanism that regulates access to the components of an object, such as methods and variables. In Java, this is achieved through access modifiers.
public class ExampleClass {
private int privateVar = 1;
public int publicVar = 2;
protected int protectedVar = 3;
int defaultVar = 4; // default access modifier
}
# Output:
# No output
In this example, ExampleClass
has four variables, each with a different access modifier. The privateVar
can only be accessed within ExampleClass
, publicVar
can be accessed from any class, protectedVar
can be accessed within ExampleClass
and its subclasses, and defaultVar
can be accessed within ExampleClass
and any class in the same package.
Importance of Access Control in Java
Access control in Java is crucial for several reasons:
- Encapsulation: Access control is a key aspect of encapsulation in OOP. It allows the internal representation of an object to be hidden from the outside world, and only a prescribed set of methods and variables can be accessed.
Data Integrity: By restricting access, you can prevent the data from being modified in unexpected ways. This helps to maintain the integrity of your data.
Security: Access control can prevent sensitive data from being exposed. For instance, a private variable in a class can only be accessed within that class, preventing unauthorized access.
Maintainability: Access control makes code easier to maintain. By limiting the parts of the code that can access certain components, you reduce the risk of introducing bugs when modifying the code.
Understanding the concept of access control and its importance in Java is crucial for effective and secure programming. It allows you to structure your code in a way that maximizes security, maintainability, and data integrity.
Access Modifiers in Larger Java Projects
Access modifiers play an integral role in larger Java projects. They help maintain a clean and manageable codebase and ensure that each part of the code interacts with the others in a controlled and predictable way.
For instance, by making a variable private
, you’re ensuring that it can only be accessed within its own class. This reduces the risk of it being accidentally modified from elsewhere in the code, which can be particularly valuable in a large project with multiple developers.
Access Modifiers and Design Principles
Access modifiers in Java are also closely tied to key design principles like encapsulation and abstraction.
Encapsulation is the concept of bundling the data and methods that operate on the data within one unit and hiding the values or state of an object inside the class. It’s achieved in Java using access modifiers.
Abstraction, on the other hand, is the concept of hiding the complexity of code by providing a simple interface. In Java, public
and protected
access modifiers are often used to expose the necessary methods while keeping the implementation details hidden.
Further Resources for Mastering Java Access Modifiers
For a Complete Guide on Variables in Java, Click Here for a full tutorial.
For those looking to delve deeper into the world of access modifiers in Java, here are some valuable resources:
- Java Protected Access Modifier – Delve into the Java protected access modifier for controlling access to class members.
Java Final Keyword Explained – Learn about the final keyword in Java for defining constants and immutable variables.
Oracle’s Java Tutorials offer a comprehensive guide to understanding and using access modifiers in Java.
GeeksforGeeks provides a detailed article about access modifiers along with examples and use cases.
Baeldung is another great resource, offering an in-depth exploration of access modifiers in Java.
By understanding the role of access modifiers in larger Java projects and in design principles like encapsulation and abstraction, and by leveraging the resources provided, you can become proficient in using access modifiers in Java.
Wrapping Up: Mastering Access Modifiers in Java
In this comprehensive guide, we’ve delved into the world of access modifiers in Java, exploring their importance, usage, and the common issues you might encounter.
We began with the basics, understanding what access modifiers are and their basic usage in Java. We then delved deeper, exploring advanced usage scenarios and the best practices for using access modifiers. We also discussed alternative approaches to access control in Java, such as using interfaces and encapsulation.
Along the way, we tackled common issues that you might face when using access modifiers, such as visibility and inheritance problems, and provided solutions and workarounds for each issue. We also explored the concept of access control in object-oriented programming and its importance in Java.
Here’s a quick comparison of the access modifiers we’ve discussed:
Access Modifier | Scope | Use Case |
---|---|---|
Public | Everywhere | When a class, method, or variable needs to be accessible from any other class |
Private | Within its own class | When a class, method, or variable should not be directly accessible outside its class |
Protected | Within its own package and by subclasses in other packages | When a class, method, or variable needs to be accessible to subclasses |
Default (no keyword) | Within its own package | When a class, method, or variable needs to be accessible within the same package |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of access modifiers in Java and their crucial role in access control.
Understanding and effectively using access modifiers is a fundamental skill in Java programming. It allows you to structure your code in a way that maximizes security, maintainability, and data integrity. Happy coding!