Jython: Python and Java Integration Made Easy
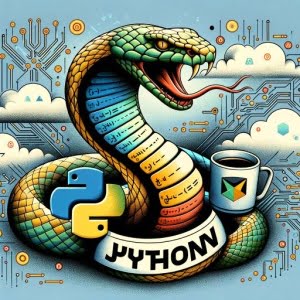
Are you a Python enthusiast looking to harness its power in a Java environment? Or perhaps a Java developer intrigued by Python’s simplicity and dynamism? Jython is the tool that bridges these two worlds, and it might just be what you’re looking for.
Jython, a Python interpreter written in Java, allows you to leverage the strengths of both languages. It’s like a bilingual interpreter, translating Python’s expressiveness and Java’s robustness into a language that your system understands.
In this guide, we will delve into the world of Jython, exploring its basic usage, advanced features, and how it seamlessly integrates Python and Java. We’ll also look at common issues you might encounter and how to troubleshoot them. So, let’s dive in and start mastering Jython!
TL;DR: What is Jython and How Do I Use It?
Jython
is an implementation of the Python programming language designed to run on the Java platform. It can be downloaded fromwww.jython.org/download
. It allows Python code to be executed as Java bytecode, enabling integration with Java frameworks and libraries, such as the Java Random class,from java.util import Random
.
Here’s a simple example of Jython code:
from java.util import Random
r = Random()
print(r.nextInt())
# Output:
# [Random integer]
In this example, we import the Random class from the java.util package, create a new Random object, and print a random integer. This demonstrates how Jython allows us to use Java classes in Python-like syntax.
This is just a basic introduction to Jython. There’s much more to learn about how it can be used to integrate Python and Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Jython
- Harnessing Jython for Python-Java Integration
- Alternative Methods for Python-Java Integration
- Navigating Jython: Troubleshooting and Key Considerations
- Python and Java: A Comparative Look
- Jython: The Best of Both Worlds
- Jython in the Real World: Enterprise Applications and Web Development
- Exploring Related Concepts: JVM and Bytecode
- Wrapping Up: Jython for Python-Java Integration
Getting Started with Jython
Installing Jython
Before we can start writing Jython scripts, we first need to install Jython on our system. Here’s how to do it:
# Download Jython installer
wget 'https://www.jython.org/downloads.html'
# Install Jython
java -jar jython_installer-2.7.2.jar
This will download the Jython installer and then use Java to run the installer. Make sure you have Java installed on your system before running these commands.
Setting Up the Jython Environment
Once Jython is installed, we need to set up the environment. This involves setting the JYTHON_HOME environment variable and adding Jython to the PATH. Here’s how to do it:
# Set JYTHON_HOME
export JYTHON_HOME=/path/to/jython
# Add Jython to PATH
export PATH=$JYTHON_HOME/bin:$PATH
Replace /path/to/jython
with the actual path where Jython is installed. These commands set up the environment so that we can run Jython from any location in the terminal.
Writing Your First Jython Script
Now that Jython is installed and the environment is set up, we can start writing Jython scripts. Here’s a simple Jython script that prints ‘Hello, Jython!’:
print('Hello, Jython!')
# Output:
# 'Hello, Jython!'
This script uses the print
function to output a string. Although this is a simple example, it demonstrates how Jython allows us to write Python-like code that runs on the Java platform.
Advantages and Potential Pitfalls
The main advantage of Jython is that it allows us to leverage the strengths of both Python and Java. We can use Python’s simplicity and dynamism along with Java’s robustness and extensive libraries. However, there are potential pitfalls. For example, not all Python libraries are compatible with Jython, and Jython scripts can be slower than native Python or Java code. It’s important to keep these considerations in mind when deciding to use Jython.
Harnessing Jython for Python-Java Integration
Accessing Java Libraries from Python Code
One of the key advantages of Jython is its ability to integrate Python and Java. This includes accessing Java libraries directly from Python code. Let’s look at an example using the ArrayList class from the java.util package:
from java.util import ArrayList
# Create an ArrayList
my_list = ArrayList()
# Add elements to the ArrayList
my_list.add('Jython')
my_list.add('Python')
my_list.add('Java')
# Print the ArrayList
print(my_list)
# Output:
# ['Jython', 'Python', 'Java']
In this example, we import the ArrayList class from the java.util package, create an ArrayList, add elements to it, and print it. The output is a list of the elements we added, demonstrating how Jython allows us to use Java classes in Python-like syntax.
Accessing Python Code from Java
Jython also allows us to access Python code from Java. This is done using the Jython interpreter. Here’s an example:
import org.python.util.PythonInterpreter;
public class Main {
public static void main(String[] args) {
PythonInterpreter interpreter = new PythonInterpreter();
interpreter.exec("print('Hello, Jython!')");
}
}
# Output:
# 'Hello, Jython!'
In this Java code, we create a PythonInterpreter object and use its exec method to execute Python code. The output is the string ‘Hello, Jython!’, demonstrating how Jython allows us to execute Python code from Java.
Analyzing the Output
The output from these examples shows that Jython provides a seamless integration between Python and Java. This enables us to leverage the strengths of both languages, such as Python’s simplicity and Java’s robustness, in the same codebase. However, it’s important to note that not all Python and Java features are compatible with Jython, so some adaptation may be required.
Alternative Methods for Python-Java Integration
While Jython provides a seamless way to integrate Python and Java, there are other tools available for achieving the same goal. Let’s take a look at two of them: GraalVM and Pyjnius.
GraalVM: A Universal Virtual Machine
GraalVM is a universal virtual machine that supports multiple languages, including Python and Java. It allows you to run programs written in different languages in the same application, which can be very useful for integrating Python and Java.
Here’s an example of how to use GraalVM to run Python code from Java:
import org.graalvm.polyglot.*;
public class Main {
public static void main(String[] args) {
try (Context context = Context.create()) {
context.eval("python", "print('Hello, GraalVM!')");
}
}
}
# Output:
# 'Hello, GraalVM!'
In this example, we create a GraalVM context and use it to evaluate Python code. The output is the string ‘Hello, GraalVM!’, demonstrating how GraalVM allows us to execute Python code from Java.
Pyjnius: Accessing Java Classes in Python
Pyjnius is a Python library for accessing Java classes. It’s a bit like Jython in reverse: instead of a Python interpreter written in Java, it’s a Java interpreter written in Python.
Here’s an example of how to use Pyjnius to access a Java class from Python:
from jnius import autoclass
# Access the System class
System = autoclass('java.lang.System')
# Print the current time in milliseconds
print(System.currentTimeMillis())
# Output:
# [Current time in milliseconds]
In this example, we use Pyjnius to access the System class from the java.lang package and print the current time in milliseconds. The output is the current time in milliseconds, demonstrating how Pyjnius allows us to use Java classes in Python.
Weighing the Options
While GraalVM and Pyjnius offer alternative approaches to integrating Python and Java, they each have their own advantages and disadvantages. GraalVM supports multiple languages and allows for high-performance code execution, but its Python support is currently experimental. Pyjnius, on the other hand, is easy to use and provides direct access to Java classes, but it doesn’t support running Python code on the Java Virtual Machine like Jython does.
In the end, the best tool for integrating Python and Java depends on your specific needs and constraints. If you need to run Python code on the JVM, Jython might be the best choice. If you need to access Java classes from Python, Pyjnius could be the way to go. And if you need to integrate multiple languages in a high-performance application, GraalVM might be worth considering.
While Jython is a powerful tool for integrating Python and Java, it may come with its own set of challenges. Let’s discuss some common issues you might encounter, along with solutions and workarounds.
Compatibility Issues with Python Libraries
One of the potential issues with Jython is that it might not be compatible with all Python libraries. For example, libraries that rely on CPython’s C extension modules may not work with Jython.
If you encounter an issue with a Python library, a possible workaround is to look for a Java library that provides similar functionality. For instance, if a Python library for handling JSON is incompatible, you could use Java’s built-in org.json
package instead.
Performance Considerations
Jython scripts can sometimes be slower than native Python or Java code. This is because Jython has to translate Python code into Java bytecode, which can add an overhead.
To mitigate this, you can use Java’s Just-In-Time (JIT) compiler, which can optimize the bytecode at runtime. Here’s how to enable the JIT compiler:
# Run Jython with the JIT compiler
java -Dpython.jythonc.compiler=modern -jar jython.jar your_script.py
In this command, -Dpython.jythonc.compiler=modern
enables the JIT compiler, which can improve the performance of your Jython scripts.
Working with Java Libraries
While Jython allows you to access Java libraries from Python code, it might not always be straightforward. Java’s static typing can sometimes clash with Python’s dynamic typing, leading to unexpected errors.
One solution is to always check the Java documentation for the types expected by a method. This way, you can ensure you’re passing the correct types from your Python code.
from java.lang import String
from java.util import ArrayList
# Create an ArrayList of Strings
my_list = ArrayList()
# Add a String to the ArrayList
my_list.add(String('Jython'))
# Print the ArrayList
print(my_list)
# Output:
# ['Jython']
In this example, we use the String
class from the java.lang
package to ensure we’re adding a Java string to the ArrayList
. This prevents a type mismatch error that could occur if we tried to add a Python string to the ArrayList
.
Remember, while Jython does an excellent job bridging the gap between Python and Java, it’s important to keep these considerations in mind to ensure smooth and efficient coding.
Python and Java: A Comparative Look
Python: The Power of Simplicity
Python is a high-level, interpreted programming language known for its simplicity and readability. It emphasizes code readability, allowing programmers to express concepts in fewer lines of code than might be possible in languages such as C++ or Java. It’s dynamically typed, meaning you don’t have to declare the data type of a variable when you create it.
Here’s an example of Python code:
# Python code to print 'Hello, Python!'
print('Hello, Python!')
# Output:
# 'Hello, Python!'
This simple Python program prints the string ‘Hello, Python!’. The simplicity and expressiveness of Python make it popular for a wide range of applications, from web development to data analysis.
Java: Robust and Versatile
Java, on the other hand, is a statically-typed, object-oriented language that’s designed to be portable and secure. It’s used for building platform-independent applications, from mobile apps to enterprise systems.
Here’s an example of Java code:
// Java code to print 'Hello, Java!'
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Java!');
}
}
# Output:
# 'Hello, Java!'
This Java program prints the string ‘Hello, Java!’. Although Java code can be more verbose than Python, it’s robust and versatile, making it a go-to language for many complex applications.
Jython: The Best of Both Worlds
While Python and Java each have their strengths, they also have fundamental differences. Python’s dynamic typing and simplicity contrast with Java’s static typing and robustness. This is where Jython comes in.
Jython is an implementation of Python that runs on the Java platform (JVM). It integrates Python’s simplicity and dynamism with Java’s robustness and versatility. With Jython, you can write Python code that interacts with Java code, making it a powerful tool for integrating Python and Java.
In the context of our search query string ‘jython’, understanding the fundamentals of Python and Java, and how Jython bridges the gap between them, is crucial. It gives us the background needed to appreciate the power and versatility of Jython.
Jython in the Real World: Enterprise Applications and Web Development
Jython’s ability to integrate Python and Java makes it a powerful tool in various real-world applications, particularly in enterprise environments and web development.
Jython in Enterprise Applications
In enterprise applications, Jython can be used to write Python scripts that interact with Java-based enterprise systems. This allows developers to leverage Python’s simplicity and dynamism while taking advantage of Java’s robustness and extensive libraries.
Jython in Web Development
In web development, Jython can be used with Java web frameworks like Spring and Struts, allowing developers to write Python code that integrates with these frameworks. This can make web development more efficient and flexible, particularly for developers who are more comfortable with Python than Java.
Exploring Related Concepts: JVM and Bytecode
To deepen your understanding of Jython, it’s helpful to explore related concepts like the Java Virtual Machine (JVM) and bytecode.
The JVM is the platform on which Jython runs. It’s what allows Jython to translate Python code into Java bytecode, which can be executed on any system with a JVM.
Bytecode is the intermediate representation of code that the JVM interprets. When you write a Jython script, it’s compiled into bytecode, which is then interpreted by the JVM.
Understanding these concepts can give you a deeper appreciation of how Jython works and why it’s such a powerful tool for integrating Python and Java.
Further Resources for Jython Journey
To understand how to import and use classes like Jython in Java programs, Click Here for a complete guide!
To continue your journey with Jython, here are some resources that you might find helpful:
- Drools Overview – Dive into Drools, a powerful open-source rules engine for Java applications
Introduction to JavaFX – Explore JavaFX, a powerful framework for building rich client applications.
Official Jython Documentation includes a getting started guide, a detailed tutorial, and API references.
Jython Book – A comprehensive book on Jython basic usage and advanced features.
Real Python offers a wealth of Python tutorials and resources that can be applied to Jython.
Wrapping Up: Jython for Python-Java Integration
In this comprehensive guide, we’ve delved into the world of Jython, a powerful tool that bridges the gap between Python and Java.
We began with the basics, learning how to install Jython, set up the environment, and write our first Jython script. We then ventured into more advanced territory, exploring how to use Jython for Python-Java integration, including accessing Java libraries from Python code and vice versa. Along the way, we tackled common issues you might encounter when using Jython, such as compatibility issues with Python libraries and performance considerations, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Python-Java integration, comparing Jython with other tools like GraalVM and Pyjnius. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Jython | Seamless Python-Java integration, access to Java libraries in Python | Compatibility issues with some Python libraries, potential performance overhead |
GraalVM | Supports multiple languages, high-performance code execution | Experimental Python support |
Pyjnius | Easy to use, direct access to Java classes in Python | Doesn’t support running Python code on the JVM |
Whether you’re just starting out with Jython or you’re looking to level up your Python-Java integration skills, we hope this guide has given you a deeper understanding of Jython and its capabilities.
With its ability to leverage the strengths of both Python and Java, Jython is a powerful tool for any developer’s toolkit. Happy coding!