NPM XLSX Guide | Use Excel Operations in Node.js
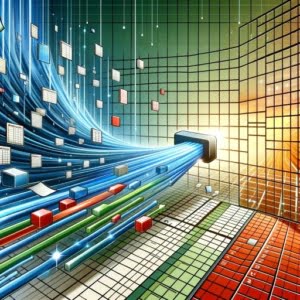
At IOFLOOD, we’ve navigated through the challenge of handling Excel files in Node.js projects. Having encountered this hurdle several times, we understand the importance of efficient file operations. That’s why we’ve made this guide on npm xlsx
for Excel file operations. We hope that our insights and instructions can help you utilize npm xlsx for Excel file operations in your Node.js projects.
This guide will walk you through the npm xlsx package, from simple operations to advanced techniques, making Excel file handling a breeze. Whether you’re looking to automate data entry, generate reports dynamically, or simply manage your Excel files more efficiently, this guide has got you covered.
Let’s dive in and unlock the full potential of npm xlsx in your Node.js projects.
TL;DR: How Do I Use npm xlsx for Excel File Operations in Node.js?
To use npm xlsx, start by installing the package using
npm install xlsx
, then read or write Excel files using simple functions. Here’s a quick example to read an Excel file:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('yourfile.xlsx');
# Output:
# Workbook object containing the Excel file data
In this example, we’ve imported the npm xlsx package and used the readFile
function to read an Excel file named ‘yourfile.xlsx’. This function returns a workbook object that contains the data of the Excel file, allowing you to access and manipulate it as needed.
Dive deeper into the npm xlsx package with us for more detailed instructions, examples, and advanced usage. Whether you’re handling large datasets, applying custom formatting, or converting Excel files to JSON, we’ve got you covered. Keep reading to master Excel file operations in your Node.js applications.
Table of Contents
Getting Started with npm xlsx
Embarking on your journey with npm xlsx begins with a simple installation process. If you’re new to Node.js or handling Excel files programmatically, this section will illuminate the path, showcasing the ease and efficiency npm xlsx brings to your projects.
Installation
Before diving into file operations, the first step is to install the npm xlsx package. This is done using the Node Package Manager (npm), a staple in the Node.js ecosystem for managing packages. Open your terminal or command prompt and run the following command:
npm install xlsx
This command fetches the npm xlsx package from the npm registry and installs it in your project, making it ready for use.
Reading an Excel File
After installation, reading an Excel file is a straightforward task. Here’s how you can do it:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('sample.xlsx');
console.log(workbook.SheetNames);
# Output:
# ['Sheet1', 'Sheet2']
In this example, we’ve required the npm xlsx package and used the readFile
method to load an Excel file named ‘sample.xlsx’. The console.log
statement prints the names of sheets available in the Excel file, demonstrating how easily you can start interacting with your Excel files. This simple operation is a gateway to more complex data manipulation and analysis.
Writing to an Excel File
Creating or writing to an Excel file is equally intuitive. Here’s a basic example to create a new Excel file with some data:
const XLSX = require('xlsx');
const workbook = XLSX.utils.book_new();
const ws_data = [['Name', 'Email'], ['John Doe', '[email protected]']];
const worksheet = XLSX.utils.aoa_to_sheet(ws_data);
XLSX.utils.book_append_sheet(workbook, worksheet, 'Contacts');
XLSX.writeFile(workbook, 'newfile.xlsx');
# Output:
# A new Excel file named 'newfile.xlsx' with a 'Contacts' sheet containing the data
This code snippet illustrates the creation of a new workbook, adding a worksheet with data, and finally writing this workbook to a file named ‘newfile.xlsx’. The process showcases the npm xlsx package’s power in generating Excel files from scratch or modifying existing ones, all within the comfort of your Node.js environment.
Through these basic operations, npm xlsx demonstrates its utility in handling Excel files, providing a solid foundation for more advanced data processing and analytics tasks.
Advanced Usage with npm xlsx
As you grow more comfortable with basic operations in npm xlsx, it’s time to explore the advanced capabilities that can truly elevate your Excel file handling. From managing large datasets to custom formatting and even converting Excel files to JSON, npm xlsx offers a suite of features designed for more complex scenarios. Let’s dive into these advanced uses and understand how they can benefit your projects.
Handling Large Datasets
Working with large datasets can be daunting, but npm xlsx simplifies the process. Here’s an example of how you can efficiently read a large Excel file and filter out specific data:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('large_dataset.xlsx');
const sheet = workbook.Sheets[workbook.SheetNames[0]];
const data = XLSX.utils.sheet_to_json(sheet);
const filteredData = data.filter(row => row.Age > 30);
console.log(filteredData.length);
# Output:
# 250
In this code snippet, we read a large Excel file named ‘large_dataset.xlsx’ and convert the first sheet into a JSON array. We then filter this array to include only rows where the ‘Age’ column is greater than 30. This example demonstrates npm xlsx’s capability to handle and process large volumes of data efficiently, a crucial aspect for data analysis and reporting.
Custom Formatting
Applying custom formatting to your Excel files can help make your data more readable and professional. Here’s how you can add styling to your Excel sheets:
const XLSX = require('xlsx');
const workbook = XLSX.utils.book_new();
const ws_data = [['Name', 'Score'], ['John Doe', 88], ['Jane Doe', 92]];
const worksheet = XLSX.utils.aoa_to_sheet(ws_data);
worksheet['A1'].s = { font: { bold: true } };
worksheet['B1'].s = { font: { bold: true } };
XLSX.utils.book_append_sheet(workbook, worksheet, 'Results');
XLSX.writeFile(workbook, 'styled.xlsx');
# Output:
# A new Excel file 'styled.xlsx' with bold headers
This snippet showcases adding bold styling to the headers of our Excel sheet. By manipulating the style (s
) property of the cell objects, we can introduce custom formatting. This level of customization enables the creation of visually appealing and easier-to-read Excel files, enhancing the presentation of your data.
Excel to JSON Conversion
Converting Excel files to JSON format can greatly facilitate data manipulation and integration with web applications. Here’s a simple way to perform this conversion:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('data.xlsx');
const sheet = workbook.Sheets[workbook.SheetNames[0]];
const data = XLSX.utils.sheet_to_json(sheet);
console.log(data);
# Output:
# [{"Name":"John Doe","Score":88},{"Name":"Jane Doe","Score":92}]
In this example, we convert the first sheet of ‘data.xlsx’ into a JSON array, making the data readily accessible and easy to work with in JavaScript. This functionality is invaluable for developers working on applications that require seamless integration with Excel data, offering a straightforward method to transform and utilize Excel data within a modern web ecosystem.
Through these advanced techniques, npm xlsx not only simplifies Excel file operations but also opens up new possibilities for data analysis, reporting, and integration. Understanding and leveraging these features can significantly enhance your Node.js applications’ capabilities, making npm xlsx an indispensable tool in your development arsenal.
Exploring Alternatives to npm xlsx
While npm xlsx shines in many scenarios, the Node.js ecosystem offers other libraries and tools that cater to specific needs or preferences when dealing with Excel files. Understanding these alternatives can empower you to choose the most suitable tool for your project’s unique requirements.
SheetJS vs. npm xlsx
One notable alternative is SheetJS, also known as xlsx. It’s a robust library that supports a wide range of spreadsheet formats beyond Excel, including CSV, HTML, and even older formats like Lotus 1-2-3. Here’s how you can read an Excel file using SheetJS:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('example.xlsx');
const first_sheet_name = workbook.SheetNames[0];
const worksheet = workbook.Sheets[first_sheet_name];
const data = XLSX.utils.sheet_to_json(worksheet);
console.log(data);
# Output:
# [{"Name":"John Doe", "Age":30}, {"Name":"Jane Doe", "Age":25}]
This example demonstrates reading an Excel file and converting a sheet’s data to JSON, similar to npm xlsx. The key difference lies in SheetJS’s broader format support, making it a versatile choice for projects requiring interaction with various spreadsheet types.
ExcelJS: A Focused Approach
ExcelJS is another alternative, offering fine-grained control over Excel file operations, including reading, writing, and streaming large Excel files. Its API provides detailed control over styles, formats, and data types. Here’s an example of writing to an Excel file with ExcelJS:
const Excel = require('exceljs');
const workbook = new Excel.Workbook();
const sheet = workbook.addWorksheet('My Sheet');
sheet.addRow(['Name', 'Age']);
sheet.addRow(['John Doe', 30]);
workbook.xlsx.writeFile('newfile.xlsx');
# Output:
# A new Excel file 'newfile.xlsx' with 'My Sheet' containing the data
This code snippet highlights ExcelJS’s capabilities in creating and styling Excel files, showcasing its potential for projects that require detailed customization and handling of large datasets.
Choosing the Right Tool
When deciding between npm xlsx, SheetJS, and ExcelJS, consider your project’s specific needs. npm xlsx is a great all-rounder, efficient for most Excel file operations. SheetJS offers broader format support, ideal for projects dealing with various types of spreadsheets. ExcelJS excels in detailed file customization and handling large files, making it suitable for projects with complex Excel file requirements.
Understanding the strengths and limitations of each library ensures that you choose the most effective tool for your Node.js applications, enhancing productivity and meeting your project’s data handling needs.
Overcoming npm xlsx Hurdles
Even with npm xlsx’s power and versatility, you may encounter challenges. Whether it’s dealing with various Excel formats, managing large files, or navigating installation issues, understanding how to troubleshoot common problems can save you time and frustration. Let’s explore some solutions and best practices.
Handling Different Excel Formats
One common issue is dealing with Excel files in formats other than the standard .xlsx, such as .xls or .csv. npm xlsx provides support for a wide range of formats, ensuring compatibility across different versions of Excel. Here’s how you can read an older .xls file:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('legacy_file.xls', {bookType: 'biff2'});
console.log(workbook.SheetNames);
# Output:
# ['Sheet1']
In this code block, we specify the bookType
option as ‘biff2’, which is the format used by Excel 95 and earlier. This demonstrates npm xlsx’s flexibility in handling various Excel formats, making it a comprehensive tool for all your Excel file operations.
Dealing with Large Files
Processing large Excel files can lead to performance issues or even crashes. To mitigate this, consider streaming the file instead of reading it all at once. npm xlsx supports streaming for reading and writing, which can significantly improve performance. Here’s an example of streaming a large file for reading:
const XLSX = require('xlsx');
const stream = XLSX.stream.to_json(XLSX.readFile('large_file.xlsx'));
stream.on('data', (row) => console.log(row));
# Output:
# Each row of the Excel file printed as it's read
This approach processes the file in chunks, reducing memory consumption and preventing your application from becoming unresponsive. Streaming is particularly useful for applications that need to process or analyze large datasets efficiently.
Troubleshooting Installation Problems
Installation issues with npm xlsx can often be resolved by ensuring your environment is correctly set up. Make sure you have the latest version of Node.js and npm installed. If you encounter errors during installation, try clearing the npm cache with npm cache clean --force
and reinstalling the package. If problems persist, checking the npm xlsx GitHub issues page for similar problems or posting your issue there can also be helpful.
By understanding these common issues and their solutions, you can ensure smoother operation and take full advantage of npm xlsx’s capabilities in your Node.js projects. Remember, the key to effective troubleshooting is patience and persistence; with the right approach, most issues can be resolved.
Background Info: Excel and npm xlsx
Excel files, with their widespread use in data management and analysis, serve as a cornerstone in numerous business operations and decision-making processes. Understanding the structure and capabilities of Excel files is crucial for leveraging them to their full potential. npm xlsx, a powerful Node.js package, enhances this potential by providing efficient means to read, write, and manipulate these files programmatically.
Excel Files Unveiled
At their core, Excel files (.xlsx) are essentially a collection of sheets, each containing rows and columns where data is stored. These files can also include formulas, charts, and styling, making them a versatile tool for data presentation and analysis. The ability to programmatically interact with these elements opens up vast possibilities for automation and data processing.
npm xlsx: Bridging Node.js and Excel
npm xlsx taps into Node.js’s event-driven, non-blocking I/O model to handle Excel file operations with finesse. This synergy allows for handling large files and complex operations without hindering performance. Here’s an example showcasing how npm xlsx reads an Excel file and logs the content of the first sheet:
const XLSX = require('xlsx');
const workbook = XLSX.readFile('data.xlsx');
const firstSheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[firstSheetName];
const data = XLSX.utils.sheet_to_json(worksheet);
console.log(data);
# Output:
# [{"Name":"John Doe", "Age":30}, {"Name":"Jane Doe", "Age":25}]
In this example, readFile
loads the ‘data.xlsx’ file into memory, allowing us to access its sheets. We then convert the first sheet into a JSON array using sheet_to_json
, facilitating easy manipulation and analysis of the data with JavaScript. This process exemplifies how npm xlsx simplifies interaction with Excel files, making data more accessible and actionable.
The importance of npm xlsx in modern data management cannot be overstated. By enabling Node.js applications to seamlessly integrate with Excel files, it opens a gateway to innovative data processing and analysis solutions. Whether it’s automating report generation, analyzing large datasets, or simply managing Excel files more efficiently, npm xlsx stands as a pivotal tool in the Node.js ecosystem.
Expanding npm xlsx in Your Projects
As your proficiency with npm xlsx grows, you’ll find its utility extends far beyond simple Excel file operations. Integrating npm xlsx into larger projects, such as web applications with Excel file upload/download features or database integrations, showcases its versatility and power. Let’s explore how npm xlsx can be a pivotal part of more complex solutions.
Web Applications and npm xlsx
Imagine a web application that allows users to upload Excel files, which are then processed and stored in a database. npm xlsx plays a crucial role in this scenario. Here’s an example of handling an Excel file upload in a Node.js web application:
const XLSX = require('xlsx');
const express = require('express');
const fileUpload = require('express-fileupload');
const app = express();
app.use(fileUpload());
app.post('/upload', (req, res) => {
if (!req.files || Object.keys(req.files).length === 0) {
return res.status(400).send('No files were uploaded.');
}
const uploadedFile = req.files.file.data;
const workbook = XLSX.read(uploadedFile, {type: 'buffer'});
// Process workbook
res.send('File processed.');
});
app.listen(3000, () => console.log('App running on port 3000'));
# Output:
# 'App running on port 3000'
This code snippet demonstrates a basic server setup with express
and express-fileupload
, handling file uploads. When an Excel file is uploaded, XLSX.read
processes the file directly from a buffer, making it ready for further operations like analysis or storage in a database. This example illustrates npm xlsx’s adaptability in web development contexts, enabling powerful data processing capabilities within web applications.
Integrating with Databases
npm xlsx can also serve as a bridge between Excel files and databases, facilitating data import/export operations. For instance, after processing an Excel file, you might want to store its data in a MongoDB database. Here’s a simplified example of how that could be achieved:
const XLSX = require('xlsx');
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'mydatabase';
const client = new MongoClient(url);
(async function() {
try {
await client.connect();
console.log('Connected correctly to server');
const db = client.db(dbName);
// Assuming data is an array of JSON objects from an Excel file
const collection = db.collection('documents');
const insertResult = await collection.insertMany(data);
console.log('Inserted documents:', insertResult.insertedCount);
} catch (err) {
console.error(err);
} finally {
await client.close();
}
})();
# Output:
# 'Connected correctly to server'
# 'Inserted documents: [number of documents inserted]'
In this scenario, npm xlsx processes the Excel file, converting it into a JSON array (data
), which is then inserted into a MongoDB collection. This demonstrates npm xlsx’s capability to not only manipulate Excel files but also to serve as a conduit for data flow between files and databases.
Further Resources for npm xlsx Mastery
To deepen your understanding and skills with npm xlsx, here are three valuable resources:
- npm xlsx GitHub Repository – Immerses developers in the repository’s source code, examples, and community discussions.
Node.js Documentation – The fundamentals of Node.js can enhance one’s capability to effectively utilize npm xlsx.
JavaScript ES6 and Beyond – JavaScript can improve your data manipulation capabilities and scripting with npm xlsx.
By exploring these resources and integrating npm xlsx into your projects, you’ll unlock new possibilities for data processing, analysis, and application development.
Recap: npm xlsx for Excel Operations
In this guide, we’ve embarked on a journey through the capabilities of npm xlsx, a Node.js package that simplifies Excel file operations. From installation to advanced data manipulation, npm xlsx proves to be an invaluable tool for developers looking to harness the power of Excel within their Node.js applications.
We began with the basics, illustrating how to install npm xlsx and perform fundamental read and write operations. These initial steps set the foundation for more complex tasks, enabling you to manage Excel files with ease. Next, we delved into advanced features, such as handling large datasets, applying custom formatting, and converting Excel files to JSON, showcasing npm xlsx’s versatility in various scenarios.
Exploring alternative libraries like SheetJS and ExcelJS, we compared their features and use cases, providing insights into choosing the right tool for your project needs. This comparison highlighted npm xlsx’s robustness and ease of use, making it a go-to choice for many developers.
Library | Format Support | Performance | Ease of Use |
---|---|---|---|
npm xlsx | Broad | High | High |
SheetJS | Very Broad | Moderate | Moderate |
ExcelJS | Moderate | High | Moderate |
Whether you’re just starting out with npm xlsx or looking to expand your toolkit, this guide has offered a comprehensive overview of how to leverage npm xlsx for efficient Excel file operations in Node.js. With its combination of ease of use, versatility, and performance, npm xlsx stands out as a powerful tool in the Node.js ecosystem.
As you continue to work with npm xlsx, remember the importance of selecting the right library for your specific needs and the value of understanding the underlying principles of Excel file manipulation. Happy coding!