Python’s ‘or’ Operator: A Comprehensive Guide
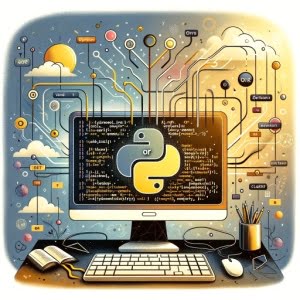
Ever found yourself grappling with the ‘or’ operator in Python? You’re not alone. This operator, much like a traffic cop at a bustling intersection, is crucial in guiding the flow of your code. But understanding how it works can sometimes feel like trying to decipher a foreign language.
Don’t worry, we’ve got you covered. This guide is designed to take you on a journey from the basics to the advanced usage of the ‘or’ operator in Python. Whether you’re a beginner just getting started or an experienced developer looking to deepen your understanding, there’s something here for you.
So buckle up, and let’s dive into the fascinating world of Python’s ‘or’ operator.
TL;DR: How Do I Use the ‘or’ Operator in Python?
The ‘or’ operator in Python is a logical operator that returns True if at least one of the conditions is True. If both conditions are False, it returns False.
Here’s a simple example:
x = 5
print(x > 3 or x < 4)
# Output:
# True
In this example, we are checking if x
is greater than 3 or less than 4. Since x
is 5, it is indeed greater than 3, so the condition x > 3 or x < 4
returns True. The ‘or’ operator only needs one condition to be True to return True.
Intrigued? Keep reading for a more detailed understanding and advanced usage scenarios of the ‘or’ operator in Python.
Table of Contents
- The ‘or’ Operator in Python: A Beginner’s Guide
- Exploring Complex Uses of Python’s ‘or’ Operator
- Alternative Techniques to Python’s ‘or’ Operator
- Troubleshooting Python’s ‘or’ Operator
- Unpacking Python’s Boolean Logic and Operator Precedence
- Broadening Your Understanding of Python’s ‘or’ Operator
- Python’s ‘or’ Operator: A Recap
The ‘or’ Operator in Python: A Beginner’s Guide
In Python, the ‘or’ operator is a logical operator that checks whether any of the conditions it is checking are True. If at least one condition is True, the ‘or’ operator returns True. If all conditions are False, it returns False. This is a fundamental aspect of Python’s boolean logic.
Let’s break down a simple example:
x = 7
y = 10
print(x > 8 or y > 8)
# Output:
# True
In this example, the ‘or’ operator is checking two conditions: x > 8
and y > 8
. Since y
is 10, which is greater than 8, the second condition is True. Therefore, even though the first condition x > 8
is False (because x
is 7), the ‘or’ operator returns True because at least one condition is True.
The ‘or’ operator is powerful because it allows you to check multiple conditions at once. However, it’s important to remember that it only needs one True condition to return True. This can sometimes lead to unexpected results if you’re not careful. Always remember to structure your conditions correctly when using the ‘or’ operator.
Exploring Complex Uses of Python’s ‘or’ Operator
As you get more comfortable with Python, you’ll discover that the ‘or’ operator is not just limited to numbers or boolean values. It can also be used with different data types, adding another layer of flexibility to your code.
Let’s look at a scenario where we use the ‘or’ operator with strings:
name = ''
default_name = 'Guest'
greeting = name or default_name
print(f'Hello, {greeting}!')
# Output:
# Hello, Guest!
In this code, we’re using the ‘or’ operator to check if the variable name
has a value. If name
is an empty string (which Python interprets as False), the ‘or’ operator will return default_name
as the value for greeting
. Hence, the output is ‘Hello, Guest!’. This is a great way to set default values in your code.
The ‘or’ operator can also be used with lists, dictionaries, and other data types. The key takeaway is that the ‘or’ operator is a powerful tool that can handle more than just simple True or False conditions. As you continue to explore Python, you’ll find even more ways to use this versatile operator.
Alternative Techniques to Python’s ‘or’ Operator
While the ‘or’ operator is a powerful tool in Python, it’s not the only way to check multiple conditions. Python provides other functions and techniques that can be used as alternatives, depending on your specific needs. One such method is the any()
function.
The any()
function takes an iterable (like a list or a tuple) and returns True if at least one element in the iterable is True. If all elements are False, it returns False. This function is similar to the ‘or’ operator, but it can be more efficient when dealing with large data sets.
Here’s an example of how to use the any()
function:
numbers = [False, 0, 0.0, '', [], {}, None]
print(any(numbers))
# Output:
# False
In this code, we’re passing a list of values to the any()
function. All of these values are interpreted as False in Python, so the function returns False.
The any()
function is a powerful tool, but it’s not always the best choice. It’s great for checking large data sets, but for simple conditions, the ‘or’ operator is usually more straightforward and easier to read.
When deciding between the ‘or’ operator and the any()
function, consider the complexity of your conditions and the size of your data. Both tools have their place in Python, and understanding when to use each one is a key skill for any Python developer.
Troubleshooting Python’s ‘or’ Operator
As with any tool, using Python’s ‘or’ operator can sometimes lead to unexpected results. One common issue is misunderstanding operator precedence, which can lead to confusing outcomes.
Operator Precedence Pitfalls
Operator precedence refers to the order in which Python evaluates different operators in an expression. For example, the multiplication operator (*
) has higher precedence than the addition operator (+
). This means that in the expression 2 + 3 * 4
, Python will first perform the multiplication, then the addition, resulting in 14
, not 20
.
Similarly, logical operators like ‘or’ also have a specific precedence. The ‘or’ operator has lower precedence than the ‘and’ operator. This means that in an expression with both ‘and’ and ‘or’, the ‘and’ operation will be performed first.
Consider this code block:
x = True
y = False
z = False
print(x or y and z)
# Output:
# True
At first glance, you might expect the output to be False
because y and z
is False
. However, due to operator precedence, the ‘and’ operation is performed first, then the ‘or’ operation. So the expression is equivalent to x or (y and z)
, which evaluates to True
because x
is True
.
To avoid confusion, it’s a good practice to use parentheses to explicitly specify the order of operations when using ‘or’ and ‘and’ in the same expression.
x = True
y = False
z = False
print((x or y) and z)
# Output:
# False
In this code, (x or y)
is evaluated first due to the parentheses, then the ‘and’ operation is performed. The result is False
.
Understanding operator precedence is crucial when working with Python’s ‘or’ operator. By keeping these considerations in mind, you can avoid common pitfalls and write more accurate and efficient code.
Unpacking Python’s Boolean Logic and Operator Precedence
To fully grasp the ‘or’ operator in Python, it’s crucial to understand the underlying concepts of boolean logic and operator precedence.
Python’s Boolean Logic
In Python, boolean logic refers to True and False values and the operations you can perform with them. The ‘or’ operator is a key part of this logic. It allows you to check multiple conditions and returns True if at least one of the conditions is True.
Consider this example:
x = 10
y = 20
print(x < 15 or y > 25)
# Output:
# True
In this code, the ‘or’ operator checks two conditions: x < 15
and y > 25
. Even though y > 25
is False, the entire expression is True because x < 15
is True.
Understanding Operator Precedence
Operator precedence is the order in which Python evaluates different operators in an expression. For example, in an expression like 2 + 3 * 4
, Python first performs the multiplication due to its higher precedence, resulting in 2 + 12
, which equals 14
.
Similarly, in boolean logic, the ‘and’ operator has higher precedence than the ‘or’ operator. This means that in an expression with both ‘and’ and ‘or’, the ‘and’ operation will be performed first.
Here’s an example to illustrate this:
x = True
y = False
z = True
print(x or y and z)
# Output:
# True
Even though y and z
is False, the entire expression is True because x
is True. This might seem counterintuitive at first, but it’s due to the ‘and’ operator’s higher precedence. The expression x or y and z
is equivalent to x or (y and z)
.
Understanding Python’s boolean logic and operator precedence is key to mastering the ‘or’ operator. With these fundamentals in mind, you’ll be better equipped to write and debug Python code.
Broadening Your Understanding of Python’s ‘or’ Operator
The ‘or’ operator in Python is more than just a tool for checking multiple conditions. It plays a crucial role in many aspects of Python programming, including control flow and conditional statements.
Control Flow and the ‘or’ Operator
Control flow is the order in which your code executes. The ‘or’ operator can significantly impact this flow. For example, in an ‘or’ operation, Python uses short-circuit evaluation. This means it will stop evaluating as soon as it finds a True condition.
Consider this code block:
x = 10
y = 0
print(x != 0 or y != 0)
# Output:
# True
In this code, Python doesn’t even check the second condition y != 0
because the first condition x != 0
is True. This can be useful for optimizing your code and avoiding errors.
Exploring Related Concepts
The ‘or’ operator is part of a trio of logical operators in Python, which also includes the ‘and’ and ‘not’ operators. These operators are all essential tools for handling boolean logic in Python.
The ‘and’ operator returns True only if all conditions are True, while the ‘not’ operator negates the truth value of the condition it’s applied to. Understanding these operators along with ‘or’ will give you a solid foundation in Python’s boolean logic.
x = True
y = False
print(not x)
print(x and y)
print(x or y)
# Output:
# False
# False
# True
In this code, not x
returns False, x and y
returns False, and x or y
returns True.
By exploring these related concepts, you can deepen your understanding of Python and become a more versatile programmer. We encourage you to continue learning and experimenting with these operators, and to seek out more resources to expand your Python knowledge.
Further Resources for Python Conditionals
If you’re eager to enhance your understanding of Python conditional statements, the resources outlined below provide valuable insights:
- Python If Statement Tutorial – Explore writing conditional code using if statements in Python.
Python “else if” Statements – Learn how to manage multiple conditions using “else if” constructs.
Bitwise XOR in Python – A guide on the bitwise XOR operator (^) in Python.
Real Python’s Guide to Python operators offers an overview of all types of operators in Python.
Python’s official tutorial on control flow tools discusses the wide variety of control flow tools.
Elif Statements Python Tutorial – A DataCamp tutorial focusing on the use of ‘elif’ statements in Python.
By exploring these resources and honing your skills in Python conditional statements, you will increase your competence as a Python programmer.
Python’s ‘or’ Operator: A Recap
We’ve embarked on a comprehensive journey through Python’s ‘or’ operator, exploring its basic and advanced uses, alternative approaches, and common pitfalls. We’ve seen how the ‘or’ operator acts as a traffic cop, directing the flow of your code based on multiple conditions.
We’ve also delved into the heart of Python’s boolean logic, understanding how the ‘or’ operator works with True and False values. We’ve tackled operator precedence, learning how it can impact the results of our ‘or’ operations.
Moreover, we’ve explored alternative techniques like the any()
function, which can be more efficient for large data sets, and we’ve seen how to troubleshoot common issues with the ‘or’ operator.
To sum it up, here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Example |
---|---|---|
‘or’ Operator | Checking multiple conditions, setting default values | x > 3 or y < 4 |
any() Function | Checking large data sets | any([x > 3, y < 4, z < 5]) |
Remember, the ‘or’ operator is a powerful tool in your Python toolkit, but it’s not the only one. Understanding when to use ‘or’ and when to use alternative approaches is a key skill for any Python developer.
We hope this guide has been helpful in your journey to mastering the ‘or’ operator in Python. Keep experimenting, keep learning, and keep coding!