XOR in Python: Usage Guide to Bitwise XOR
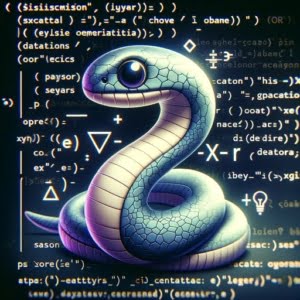
XOR, standing for ‘exclusive or’, is a type of bitwise operation that’s a potent tool in the Python toolbox. It can open up a whole new realm of coding opportunities.
XOR operations are a fundamental part of Python programming. They’re utilized in a wide array of applications, from data manipulation to error detection and cryptography, making them an indispensable skill for any serious Python programmer.
By the end of this guide, you’ll have a comprehensive understanding of what XOR operations are, how to implement them in Python, and their practical applications in real-world programming scenarios. So, let’s embark on this journey and explore the fascinating world of XOR operations in Python.
TL;DR: What are XOR Operations in Python?
XOR (exclusive or) operations in Python are a type of bitwise operation that return ‘true’ if the inputs are different and ‘false’ if the inputs are the same. They are represented using the caret operator (^). XOR operations are used in various aspects of programming, including data manipulation, error detection, and cryptography. For a direct example of XOR operation:
# XOR operation of 10 and 6
result = 10 ^ 6
print(result) # Outputs: 12
For more advanced methods, background, tips, and tricks on XOR operations in Python, continue reading the article.
Table of Contents
XOR in Python: An Overview
If you’ve come across XOR (exclusive OR), don’t be taken aback by the term. In Python, XOR is a type of bitwise operation primarily used with binary numbers. But what does this signify, and why is it crucial?
Let’s break it down to the basics. XOR is a logical operation that takes two binary inputs and returns a binary output. The output is ‘true’ if the inputs differ (one is true, and the other is false), and ‘false’ if the inputs are identical (both true or both false).
In Python, XOR operations are signified using the caret operator (^). For instance, if we have two binary numbers, 1010 (10 in decimal) and 0110 (6 in decimal), the XOR operation would resemble this:
Bit Value (Decimal) | 8 | 4 | 2 | 1 |
---|---|---|---|---|
10 (1010) | 1 | 0 | 1 | 0 |
6 (0110) | 0 | 1 | 1 | 0 |
XOR Result (1100) | 1 | 1 | 0 | 0 |
XOR Carat Syntax
You would execute this XOR operation as follows:
10 ^ 6
The result of this operation will be 12. But why?
Python initially converts the integer values into binary format. So, 10 becomes 1010 and 6 becomes 0110. Subsequently, it performs the XOR operation on each pair of bits. The result is 1100, which is 12 in decimal format. I.E. as you add together the bit value of each resulting “1” bit, you get 8 + 4 = 12.
Uses for XOR
XOR operations have a pivotal role in various facets of computing and programming. They are employed in error detection to ensure data integrity during transmission. In cryptography, XOR operations are used for data encryption and decryption. And in data manipulation, XOR operations can be used to toggle bits in a binary number, among other uses.
Perhaps the most intriguing aspect of XOR operations is their problem-solving potential. They can be utilized to find a missing number in an array, detect duplicate elements, and more. Plus, XOR operations in Python aren’t restricted to integers. They can also be applied to boolean values (True and False), making them a versatile tool in a programmer’s toolkit.
XOR Syntax and Implementation in Python
Having introduced XOR operations via the ^ carat operator, it’s time to delve deeper into their syntax and how they are implemented in Python.
XOR operations aren’t exclusively for integers. You can also craft a custom XOR function in Python to handle boolean values.
Consider a function that checks for two conditions: either the first variable is true and the second is false, or the first is false and the second is true. Here’s an example:
def xor(a, b):
return (a and not b) or (not a and b)
print(xor(True, False)) # Outputs: True
print(xor(True, True)) # Outputs: False
In this code, the xor
function returns True
if the inputs are different and False
if they are the same, mirroring the XOR operation with binary numbers.
A unique aspect of XOR operations is their potential for data comparison. For instance, you can use an XOR operation to determine whether two integers are equal without using the equality operator (==
). If the XOR operation results in 0, the integers are equal. If not, they differ. This can be particularly handy in situations where you need to compare data without revealing the data itself.
Built-in XOR Function
Python also provides a built-in function, functools.reduce()
, to perform XOR operations on a list of numbers.
Here’s a breakdown of the XOR operation on the list of numbers:
| Step | Operation | Result |
|—|—|—|
| 1 | 10 ^ 6 | 12 |
| 2 | 12 ^ 12 | 0 |
This function applies a binary function (in this case, XOR) to all elements of an iterable (like a list) in a cumulative manner.
import functools
# XOR of a list of numbers
numbers = [10, 6, 12]
result = functools.reduce(lambda x, y: x ^ y, numbers)
print(result) # Outputs: 10
The output of XOR operations can shift depending on the input types. For instance, the bitwise XOR operator (^) returns an integer when used with integers, and a boolean when used with booleans.
XOR with NumPy
XOR operations in Python extend beyond basic data manipulation and comparison. They can be employed for advanced techniques, including working with NumPy arrays, executing mathematical operations, and implementing sequences and protocols. Let’s delve deeper into these advanced XOR techniques.
NumPy, a potent library for numerical computing in Python, supports XOR operations on arrays. This becomes incredibly useful when you need to perform element-wise XOR operations on extensive datasets.
import numpy as np
# XOR of two NumPy arrays
a = np.array([True, False, True, False])
b = np.array([True, True, False, False])
result = np.logical_xor(a, b)
Here's a breakdown of the element-wise XOR operation on the arrays:
| Index | a | b | XOR Result |
|---|---|---|---|
| 0 | True | True | False |
| 1 | False | True | True |
| 2 | True | False | True |
| 3 | False | False | False |
print(result) # Outputs: [False True True False]
XOR for Swapping Variables
Did you know that you can use XOR operations to swap two numbers without needing an additional variable? This operation is performed at the bit level, providing an efficient method for swapping values. Here’s how you can do it in Python:
# XOR swap algorithm
a = 10
b = 6
a = a ^ b
b = a ^ b
a = a ^ b
print('a =', a) # Outputs: a = 6
print('b =', b) # Outputs: b = 10
In this code, the XOR operator records the positions where the values of the two variables differ, permitting the swapping of integer variables without the need for a temporary variable.
When working with XOR operations, it’s important to remember the significance of data types. XOR operations work with binary numbers, which in Python can be represented as integers or booleans. Using the correct data type for your XOR operations is crucial for getting the desired result.
Error Handling in XOR Operations
One prevalent error when working with XOR operations is the TypeError
. This error is triggered when you attempt to perform an XOR operation on incompatible data types, such as an integer and a string.
For instance, the following code will raise a TypeError
:
result = 10 ^ '6' # Raises TypeError: unsupported operand type(s) for ^: 'int' and 'str'
To remedy this error, you can employ a try...except
block. This allows you to catch the error and take appropriate action, such as converting the string to an integer or alerting the user about the incorrect input.
try:
result = 10 ^ '6'
except TypeError:
print('Error: XOR operation requires two integers or two booleans.')
This code will catch the TypeError
and print a helpful error message instead of crashing the program.
Further Resources for Python Conditionals
To amplify your command of Python conditional statements, the following resources are rich with information:
- Python If Statement Flow Control – Explore if statements and list comprehensions for concise data filtering.
Python’s “or” Operator – A guide to combining boolean values using “or” in Python
“not” in Python – Dive into advanced conditional logic with “not” to verify if an item is not present in a collection.
The Official Python Documentation: Boolean Operations provides an overview of booleans and operators in Python.
Conditional Statements in Python on Medium – A Medium article, discussing the use of conditional statements in Python.
Python If-Else Statement – A FreeCodeCamp guide providing explanations on the use of “if-else” statements in Python.
Dive into these resources and bolster your skillset in Python conditional statements!
Wrapping Up
Throughout this comprehensive guide, we’ve navigated the intriguing world of XOR operations in Python. We’ve dissected the concept of XOR operations, their syntax, and the methods to implement them in Python.
We’ve also examined their practical applications in real-world programming scenarios, from rudimentary data manipulation and comparison to advanced techniques like working with NumPy arrays and executing mathematical operations.
Discover the nuances of Python language with our definitive syntax cheat sheet.
In conclusion, XOR operations are a potent tool in Python programming. By understanding and effectively using XOR operations, you can enhance your data manipulation and problem-solving capabilities, write more efficient and cleaner code, and elevate your Python programming skills.