Django REST Framework | Ultimate Guide
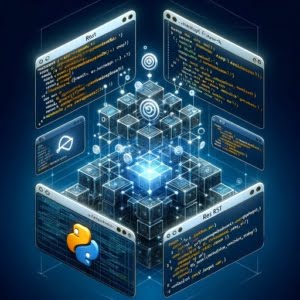
Are you finding it challenging to build robust APIs in Python? You’re not alone. Many developers face this task, but there’s a tool that can make this process a breeze.
Like a skilled architect, Django REST Framework is a powerful toolkit that can help you construct flexible and powerful APIs. These APIs can run on any system, even those without Python installed.
This guide will take you from the basics to advanced techniques of using Django REST Framework. We cover installation, basic use, and advanced techniques / alternatives — perfect for beginners and seasoned Python experts alike.
So, let’s dive in and start mastering Django REST Framework!
TL;DR: What is Django REST Framework?
Django REST Framework is a powerful toolkit for building Web APIs in Python. It provides a set of tools and functionalities that make it easy to build and maintain robust APIs.
Here’s a simple example of a Django REST API:
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
In this example, we’re creating a serializer for a model called MyModel
. The serializer allows us to convert complex data types, like Django models, into Python native data types that can then be easily rendered into JSON.
This is a basic way to use Django REST Framework, but there’s much more to learn about creating and manipulating APIs with it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Setting Up Django REST Framework
- Creating Your First API with Django REST Framework
- Digging Deeper: Advanced Django REST Framework Features
- Exploring Alternative Approaches: Flask and FastAPI
- Troubleshooting Django REST Framework
- Understanding RESTful APIs
- Django REST Framework in Complex Web Applications
- Wrapping Up: Mastering Django REST Framework
Setting Up Django REST Framework
The first step to using Django REST Framework is setting it up. Here’s a step-by-step guide on how to install and set up Django REST Framework in your Python environment.
pip install djangorestframework
This command installs Django REST Framework using pip, Python’s package manager. Once it’s installed, you need to add it to your Django project. To do this, add 'rest_framework'
to your INSTALLED_APPS
in your project’s settings.py file.
INSTALLED_APPS = [
...
'rest_framework',
...
]
Creating Your First API with Django REST Framework
With Django REST Framework set up, let’s create a simple API. We’ll use Django’s built-in models and Django REST Framework’s serializers for this.
First, let’s create a model in your Django app.
from django.db import models
class MyModel(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
This model represents an object with a name
and a description
. Now, let’s create a serializer for this model.
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
The serializer transforms the model data into a format that can be easily rendered into JSON, XML, or other content types. In this case, we’re telling Django REST Framework to include all fields in the serialized output.
Django REST Framework’s simplicity and integration with Django models are some of its key advantages. However, it’s essential to understand that it’s a powerful tool that requires careful handling. Incorrect usage can lead to pitfalls such as exposing sensitive data or causing performance issues.
Digging Deeper: Advanced Django REST Framework Features
Once you’re comfortable with the basics of Django REST Framework, you can start exploring its more advanced functionalities. These include authentication, permissions, viewsets, and routers. Let’s delve into each of these in more detail.
Authentication and Permissions
Django REST Framework provides robust authentication and permissions systems. These systems help ensure that only authorized users can access and manipulate your API’s data. Here’s an example of how you can implement authentication in your Django REST API.
from rest_framework.authentication import BasicAuthentication
from rest_framework.permissions import IsAuthenticated
from rest_framework import viewsets
from .models import MyModel
from .serializers import MyModelSerializer
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
authentication_classes = [BasicAuthentication]
permission_classes = [IsAuthenticated]
In this example, we’re using Django REST Framework’s built-in BasicAuthentication
and IsAuthenticated
classes to authenticate users and check their permissions. If a user isn’t authenticated or doesn’t have the necessary permissions, they won’t be able to access the data.
Viewsets and Routers
Viewsets and routers are another pair of Django REST Framework’s advanced features. They allow you to quickly and easily define the logic for a complete set of views. Here’s an example of how you can use viewsets and routers in your Django REST API.
from rest_framework import routers
from .views import MyModelViewSet
router = routers.DefaultRouter()
router.register(r'mymodel', MyModelViewSet)
# In your urls.py
from django.urls import include, path
from . import views
urlpatterns = [
path('', include(views.router.urls)),
]
In this example, we’re using Django REST Framework’s DefaultRouter
to create routes for our MyModelViewSet
. This automatically creates routes for all the standard create, read, update, and delete (CRUD) operations.
These advanced features of Django REST Framework allow you to create powerful, flexible, and secure APIs. However, they also require a deeper understanding of Django REST Framework and careful implementation to avoid potential pitfalls.
Exploring Alternative Approaches: Flask and FastAPI
While Django REST Framework is a powerful tool for building APIs in Python, it’s not the only game in town. Other Python frameworks, such as Flask and FastAPI, also offer robust features for API development. Let’s take a quick look at these alternatives.
Flask: A Micro Web Framework
Flask is a lightweight and flexible micro web framework for Python. It’s known for its simplicity and fine-grained control over its components. Here’s a basic example of how to build an API with Flask:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api', methods=['GET'])
def api():
return jsonify({'message': 'Hello, Flask!'}), 200
# Running the app
# $ python app.py
# * Running on http://127.0.0.1:5000/api (Press CTRL+C to quit)
# Output:
# {
# "message": "Hello, Flask!"
# }
In this example, we’re defining a route /api
that returns a JSON response with a greeting message. The simplicity of Flask makes it a good choice for small to medium-sized projects or when you need more control over the components of your application.
FastAPI: High Performance with Easy Syntax
FastAPI is a relatively new Python framework for building APIs. It’s known for its high performance and easy-to-use syntax. Here’s a basic example of how to build an API with FastAPI:
from fastapi import FastAPI
app = FastAPI()
@app.get('/api')
def api():
return {'message': 'Hello, FastAPI!'}
# Running the app
# $ uvicorn main:app --reload
# INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
# Output:
# {
# "message": "Hello, FastAPI!"
# }
In this example, we’re defining a route /api
that returns a JSON response with a greeting message. FastAPI’s syntax is very similar to Flask’s, but it also includes type checking, automatic interactive API documentation, and other advanced features out of the box.
While Django REST Framework, Flask, and FastAPI all allow you to build APIs in Python, they each have their own strengths and weaknesses. Django REST Framework integrates seamlessly with Django models and provides a lot of features out of the box, but it can be overkill for small projects or when you need more control over your application’s components. Flask’s simplicity and control make it a good choice for small to medium-sized projects, while FastAPI’s performance and easy-to-use syntax make it a strong contender for both small and large projects.
Troubleshooting Django REST Framework
As with any software development tool, you may encounter issues when using Django REST Framework. Let’s discuss some common problems and their solutions.
Serialization Errors
One common issue in Django REST Framework is serialization errors. These occur when Django REST Framework fails to convert a complex data type into a Python native data type. For example, if your model has a field that your serializer doesn’t account for, you’ll get a serialization error.
# Model with an extra field
class MyModel(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
extra_field = models.CharField(max_length=100)
# Serializer without the extra field
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = ['name', 'description']
In this case, the extra_field
in MyModel
is not included in MyModelSerializer
‘s fields
. This will cause a serialization error. The solution is to include extra_field
in MyModelSerializer
‘s fields
.
# Corrected Serializer
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = ['name', 'description', 'extra_field']
This will ensure that all fields in MyModel
are accounted for in the serialization process, preventing serialization errors.
When working with Django REST Framework, always ensure your serializers match your models. Also, remember to handle any exceptions that may occur during the serialization process. This will help you avoid many common issues and keep your APIs robust and reliable.
Understanding RESTful APIs
Before we delve deeper into Django REST Framework, let’s take a step back and understand the fundamentals behind it – RESTful APIs and HTTP methods.
What are RESTful APIs?
REST stands for Representational State Transfer. It’s an architectural style for designing networked applications. A RESTful API — or service — is based on REST principles and uses HTTP protocols to perform operations on data.
RESTful APIs are stateless, meaning each request from a client to a server must contain all the information needed to understand and process the request. The server should not store anything about the latest HTTP request the client made. Each request is treated as independent from others.
HTTP Methods in RESTful APIs
RESTful APIs use HTTP methods to perform four basic operations on data, also known as CRUD operations:
- Create –
POST
- Read –
GET
- Update –
PUT
orPATCH
- Delete –
DELETE
Each of these methods corresponds to a specific action on the data.
Django REST Framework and RESTful APIs
Django REST Framework is designed to work with RESTful APIs. It provides functionalities for handling HTTP requests and responses according to the principles of REST. Here’s a simple example of how Django REST Framework handles a GET
request:
from rest_framework import viewsets
from .models import MyModel
from .serializers import MyModelSerializer
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
In this example, MyModelViewSet
is a Django REST Framework viewset that handles HTTP requests for MyModel
. The queryset
attribute tells Django REST Framework where to get the data (i.e., MyModel.objects.all()
), and the serializer_class
attribute tells it how to serialize the data (i.e., with MyModelSerializer
).
When a GET
request is made to this viewset, Django REST Framework will return a list of all MyModel
instances, serialized according to MyModelSerializer
.
Understanding these fundamental concepts is crucial when working with Django REST Framework. It allows you to leverage the framework’s features to build robust, flexible, and efficient RESTful APIs.
Django REST Framework in Complex Web Applications
Django REST Framework is not just a tool for creating simple APIs. It’s a powerful framework that can handle the complexities of large-scale web applications. It provides a robust set of tools and functionalities that make it easy to build, test, and maintain APIs.
Exploring Related Concepts
To fully leverage Django REST Framework, it’s helpful to understand related concepts such as database models and Django views.
- Database Models: Django models are the single, definitive source of information about your data. They contain the essential fields and behaviors of the data you’re storing. Django’s models provide a simple and intuitive way to define your data structure.
Django Views: Views are Python functions that take a web request and return a web response. This response can be the HTML contents of a document, a redirect, a 404 error, an XML document, an image, or anything else you can think of.
Understanding these concepts will allow you to create more complex and flexible APIs with Django REST Framework.
Further Resources for Mastering Django REST Framework
To deepen your understanding of Django REST Framework and related concepts, here are some resources you might find useful:
- Python GUI: Building Interactive Applications – Discover Python’s role in game development with GUIs.
Simplifying Web Templating with Jinja in Python – Master Jinja for generating dynamic content.
Getting Started with Flask: A Quick Guide – Dive into Flask’s lightweight and flexible architecture.
Django REST Framework Official Documentation provides a comprehensive overview of the framework and its features.
Django for APIs by William S. Vincent is a great guide to building APIs with Django and Django REST Framework.
Classy Django REST Framework is a detailed guide that provides a deeper understanding of Django REST Framework.
Remember, mastering Django REST Framework is not just about learning the syntax. It’s about understanding the principles behind it and knowing how to apply them to build robust, efficient, and secure APIs.
Wrapping Up: Mastering Django REST Framework
In this comprehensive guide, we’ve journeyed through the world of Django REST Framework, a powerful toolkit for building Web APIs in Python.
We began with the basics, learning how to set up Django REST Framework and create a simple API. We then ventured into more advanced territory, exploring complex features such as authentication, permissions, viewsets, and routers. We also discussed common issues you might encounter when using Django REST Framework, such as serialization errors, and provided solutions to these challenges.
In addition to Django REST Framework, we looked at alternative approaches to handle API development in Python, comparing Django REST Framework with other Python frameworks like Flask and FastAPI. Here’s a quick comparison of these frameworks:
Framework | Flexibility | Learning Curve | Speed |
---|---|---|---|
Django REST Framework | High | Moderate | Fast |
Flask | High | Low | Moderate |
FastAPI | High | Moderate | Fast |
Whether you’re a beginner just starting out with Django REST Framework or an experienced Python developer looking to level up your API development skills, we hope this guide has given you a deeper understanding of Django REST Framework and its capabilities.
With its balance of flexibility, ease of learning, and speed, Django REST Framework is a powerful tool for API development in Python. Happy coding!