Python ‘pass’ Statement | Guide (With Examples)
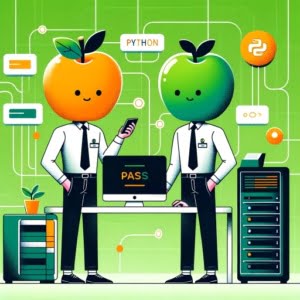
While programing software for use at IOFLOOD we have become accustomed to using the ‘pass’ statement in Python for placeholder code implementation. In our experience, the ‘pass’ statement serves as a placeholder for future code development, ensuring smooth syntax and logic flow. Today’s article delves into what the ‘pass’ statement is in Python, providing examples and insights to empower our dedicated hosting customers with effective coding practices on their cloud servers.
This article will guide you through the usage of the ‘pass’ statement, from basic to advanced levels. We’ll explore its syntax, its use cases, and some common pitfalls to avoid.
So let’s dive in and demystify Python’s ‘pass’ statement together.
TL;DR: What is the ‘pass’ statement in Python?
The
pass
statement in Python is a placeholder for future code. It does nothing when executed, but prevents errors in places where empty code is not allowed.
Here’s a simple example:
def my_function():
pass
my_function()
# Output:
# No output, because the function does nothing.
In this example, we’ve defined a function named ‘my_function’. Inside the function, we’ve placed the ‘pass’ statement as a placeholder. When we call ‘my_function()’, Python executes the ‘pass’ statement, which does nothing, and then moves on. This function does nothing, but it’s valid Python code.
If you’re intrigued by this unique feature of Python and want to know more about how and when to use the ‘pass’ statement, keep reading. We’ll dive deeper into its usage, its advantages, and some common pitfalls to avoid.
Table of Contents
- Grasping the ‘pass’ Statement: Python for Beginners
- ‘pass’ in Complex Scenarios
- Best Practices with ‘pass’
- Exploring Alternatives to ‘pass’
- Troubleshooting Python’s ‘pass’ Statement
- The Role of ‘pass’ in Python Programming
- ‘pass’ Statement in Larger Scripts
- Exploring Related Concepts
- Deepening Your Python Knowledge
- Wrapping Up Python’s ‘pass’ Statement
Grasping the ‘pass’ Statement: Python for Beginners
In Python, the ‘pass’ statement is a null operation. When it’s executed, nothing happens. It’s a placeholder and is used where the syntax requires a statement, but you don’t want any command or code to be executed.
Let’s look at a simple example:
for i in range(5):
if i == 3:
pass
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this code, we’ve used a ‘for’ loop to iterate over a range of 5 numbers. Inside the loop, we’ve set a condition: if ‘i’ equals 3, then pass. The ‘pass’ statement here means that Python should do nothing. It should simply continue with the next iteration. As a result, all numbers from 0 to 4 are printed.
The ‘pass’ statement can be particularly useful when you’re sketching out the structure of your code and you’re not yet ready to implement certain parts. It allows you to create a syntactically correct Python script that runs without throwing errors, even though some parts of it are still under construction.
However, it’s important to remember that ‘pass’ is not a comment. A comment in Python starts with a ‘#’ and is ignored by the interpreter, while ‘pass’ is not ignored. It’s a valid Python statement that serves a specific purpose.
‘pass’ in Complex Scenarios
As you advance in Python, you’ll find that the ‘pass’ statement can be used in more complex scenarios, such as in loops or conditional statements. Let’s dive into an example:
for i in range(10):
if i % 2 == 0:
pass
else:
print(i)
# Output:
# 1
# 3
# 5
# 7
# 9
In this code, we’re using a ‘for’ loop to iterate over a range of 10 numbers. Inside the loop, we’ve set a condition: if ‘i’ is an even number (i.e., ‘i’ modulo 2 equals 0), then pass. If ‘i’ is not an even number (i.e., ‘i’ is an odd number), then print ‘i’. The ‘pass’ statement here allows Python to ignore even numbers and only print odd numbers from 1 to 9.
This example illustrates how the ‘pass’ statement can be used in conjunction with conditional statements to control the flow of your program. It’s a powerful tool that can make your code more readable and efficient.
Best Practices with ‘pass’
While the ‘pass’ statement is a useful tool, it’s important to use it wisely. It’s not a substitute for proper error handling or a way to ignore problems in your code. Always aim to write clear, concise code and use ‘pass’ as a placeholder during the development process.
Exploring Alternatives to ‘pass’
Beyond the ‘pass’ statement, Python offers other ways to handle empty code blocks, such as the ‘continue’ and ‘break’ statements.
The ‘continue’ Statement
The ‘continue’ statement, unlike ‘pass’, skips the rest of the loop for the current iteration and moves on to the next iteration. Here’s an example:
for i in range(5):
if i == 3:
continue
print(i)
# Output:
# 0
# 1
# 2
# 4
In this code, when ‘i’ equals 3, the ‘continue’ statement is executed and the print statement for that iteration is skipped. As a result, the number 3 is not printed.
The ‘break’ Statement
The ‘break’ statement, on the other hand, terminates the loop entirely and the program continues with whatever code follows the loop. Here’s an example:
for i in range(5):
if i == 3:
break
print(i)
# Output:
# 0
# 1
# 2
In this code, when ‘i’ equals 3, the ‘break’ statement is executed and the loop is terminated. As a result, the numbers 3 and 4 are not printed.
Comparing ‘pass’, ‘continue’, and ‘break’
Statement | Action | Use Case |
---|---|---|
pass | Does nothing | Placeholder for future code |
continue | Skips the rest of the loop for the current iteration | When you want to skip a specific iteration |
break | Terminates the loop | When you want to end the loop prematurely |
While ‘pass’, ‘continue’, and ‘break’ can all be used in loops and conditional statements, they serve different purposes and should be used in different situations. Understanding the differences between them and when to use each one is key to writing efficient Python code.
Troubleshooting Python’s ‘pass’ Statement
While the ‘pass’ statement in Python is straightforward, you may encounter some issues or unexpected behavior, especially when it’s used in loops or conditional statements.
Unexpected Behavior in Loops
One common issue is expecting the ‘pass’ statement to behave like ‘continue’ or ‘break’. Remember, ‘pass’ does nothing and is merely a placeholder. It won’t skip an iteration or end a loop prematurely. Here’s an example to illustrate this point:
for i in range(5):
if i == 3:
pass
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this code, even though the ‘pass’ statement is executed when ‘i’ equals 3, the number 3 is still printed. This is because ‘pass’ doesn’t affect the flow of the program.
Misusing ‘pass’ as a Placeholder
Another common issue is leaving ‘pass’ in your code as a placeholder for too long. While it’s useful for sketching out the structure of your code, you should aim to replace ‘pass’ with actual code as soon as possible. Leaving ‘pass’ in your code can lead to functions or classes that don’t do anything, which can cause confusion and bugs.
In conclusion, while the ‘pass’ statement is a useful tool in Python, it’s important to understand its purpose and how to use it correctly. Always remember that ‘pass’ is a placeholder for future code and does not affect the flow of your program.
The Role of ‘pass’ in Python Programming
To fully grasp the ‘pass’ statement, it’s important to understand its role in Python programming. In essence, ‘pass’ is a placeholder that allows you to write syntactically correct Python code, even if some parts of it are not yet implemented.
‘pass’: A Placeholder in Python
In Python, the ‘pass’ statement is often used when you’re sketching out the structure of your code. For example, you might know that you want to include a function in your code, but you’re not yet sure what this function should do. In this case, you can use the ‘pass’ statement to indicate where the function will go. Here’s an example:
def my_function():
pass
In this code, we’ve defined a function named ‘my_function’. Inside the function, we’ve placed the ‘pass’ statement as a placeholder. This function does nothing, but it’s valid Python code and it won’t cause any errors.
The Importance of Placeholders in Code Development
Placeholders like the ‘pass’ statement are crucial in code development. They allow you to focus on the overall structure of your code before you dive into the details. They also make it easier to collaborate with others, as you can sketch out the structure of the code and then assign different parts to different people.
In conclusion, the ‘pass’ statement is a unique feature of Python that serves a specific purpose. While it does nothing when executed, it plays a crucial role in the development process by allowing you to write syntactically correct Python code at all stages of development.
‘pass’ Statement in Larger Scripts
The ‘pass’ statement, while simple, plays a significant role in larger scripts or projects. It allows developers to sketch out the structure of their code and focus on the bigger picture before diving into the details. This can be particularly useful in complex projects where the overall structure of the code is crucial.
Exploring Related Concepts
If you’re interested in the ‘pass’ statement, you might also want to explore related concepts in Python, such as control flow and error handling. Control flow is a fundamental concept in programming that refers to the order in which individual statements, instructions, or function calls are executed or evaluated. Error handling, on the other hand, is a crucial aspect of writing robust code. Python provides several tools for handling errors, such as the ‘try’ and ‘except’ statements.
Here’s an example of how you might use the ‘pass’ statement in conjunction with error handling:
try:
x = 1 / 0
except ZeroDivisionError:
pass
# Output:
# No output, because the error is silently ignored.
In this code, we’re trying to divide 1 by 0, which raises a ZeroDivisionError. However, we’ve used the ‘pass’ statement in the ‘except’ block to silently ignore this error. This means that our program won’t crash, but it also won’t provide any feedback about the error.
Deepening Your Python Knowledge
To deepen your understanding of Python and its many features, consider exploring these online resources:
- Beginner’s Guide to Python Loops – Discover the importance of loop control statements like break and continue.
Python “yield” Keyword – Explore Python’s “yield” keyword and its role in creating generator functions.
Handling Multiple Conditions in Python – Learn about the limitations of switch-case and how to achieve similar logic in Python.
Python For Loop Guide – A guide by IONOS dedicated to the ‘for’ loop in Python.
Python Generators Overview – Another article by IONOS detailing an overview of Python generators and their usage.
Control Statements in Python – An informative guide from Python Tricks discussing control statements in Python.
Wrapping Up Python’s ‘pass’ Statement
The ‘pass’ statement in Python, while simple, plays a significant role in code development. It acts as a placeholder, allowing you to draft the structure of your code without having to fill in all the details immediately. This is especially useful in larger projects where planning the overall structure is crucial.
def placeholder_function():
pass
# Output:
# No output, as the function does nothing.
In this example, ‘pass’ is used to create a placeholder function. The function doesn’t do anything yet, but it’s syntactically correct and won’t cause any errors.
It’s important to note that ‘pass’ is not a comment and does not affect the flow of your program. Misunderstanding this can lead to unexpected behavior, particularly in loops and conditional statements.
Beyond ‘pass’, Python offers other ways to handle empty code blocks, such as the ‘continue’ and ‘break’ statements. These statements, while serving different purposes, can be used to control the flow of your program.
Statement | Action | Use Case |
---|---|---|
pass | Does nothing | Placeholder for future code |
continue | Skips the rest of the loop for the current iteration | When you want to skip a specific iteration |
break | Terminates the loop | When you want to end the loop prematurely |
In conclusion, understanding the ‘pass’ statement and its alternatives is key to writing efficient Python code. Always aim to write clear, concise code, and use ‘pass’ as a temporary placeholder during the development process.