Python Global Variables | Usage Guide (With Examples)
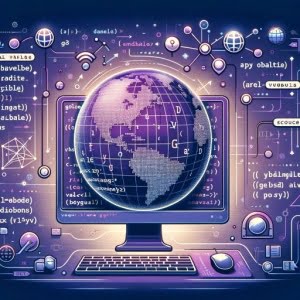
Ever found yourself wondering how to share data across functions in Python? You’re not alone. One solution to this common programming dilemma is the use of global variables.
Think of global variables as a public bulletin board – a space where data can be accessed from anywhere in your program, just like how a notice on a bulletin board can be read by anyone passing by.
In this comprehensive guide, we’ll walk you through the ins and outs of using global variables in Python. We’ll cover everything from the basics of declaring and using global variables, to more advanced topics like when and why to use them in larger programs.
Whether you’re a Python newbie or a seasoned pro looking for a refresher, there’s something here for everyone. Let’s dive in!
TL;DR: How Do I Use Global Variables in Python?
You declare a global variable outside of all functions and can access it from any function in your program. Let’s take a look at a simple example:
x = 10 # This is a global variable
def print_x():
print(x) # This function can access the global variable x
print_x()
# Output:
# 10
In this example, x
is a global variable that we’ve declared outside of all functions. We then define a function print_x()
, which prints the value of x
. When we call print_x()
, it outputs 10
, the value of our global variable x
. This demonstrates how a global variable can be accessed from any function in your program.
Intrigued? Keep reading for a more detailed explanation and advanced usage scenarios of global variables in Python.
Table of Contents
- Understanding Python Global Variables: A Beginner’s Guide
- Global Variables in Larger Programs
- Exploring Alternatives to Global Variables
- Common Pitfalls and Solutions with Global Variables
- Understanding Variable Scope in Python
- The Relevance of Global Variables in Larger Programs
- Further Exploration
- Finding More Resources
- Wrapping Up Python Global Variables
Understanding Python Global Variables: A Beginner’s Guide
In Python, a variable declared outside of the function or in global scope is known as a global variable. This means that a global variable can be accessed inside or outside of the function.
Let’s take a look at how to declare and use global variables in Python with a simple code example:
# Declare a global variable
y = 20
def multiply_by_two():
global y
y = y * 2
multiply_by_two()
print(y)
# Output:
# 40
In this example, we first declare a global variable y
and assign it a value of 20
. We then define a function multiply_by_two()
that multiplies y
by 2
. Inside this function, we use the global
keyword before y
to indicate that we want to use the global y
, not create a new local y
. After we call multiply_by_two()
, y
is updated to 40
in the global scope. So when we print y
, it outputs 40
, the updated value of our global variable y
.
Advantages of Global Variables
One of the main advantages of using global variables in Python is the ability to share data across functions. This can be particularly useful in larger programs where you need to maintain and access certain data throughout your code.
Potential Pitfalls of Global Variables
While global variables can be quite useful, they should be used sparingly. Overuse of global variables can lead to code that’s hard to debug and maintain. This is because any function can change the value of a global variable, so you may have to look through all your functions to figure out why a global variable has a certain value at a certain point in your program. Always remember, with great power comes great responsibility!
Global Variables in Larger Programs
As you delve deeper into Python programming, you’ll inevitably encounter situations where you’ll need to manage data across multiple functions or even across different modules. In such scenarios, global variables can be a lifesaver.
Let’s consider an example where we use a global variable to maintain a counter across multiple functions:
# Declare a global variable
counter = 0
def increment_counter():
global counter
counter += 1
def decrement_counter():
global counter
counter -= 1
increment_counter()
increment_counter()
decrement_counter()
print(counter)
# Output:
# 1
In this example, we have a global variable counter
and two functions: increment_counter()
and decrement_counter()
. These functions increment and decrement the counter
respectively. After calling increment_counter()
twice and decrement_counter()
once, the counter
value is 1
. This shows how a global variable can be used to maintain state across multiple functions.
Best Practices When Using Global Variables
While global variables can be useful, it’s important to use them judiciously. Here are a few best practices to keep in mind:
- Limit the use of global variables: While they offer convenience, overuse of global variables can lead to code that’s hard to debug and maintain.
- Use clear, descriptive names for your global variables: This makes your code easier to understand and maintain.
- Avoid modifying global variables in functions: It’s generally a good idea to avoid modifying global variables in functions. Instead, consider using them to store constants that don’t change.
Remember, the best code is not only about getting the job done but also about being clear, clean, and maintainable.
Exploring Alternatives to Global Variables
While global variables are a straightforward way to share data across functions or modules, Python provides other methods that can be more effective in certain scenarios. Let’s explore some of these alternatives and understand their pros and cons.
Sharing Data Using Classes
One method is to use classes, which can encapsulate related data and functions. Here’s an example:
# Using a class to share data
class Counter:
def __init__(self):
self.count = 0
def increment(self):
self.count += 1
def decrement(self):
self.count -= 1
my_counter = Counter()
my_counter.increment()
my_counter.increment()
my_counter.decrement()
print(my_counter.count)
# Output:
# 1
In this code, we define a Counter
class with a count
attribute and two methods: increment()
and decrement()
. We create an instance my_counter
and call the methods to increment and decrement the count
. The final value of count
is 1
, just like in our previous example with global variables.
Using Modules to Share Data
Another approach is to use modules to share data. You can define a variable in one module and import it in another. This approach is particularly useful when working with larger programs that are split across multiple files.
While these methods offer more control and better organization of your code, they also require a deeper understanding of Python’s object-oriented features. If you’re a beginner, it might be easier to stick with global variables until you’re more comfortable with classes and modules.
Remember, the key is to choose the right tool for the job. While global variables are easy to use, classes and modules offer more control and scalability, especially in larger programs.
Common Pitfalls and Solutions with Global Variables
While global variables can be a powerful tool in Python programming, they can also lead to some common issues and challenges. Let’s discuss some of these problems and explore potential solutions.
Naming Conflicts
One common issue is naming conflicts. This happens when a global variable and a local variable share the same name. Let’s look at an example:
z = 5 # This is a global variable
def change_z():
z = 10 # This is a local variable
print('Inside function:', z)
change_z()
print('Outside function:', z)
# Output:
# Inside function: 10
# Outside function: 5
In this example, we have a global variable z
and a local variable z
inside the change_z()
function. When we print z
inside the function, it outputs 10
, the value of the local z
. But when we print z
outside the function, it outputs 5
, the value of the global z
. This can lead to confusion and unexpected behavior.
The solution to this issue is to use unique names for your global variables or use the global
keyword inside the function to indicate that you want to use the global variable.
Unintended Side Effects
Another issue is unintended side effects, where a function modifies a global variable in a way that affects other parts of your program. This can make your code hard to debug and maintain. The solution is to limit the use of global variables and avoid modifying them inside functions whenever possible.
Remember, while global variables can make your life easier in certain scenarios, they can also introduce new challenges. It’s important to understand these potential issues and how to navigate them.
Understanding Variable Scope in Python
To fully grasp the concept of global variables, it’s important to understand the idea of variable scope in Python. In simple terms, the scope of a variable refers to the regions of the code where the variable can be seen or accessed.
There are two types of variable scope in Python – local and global.
Local Variables and Their Scope
A variable declared inside a function is known as a local variable. This variable is only accessible within the function it is declared and ceases to exist once the function has finished executing. Let’s look at an example:
def my_function():
local_var = 'Hello, World!'
print(local_var)
my_function()
print(local_var)
# Output:
# Hello, World!
# NameError: name 'local_var' is not defined
In this example, local_var
is a local variable declared inside my_function()
. It can be printed inside my_function()
, but when we try to print local_var
outside of the function, Python throws a NameError
. This is because local_var
is not defined in the global scope.
Global Variables and Their Scope
On the other hand, a variable declared outside of all functions, like the ones we’ve been discussing, is a global variable. Global variables are accessible throughout the entire program, both inside and outside of functions.
Understanding the difference between local and global scope is crucial for effective Python programming. It helps you manage your variables effectively and avoid potential naming conflicts or unexpected behavior in your code.
The Relevance of Global Variables in Larger Programs
Global variables play a significant role in Python programming, especially when dealing with larger programs. They provide a way to maintain and access data across different parts of your program, making them a valuable tool in your Python toolkit.
However, as we’ve seen, they should be used judiciously. Overuse or misuse of global variables can lead to code that’s hard to debug and maintain. Therefore, it’s essential to understand when and where to use them effectively.
Further Exploration
While this guide provides a comprehensive overview of global variables in Python, there’s always more to learn. If you’re interested in deepening your knowledge, we recommend exploring related concepts like classes, modules, and variable scope. These concepts offer alternative ways to manage and share data in your Python programs and can give you more control and flexibility.
Finding More Resources
The Python community is vibrant and resourceful, with countless tutorials, guides, and forums available online. Some recommended resources for further reading include the official Python documentation, Python-focused blogs, and interactive platforms like StackOverflow and GitHub. By diving deeper into these resources, you can gain a more profound understanding of Python global variables and other fundamental concepts.
Remember, mastering Python or any programming language is a journey. It’s about continuous learning, practice, and application. So, keep exploring, keep coding, and most importantly, have fun along the way!
Wrapping Up Python Global Variables
In this comprehensive guide, we’ve explored the concept of global variables in Python, a powerful tool that allows data sharing across functions. We’ve examined how to declare and use these variables, discussed potential pitfalls, and offered solutions to common issues.
We’ve also delved into the advanced usage of global variables in larger programs, providing insights into best practices and potential challenges. Moreover, we’ve highlighted alternative approaches for data sharing across functions, such as using classes and modules, adding another layer of depth to your Python programming knowledge.
Here’s a quick comparison of the methods discussed:
Method | Pros | Cons |
---|---|---|
Global Variables | Easy to use, Share data across functions | Can lead to naming conflicts, Hard to debug if overused |
Classes | Encapsulate related data and functions, More control | Require understanding of object-oriented programming |
Modules | Share data across different files, Good for larger programs | Require understanding of Python’s import system |
While global variables are an easy and straightforward method, classes and modules offer more control and are more suitable for larger programs. The key is to understand the requirements of your program and choose the right method accordingly.
Remember, mastering Python is a journey of continuous learning. We hope this guide has deepened your understanding of global variables and equipped you with the knowledge to use them effectively in your Python journey.