Python Yield | Keyword Guide (With Examples)
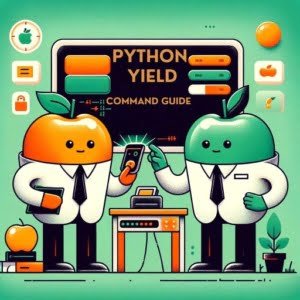
The ‘yield’ keyword in Python programming is crucial while automating tasks at IOFLOOD, facilitating the creation of generators and lazy evaluation of data. In our experience, ‘yield’ enhances memory efficiency and streamlines data processing, particularly in scenarios involving large datasets or infinite sequences. Today’s article explores what ‘yield’ does in Python, providing examples and explanations to empower our dedicated server hosting customers with valuable scripting techniques
This guide is your roadmap to mastering the use of ‘yield’ in Python. We’ll start from the basics and gradually delve into more advanced techniques. By the end of this journey, you’ll be wielding ‘yield’ like a true Python wizard!
So without further ado, let’s get started with generator functions in Python!
TL;DR: What does ‘yield’ do in Python?’
Yield
in Python is used to define a generator function, which can produce a sequence of results instead of a single value.
Let’s see it in action with a simple example:
def simple_generator():
yield 1
yield 2
yield 3
for value in simple_generator():
print(value)
# Output:
# 1
# 2
# 3
The function simple_generator
is not just a regular function. It’s a generator function, thanks to the ‘yield’ keyword. When we loop over simple_generator()
, it yields the numbers 1, 2, and 3, one at a time. This is just scratching the surface of Python’s ‘yield’. Continue reading for a deeper dive into more advanced usage scenarios and a comprehensive understanding of this powerful keyword.
Table of Contents
- The Fundamentals of Python’s ‘yield’
- Python Yield: Advanced Techniques
- Exploring Alternatives to Python’s ‘yield’
- Navigating Challenges with Python’s ‘yield’
- Understanding Generators and Iteration in Python
- Leveraging ‘yield’ in Large-Scale Python Projects
- Further Reading: Coroutines and the ‘itertools’ Module
- Python Yield: A Powerful Tool for Generators
The Fundamentals of Python’s ‘yield’
In Python, ‘yield’ is the keyword that gives a function the power to become a generator. But what does that mean, exactly? Let’s break it down with a simple example.
def simple_generator():
yield 'Hello'
yield 'World'
gen = simple_generator()
print(next(gen))
print(next(gen))
# Output:
# Hello
# World
In this code, simple_generator
is a generator function. We use the ‘yield’ keyword to produce values one at a time. When we call next(gen)
, it runs the function until it hits a ‘yield’ statement, then pauses and returns the yielded value. The next time we call next(gen)
, it picks up where it left off and continues running until it hits the next ‘yield’ statement.
Advantages and Pitfalls of ‘yield’
One of the main advantages of using ‘yield’ is that it allows us to handle large data sets efficiently. When we use a generator function with ‘yield’, it doesn’t store all the values in memory at once. Instead, it generates them on the fly as we need them. This can be a huge advantage when working with large data sets.
However, there’s a potential pitfall to keep in mind. Once a generator function has yielded all of its values, it can’t be reused. If you try to get more values out of it, you’ll get a StopIteration
exception. This is because a generator function maintains its state only until it has yielded all its values.
Python Yield: Advanced Techniques
As we delve deeper into the world of Python’s ‘yield’, we encounter more complex and intriguing uses. Let’s explore two of these advanced techniques: creating infinite sequences and using ‘yield’ in recursive functions.
Infinite Sequences with ‘yield’
‘Yield’ can be used to create infinite sequences. This is possible because a generator function only generates the next value when it’s needed. Here’s an example of an infinite sequence generator:
def infinite_sequence():
num = 0
while True:
yield num
num += 1
gen = infinite_sequence()
print(next(gen)) # Output: 0
print(next(gen)) # Output: 1
print(next(gen)) # Output: 2
# ... and so on, infinitely
In this code, the infinite_sequence
generator function yields an infinite sequence of numbers. Each time we call next(gen)
, it gives us the next number in the sequence.
Recursive Functions and ‘yield’
‘Yield’ can also be used in recursive functions to create more complex sequences. Here’s an example of a function that generates the Fibonacci sequence:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
gen = fibonacci()
print(next(gen)) # Output: 0
print(next(gen)) # Output: 1
print(next(gen)) # Output: 1
print(next(gen)) # Output: 2
# ... and so on, following the Fibonacci sequence
In this code, the fibonacci
generator function uses ‘yield’ to produce the Fibonacci sequence. Each number is the sum of the two preceding ones, starting from 0 and 1.
These advanced techniques show the power and flexibility of Python’s ‘yield’. However, they also come with their own set of challenges. For instance, when creating infinite sequences, we must be careful to avoid entering an infinite loop. And when using ‘yield’ in recursive functions, we must ensure that the recursion has a base case to prevent it from running indefinitely.
Exploring Alternatives to Python’s ‘yield’
While ‘yield’ is a powerful tool for creating generators in Python, it’s not the only method. Let’s explore two alternative approaches: generator expressions and built-in functions like ‘range’.
Generator Expressions: The Compact Alternative
Generator expressions are a high-performance, memory-efficient generalization of list comprehensions and lambdas. Here’s an example of a generator expression that produces the same sequence as our simple_generator
function from earlier:
gen = (i for i in ['Hello', 'World'])
print(next(gen)) # Output: Hello
print(next(gen)) # Output: World
This generator expression does the same thing as the simple_generator
function, but in a more compact form. However, generator expressions can be less flexible and harder to read when they get complex.
Built-in Functions: Python’s ‘range’
Python’s built-in range
function is another way to create generators. range
generates a sequence of numbers and is often used in loops. Here’s an example:
for i in range(3):
print(i)
# Output:
# 0
# 1
# 2
In this code, range(3)
generates the numbers 0, 1, and 2. It’s a simple and efficient way to create a sequence of numbers, but it’s less flexible than ‘yield’ because it can only generate sequences of numbers.
In conclusion, while ‘yield’ is a powerful and flexible tool for creating generators in Python, generator expressions and built-in functions like ‘range’ offer alternative approaches. The best method to use depends on the specific needs of your code.
While ‘yield’ is a powerful tool for creating generators in Python, it’s not without its challenges. Let’s discuss some common issues and how to navigate them.
Understanding the ‘StopIteration’ Exception
One common issue when working with ‘yield’ is the StopIteration
exception. This happens when you try to get a value from a generator that has already yielded all its values. Here’s an example:
def simple_generator():
yield 'Hello'
yield 'World'
gen = simple_generator()
print(next(gen)) # Output: Hello
print(next(gen)) # Output: World
print(next(gen)) # Raises StopIteration
In this code, the simple_generator
function yields two values. When we try to get a third value with next(gen)
, it raises a StopIteration
exception because the generator has no more values to yield.
Handling ‘StopIteration’
One way to handle the StopIteration
exception is to use a loop to iterate over the generator. The loop will automatically stop when the generator runs out of values. Here’s an example:
def simple_generator():
yield 'Hello'
yield 'World'
for value in simple_generator():
print(value)
# Output:
# Hello
# World
In this code, the for
loop automatically stops when the simple_generator
function runs out of values. This prevents the StopIteration
exception from being raised.
Other Considerations
Another thing to keep in mind when using ‘yield’ is that generator functions maintain their state only until they have yielded all their values. Once a generator has been exhausted, it can’t be reused. If you need to use the same sequence of values again, you’ll need to create a new generator.
In conclusion, while ‘yield’ can be a powerful tool for creating generators in Python, it’s important to understand its potential pitfalls and how to navigate them.
Understanding Generators and Iteration in Python
Python’s ‘yield’ keyword is closely tied to the concept of generators and iteration. But what are these concepts, and how does ‘yield’ fit into them?
Generators: The Power Behind ‘yield’
In Python, a generator is a type of iterable, like a list or a tuple. But unlike lists or tuples, generators don’t store all their values in memory. Instead, they generate each value on-the-fly as you loop over them. This makes them much more memory-efficient when dealing with large sequences of data.
Here’s a simple example of a generator function:
def count_up_to(n):
num = 1
while num <= n:
yield num
num += 1
for num in count_up_to(5):
print(num)
# Output:
# 1
# 2
# 3
# 4
# 5
In this code, the count_up_to
function is a generator function. It uses the ‘yield’ keyword to produce a sequence of numbers from 1 up to n
. Each time we loop over the generator, it yields the next number in the sequence.
Iteration: The Process that Drives ‘yield’
Iteration is the process of looping over an iterable. When you use a for
loop to go over a list, you’re iterating over that list. When you use ‘yield’ in a function, you’re creating a generator that can be iterated over.
The power of ‘yield’ lies in its ability to pause the function’s execution after each ‘yield’ statement, and resume it the next time the generator’s next
method is called. This allows the function to produce a sequence of values over time, rather than calculating them all at once and returning them in a huge list.
In conclusion, ‘yield’ plays a crucial role in creating generators and enabling iteration in Python. It provides a memory-efficient way to produce large or even infinite sequences of data, making it a powerful tool in any Python programmer’s toolkit.
Leveraging ‘yield’ in Large-Scale Python Projects
The ‘yield’ keyword isn’t just for small scripts or simple sequences. It can also be a powerful tool in larger scripts or projects, especially when dealing with large data sets or creating data pipelines.
Data Pipelines with ‘yield’
In data analysis or machine learning projects, you often need to process large amounts of data. This data might need to be filtered, transformed, or otherwise processed before it can be used. ‘Yield’ can help you create efficient data pipelines for these tasks.
Here’s a simple example of a data pipeline that reads a large file line by line, filters out the empty lines, and yields the remaining lines one by one:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
if line.strip():
yield line
for line in read_large_file('large_file.txt'):
# process the line
pass
In this code, the read_large_file
function is a generator that reads a large file line by line. It uses ‘yield’ to produce the lines one at a time, allowing you to process each line individually without loading the entire file into memory.
‘yield’ and Large Data Sets
When working with large data sets, memory efficiency is a key concern. ‘Yield’ allows you to create generators that produce data on-the-fly as you loop over them, making them much more memory-efficient than lists or other iterables that store all their values in memory.
Further Reading: Coroutines and the ‘itertools’ Module
If you’re interested in diving deeper into the world of generators and iteration in Python, two topics you might want to explore are coroutines and the ‘itertools’ module.
Coroutines are a more advanced form of generators that can not only produce values with ‘yield’, but also consume values sent to them using the .send()
method. This makes them incredibly powerful for creating complex data pipelines or handling asynchronous tasks.
The ‘itertools’ module, on the other hand, provides a set of tools for creating and working with iterators. It includes functions for creating infinite iterators, cycling over iterables, and more.
Further Resources for Python Iterator Mastery
If you’re eager to expand your understanding of Python’s iterators, control statements and loops, look no further. Here are a few handpicked resources to stimulate your knowledge:
- Python Loop Use Cases Unveiled – Dive into Python’s itertools module for advanced looping techniques.
Simplifying Loops with Python “while” Loop – Explore how “while” loops handle conditions and control program flow.
Python “pass” Statement: Do Nothing with Purpose – Discover the “pass” statement in Python and its use as a placeholder.
Codecademy’s Python Loops Cheatsheet is an easy-to-reference cheatsheet on loops in Python.
Python Generators Explanation – Codecademy’s Python documentation explaining the functionality of generators in Python.
Control Structures in Python – A JavaTpoint tutorial that provides a comprehensive overview of control structures in Python.
By delving into these resources and enhancing your understanding of Python’s control structure you can become a more adept Python developer.
Python Yield: A Powerful Tool for Generators
Python’s ‘yield’ keyword is a powerful tool for creating generators. It allows a function to produce a sequence of results over time, rather than calculating them all at once and returning them in a list. This makes it incredibly memory-efficient, especially when dealing with large sequences of data.
From simple sequences to infinite and complex sequences, ‘yield’ offers a wide range of possibilities. It can be used in a simple function to create a generator, or in a recursive function to create more complex sequences. However, it also comes with challenges, such as understanding the StopIteration
exception and ensuring the generator does not run indefinitely.
While ‘yield’ is a powerful tool, it’s not the only way to create generators in Python. Generator expressions offer a more compact alternative, while built-in functions like ‘range’ provide a simple and efficient way to create sequences of numbers.
Working with ‘yield’ can sometimes lead to issues like the StopIteration
exception. This exception is raised when you try to get a value from a generator that has already yielded all its values. To handle this, you can use a loop to iterate over the generator, which will automatically stop when the generator runs out of values.
A Comparison of Generator Creation Methods:
Method | Flexibility | Memory Efficiency | Complexity |
---|---|---|---|
‘yield’ | High | High | Medium |
Generator expressions | Medium | High | Low |
‘range’ function | Low | High | Low |
In conclusion, ‘yield’ is a powerful and versatile tool in Python. Whether you’re creating a simple generator or a complex data pipeline, ‘yield’ can help you do it more efficiently and effectively.