Learn Flask for Python | Quick Start Guide (With Examples)
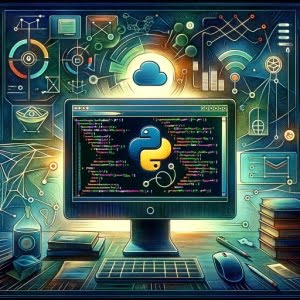
Ever felt overwhelmed by web development in Python? You’re not alone. Web development can be a complex beast, especially when you’re dealing with a powerful language like Python. But, just like a skilled craftsman has a toolbox full of tools, Python developers have Flask.
Flask is a lightweight web framework that can help you build web applications with ease and efficiency. It’s a tool that can simplify your Python web development process, making it more manageable and less overwhelming.
In this guide, we’ll walk you through the basics of Flask in Python, taking you from a novice to a seasoned developer. We’ll start with the fundamentals, explaining what Flask is and how it works. Then, we’ll gradually delve into more advanced topics, showing you how to leverage Flask’s features to build robust web applications.
TL;DR: What is Flask in Python?
Flask is a micro web framework written in Python. It’s designed to make getting started quick and easy, with the ability to scale up to complex applications. It provides you with tools, libraries, and technologies that allow you to build a web application. This web application could be a website, a web API, a web service, or a complex web-based application.
Here’s a simple Flask application:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
# Output:
# Hello, World!
The above code will start a web server on your local machine that you can visit at http://localhost:5000. The ‘@app.route(‘/’)’ decorator in Flask is used to bind URL to a function. Here, it’s binding the root URL of our web application to a function called hello_world. When you visit the root URL, you’ll see ‘Hello, World!’ displayed.
Excited to learn more? Continue reading for a deeper understanding and more advanced usage scenarios of Flask in Python.
Table of Contents
- Flask Basics: Building Your First Application
- Flask’s Advanced Features: Blueprints, Databases, and User Sessions
- Exploring Alternatives: Django and Pyramid
- Flask Troubleshooting: Common Issues and Solutions
- Flask: Powered by Python’s Web Development Fundamentals
- Flask: Powering Large-Scale Web Applications
- Further Resources for Web Development with Python
- Flask in Python: A Powerful Tool for Web Development
Flask Basics: Building Your First Application
Now that we’ve introduced Flask, let’s dive into the basics and create a simple Flask application. We’ll cover routing, templates, and forms — the fundamental building blocks of any Flask app.
Routing in Flask
Routing is the mechanism by which requests to a specific URL are handled by a specific function. In Flask, this is achieved using decorators. Here’s an example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Home Page'
@app.route('/about')
def about():
return 'About Page'
if __name__ == '__main__':
app.run()
# Output when you visit 'http://localhost:5000/':
# Home Page
# Output when you visit 'http://localhost:5000/about':
# About Page
In the code above, we’ve defined two routes — ‘/’ (the homepage) and ‘/about’ (the about page). When you visit ‘http://localhost:5000/’, you’ll see ‘Home Page’ displayed, and when you visit ‘http://localhost:5000/about’, you’ll see ‘About Page’.
Using Templates
While returning strings from our routes works, it’s not very flexible. That’s where templates come in. Templates allow us to return HTML from our routes, making our pages more dynamic. Here’s how to use templates in Flask:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
@app.route('/about')
def about():
return render_template('about.html')
if __name__ == '__main__':
app.run()
In this example, we’re using the ‘render_template’ function to return an HTML file instead of a string. The HTML files (‘home.html’ and ‘about.html’) should be placed in a ‘templates’ folder in the same directory as your Flask application.
Handling Forms
Forms are essential for any web application that requires user input. Flask makes handling forms easy. Here’s a simple example:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
return 'Hello ' + request.form['username']
return render_template('login.html')
if __name__ == '__main__':
app.run()
In the above example, we’re handling a login form. If the method of the request is ‘POST’, we return a greeting with the username from the form. Otherwise, we render the ‘login.html’ template.
These are the basics of building a Flask application. With just these tools, you can build a wide variety of web applications. But remember, every tool has its limitations. Flask is no exception. It’s designed to be a micro-framework, meaning it’s lightweight and simple. It doesn’t come with some of the features of larger frameworks like Django. However, its simplicity and flexibility make it a great choice for many projects.
Flask’s Advanced Features: Blueprints, Databases, and User Sessions
After mastering the basics of Flask, you can start exploring its more advanced features. These features, such as blueprints, database integration, and user sessions, can significantly enhance your Flask applications. Let’s delve into each of these features.
Blueprints in Flask
Blueprints are a way to organize your Flask application into components. They allow you to define routes in one place and use them in another, making your code more modular and easier to understand. Here’s an example of how to use blueprints:
from flask import Flask, Blueprint
app = Flask(__name__)
main = Blueprint('main', __name__)
@main.route('/')
def home():
return 'Home Page'
app.register_blueprint(main)
if __name__ == '__main__':
app.run()
In the above example, we’re defining a blueprint named ‘main’. We define a route (‘/’) within this blueprint and then register it with our Flask application using ‘app.register_blueprint(main)’. Now, when you visit ‘http://localhost:5000/’, you’ll see ‘Home Page’ displayed.
Database Integration
Flask doesn’t come with built-in database support, but it can be easily integrated with a database using extensions like Flask-SQLAlchemy. Here’s a simple example of how to integrate Flask with a SQLite database:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:////tmp/test.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return '<User %r>' % self.username
In this example, we’re defining a ‘User’ model with ‘id’, ‘username’, and ’email’ fields. We can use this model to interact with our SQLite database.
User Sessions
User sessions allow you to store information specific to a user from one request to the next. This is essential for things like login systems. Here’s how you can use sessions in Flask:
from flask import Flask, session
app = Flask(__name__)
app.secret_key = 'super secret key'
@app.route('/setuser/<username>')
def setuser(username):
session['user'] = username
return 'User set'
@app.route('/getuser')
def getuser():
return 'User: ' + session['user']
if __name__ == '__main__':
app.run()
In this example, we’re setting a ‘user’ in the session when you visit 'http://localhost:5000/setuser/<username>'
. When you visit 'http://localhost:5000/getuser'
, you’ll see the user that was set.
These advanced features can make your Flask applications more powerful and flexible. However, they also introduce more complexity. It’s important to understand these features well before using them in your applications.
Exploring Alternatives: Django and Pyramid
While Flask is a powerful tool in the Python web development toolbox, it’s not the only one. There are other web frameworks in Python, such as Django and Pyramid, which offer different features and advantages. Let’s explore these alternatives and see how they compare to Flask.
Django: The Full-Featured Framework
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It’s built by experienced developers and takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel.
# Sample Django application
from django.http import HttpResponse
from django.shortcuts import render
def hello_world(request):
return HttpResponse('Hello, World!')
# Expected output when you visit 'http://localhost:8000/':
# Hello, World!
In the Django example above, we define a view that returns ‘Hello, World!’ when visited. Unlike Flask, Django comes with a built-in administrative interface, ORM, and an authentication system, making it more feature-rich out of the box, but also heavier.
Pyramid: Flexibility and Power
Pyramid is a general, open source, Python web framework. Its main goal is to do as much as possible with minimum complexity. It’s designed to scale both up and down, making it suitable for both simple and complex applications.
# Sample Pyramid application
from pyramid.response import Response
from pyramid.view import view_config
@view_config(route_name='hello')
def hello_world(request):
return Response('Hello, World!')
# Expected output when you visit 'http://localhost:6543/hello':
# Hello, World!
In the Pyramid example above, we define a view that returns ‘Hello, World!’ when visited. Pyramid provides more flexibility than Flask and Django, allowing you to choose your own components, such as the database layer or template style.
Framework | Complexity | Flexibility | Use Case |
---|---|---|---|
Flask | Low | High | Small to medium projects, APIs |
Django | High | Low | Large projects, full-featured web applications |
Pyramid | Medium | High | Both small and large projects, flexible applications |
Each of these frameworks has its own strengths and weaknesses. Flask’s simplicity and flexibility make it a great choice for small to medium projects and APIs. Django’s built-in features make it suitable for larger, full-featured web applications. Pyramid’s flexibility allows it to handle both small and large projects effectively. The best framework for your project depends on your specific needs and expertise.
Flask Troubleshooting: Common Issues and Solutions
As with any tool, using Flask in Python is not without its challenges. You may encounter issues with routing, database integration, or deployment. But don’t worry! We’ve got you covered. Let’s discuss some common problems and their solutions.
Routing Issues
One common issue in Flask is incorrect routing, which can result in 404 errors. This is often due to a simple typo or misunderstanding of the routing syntax.
@app.route('/home')
def home():
return 'Home Page'
# Expected output when you visit 'http://localhost:5000/home':
# Home Page
In the example above, the route is '/home'
, not '/'
. So, visiting 'http://localhost:5000/'
will result in a 404 error. The correct URL is 'http://localhost:5000/home'
.
Database Integration Problems
Another common issue is trouble integrating with databases. This can be due to a variety of reasons, such as incorrect configuration or misunderstanding of the ORM syntax.
from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:////tmp/test.db'
db = SQLAlchemy(app)
In the example above, we’re configuring a SQLite database. If the database file (‘/tmp/test.db’) does not exist or is not accessible, you’ll encounter an error. Make sure your database URI is correct and the database file is accessible.
Deployment Challenges
Deploying a Flask application can also pose challenges. These can range from server configuration issues to problems with environment variables.
if __name__ == '__main__':
app.run(host='0.0.0.0')
In the example above, we’re configuring the Flask application to run on all available network interfaces. If your server is not configured to allow this, you’ll encounter an error. Make sure your server is properly configured for your Flask application.
Remember, troubleshooting is a normal part of the development process. When you encounter an issue, take a step back and analyze the problem. Check your code, review the documentation, and don’t hesitate to seek help from the community. With patience and persistence, you can overcome any challenge that comes your way.
Flask: Powered by Python’s Web Development Fundamentals
To fully grasp the power and efficiency of Flask in Python, it’s crucial to understand the fundamental concepts that underpin it. These include the Web Server Gateway Interface (WSGI) standard, the HTTP protocol, and the Model-View-Controller (MVC) pattern. Let’s delve into each of these elements.
The WSGI Standard
WSGI is the standard for Python web applications to communicate with web servers. It’s the glue between your Flask application and the web server that serves your application to users.
from wsgiref.simple_server import make_server
def application(environ, start_response):
start_response('200 OK', [('Content-Type', 'text/html')])
return [b'Hello World!']
with make_server('', 8000, application) as server:
server.serve_forever()
# Expected output when you visit 'http://localhost:8000':
# Hello World!
In the above example, we create a simple WSGI application that returns 'Hello World!'
when visited. Flask applications are WSGI applications, and understanding this standard can help you better understand how Flask works.
The HTTP Protocol
HTTP is the protocol that powers the web. It’s the language that browsers and servers use to communicate. Understanding HTTP is essential for web development in Python, and Flask makes it easy to handle HTTP requests and responses.
@app.route('/hello', methods=['GET'])
def hello():
return 'Hello, World!'
# Expected output when you visit 'http://localhost:5000/hello':
# Hello, World!
In the Flask example above, we define a route that responds to HTTP GET requests. When you visit 'http://localhost:5000/hello'
, the server sends an HTTP response with the text 'Hello, World!'
.
The MVC Pattern
The Model-View-Controller (MVC) pattern is a design pattern that separates an application into three interconnected components: the model (data), the view (user interface), and the controller (processes that handle input). Flask doesn’t enforce the MVC pattern like Django does, but it’s flexible enough to allow for it if you prefer.
# Model
class User(db.Model):
username = db.Column(db.String(80), unique=True)
# View
@app.route('/hello')
def hello():
user = User.query.get(1)
return 'Hello, ' + user.username
# Controller
@app.route('/login', methods=['POST'])
def login():
user = User(request.form['username'])
db.session.add(user)
db.session.commit()
return redirect(url_for('hello'))
# Expected output when you visit 'http://localhost:5000/hello':
# Hello, [username]
In this simplified Flask example, the User class is the model, the ‘hello’ route is the view, and the ‘login’ route is the controller. Understanding the MVC pattern can help you structure your Flask applications more effectively.
Flask: Powering Large-Scale Web Applications
Flask’s flexibility and simplicity have made it a favorite among developers for small to medium-sized projects. But, can Flask handle larger, more complex web applications? Absolutely! Let’s explore how you can use Flask to build RESTful APIs, microservices, and real-time applications.
Building RESTful APIs with Flask
A RESTful API (Representational State Transfer) is an architectural style for an application program interface (API) that uses HTTP requests to access and use data. Flask, with its easy-to-use route decorators and request handlers, is an ideal framework for building these APIs.
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
data = {'name': 'John', 'age': 30, 'job': 'developer'}
return jsonify(data)
if __name__ == '__main__':
app.run()
# Expected output when you visit 'http://localhost:5000/api/data':
# {
# "age": 30,
# "job": "developer",
# "name": "John"
# }
In the above example, we define a simple RESTful API that returns a JSON object when accessed. This is a basic example, but Flask can handle much more complex APIs as well.
Microservices with Flask
Microservices architecture is a method of developing software systems that are made up of independently deployable, modular services. Each service runs a unique process and communicates through a well-defined, lightweight mechanism (like a RESTful API) to serve a business goal. Flask, with its modular and lightweight design, is a great choice for developing these services.
Real-Time Applications with Flask
Real-time applications require a technology that can push server-side content to the client instantly as it happens. Flask-SocketIO is a Flask extension that provides support for handling WebSockets, allowing for real-time bi-directional communication.
from flask import Flask, render_template
from flask_socketio import SocketIO, emit
app = Flask(__name__)
socketio = SocketIO(app)
@socketio.on('message')
def handle_message(message):
emit('response', {'data': message['data']})
if __name__ == '__main__':
socketio.run(app)
In the above example, we’re using Flask-SocketIO to handle WebSocket connections. When a ‘message’ event is received, it emits a ‘response’ event with the same data.
Flask’s flexibility and simplicity make it a powerful tool for building a wide variety of web applications. However, with larger applications come more challenges. As you venture into building larger applications with Flask, consider exploring related topics like web security, performance optimization, and testing. These are critical aspects of web development that can help ensure the success of your project.
Further Resources for Web Development with Python
To find more about general GUI Insights, you can Click Here for info on Python GUI programming for desktop applications.
There are also many resources available online, including documentation, tutorials, and forums, that can help you navigate Python. Here are some that we’ve gathered:
- Creating RESTful APIs with Django Rest Framework – Master DRF for creating RESTful web services with Django.
Simplifying Web Development with Django Framework – Master Django for building robust web applications.
Introduction to Flask on Pythonbasics – Get started with understanding what Flask is and its usage in Python.
Flask Tutorial on TutorialsPoint – A step-by-step tutorial teaching the basics of Flask.
Official Flask Documentation – An authoritative guide to Flask provided directly by its developers.
These resources can deepen your understanding of Flask and web development in general.
Flask in Python: A Powerful Tool for Web Development
Throughout this guide, we’ve explored Flask, a lightweight yet powerful web framework in Python. We’ve journeyed from understanding its basics to utilizing its advanced features, and even delved into troubleshooting common issues. We’ve seen how Flask shines in its simplicity and flexibility, making it an excellent choice for both novice developers and seasoned coders.
We’ve also discussed how Flask handles HTTP requests and responses, adheres to the WSGI standard, and can be structured based on the MVC pattern. These fundamental concepts power Flask and, by extension, your web applications.
Moreover, we’ve discovered that Flask’s capabilities extend beyond simple web applications. It’s equally adept at building RESTful APIs, microservices, and even real-time applications.
But remember, Flask is not the only tool in the Python web development toolbox. We’ve compared it with other frameworks like Django, known for its feature-rich environment, and Pyramid, appreciated for its flexibility. Each framework has its strengths and weaknesses, and the best choice depends on your project’s specific needs.
Framework | Complexity | Flexibility | Use Case |
---|---|---|---|
Flask | Low | High | Small to medium projects, APIs |
Django | High | Low | Large projects, full-featured web applications |
Pyramid | Medium | High | Both small and large projects, flexible applications |
Whether you’re building a small project or a complex web application, Flask offers the tools and simplicity to make your Python web development journey smoother. Keep exploring, keep learning, and happy coding!