Drools in Java | Guide for Setup, Rules and, Syntax
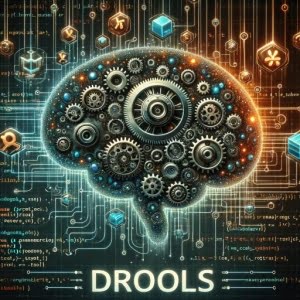
Are you finding it challenging to implement business rules in your Java application? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can make this process a breeze.
Like a skilled craftsman, Drools, a powerful Business Rules Management System (BRMS), is a handy utility that can seamlessly integrate with your Java code. These rules can run on any system, even those without Java installed.
This guide will walk you through the process of using Drools in Java, from the basics to more advanced techniques. We’ll explore Drools’ core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Drools in Java!
TL;DR: How Do I Use Drools in Java?
To use
Drools
in Java, you need to create a KnowledgeBase and a StatefulSession with the syntax,KnowledgeBase kbase = KnowledgeBaseFactory.newKnowledgeBase();
andStatefulKnowledgeSession ksession = kbase.newStatefulKnowledgeSession();
. Then, you can load your rules into the KnowledgeBase and fire them using the StatefulSession.
Here’s a simple example:
import org.drools.KnowledgeBase;
import org.drools.KnowledgeBaseFactory;
import org.drools.runtime.StatefulKnowledgeSession;
KnowledgeBase kbase = KnowledgeBaseFactory.newKnowledgeBase();
StatefulKnowledgeSession ksession = kbase.newStatefulKnowledgeSession();
// Load rules
// kbase.addKnowledgePackages( ... );
// Fire rules
// ksession.fireAllRules();
# Output:
# (Depends on the rules loaded and the data provided)
In this example, we first import the necessary classes from the Drools library. We then create a new KnowledgeBase and a StatefulSession. The rules would be loaded into the KnowledgeBase using the addKnowledgePackages
method, and then fired using the fireAllRules
method on the StatefulSession.
This is just a basic way to use Drools in Java, but there’s much more to learn about managing business rules effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started: Using Drools in Java
Let’s begin by understanding the core components of Drools and how to use them in Java. We’ll focus on three main elements: the KnowledgeBase, the StatefulSession, and the process of loading and firing rules.
Creating a KnowledgeBase
The KnowledgeBase is a repository of all the application’s knowledge definitions. It’s where your rules will live. Here’s how you create one:
import org.drools.KnowledgeBase;
import org.drools.KnowledgeBaseFactory;
KnowledgeBase kbase = KnowledgeBaseFactory.newKnowledgeBase();
In this code, we’re importing the necessary classes and creating a new instance of KnowledgeBase using the KnowledgeBaseFactory.
Creating a StatefulSession
The StatefulSession is what allows you to interact with the KnowledgeBase. It’s where you’ll fire your rules. Here’s how you create one:
import org.drools.runtime.StatefulKnowledgeSession;
StatefulKnowledgeSession ksession = kbase.newStatefulKnowledgeSession();
Here, we’re creating a new StatefulKnowledgeSession from the KnowledgeBase we created earlier.
Loading and Firing Rules
Now that we have our KnowledgeBase and StatefulSession, we can load and fire rules. Here’s a basic example:
// Load rules
// kbase.addKnowledgePackages( ... );
// Fire rules
// ksession.fireAllRules();
# Output:
# (Depends on the rules loaded and the data provided)
In this example, we’re adding rules to the KnowledgeBase using the addKnowledgePackages
method and then firing them using the fireAllRules
method on the StatefulSession. The output will depend on the rules you’ve loaded and the data you’ve provided.
By mastering these basic concepts, you’re well on your way to effectively using Drools in your Java applications. But don’t stop here – there’s plenty more to learn!
Handling Rule Conflicts and Decision Tables
As you delve deeper into using Drools with Java, you’ll encounter more complex scenarios. Two such instances are handling rule conflicts and using decision tables. Let’s explore each one.
Resolving Rule Conflicts
When multiple rules are eligible to fire, conflicts may arise. Drools resolves this by using a conflict resolution strategy. Here’s an example:
// Assume we have two rules: Rule1 and Rule2
// Rule1
rule "Rule1"
when
// condition
then
// action
end
// Rule2
rule "Rule2"
when
// condition
then
// action
end
// Fire rules
ksession.fireAllRules();
# Output:
# (Depends on the conflict resolution strategy and the conditions provided)
In this example, both Rule1 and Rule2 are eligible to fire. Drools uses a conflict resolution strategy (like salience or activation groups) to decide which rule fires first.
Using Decision Tables
Decision tables are a more readable and manageable way to define rules. They’re especially useful when you have a large number of rules. Here’s a basic example:
// Assume we have a decision table: DecisionTable
// Load decision table
// kbase.addKnowledgePackages( ... );
// Fire rules
ksession.fireAllRules();
# Output:
# (Depends on the decision table loaded and the data provided)
In this example, we’re loading a decision table into the KnowledgeBase and firing the rules. The output will depend on the decision table and the data you’ve provided.
Understanding these advanced aspects of using Drools in Java will allow you to manage business rules more effectively and efficiently in larger, more complex applications.
Exploring Alternative Approaches
While Drools is an excellent tool for managing business rules in Java, it’s not the only one. Other Business Rules Management Systems (BRMS) and manual coding can also be used. Let’s discuss these alternatives, their benefits, drawbacks, and when to use them.
Other BRMS Solutions
There are other BRMS solutions like jBPM, OpenRules, and EasyRules that you can use. Here’s a simple example of using EasyRules:
// Import EasyRules library
import org.jeasy.rules.api.Rules;
import org.jeasy.rules.core.DefaultRulesEngine;
// Create a new rule
// Rule myRule = new Rule( ... );
// Create a rules engine
Rules rules = new Rules();
DefaultRulesEngine rulesEngine = new DefaultRulesEngine();
// Register the rule
rules.register(myRule);
// Fire rules
rulesEngine.fire(rules, facts);
# Output:
# (Depends on the rule and the facts provided)
In this example, we’re creating a new rule, registering it with the rules engine, and then firing the rules. The output will depend on the rule you’ve created and the facts provided.
Manual Coding of Business Rules
You can also manually code business rules in Java. This approach gives you the most control but can be time-consuming and complex. Here’s a basic example:
// Define a business rule
if (condition) {
// action
}
# Output:
# (Depends on the condition and action provided)
In this example, we’re defining a business rule using an if statement. The output will depend on the condition and action you’ve provided.
When choosing between Drools, another BRMS, or manual coding, consider factors like the complexity of your rules, the size of your project, and your team’s familiarity with the tools. Each approach has its benefits and drawbacks, so choose the one that best fits your needs.
Troubleshooting Common Issues
Using Drools in Java can sometimes present challenges. Let’s discuss some common issues you might encounter and their solutions, along with best practices to ensure smooth operation.
Issue: Rules Not Firing
One of the most common issues is that rules sometimes don’t fire as expected. This could be due to a variety of reasons, such as a mistake in the rule conditions or a problem with the data provided.
// Rule that should fire but doesn't
rule "Rule1"
when
// condition
then
// action
end
ksession.fireAllRules();
# Output:
# (No output, as the rule didn't fire)
In this example, Rule1 should fire based on the condition, but it doesn’t. The solution could be to review the rule condition and the data provided to ensure they match.
Issue: Rule Conflicts
Another common issue is rule conflicts, where multiple rules are eligible to fire at the same time. Drools has conflict resolution strategies to handle this, but it’s essential to understand them to avoid unexpected results.
// Two conflicting rules
rule "Rule1"
when
// condition
then
// action
end
rule "Rule2"
when
// condition
then
// action
end
ksession.fireAllRules();
# Output:
# (Depends on the conflict resolution strategy)
In this example, both Rule1 and Rule2 are eligible to fire. The output will depend on the conflict resolution strategy used. Understanding these strategies can help you manage rule conflicts effectively.
Best Practices
To avoid these and other issues, follow best practices like writing clear and unambiguous rule conditions, testing your rules thoroughly, and understanding Drools’ conflict resolution strategies. With these tips in mind, you can use Drools in Java effectively and efficiently.
Understanding Drools and Java
Before we dive deeper into how to use Drools in Java, let’s take a step back and understand what Drools is, how it works, and why Java is relevant in business rule management.
What is Drools?
Drools is a Business Rule Management System (BRMS) solution. It provides a core Business Rules Engine (BRE), a web authoring and rules management application (Drools Workbench), and an Eclipse IDE plugin for core development.
// Basic structure of a rule in Drools
rule "Rule1"
when
// condition
then
// action
end
In this example, we see the basic structure of a rule in Drools. It includes a when
part (the condition under which the rule fires) and a then
part (the action taken when the rule fires).
How Does Drools Work?
Drools operates on the principle of rule-based programming. It uses a forward and backward chaining inference based algorithm. Forward chaining is data-driven and starts with the available data to infer what can be concluded from it, while backward chaining is goal-driven and starts with the end goal, working backward to determine what data is needed to achieve that goal.
Java and Business Rule Management
Java plays a crucial role in business rule management. Its robustness, ease of use, and wide range of libraries make it a popular choice for developers. When combined with a powerful BRMS like Drools, Java can help manage complex business rules efficiently and effectively.
Expanding Your Horizons: Drools and Java in Larger Projects
Drools and Java are not just for small projects or simple rule management. They can be used together in larger, more complex projects, providing a powerful, scalable solution for business rule management.
Leveraging Drools in Complex Java Projects
In larger projects, you might encounter complex rule sets, multiple rule sources, and the need for dynamic rule updates. Drools provides features like rule templates, decision tables, and dynamic rule updates to handle these scenarios.
// Example of a complex rule set
rule "Complex Rule"
when
// complex condition
then
// complex action
end
ksession.fireAllRules();
# Output:
# (Depends on the complex rule set and the data provided)
In this example, we have a complex rule set that includes multiple conditions and actions. The output will depend on the rule set and the data provided.
Further Resources for Mastering Drools in Java
To continue your journey in mastering Drools in Java, here are some resources that you might find helpful:
- IOFlood’s Article on Java Packages explains how Java packages help code organization and readability.
Jython Usage Cases – Explore using of Jython for scripting and extending Java applications with Python functionality.
Exploring Hibernate Framework – How Hibernate enables database interaction via plain Java objects and annotations.
The Official Drools Documentation is a comprehensive resource on all aspects of Drools.
Java Code Geeks Drools Tutorial provides a practical, hands-on approach to learning Drools.
Baeldung’s Guide on Drools helps you understand how to use features and techniques in Drools.
By understanding how to use Drools and Java in larger projects and exploring further resources, you can become a master at managing business rules in Java applications.
Wrapping Up: Mastering Drools in Java
In this comprehensive guide, we’ve delved into the world of using Drools in Java, a powerful combination for managing business rules effectively and efficiently.
We started with the basics, understanding how to create a KnowledgeBase and a StatefulSession, and how to load and fire rules. We then ventured into more advanced territory, exploring complex scenarios like handling rule conflicts and using decision tables.
Along the way, we tackled common challenges you might encounter when using Drools in Java, such as rules not firing as expected and rule conflicts. We provided solutions and best practices to help you overcome these hurdles.
We also discussed alternative approaches to business rule management in Java, comparing Drools with other BRMS solutions and manual coding. Here’s a quick comparison of these approaches:
Approach | Flexibility | Complexity | Control |
---|---|---|---|
Drools | High | Moderate | Moderate |
Other BRMS Solutions | Moderate | Low | Low |
Manual Coding | Low | High | High |
Whether you’re just starting out with Drools in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to use Drools in Java effectively.
With its balance of flexibility, moderate complexity, and control, Drools is a powerful tool for business rule management in Java. Now, you’re well equipped to leverage these benefits in your Java applications. Happy coding!