The Java main() Method: Basics to Advanced Usage
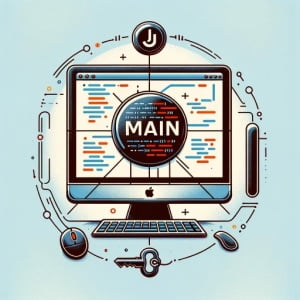
Are you finding it challenging to understand the Java main method? You’re not alone. Many developers, especially beginners, find it a bit daunting. Think of the Java main method as the ‘ignition key’ of your Java program – it’s the entry point that gets your code running.
The Java main method is crucial in any Java application. It’s where the Java Virtual Machine (JVM) starts executing your program. Understanding how it works and how to use it effectively is a vital skill for any Java developer.
In this guide, we’ll walk you through the ins and outs of the Java main method, from basic usage to advanced techniques. We’ll cover everything from the syntax and components of the main method, how to use command-line arguments, alternative ways to structure a Java program, to troubleshooting common issues.
So, let’s dive in and start mastering the Java main method!
TL;DR: What is the Java main method?
The Java main method is the entry point of any Java application. The most common method to call main is
public static void main(String[] args)
It’s the starting point where the Java Virtual Machine (JVM) begins executing your program. Here’s a simple example:
public static void main(String[] args) {
System.out.println('Hello, World!');
}
# Output:
# 'Hello, World!'
In this example, we’ve defined a main method that prints ‘Hello, World!’ to the console. The public static void main(String[] args)
line is the standard declaration for the main method in Java. The JVM looks for this method signature to start the program.
This is a basic way to use the Java main method, but there’s much more to learn about it. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- The Basics of Java Main Method
- Leveraging Command-Line Arguments in Java Main Method
- Exploring Alternative Approaches to Java Main Method
- Troubleshooting Common Java Main Method Errors
- Understanding the JVM and Java Main Method
- The Role of Java Main Method in Larger Applications
- Wrapping Up: Mastering the Java Main Method
The Basics of Java Main Method
The Java main method is the entry point of any Java application. When you run a Java program, the Java Virtual Machine (JVM) looks for the main method to start the program. The main method must have a specific signature to be recognized by the JVM.
Here’s the syntax of the main method:
public static void main(String[] args) {
// your code goes here
}
Let’s break down the components of this syntax:
public
: This is an access modifier that means the main method is accessible everywhere.static
: This keyword means the main method belongs to the class, not an instance of the class. Thus, the JVM can call it without creating an instance of the class.void
: This keyword indicates that the main method doesn’t return anything.main
: This is the name of the method. The JVM looks for this method to start the program.String[] args
: This is an array of Strings passed as command-line arguments.
Here’s a simple example of a Java program with a main method:
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
In this example, the main method prints ‘Hello, World!’ to the console. When you run this program, the JVM calls the main method, and ‘Hello, World!’ gets printed on your console.
The main method is crucial in any Java program. Without it, the JVM wouldn’t know where to start executing your program. It’s like the ‘ignition key’ of your Java program.
Leveraging Command-Line Arguments in Java Main Method
The Java main method isn’t just the starting point of your Java program. It also provides a way to accept input from the user through command-line arguments. These are values that you can pass to your program at the time of execution.
The command-line arguments are represented as an array of Strings (String[] args
) in the main method signature. The args
array is created and populated by the Java runtime system when your program starts. Each value in the args
array is a separate command-line argument.
Here’s a simple example to demonstrate how command-line arguments work:
public class CommandLineArgs {
public static void main(String[] args) {
for (String arg : args) {
System.out.println(arg);
}
}
}
# If you run this program with the command-line arguments 'Hello' and 'World', the output will be:
# 'Hello'
# 'World'
In this example, the main method iterates over the args
array and prints each command-line argument to the console. When you run this program with ‘Hello’ and ‘World’ as command-line arguments, it prints ‘Hello’ and ‘World’ on separate lines.
Command-line arguments are a powerful feature of the Java main method. They allow you to customize the behavior of your program at runtime without changing the code.
Exploring Alternative Approaches to Java Main Method
While the standard public static void main(String[] args)
method is the most common entry point for Java applications, there are alternative ways to structure your Java program. Let’s explore some of these approaches.
Non-Static Main Method
It’s possible to have a non-static main method in your Java class, but you should be aware that the JVM won’t recognize this as the entry point of your application. Here’s an example:
public class NonStaticMain {
public void main(String[] args) {
System.out.println('Hello, World!');
}
}
# If you try to run this program, the JVM will throw an error:
# 'Main method not found in class NonStaticMain'
In this example, we’ve defined a main method that’s not static. When you try to run this program, the JVM can’t find the main method because it’s looking for a static main method.
Multiple Main Methods
A Java program can have multiple main methods across different classes. This can be useful in large applications where different entry points are needed. Here’s an example:
public class MainMethod1 {
public static void main(String[] args) {
System.out.println('Hello from MainMethod1!');
}
}
public class MainMethod2 {
public static void main(String[] args) {
System.out.println('Hello from MainMethod2!');
}
}
# If you run MainMethod1, the output will be:
# 'Hello from MainMethod1!'
# If you run MainMethod2, the output will be:
# 'Hello from MainMethod2!'
In this example, we have two classes, each with its own main method. You can run each class separately, and the JVM will start the program from the main method of the class you run.
These alternative approaches offer flexibility in structuring your Java program. However, they come with their own set of considerations. For instance, a non-static main method won’t be recognized by the JVM as the entry point of your application, and having multiple main methods can make your program more complex to understand and maintain.
Troubleshooting Common Java Main Method Errors
Even with the best understanding of the Java main method, you might encounter some common errors. Let’s discuss these errors and how to solve them.
‘Main Method Not Found’ Error
This error typically occurs when the JVM can’t find a main method with the correct signature. Here’s an example of a program that will produce this error:
public class MainMethodError {
public void main(String[] args) {
System.out.println('Hello, World!');
}
}
# If you try to run this program, the JVM will throw an error:
# 'Main method not found in class MainMethodError'
In this example, the main method is not static, so the JVM can’t find it. To solve this error, you need to make the main method static:
public class MainMethodFixed {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# If you run this program, the output will be:
# 'Hello, World!'
In the fixed program, the main method is static, so the JVM can find it and start the program.
Best Practices for the Java Main Method
Here are some best practices for using the Java main method:
- Always make the main method static. This allows the JVM to call it without creating an instance of the class.
- Keep the main method clean and concise. It should only contain the logic needed to start your program. Any additional logic should be placed in separate methods or classes.
- If your program needs to accept user input, consider using command-line arguments. They provide a flexible way to pass values to your program at runtime.
By following these best practices and understanding how to troubleshoot common errors, you can use the Java main method more effectively in your programs.
Understanding the JVM and Java Main Method
To fully understand the Java main method, it’s crucial to have a deeper understanding of the Java Virtual Machine (JVM) and the concepts of ‘static’, ‘void’, and ‘public’.
The Role of JVM in Java Main Method
The JVM is an abstract computing machine that enables a computer to run a Java program. When you run a Java program, the JVM is responsible for loading the class files and starting the execution from the main method.
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
In this example, when you run the HelloWorld
class, the JVM loads the HelloWorld
class into memory, finds the main method, and starts executing the program from there.
The ‘static’, ‘void’, and ‘public’ Keywords
The main method in Java is typically declared as public static void main(String[] args)
. Each of these keywords plays a crucial role:
public
: This keyword means the main method is accessible everywhere, including from outside the class. This is necessary because the main method is called by the JVM, which is outside the scope of the class.static
: This keyword allows the JVM to call the main method without creating an instance of the class. If the main method wasn’t static, the JVM would need to instantiate the class before calling the main method, which isn’t desirable because the main method needs to start executing as soon as the class is loaded.void
: This keyword indicates that the main method doesn’t return a value. This is because the main method is the starting point of the program, not a method to perform a calculation and return a result.
Understanding these fundamentals is key to mastering the Java main method. It helps you understand not just how to use the main method, but also why it’s designed the way it is.
The Role of Java Main Method in Larger Applications
The Java main method is not just the starting point of small Java programs; it also plays a crucial role in larger Java applications. In a large application, the main method can be used to initialize the application, load configuration settings, start threads, or perform any other setup tasks required by the application.
Exploring Related Topics
Understanding the Java main method is a great start, but there’s much more to learn in Java. Here are some related topics that you might find interesting:
- Java Object-Oriented Programming (OOP) Concepts: Java is an object-oriented programming language. Learning about OOP concepts like classes, objects, inheritance, polymorphism, and encapsulation can help you write more efficient and maintainable Java programs.
Java Threads: Threads are a fundamental part of concurrent programming in Java. Understanding how to create and manage threads can help you write programs that perform multiple tasks simultaneously.
Further Resources for Mastering Java
Ready to dive deeper into Java? Here are some resources that you might find helpful:
- Java Methods Tutorial: Best Practices and Tips – Discover comprehensive guidance on Java methods for all levels.
Simple Method Invocation in Java explore different techniques to invoke methods in Java.
Main Method Syntax in Java – Master the main method in Java for starting Java programs.
Oracle’s official Java Tutorials cover basic to advanced topics of Java programming.
Java Code Geeks offers tutorials and articles on the Java main method, threads, and more.
Baeldung’s Guide to Java covers the Java core language, Spring framework, and more.
Wrapping Up: Mastering the Java Main Method
In this comprehensive guide, we’ve delved into the Java main method, a critical component in any Java application. We’ve explored its role as the entry point of Java programs and how it interacts with the Java Virtual Machine (JVM).
We began with the basics, understanding the syntax and components of the main method and how to use it in a simple Java program. We then delved into more advanced usage, learning how to leverage command-line arguments to pass values to the program at runtime.
We also explored alternative approaches to the Java main method, such as using non-static methods or having multiple main methods across different classes. We discussed the benefits and drawbacks of these approaches, helping you understand when to use them.
We tackled common issues you might encounter, such as the ‘Main method not found’ error, and provided solutions to help you troubleshoot these challenges. We also provided best practices for using the Java main method effectively in your programs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Standard Main Method | Recognized by JVM, can accept command-line arguments | None |
Non-Static Main Method | Can be part of an instance of a class | Not recognized by JVM as the program’s entry point |
Multiple Main Methods | Provides multiple entry points for large applications | Can make the program more complex |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of the language, we hope this guide has given you a more profound understanding of the Java main method and its role in Java applications.
With this knowledge, you’re now equipped to write more efficient and effective Java programs. Happy coding!