Using ‘If Else’ Statements in Bash | Scripting Syntax Guide
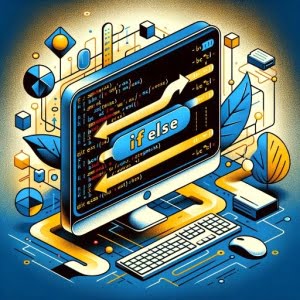
Are you finding it challenging to work with if-else statements in Bash? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of Bash if-else statements as a traffic cop – controlling the flow of your script, making decisions based on conditions. These statements are a powerful way to control the execution of your Bash scripts, making them extremely popular for conditional scripting.
This guide will walk you through the ins and outs of using if-else statements in Bash, from the basics to more advanced techniques. We’ll cover everything from writing simple if-else statements, handling different types of conditions, to dealing with nested if-else statements and even troubleshooting common issues.
So, let’s dive in and start mastering if-else statements in Bash!
TL;DR: How Do I Use If-Else in Bash?
The basic syntax for if-else in Bash is as follows:
if [ condition ]; then # code if condition is true else # code if condition is false fi
. This structure allows your script to make decisions based on conditions.
Here’s a simple example:
#!/bin/bash
num=10
if [ $num -gt 5 ]; then
echo 'The number is greater than 5'
else
echo 'The number is not greater than 5'
fi
# Output:
# 'The number is greater than 5'
In this example, we have a variable num
set to 10. The if-else statement checks if num
is greater than 5. If it is, it prints ‘The number is greater than 5’. If not, it would print ‘The number is not greater than 5’. In this case, since 10 is greater than 5, the output is ‘The number is greater than 5’.
This is a basic way to use if-else in Bash, but there’s much more to learn about controlling script flow with conditional statements. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Bash If Else: Basic Usage
- Advanced Bash If-Else: Nested Statements and Logical Operators
- Alternative Approaches to Control Script Flow in Bash
- Troubleshooting Common Issues with Bash If-Else
- Understanding Bash Scripting and Conditional Statements
- Exploring If-Else in Larger Bash Projects
- Wrapping Up: Mastering If-Else Statements in Bash
Bash If Else: Basic Usage
Understanding the basic use of if-else statements in Bash is the first step to mastering this powerful tool. The if-else statement in Bash is a form of conditional statement that allows you to make decisions in your script based on the outcome of a condition.
Let’s start with a simple example to illustrate how this works:
#!/bin/bash
read -p 'Enter your name: ' name
if [ -z $name ]; then
echo 'You did not enter your name.'
else
echo "Hello, $name!"
fi
# Output:
# If you enter 'Alice', the output will be 'Hello, Alice!'
# If you don't enter anything, the output will be 'You did not enter your name.'
In this script, we first use the read
command to prompt the user to enter their name. The entered name is stored in the variable name
. The if-else statement then checks if the name
variable is empty using the -z
test operator. If the name
variable is empty (i.e., the user did not enter anything), it echoes ‘You did not enter your name.’ If the name
variable is not empty, it echoes ‘Hello, [entered name]!’
This basic use of if-else in Bash is a simple yet effective way to control the flow of your script based on user input. However, it’s essential to remember that Bash is very sensitive to syntax. For example, forgetting to close the if-else statement with fi
can lead to unexpected behavior or errors. Therefore, always check your syntax when working with if-else statements in Bash.
Advanced Bash If-Else: Nested Statements and Logical Operators
Once you’ve mastered the basics of if-else statements in Bash, you can start exploring more complex uses. Two such advanced techniques involve nested if-else statements and the use of logical operators.
Nested If-Else Statements
Nested if-else statements allow you to check multiple conditions within your script. Here’s an example:
#!/bin/bash
read -p 'Enter your age: ' age
if [ $age -ge 18 ]; then
echo 'You are an adult.'
if [ $age -ge 65 ]; then
echo 'You are also a senior citizen.'
fi
else
echo 'You are a minor.'
fi
# Output:
# If you enter '70', the output will be 'You are an adult.' followed by 'You are also a senior citizen.'
# If you enter '30', the output will be 'You are an adult.'
# If you enter '10', the output will be 'You are a minor.'
In this script, we first check if the entered age is 18 or above. If it is, we print ‘You are an adult.’ and then check if the age is 65 or above. If it is, we print ‘You are also a senior citizen.’ If the entered age is less than 18, we print ‘You are a minor.’
Using Logical Operators
Logical operators like &&
(AND) and ||
(OR) can be used in conjunction with if-else statements to add more complexity to the conditions you’re checking. Here’s an example:
#!/bin/bash
read -p 'Enter a number: ' num
if [ $num -gt 10 ] && [ $num -lt 20 ]; then
echo 'The number is between 10 and 20.'
else
echo 'The number is not between 10 and 20.'
fi
# Output:
# If you enter '15', the output will be 'The number is between 10 and 20.'
# If you enter '25', the output will be 'The number is not between 10 and 20.'
In this script, we use the &&
operator to check if the entered number is greater than 10 AND less than 20. If it is, we print ‘The number is between 10 and 20.’ If not, we print ‘The number is not between 10 and 20.’
These advanced techniques allow you to create more complex and powerful scripts with Bash if-else statements. However, they also require a deeper understanding of Bash syntax and logic, so it’s essential to practice and experiment with these techniques to fully understand how they work.
Alternative Approaches to Control Script Flow in Bash
While if-else statements are a powerful tool for controlling script flow in Bash, they are not the only tool available. There are other methods you can use, such as ‘case’ statements and ‘select’ loops. Let’s explore these alternatives and see how they can be utilized.
Using Case Statements
Case statements in Bash are a type of conditional statement that allow you to compare a value against several patterns and execute code based on which pattern matches. Here’s an example:
#!/bin/bash
read -p 'Enter a day of the week: ' day
case $day in
'Monday')
echo 'The start of the work week.'
;;
'Friday')
echo 'The end of the work week.'
;;
'Saturday' | 'Sunday')
echo 'The weekend!'
;;
*)
echo 'A regular work day.'
;;
esac
# Output:
# If you enter 'Monday', the output will be 'The start of the work week.'
# If you enter 'Friday', the output will be 'The end of the work week.'
# If you enter 'Saturday' or 'Sunday', the output will be 'The weekend!'
# If you enter any other day, the output will be 'A regular work day.'
In this script, the case statement checks the entered day against several patterns. If the day matches a pattern, it executes the corresponding code. The *)
pattern serves as a catch-all for any value that doesn’t match the other patterns.
Using Select Loops
Select loops in Bash provide a simple way to create menus. Here’s an example:
#!/bin/bash
PS3='Please enter your choice: '
select option in "Option 1" "Option 2" "Quit"
do
case $option in
"Option 1")
echo 'You chose Option 1.'
;;
"Option 2")
echo 'You chose Option 2.'
;;
"Quit")
break
;;
*)
echo 'Invalid option.'
;;
esac
done
# Output:
# The script will present a menu with three options: 'Option 1', 'Option 2', and 'Quit'.
# If you enter '1', the output will be 'You chose Option 1.'
# If you enter '2', the output will be 'You chose Option 2.'
# If you enter '3', the script will quit.
# If you enter any other number, the output will be 'Invalid option.'
In this script, the select loop presents a menu to the user with three options. The user can enter the number of the option they want to choose. The case statement then checks the chosen option against several patterns and executes the corresponding code.
These alternative methods for controlling script flow in Bash can be more suitable than if-else statements in certain situations. However, they also require a deeper understanding of Bash syntax and logic. Therefore, it’s vital to practice and experiment with these methods to fully understand how they work and when to use them.
Troubleshooting Common Issues with Bash If-Else
As powerful as Bash if-else statements are, they can sometimes lead to unexpected behaviors or syntax errors. This section aims to discuss some of these common issues and provide solutions and workarounds.
Syntax Errors
One of the most common issues when dealing with if-else statements in Bash is syntax errors. Bash is very particular about its syntax, and even a small mistake can lead to errors. For example:
#!/bin/bash
read -p 'Enter a number: ' num
if [ $num -gt 10 ]
echo 'The number is greater than 10.'
else
echo 'The number is not greater than 10.'
fi
# Output:
# You will encounter a syntax error because the 'then' keyword is missing after the condition.
To avoid syntax errors, always double-check your code and ensure that the ‘then’ keyword is present after the condition, and the if-else statement is closed with ‘fi’.
Unexpected Behavior
Sometimes, your if-else statement might not behave as expected. This is often due to incorrect conditions or logic within the if-else statement. For instance, if you use the -lt
(less than) operator instead of -gt
(greater than), you might get unexpected results.
#!/bin/bash
read -p 'Enter a number: ' num
if [ $num -lt 10 ]; then
echo 'The number is greater than 10.'
else
echo 'The number is not greater than 10.'
fi
# Output:
# If you enter '15', the output will be 'The number is not greater than 10.' which is not correct.
In this script, the condition checks if the number is less than 10, but the echo statement says ‘The number is greater than 10.’ This discrepancy leads to confusing and incorrect output.
To avoid such issues, ensure that your conditions and the logic within your if-else statements align correctly. Always test your script with different inputs to confirm that it behaves as expected.
Bash if-else statements are a powerful tool for controlling script flow. However, they require careful attention to syntax and logic. With practice and attention to detail, you can avoid common issues and use if-else statements effectively in your Bash scripts.
Understanding Bash Scripting and Conditional Statements
To fully appreciate the power of Bash if-else statements, we need to understand some fundamental concepts in Bash scripting and conditional statements. This includes logical operators, exit statuses, and the importance of syntax.
Logical Operators in Bash
Logical operators allow you to combine multiple conditions in your if-else statements. Bash supports several logical operators, including -eq
(equal), -ne
(not equal), -gt
(greater than), -ge
(greater than or equal to), -lt
(less than), -le
(less than or equal to), &&
(AND), and ||
(OR).
#!/bin/bash
read -p 'Enter a number: ' num
if [ $num -gt 0 ] && [ $num -lt 10 ]; then
echo 'The number is between 0 and 10.'
else
echo 'The number is not between 0 and 10.'
fi
# Output:
# If you enter '5', the output will be 'The number is between 0 and 10.'
# If you enter '15', the output will be 'The number is not between 0 and 10.'
In this script, we use the &&
operator to check if the entered number is greater than 0 AND less than 10. If it is, we print ‘The number is between 0 and 10.’ If not, we print ‘The number is not between 0 and 10.’
Understanding Exit Statuses
In Bash, every command that is executed returns an exit status. The exit status is a numeric value that indicates whether the command was successful or not. An exit status of 0 indicates success, while any other number indicates an error.
#!/bin/bash
read -p 'Enter a file name: ' filename
if [ -f $filename ]; then
echo 'The file exists.'
else
echo 'The file does not exist.'
fi
# Output:
# If you enter the name of a file that exists in the current directory, the output will be 'The file exists.'
# If you enter the name of a file that does not exist in the current directory, the output will be 'The file does not exist.'
In this script, we use the -f
test operator to check if a file exists. If the file exists, the -f
operator returns an exit status of 0, and we print ‘The file exists.’ If the file does not exist, the -f
operator returns a non-zero exit status, and we print ‘The file does not exist.’
Understanding these fundamental concepts is crucial for mastering Bash if-else statements. With this knowledge, you can write more complex and powerful scripts.
Exploring If-Else in Larger Bash Projects
If-else statements are not just for small scripts. In fact, they are a fundamental part of larger Bash projects. The ability to control the flow of your script based on conditions is crucial in complex scripts and projects. From automating system tasks to processing data, if-else statements in Bash can be used in a variety of scenarios.
Exploring Related Concepts
While if-else is a powerful tool, it’s not the only one you should have in your Bash scripting toolkit. Other related concepts, such as loops and functions, can help you write more efficient and effective scripts.
For instance, loops allow you to perform a set of commands repeatedly, which can be especially useful when processing files or data. Functions, on the other hand, let you group commands together and reuse them throughout your script, promoting code reusability and readability.
Here’s an example of a script that combines if-else, loops, and functions:
#!/bin/bash
function greet() {
if [ $1 == 'morning' ]; then
echo 'Good morning!'
else
echo 'Good evening!'
fi
}
for time in morning evening; do
greet $time
# Output:
# Good morning!
# Good evening!
In this script, we define a function greet
that takes one argument. The function uses an if-else statement to print a different greeting based on the argument. We then use a for loop to call the greet
function with different arguments.
Further Resources for Mastering Bash If-Else
To further your understanding of if-else statements in Bash and related concepts, here are some resources you might find useful:
- GNU Bash Manual: The official manual for Bash, which provides a comprehensive guide to its features, including if-else statements.
- Bash if-else Statement: This Linuxize tutorial focuses on the if-else statement in Bash scripting.
- Bash Scripting Tutorial: A detailed tutorial covering various aspects of Bash scripting, including conditional statements, loops, and functions.
These resources can provide you with a deeper understanding of Bash if-else statements and related concepts, helping you become a more proficient Bash scripter.
Wrapping Up: Mastering If-Else Statements in Bash
In this comprehensive guide, we’ve delved into the heart of using if-else statements in Bash, an essential tool in controlling the flow of your scripts.
We started with the basics, learning how to write simple if-else statements and handle a variety of conditions. We then explored more advanced techniques, such as nested if-else statements and using logical operators to add more complexity to our conditions.
We also discussed common issues one might encounter when using if-else in Bash, such as syntax errors and unexpected behavior. We provided solutions and workarounds for these issues, helping you create more robust and reliable scripts.
In addition, we introduced alternative methods to control script flow, such as using ‘case’ statements and ‘select’ loops. These alternatives can sometimes be more suitable than if-else statements, depending on the situation.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
If-Else | Flexible, powerful | Can be complex, requires careful syntax |
Case Statement | Handles multiple conditions cleanly | Limited to matching patterns |
Select Loop | Creates menus easily | Limited to menu-based choices |
Whether you’re a beginner just starting out with Bash or an experienced scripter looking to deepen your knowledge, we hope this guide has helped you better understand and use if-else statements in Bash.
The ability to control the flow of your script based on conditions is a powerful tool in Bash scripting. Now, you’re better equipped to harness this power in your scripts. Happy scripting!