Inheritance in Java: Guide to Is-A Class Relationships
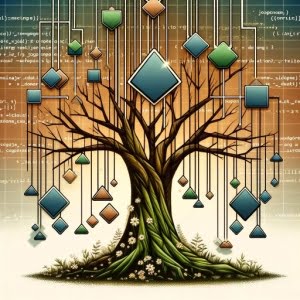
Are you finding it challenging to grasp the concept of inheritance in Java? You’re not alone. Many developers find themselves puzzled when it comes to understanding inheritance in Java, but we’re here to help.
Think of Java’s inheritance as a family tree – just like a child inherits traits from their parents, classes in Java can inherit fields and methods from other classes. It’s a fundamental concept in Java and object-oriented programming that provides a powerful and flexible mechanism for building complex software systems.
This guide will walk you through the basics and advanced concepts of inheritance in Java. We’ll cover everything from the use of the ‘extends’ keyword, superclasses, subclasses, to more complex uses of inheritance such as multiple inheritance with interfaces, method overriding, and the use of abstract classes.
So, let’s dive in and start mastering inheritance in Java!
TL;DR: How Does Inheritance Work in Java?
In Java, a class (known as the child class) can inherit the attributes and methods of another class (referred to as the parent class) using the
extends
keyword:class Child extends Parent {}
. This is a fundamental concept in Java and object-oriented programming that allows for code reusability and a logical, hierarchical structure in your code.
Here’s a simple example:
class Parent {
void parentMethod() {
System.out.println("Parent method");
}
}
class Child extends Parent {
void childMethod() {
System.out.println("Child method");
}
}
Child child = new Child();
child.parentMethod(); // Outputs 'Parent method'
In this example, we have a Parent
class with a method parentMethod()
. We then create a Child
class that extends the Parent
class, meaning it inherits all its attributes and methods. The Child
class also has its own method childMethod()
. When we create an object of the Child
class and call the parentMethod()
, it successfully outputs ‘Parent method’, demonstrating that the Child
class has indeed inherited the method from the Parent
class.
This is just a basic demonstration of how inheritance works in Java. There’s much more to learn about inheritance, including advanced concepts and alternative approaches. Continue reading for a deeper understanding of inheritance in Java.
Table of Contents
- Unveiling Java Inheritance: The Basics
- Delving Deeper: Advanced Inheritance in Java
- Exploring Alternatives to Inheritance in Java
- Troubleshooting Inheritance in Java: Common Issues and Solutions
- Understanding Object-Oriented Programming
- Inheritance in Java: Beyond the Basics
- Wrapping Up: Inheritance in Java
Unveiling Java Inheritance: The Basics
In Java, inheritance is a mechanism where a new class is derived from an existing class. In such a scenario, the existing class is known as the superclass or parent class, and the new class is known as the subclass or child class.
The keyword that makes inheritance happen in Java is ‘extends’. When a class declares that it extends
another class, it inherits all the fields and methods from that class.
Let’s look at a basic example:
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
Dog dog = new Dog();
dog.eat(); // Outputs 'Eating...'
dog.bark(); // Outputs 'Barking...'
# Output:
# Eating...
# Barking...
In this example, Dog
is the subclass that extends the Animal
superclass. This means Dog
inherits the eat()
method from Animal
and can use it as its own. That’s why we can call dog.eat()
, and it will output ‘Eating…’.
The Dog
class also has its own method bark()
, which is not present in the superclass. This shows that a subclass can also have its own unique methods while still inheriting methods from the superclass.
The Super Keyword
Java provides a special keyword called super
that allows a subclass to access methods and fields of its superclass. This is particularly useful when you need to call the superclass’s version of a method.
Here’s a simple demonstration:
class Animal {
void eat() {
System.out.println("Animal is eating...");
}
}
class Dog extends Animal {
void eat() {
System.out.println("Dog is eating...");
}
void eatLikeAnAnimal() {
super.eat();
}
}
Dog dog = new Dog();
dog.eat(); // Outputs 'Dog is eating...'
dog.eatLikeAnAnimal(); // Outputs 'Animal is eating...'
# Output:
# Dog is eating...
# Animal is eating...
In this example, both Animal
and Dog
have an eat()
method. When we call dog.eat()
, it calls the Dog
class’s eat()
method, not the Animal
class’s. However, when we call dog.eatLikeAnAnimal()
, it calls the Animal
class’s eat()
method, thanks to the super
keyword.
Inheritance in Java allows for code reusability and a logical, hierarchical structure in your code. However, it’s essential to be aware of potential pitfalls, such as the risk of creating overly complex inheritance hierarchies. Always strive for simplicity and clarity in your code.
Delving Deeper: Advanced Inheritance in Java
As we move beyond the basics, we encounter more complex uses of inheritance in Java. Let’s explore some of these advanced concepts: multiple inheritance with interfaces, method overriding, and the use of abstract classes.
Multiple Inheritance with Interfaces
Java doesn’t support multiple inheritance directly in classes due to the “Diamond Problem”, but it can be achieved with interfaces. An interface is a completely abstract class that contains only abstract methods. A class can implement multiple interfaces, thereby achieving multiple inheritance.
Here’s an example:
interface A {
void methodA();
}
interface B {
void methodB();
}
class C implements A, B {
public void methodA() {
System.out.println("Method A");
}
public void methodB() {
System.out.println("Method B");
}
}
C c = new C();
c.methodA(); // Outputs 'Method A'
c.methodB(); // Outputs 'Method B'
# Output:
# Method A
# Method B
In this example, C
is a class that implements two interfaces A
and B
. This means C
inherits methods from both A
and B
, achieving multiple inheritance.
Method Overriding
Method overriding is a feature in Java that allows a subclass to provide a specific implementation of a method that is already provided by its superclass. The method in the subclass must have the same name, return type, and parameters as the one in its superclass.
Here’s an example of method overriding:
class Animal {
void eat() {
System.out.println("The animal eats");
}
}
class Dog extends Animal {
void eat() {
System.out.println("The dog eats");
}
}
Dog d = new Dog();
d.eat(); // Outputs 'The dog eats'
# Output:
# The dog eats
In this example, the Dog
class overrides the eat()
method of the Animal
class. When we call d.eat()
, it calls the Dog
class’s eat()
method, not the Animal
class’s.
Using Abstract Classes
An abstract class in Java is a class that can’t be instantiated. It’s used to declare common characteristics of subclasses. An abstract class can have abstract methods (methods without bodies) as well as concrete methods (regular methods with bodies).
Here’s an example of an abstract class:
abstract class Animal {
abstract void eat();
void sleep() {
System.out.println("The animal sleeps");
}
}
class Dog extends Animal {
void eat() {
System.out.println("The dog eats");
}
}
Dog d = new Dog();
d.eat(); // Outputs 'The dog eats'
d.sleep(); // Outputs 'The animal sleeps'
# Output:
# The dog eats
# The animal sleeps
In this example, Animal
is an abstract class with an abstract method eat()
and a concrete method sleep()
. The Dog
class extends Animal
and provides an implementation for the eat()
method. When we create a Dog
object and call d.eat()
and d.sleep()
, it successfully calls the respective methods.
These advanced concepts allow for more flexibility and complexity in your Java programs. However, they should be used judiciously to maintain clear, understandable code.
Exploring Alternatives to Inheritance in Java
While inheritance is a powerful tool in Java, it’s not always the best solution. There are alternative approaches to structuring your code that may be more suitable in certain situations, such as composition and interface implementation.
Composition Over Inheritance
Composition is a design principle in Java that allows you to build complex objects by composing them of simpler ones. Instead of inheriting properties from a superclass, you define properties as objects of other classes.
Here’s an example of composition:
class Engine {
void start() {
System.out.println("Engine starts");
}
}
class Car {
private Engine engine;
Car() {
engine = new Engine();
}
void startEngine() {
engine.start();
}
}
Car car = new Car();
car.startEngine(); // Outputs 'Engine starts'
# Output:
# Engine starts
In this example, instead of Car
inheriting from Engine
, Car
has an Engine
. When startEngine()
is called, it calls the start()
method on engine
. This is a simple demonstration of the principle of composition over inheritance.
Interface Implementation as an Alternative
Interfaces in Java can be a great alternative to inheritance. An interface is a completely abstract class that contains only abstract methods. A class can implement multiple interfaces, which can be a powerful way to add functionality to a class without the constraints of inheritance.
Here’s an example of interface implementation:
interface Eater {
void eat();
}
interface Sleeper {
void sleep();
}
class Animal implements Eater, Sleeper {
public void eat() {
System.out.println("The animal eats");
}
public void sleep() {
System.out.println("The animal sleeps");
}
}
Animal animal = new Animal();
animal.eat(); // Outputs 'The animal eats'
animal.sleep(); // Outputs 'The animal sleeps'
# Output:
# The animal eats
# The animal sleeps
In this example, Animal
is a class that implements two interfaces, Eater
and Sleeper
. This means Animal
inherits methods from both Eater
and Sleeper
, achieving multiple inheritance.
These alternative approaches can provide more flexibility and modularity in your code. However, they come with their own set of considerations and should be used when most appropriate.
Troubleshooting Inheritance in Java: Common Issues and Solutions
While inheritance in Java provides a powerful way to structure your code, it’s not without its pitfalls. Here, we’ll discuss some common issues that developers encounter when using inheritance, including dealing with private and protected members and the infamous diamond problem.
Dealing with Private and Protected Members
In Java, private members of a superclass are not directly accessible in the subclass. This can lead to unexpected behavior if not properly understood. However, protected members are accessible in subclasses, but only within the same package.
Here’s an example demonstrating this behavior:
class Animal {
private String privateField = "Private Field";
protected String protectedField = "Protected Field";
}
class Dog extends Animal {
void accessFields() {
// System.out.println(privateField); // This would throw a compile error
System.out.println(protectedField); // Outputs 'Protected Field'
}
}
Dog dog = new Dog();
dog.accessFields();
# Output:
# Protected Field
In this example, the Dog
class can access the protectedField
from the Animal
class, but trying to access the privateField
would result in a compile error.
The Diamond Problem
The diamond problem is a common issue in programming languages that support multiple inheritance. It occurs when a class inherits from two classes that have a common superclass, leading to ambiguity in method resolution.
Java avoids the diamond problem by not supporting multiple inheritance directly in classes. However, it’s still possible to encounter a similar issue with interfaces, as Java allows a class to implement multiple interfaces.
Here’s an example:
interface A {
default void method() {
System.out.println("Method from A");
}
}
interface B extends A {}
interface C extends A {}
class D implements B, C {
public static void main(String[] args) {
D d = new D();
d.method();
}
}
# This would throw a compile error
In this example, the D
class implements B
and C
, which both extend A
. This leads to ambiguity as to which method()
D
should inherit, resulting in a compile error.
To resolve this, D
must override the method to provide its own implementation or specify which method()
from B
or C
it wants to use.
class D implements B, C {
public void method() {
B.super.method();
}
public static void main(String[] args) {
D d = new D();
d.method(); // Outputs 'Method from A'
}
}
# Output:
# Method from A
In this revised example, D
explicitly calls B
‘s method()
, resolving the ambiguity and allowing the code to compile and run successfully.
Understanding these common issues and knowing how to address them will help you leverage inheritance effectively in your Java programs.
Understanding Object-Oriented Programming
To fully grasp the concept of inheritance in Java, it’s essential to understand the principles of Object-Oriented Programming (OOP). OOP is a programming paradigm based on the concept of ‘objects’, which can contain data and code: data in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods).
There are four fundamental principles of OOP: encapsulation, abstraction, polymorphism, and inheritance.
Encapsulation
Encapsulation is the mechanism that binds together code and the data it manipulates and keeps both safe from outside interference and misuse. It’s achieved by making the fields in a class private and providing access to them via public methods.
public class Student {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Student student = new Student();
student.setName("Alice");
System.out.println(student.getName()); // Outputs 'Alice'
# Output:
# Alice
In this example, the name
field is encapsulated in the Student
class. It’s made private, and we’ve provided a getter and setter to access and modify it.
Abstraction
Abstraction is the process of hiding the complex details and showing only the essential features of the object. In other words, it deals with the outside view of an object (interface).
public abstract class Animal {
public abstract void sound();
}
public class Dog extends Animal {
public void sound() {
System.out.println("Woof");
}
}
Animal dog = new Dog();
dog.sound(); // Outputs 'Woof'
# Output:
# Woof
In this example, Animal
is an abstract class with an abstract method sound()
. Dog
is a class that extends Animal
and provides the implementation for the sound()
method.
Polymorphism
Polymorphism is the ability of an object to take on many forms. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object.
public class Animal {
void sound() {
System.out.println("The animal makes a sound");
}
}
public class Dog extends Animal {
void sound() {
System.out.println("Woof");
}
}
Animal myDog = new Dog();
myDog.sound(); // Outputs 'Woof'
# Output:
# Woof
In this example, myDog
is a reference of type Animal
but points to an object of type Dog
. This allows myDog
to be used to call the Dog
class’s sound()
method, demonstrating polymorphism.
Inheritance
As we’ve discussed, inheritance is a mechanism in which one object acquires all the properties and behaviors of a parent object. It’s an important part of OOP for code reusability and method overriding.
These principles of OOP are the building blocks for Java programming. Understanding them will give you a broader perspective of where inheritance fits into the larger picture of Java programming.
Inheritance in Java: Beyond the Basics
Understanding inheritance in Java is not only crucial for mastering the language but also for applying these concepts in real-world applications. From creating graphical user interfaces (GUIs) with JavaFX to building robust web applications with Spring, the principles of inheritance come into play.
Inheritance in JavaFX
JavaFX is a software platform used to create and deliver desktop applications as well as rich internet applications that can run across a wide variety of devices. Inheritance is often used in JavaFX to extend classes like Application
, Stage
, and Scene
to create custom GUI components.
Building Web Applications with Spring
Spring is a popular framework for building enterprise-grade applications in Java. Inheritance can be used in Spring to create hierarchies of @Component
or @Service
classes, allowing for shared behavior across different parts of an application.
Diving Deeper: Polymorphism and Interfaces
If you’re interested in learning more about Java’s object-oriented principles, polymorphism and interfaces are excellent next steps. Polymorphism, a companion concept to inheritance, allows objects of different types to be treated as objects of a common super type. Interfaces, on the other hand, define a contract for classes and play a crucial role in Java’s type system.
Further Resources for Mastering Java Inheritance
If you wish to delve deeper into the world of Java inheritance, here are some resources that might be of interest:
- Guide to Exploring Java OOPs Concepts – Dive into Java’s Object-Oriented Programming (OOP) principles.
Java Multiple Inheritance – Understand how Java achieves multiple inheritance through interfaces.
Encapsulation in Java – Understand the concept of encapsulation in Java for bundling data and methods within a class.
Oracle’s Java Tutorials cover all aspects of the language, including inheritance, in great detail.
Java Programming Masterclass for Software Developers course on Udemy covers Java in depth, including the concept of inheritance.
Java Inheritance Tutorial provides a deep dive into Java inheritance, with examples and explanations.
Remember, mastering a concept like inheritance takes time and practice. Don’t rush the process and try to write plenty of your own code to reinforce your understanding.
Wrapping Up: Inheritance in Java
In this comprehensive guide, we’ve journeyed through the concept of inheritance in Java, a fundamental pillar of object-oriented programming. We’ve explored how classes in Java can inherit fields and methods from other classes, similar to how a child inherits traits from their parents, and how this forms the basis for more complex programming constructs.
We began with the basics, explaining how inheritance works in Java, including the use of the ‘extends’ keyword, superclasses, subclasses, and the ‘super’ keyword. We then delved into more advanced uses of inheritance, such as multiple inheritance with interfaces, method overriding, and the use of abstract classes. We also discussed alternative approaches to inheritance, such as composition and interface implementation, providing different perspectives on structuring your code.
Along the way, we tackled common issues that developers may encounter when using inheritance, such as dealing with private and protected members, and the diamond problem, providing you with solutions and workarounds for each issue. We also provided a broader understanding of where inheritance fits into the larger picture of Java programming by explaining the principles of object-oriented programming.
Whether you’re just starting out with Java or looking to deepen your understanding of inheritance, we hope this guide has been a valuable resource. With its balance of theoretical knowledge and practical examples, it should provide a solid foundation for mastering inheritance in Java. Happy coding!