“For Loop” in Python | Syntax, usage, and examples
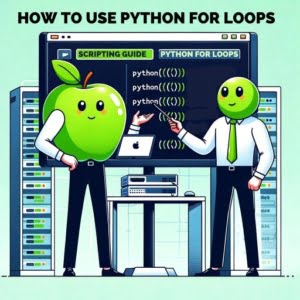
When programming for automation at IOFLOOD, a ‘for’ loop is our go-to tool for iteration over iterable objects and sequences. In our experience, for loops simplify repetitive tasks and enhance code organization, particularly in tasks requiring sequential processing. Today’s article explains how to effectively use a for loop in Python, providing examples and explanations to assist our customers with scripting tips for their custom server configurations.
In this blog post, we will embark on an in-depth exploration of Python loops. We will examine the different types of loops in Python, such as the ‘for loop’, ‘while loop’, and ‘nested loops’. We will discuss their syntax, usage, and the unique characteristics that distinguish Python loops from those in other programming languages.
So, let’s dive in and start looping with Python!
TL;DR How do I use a For Loop in Python?
In Python, a
for
loop is used to iterate over a sequence such as alist
,tuple
, orstring
. The syntax isfor item in sequence:
, where'item'
is a variable that takes on each value in the sequence, and'sequence'
is the collection of items you want to iterate over, followed by your block of code indented underneath.
See example:
for i in range(5):
print(i)
Table of Contents
Understanding Python’s Looping Structures
Python is a favored language among many programmers, primarily due to its unique and powerful features. One such feature is its looping structures. Python primarily uses three types of loops: the ‘while loop’, the ‘for loop’, and ‘nested loops’. Each loop has its unique characteristics and use cases, making them indispensable tools in a programmer’s toolkit.
The Syntax and Condition-Checking Time of Each Loop Type
Let’s start with the syntax and condition-checking time of each loop type. The ‘while loop’ continues execution as long as its condition remains true. The ‘for loop’ in Python, on the other hand, is more like an iterator-based loop and is used to iterate over an iterable object (like list, tuple, set, dictionary, or string). Nested loops, as the name suggests, are loops within loops, allowing for more complex looping mechanisms.
Python’s Use of Indentation for Grouping Statements
A unique feature of Python is its use of indentation for grouping statements. Unlike other languages that use braces to define a block of code, Python uses indentation. This means the code within the loop is indented, and when the indentation ends, so does the loop.
Understanding these loop structures is vital for efficient programming in Python. It allows you to control the flow of your program and repeat certain operations, thereby reducing the amount of code you need to write.
But there’s more to Python’s indentation-based grouping than just aesthetics. It significantly impacts code readability and reduces errors. By forcing you to indent your code, Python ensures your code is neat and clean, making it easier to read and understand. It also eliminates the common errors that occur in languages that use braces for grouping, where a missing or extra brace can break the entire code. So, the next time you find yourself appreciating the neatness of your Python code, remember to thank its indentation-based grouping!
Diving Deeper into the While Loop
Having introduced Python loops, let’s now delve deeper into the ‘while loop’. The ‘while loop’ in Python is a control flow statement that facilitates repeated execution of code based on a given Boolean condition. The loop persists as long as the condition remains true. The syntax is simple and straightforward:
while condition:
statement(s)
The ‘while loop’ is versatile and can be employed in numerous scenarios. For example, if you’re writing a program that should run until receiving a specific input, a ‘while loop’ would be a perfect choice. It’s also ideal for maintaining a program state until a certain number of conditions are met.
The Unique ‘Else’ Statement in Python’s While Loop
One unique feature of Python’s ‘while loop’ is the inclusion of an ‘else’ statement. Unlike many other programming languages, in Python, the ‘else’ statement can be used with the ‘while loop’. The ‘else’ block executes when the condition in the ‘while’ statement is no longer true. Here’s how it looks:
while condition:
statement(s)
else:
statement(s)
This unique feature sets Python apart.
However, caution is advised when dealing with ‘while loops’. If the condition never becomes false, the ‘while loop’ can run indefinitely, leading to an infinite loop. This underscores the importance of condition accuracy. You must ensure that the loop’s condition will eventually become false; otherwise, you could find yourself stuck in an endless loop!
Understanding the ‘while loop’ and its unique features is a significant advantage in Python programming. It not only enhances your programming skills but also paves the way for solving problems in more efficient ways.
Exploring the For Loop
Continuing our exploration of Python loops, we now turn our attention to the ‘for loop’. The ‘for loop’ in Python is used for sequential traversal. It iterates over items in a sequence (like a list or a string) in the order they appear. You can liken it to a treasure hunt where you follow the map, point by point, until you find the treasure.
The Syntax of the For Loop
The syntax of the ‘for loop’ in Python is quite simple:
for val in sequence:
statement(s)
Here, ‘val’ is the variable that takes the value of the item inside the sequence on each iteration. The ‘for loop’ will continue to execute until it has gone through all items in the sequence. For instance, if you have a list of names and you want to print each one, a ‘for loop’ is an excellent way to do it.
names = ['Alice', 'Bob', 'Charlie']
for name in names:
print(name)
But the ‘for loop’ isn’t limited to lists. You can use it with any iterable object in Python. This includes tuples, strings, and dictionaries, making the ‘for loop’ a versatile tool in Python programming.
Using the ‘Else’ Statement in Python’s For Loop
Just like the ‘while loop’, the ‘for loop’ in Python also supports an ‘else’ statement. The ‘else’ block executes after the ‘for loop’ has finished iterating over the items in the sequence. Here’s what it looks like:
for val in sequence:
statement(s)
else:
statement(s)
This feature extends the utility of the ‘for loop’ beyond numerical repetition and allows for more complex programming patterns. The ‘for loop’ in Python is more than just a looping construct—it’s a powerful tool that every Python programmer should master.
Creating Custom Iterable Objects
Python’s flexibility extends beyond its built-in iterable objects. One of the most powerful features of the language is the ability to create custom iterable objects. This feature allows you to define your own iteration behavior for your objects, thereby enhancing Python’s looping mechanisms.
How Do Custom Iterable Objects Work?
In Python, an object is considered iterable if it has a defined __iter__
method. This method should return an iterator object that defines a __next__
method. The __next__
method accesses elements in the container one at a time. Once the __next__
method has accessed all items, it should raise the StopIteration
exception, signaling that all items have been accessed.
Custom Range Function Example
Let’s consider an example. Suppose you want to create a custom range function that generates a sequence of numbers within a given range, but instead of incrementing by 1, it increments by 2. Here’s how you could do it:
class MyRange:
def __init__(self, start, end):
self.value = start
self.end = end
def __iter__(self):
return self
def __next__(self):
if self.value >= self.end:
raise StopIteration
current = self.value
self.value += 2
return current
for num in MyRange(1, 10):
print(num)
This script will output the numbers 1, 3, 5, 7, and 9. As you can see, by creating a custom iterable object, you can control exactly how your ‘for loop’ iterates over the object.
Fibonacci Sequence Example
Another example could be an object that generates Fibonacci numbers:
class Fibonacci:
def __init__(self, max):
self.max = max
self.a, self.b = 0, 1
def __iter__(self):
return self
def __next__(self):
fib = self.a
if fib > self.max:
raise StopIteration
self.a, self.b = self.b, self.a + self.b
return fib
for num in Fibonacci(100):
print(num)
This script will print the Fibonacci sequence up to 100.
Creating custom iterable objects in Python provides you with more control over your loops, enabling you to tailor your loop’s behavior to your specific needs. It’s a powerful tool that can aid in writing more efficient and flexible code.
As we delve deeper into Python’s looping mechanisms, we encounter nested loops and loop control statements. These advanced concepts enable more complex programming patterns and can significantly enhance your code’s efficiency.
Nested Loops
First, let’s discuss nested loops. In Python, you can place one loop inside another, creating a ‘nested loop’. This is especially useful when you want to iterate over multiple sequences or a multi-dimensional data structure, such as a list of lists or a matrix. Here’s a simple example of a nested ‘for loop’ in Python:
for i in range(3):
for j in range(3):
print(i, j)
This script will print the pairs (0, 0), (0, 1), (0, 2), (1, 0), (1, 1), and so on. As you can see, the outer loop runs three times, and for each iteration of the outer loop, the inner loop also runs three times.
Loop Control Statements
Next, let’s explore loop control statements. These are special statements that can alter a loop’s execution from its normal sequence. Python supports three types of loop control statements: ‘break’, ‘continue’, and ‘pass’.
The ‘break’ statement terminates the loop and transfers execution to the statement immediately following the loop. The ‘continue’ statement skips the rest of the code inside the current iteration and moves on to the next iteration. The ‘pass’ statement is a placeholder and does nothing; it’s used when syntax requires a statement, but you don’t want to execute any code.
Here’s an example that uses all three loop control statements:
for num in range(10):
if num == 3:
continue
if num == 5:
break
if num == 4:
pass # do nothing
print(num)
This script will print the numbers 0, 1, 2, and 4. The number 3 is skipped due to the ‘continue’ statement, the number 4 is printed despite the ‘pass’ statement (because it does nothing), and the loop terminates when the number is 5 due to the ‘break’ statement.
Understanding nested loops and loop control statements is crucial for tackling complex programming tasks. They provide more control over your loops and allow you to handle a wide range of scenarios more efficiently.
The Use of an Underscore (_) in a ‘For Loop’
Lastly, let’s discuss a neat trick with Python’s ‘for loop’: the use of an underscore (). In Python, a ‘for loop’ doesn’t always require the variable that’s getting the values of the sequence. If you don’t need to use the variable, you can use an underscore () instead, providing a clean, intentional syntax for loops that don’t require element access. Here’s an example:
for _ in range(5):
print('Hello, World!')
This script will print ‘Hello, World!’ five times. The underscore (_) is a convention in Python that indicates to other programmers that the loop variable isn’t actually used in the loop.
Mastering nested loops, loop control statements, and the use of an underscore (_) in a ‘for loop’ can significantly enhance your Python programming skills. These advanced features of Python’s looping mechanisms enable you to write more efficient and cleaner code.
Further Resources for Python Iterator Mastery
To hone your understanding of Python’s iterators, control statements and loops, here is a collection of useful resources:
- Python Loop Fundamentals – Explore Python’s loop optimization techniques for improved performance.
Enumerating Iterables in Python: – A quick guide on “enumerate” in Python for tracking loop iteration and values.
Python “exit()” – Explore how “exit” can interrupt programs and help manage control flow.
Python ‘For’ Loops – A detailed guide by TutorialsPoint to demonstrate the use of ‘for’ loops in Python.
Python Iterators & Generators – A Medium article providing an in-depth explanation of Python’s iterators and generators.
Python Control Flow – A Tutorialspoint guide providing details of Python’s control flow.
Utilize these tools and strengthen your understanding of Python’s controls structures, iterating processes, and looping mechanisms.
Conclusion
In conclusion, we’ve traversed the intricate landscape of Python loops, uncovering their unique features, syntax, and practical applications. We embarked on this journey by grasping the fundamentals: the ‘while loop’, the ‘for loop’, and ‘nested loops’. Each of these loops, with its distinctive syntax and use cases, is an essential tool for Python programming.
We then dove into the unique characteristics of Python loops, such as the ‘else’ clause in ‘while’ and ‘for’ loops. This feature, uncommon in many programming languages, significantly enhances the flexibility of Python’s looping mechanisms. Our exploration further led us to the creation of custom iterable objects, empowering you to define your own iteration behavior.
We also discovered Python’s loop control statements: ‘break’, ‘continue’, and ‘pass’. These statements offer another layer of control to your loops, enabling you to manipulate the execution of a loop based on specific conditions. And of course, we must not forget the use of an underscore (_) in a ‘for loop’, a neat trick that provides a clean syntax for loops that don’t require element access.
To master Python’s subtleties, click here for a treasure trove of tips and tricks.
Understanding Python’s looping mechanisms is indispensable for any Python programmer. They not only allow you to execute a block of code multiple times but also offer a plethora of features to make your loops more efficient and flexible. So, keep practicing and exploring Python loops, and you’ll soon find yourself writing cleaner, more efficient code. Keep looping!