JavaFX: Guide to Creating Visual Desktop Applications
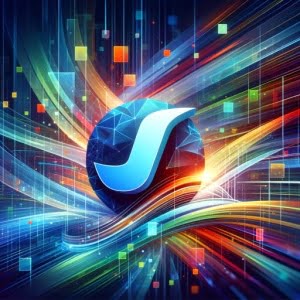
Are you finding it challenging to create rich, stunning desktop applications? You’re not alone. Many developers find themselves puzzled when it comes to handling JavaFX, a software platform for creating and delivering desktop applications.
Think of JavaFX as a master artist’s palette – it provides a rich set of graphics and media APIs, allowing you to create visually engaging applications with ease.
This guide will walk you through the process of mastering JavaFX, from the basics to more advanced techniques. We’ll cover everything from creating a simple JavaFX application to handling complex features such as animations, event handling, and styling with CSS.
So, let’s dive in and start mastering JavaFX!
TL;DR: What is JavaFX and How Do I Use It?
JavaFX
is a software platform for creating and delivering desktop applications. You use the JavaFX software, by creating a class that extends theApplication
class, such as:public class HelloWorld extends Application {}
. You will then need to provide an implementation of the start() method, such aspublic static void main(String[] args){launch(args)};
It provides a rich set of graphics and media APIs, enabling developers to create visually engaging applications with ease. Here’s a simple example of a JavaFX application:
public class HelloWorld extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Hello World!");
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Hello World!");
}
});
StackPane root = new StackPane();
root.getChildren().add(btn);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
}
In this example, we create a simple JavaFX application that displays a button labeled ‘Say ‘Hello World”. When the button is clicked, ‘Hello World!’ is printed to the console.
This is just a basic way to use JavaFX, but there’s much more to learn about creating and manipulating applications with this powerful platform. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- JavaFX Basics: Creating Your First Application
- JavaFX Advanced Features: Animations, Event Handling, and Styling with CSS
- Exploring Alternative Java GUI Frameworks
- Navigating JavaFX: Common Issues and Solutions
- JavaFX Architecture: The Backbone of Your Application
- JavaFX vs Other Java GUI Frameworks
- JavaFX in Modern Application Development
- Wrapping Up: JavaFX for Rich Client Applications
JavaFX Basics: Creating Your First Application
Let’s start at the beginning: creating a basic JavaFX application. This is a crucial first step to understanding JavaFX and its components.
Step-by-Step Guide to Your First JavaFX Application
Here’s a simple step-by-step guide to creating your first JavaFX application:
- Extend the Application Class: Every JavaFX application must extend the
Application
class. This class is abstract, so you’ll need to provide an implementation of thestart()
method.
public class HelloWorld extends Application {
public static void main(String[] args) {
launch(args);
}
}
In the above code, we’ve created a HelloWorld
class that extends the Application
class. The main()
method calls the launch()
method, which is part of the Application
class.
- Override the start() Method: The
start()
method is the main entry point for all JavaFX applications. Here, you’ll set the stage (the top-level JavaFX container) and scene (the content inside the stage).
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Hello World!");
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Hello World!");
}
});
StackPane root = new StackPane();
root.getChildren().add(btn);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
In this code block, we’ve set the title of the stage, created a button, and added an action event to the button. When the button is clicked, “Hello World!” will be printed to the console. We then add the button to a layout pane and set the scene.
- Run the Application: Finally, you can run your JavaFX application. You should see a window with a button labeled “Say ‘Hello World'”. When you click the button, “Hello World!” will be printed to the console.
These are the basic components and steps of a JavaFX application. In the next section, we’ll dive deeper into more advanced features of JavaFX.
JavaFX Advanced Features: Animations, Event Handling, and Styling with CSS
Once you’ve mastered the basics of JavaFX, it’s time to move on to more complex features. These include animations, event handling, and styling with CSS. Let’s explore these in detail.
Animations in JavaFX
JavaFX provides a powerful animation API. You can create complex animations with smooth transitions and effects. Here’s an example of a simple animation in JavaFX:
RotateTransition rt = new RotateTransition(Duration.millis(3000), btn);
rt.setByAngle(360);
rt.setCycleCount(4);
rt.setAutoReverse(true);
rt.play();
In this example, we create a RotateTransition
for the button we created earlier. The button will rotate 360 degrees over 3 seconds, repeating 4 times, and reversing direction after each cycle.
Event Handling in JavaFX
JavaFX provides a robust framework for handling user events like button clicks or key presses. We’ve already seen a basic example of event handling in the ‘Hello World’ application. Here’s how you might handle a key press event:
scene.setOnKeyPressed(new EventHandler<KeyEvent>() {
public void handle(KeyEvent event) {
System.out.println("Key Pressed: " + event.getCode());
}
});
In this example, we set an event handler on the scene that prints the code of any key pressed.
Styling with CSS in JavaFX
JavaFX allows you to style your application using CSS, similar to how you would style a web page. Here’s an example of setting a CSS style on a button:
btn.setStyle("-fx-background-color: #ff0000; -fx-text-fill: #0000ff;");
In this example, we set the background color of the button to red (#ff0000
) and the text color to blue (#0000ff
).
These are just a few examples of the advanced features available in JavaFX. With these tools, you can create rich, interactive desktop applications.
Exploring Alternative Java GUI Frameworks
While JavaFX is a powerful tool for creating rich, interactive desktop applications, it’s not the only game in town. There are other Java GUI frameworks, such as Swing and AWT, that you might consider using depending on your specific needs.
Swing vs. AWT vs. JavaFX
Let’s take a look at these three frameworks and discuss when you might want to use each one.
- Swing: Swing is a GUI widget toolkit for Java. It’s part of Oracle’s Java Foundation Classes (JFC) — an API for providing a graphical user interface (GUI) for Java programs. Swing was designed to provide a more sophisticated set of GUI components than the earlier Abstract Window Toolkit (AWT).
AWT: AWT (Abstract Window Toolkit) is Java’s original platform-dependent windowing, graphics, and user-interface widget toolkit. AWT components are heavy-weight, which means they are dependent on the local windowing toolkit. For example, a java.awt.Button is a real Motif button (in Unix), whereas a javax.swing.JButton is emulated by the JVM.
JavaFX: As we’ve seen, JavaFX is a software platform for creating and delivering desktop applications, as well as rich internet applications (RIAs) that can run across a wide variety of devices.
Here’s a comparison table to help you understand the pros and cons of each framework:
Framework | Pros | Cons |
---|---|---|
Swing | More sophisticated set of GUI components than AWT | Slower performance than JavaFX |
AWT | Native system appearance | Less advanced than Swing and JavaFX |
JavaFX | Rich set of graphics and media APIs, CSS for styling | Requires more resources than Swing or AWT |
Choosing the right framework for your project depends on a variety of factors, including the complexity of your application, the resources available, and your personal preference.
As with any technology, you’re likely to encounter some hurdles when working with JavaFX. Here, we’ll discuss common issues, including setup problems, compatibility issues, and performance considerations, and offer solutions and workarounds.
Setup Problems
Setting up JavaFX can sometimes be a challenge, especially if you’re new to the platform. One common issue is not having the correct version of Java installed. JavaFX requires Java 8 or later, so make sure to update if you’re using an older version.
Another common setup problem is not having the JavaFX SDK installed or not having it properly configured in your IDE. You can download the JavaFX SDK from the official website, and most IDEs have specific instructions for configuring JavaFX.
Compatibility Issues
JavaFX applications can sometimes encounter compatibility issues, especially when trying to run on different operating systems. To mitigate these issues, it’s important to test your application on all target platforms.
If you’re encountering specific compatibility issues, consider using tools like JLink and JPackage, which can help you bundle a Java runtime environment with your application, ensuring it runs consistently across platforms.
Performance Considerations
JavaFX applications, particularly those with complex UIs or animations, can sometimes experience performance issues. Here are a few tips to improve the performance of your JavaFX application:
- Use the latest version of Java and JavaFX. Newer versions often come with performance improvements.
Optimize your scene graph. Try to minimize the number of nodes and avoid unnecessary nesting.
Use CSS sparingly. While CSS is great for styling your application, overuse can lead to performance issues.
Navigating these common issues will help you become more proficient in JavaFX and enable you to develop robust, efficient applications.
JavaFX Architecture: The Backbone of Your Application
To truly master JavaFX, it’s essential to understand its architecture. The JavaFX architecture is the backbone of your application, influencing how you design and build your programs.
Understanding the JavaFX Scene Graph
At the heart of JavaFX is the scene graph. The scene graph is a hierarchical tree of nodes that represents all of the visual elements of your application’s user interface. It includes nodes for geometric shapes, text, images, media, and UI controls.
Group root = new Group();
Circle circle = new Circle(50, Color.BLUE);
root.getChildren().add(circle);
Scene scene = new Scene(root);
In this code block, we create a Group
(which is a type of Parent
node), a Circle
(a type of Shape
node), and add the circle to the group. We then create a Scene
with the group as its root.
JavaFX Application Lifecycle
A JavaFX application follows a specific lifecycle. It begins with the launch()
method, which then calls the init()
method, the start()
method, and finally the stop()
method.
public class HelloWorld extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void init() {
System.out.println("Before the start method");
}
@Override
public void start(Stage primaryStage) {
System.out.println("Start method");
}
@Override
public void stop() {
System.out.println("After the start method");
}
}
In this code block, we override the init()
, start()
, and stop()
methods to print out messages that indicate their execution order. When you run this application, you’ll see that it prints “Before the start method”, then “Start method”, and finally “After the start method”.
JavaFX vs Other Java GUI Frameworks
JavaFX offers several advantages over other Java GUI frameworks, such as Swing and AWT. It provides a more modern and comprehensive API for creating rich client applications. It also includes features like CSS styling and hardware-accelerated graphics, which are not available in Swing or AWT.
Understanding these fundamentals of JavaFX will provide a solid foundation for mastering this powerful platform.
JavaFX in Modern Application Development
JavaFX is not just a tool of the past; it’s highly relevant in today’s world of application development. Its rich set of graphics and media APIs, combined with the ability to create complex, rich client applications, makes it a go-to choice for many developers.
JavaFX: Building Complex, Rich Client Applications
JavaFX shines when it comes to building complex, rich client applications. Its scene graph, CSS styling capabilities, and hardware-accelerated graphics allow developers to create visually stunning applications that provide a superior user experience.
Consider a data visualization application, for example. With JavaFX, you could create interactive charts and graphs, incorporate multimedia elements, and provide a highly responsive user interface.
PieChart pieChart = new PieChart();
PieChart.Data slice1 = new PieChart.Data("Desktop", 213);
PieChart.Data slice2 = new PieChart.Data("Mobile", 67);
PieChart.Data slice3 = new PieChart.Data("Tablet", 36);
pieChart.getData().add(slice1);
pieChart.getData().add(slice2);
pieChart.getData().add(slice3);
In this code block, we create a PieChart
and add three slices to it. This is a simple example, but imagine the possibilities when you start incorporating animations, event handling, and custom styling.
The Future of JavaFX
JavaFX has a promising future. It continues to be updated and improved, with new features being added and existing ones being refined. The active community around JavaFX also contributes to its growth and development.
Moreover, with the advent of tools like GraalVM, JavaFX applications can now be compiled to native code, improving performance and reducing resource usage.
Further Resources for JavaFX Mastery
To continue your journey with JavaFX, check out these resources:
- Exploring Java Package Management – Covers tips for package declaration, naming conventions and more.
Exploring Jython – Learn how Jython enables seamless integration of Python code with Java applications.
Java Swing Overview – Dive into Java Swing, the standard GUI toolkit for Java applications.
JavaFX Official Documentation is a comprehensive resource that covers all aspects of JavaFX.
JavaFX Tutorial by Oracle provides a good starting point for beginners to JavaFX.
JavaFX on GitHub is a great place to see the latest developments in JavaFX, report issues, and even contribute.
Wrapping Up: JavaFX for Rich Client Applications
In this comprehensive guide, we’ve delved into the world of JavaFX, a powerful software platform for creating and delivering desktop applications. We’ve explored its rich set of graphics and media APIs, which provide the tools necessary to build visually stunning applications.
We began with the basics, learning how to create a simple JavaFX application and understanding the key components involved. We then ventured into more advanced territory, exploring complex features such as animations, event handling, and styling with CSS.
Along the way, we tackled common challenges one might face when using JavaFX, such as setup problems, compatibility issues, and performance considerations, providing solutions and workarounds for each issue.
We also looked at alternative approaches to GUI development in Java, comparing JavaFX with other Java GUI frameworks like Swing and AWT. Here’s a quick comparison of these frameworks:
Framework | Pros | Cons |
---|---|---|
JavaFX | Rich set of graphics and media APIs, CSS for styling | Requires more resources than Swing or AWT |
Swing | More sophisticated set of GUI components than AWT | Slower performance than JavaFX |
AWT | Native system appearance | Less advanced than Swing and JavaFX |
Whether you’re just starting out with JavaFX or looking to level up your skills, we hope this guide has given you a deeper understanding of JavaFX and its capabilities. With this knowledge in hand, you’re well-equipped to create rich, interactive desktop applications using JavaFX. Happy coding!