Python’s Django Framework | Complete Tutorial
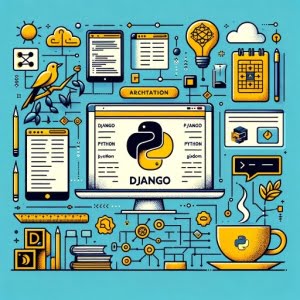
Are you on the hunt for a comprehensive Django Framework Python tutorial? You’re in the right place! Django, Python’s popular web framework, is a powerful tool for building robust web applications.
Much like a skilled architect, Django provides a solid foundation and structure for your projects, allowing you to focus on creating unique, high-quality applications.
This tutorial is designed to guide you from the basics to more advanced features of Django. We’ll explore Django’s core concepts, delve into its advanced features, and even discuss common issues and their solutions.
By the end of this tutorial, you’ll have a strong grasp of Django and be well on your way to becoming a Django master.
TL;DR: How Do I Start with Django Framework in Python?
To kickstart your journey with Django, Python’s popular web framework, you first need to install it. Django can be installed using pip, Python’s package installer. Here’s how you do it:
pip install Django
This command installs Django on your system. Once Django is installed, you can create a new project. Django provides a command-line tool, django-admin
, to create a new project. Here’s how you use it:
django-admin startproject ProjectName
Replace ‘ProjectName’ with the name you want for your project. This command creates a new Django project with the specified name.
This tutorial will guide you through the process of building a web application using Django, from setting up your project to deploying it. So, let’s dive in!
Table of Contents
- Getting Started with Django: A Beginner’s Guide
- Advanced Django Topics: Databases, Views, URLs, and Templates
- Exploring Django’s REST Framework and Advanced Topics
- Troubleshooting Common Django Issues
- Understanding MVC Architecture in Django
- Django in Real-World Projects
- Wrapping Up Your Django Framework Python Tutorial
Getting Started with Django: A Beginner’s Guide
After installing Django and creating a new project, it’s time to dive into the basics of Django. In this section, we’ll guide you through setting up a Django project, creating a simple application, understanding the project structure, and running the server.
Setting Up a Django Project
Once Django is installed, you can create a new project using the django-admin
tool. Here’s how you do it:
django-admin startproject mysite
This command creates a new Django project named ‘mysite’.
Creating a Simple Application
Inside your Django project, you can create a new application. An application is a self-contained module that encapsulates a specific functionality of the web project. To create a new application, navigate to your project directory and run:
python manage.py startapp myapp
This command creates a new Django application named ‘myapp’.
Understanding the Project Structure
A Django project has a specific structure. Here’s a brief overview:
manage.py
: This is the command-line utility that lets you interact with your project.mysite/
: This directory is the actual Python package for your project.mysite/settings.py
: This file contains all the settings for your Django project.mysite/urls.py
: This file is used to declare the URLs for your web application.myapp/
: This is the directory for your application.
Running the Server
To run your Django server, navigate to the directory containing manage.py
and run:
python manage.py runserver
# Output:
# Starting development server at http://127.0.0.1:8000/
# Quit the server with CONTROL-C.
This command starts the Django development server. You can now access your project at http://127.0.0.1:8000/
.
That’s it! You’ve set up a Django project, created an application, understood the basic project structure, and run the server. In the next section, we’ll dive into more complex Django topics.
Advanced Django Topics: Databases, Views, URLs, and Templates
After setting up your Django project and understanding the basic structure, it’s time to address more complex Django topics. In this section, we’ll cover working with databases, creating views, URL routing, and using Django’s templating engine.
Working with Databases in Django
Django comes with a high-level Object-Relational Mapping (ORM) that allows you to interact with your database, like you would with SQL. In other words, it’s a way to create, retrieve, update, and delete records in your database using Python code.
Let’s say you have a model in your Django application named ‘Blog’. You can create a new blog post like this:
from myapp.models import Blog
post = Blog(title='My First Post', content='This is my first post.')
post.save()
This creates and saves a new Blog post.
Creating Views in Django
A view in Django is a Python function that receives a web request and returns a web response. This response can be the HTML contents of a web page, a redirect, a 404 error, an XML document, an image, or anything else.
Here’s an example of a simple view in Django:
from django.http import HttpResponse
def hello(request):
return HttpResponse('Hello, World!')
This view returns a simple HTTP response saying ‘Hello, World!’.
URL Routing in Django
Django uses a URL dispatcher which maps URL patterns to views. You define these patterns in your application’s urls.py
file. Here’s an example:
from django.urls import path
from . import views
urlpatterns = [
path('hello/', views.hello, name='hello'),
]
This code maps the URL ‘/hello/’ to the ‘hello’ view.
Using Django’s Templating Engine
Django’s templating engine allows you to create dynamic HTML templates for your web pages. You can use variables, loops, and other Python constructs in your templates. Here’s a simple example:
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ content }}</p>
</body>
</html>
This is a simple HTML template with Django template tags. The variables inside the double curly braces will be replaced by their respective values.
There you have it! You’ve delved into more complex Django topics, including working with databases, creating views, URL routing, and using Django’s templating engine. Up next, we’ll discuss even more advanced Django topics.
Exploring Django’s REST Framework and Advanced Topics
As you continue your journey with Django, you’ll come across more advanced topics and alternative approaches. In this section, we’ll introduce Django’s REST framework, discuss authentication and authorization, testing, and deployment.
Django’s REST Framework for Building APIs
Django’s REST Framework is a powerful toolkit for building Web APIs. It’s easy to use and provides functionalities like serialization, viewsets, and routers. Here’s a simple example of a viewset:
from rest_framework import viewsets
from .models import Blog
from .serializers import BlogSerializer
class BlogViewSet(viewsets.ModelViewSet):
queryset = Blog.objects.all()
serializer_class = BlogSerializer
This code defines a viewset for the ‘Blog’ model. The viewset automatically provides ‘list’, ‘create’, ‘retrieve’, ‘update’, and ‘destroy’ actions.
Authentication and Authorization in Django
Django provides several methods for authentication and authorization. It includes a built-in User model and authentication system. Here’s an example of how to authenticate a user in Django:
from django.contrib.auth import authenticate, login
def login_view(request):
user = authenticate(request, username='myuser', password='mypassword')
if user is not None:
login(request, user)
return HttpResponse('Logged in')
else:
return HttpResponse('Invalid credentials')
This code authenticates a user and logs them in. If the credentials are invalid, it returns ‘Invalid credentials’.
Testing in Django
Django provides a built-in testing framework that you can use to write unit tests for your application. Here’s an example of a simple test case:
from django.test import TestCase
from .models import Blog
class BlogModelTest(TestCase):
@classmethod
def setUpTestData(cls):
Blog.objects.create(title='My First Post', content='This is my first post.')
def test_title_content(self):
post = Blog.objects.get(id=1)
expected_title = f'{post.title}'
expected_content = f'{post.content}'
self.assertEqual(expected_title, 'My First Post')
self.assertEqual(expected_content, 'This is my first post.')
This code creates a test case for the ‘Blog’ model. It tests whether the title and content of the post are correct.
Deployment in Django
Django applications can be deployed on several platforms, including Heroku, AWS, and Google Cloud. The deployment process involves setting up a production-ready database, serving static files, and configuring your production settings.
There you have it! You’ve explored Django’s REST framework, delved into authentication and authorization, learned about testing, and brushed up on deployment. With these advanced topics under your belt, you’re well on your way to becoming a Django expert.
Troubleshooting Common Django Issues
Like any technology, Django has its quirks and potential pitfalls. In this section, we’ll discuss some common issues encountered when working with Django and provide solutions to these problems.
Dealing with Migrations
Migrations are Django’s way of propagating changes you make to your models (adding a field, deleting a model, etc.) into your database schema. However, sometimes you might encounter issues when running migrations. For instance, you might see a django.db.migrations.exceptions.InconsistentMigrationHistory
error. This usually arises when there are conflicts between migrations.
To resolve this, you can use the makemigrations
command with the --merge
flag:
python manage.py makemigrations --merge
This command tells Django to create a new migration that merges the conflicting migrations.
Debugging Template Errors
Django’s templating engine is powerful, but it can sometimes be a source of errors. For instance, you might encounter a TemplateDoesNotExist
error. This error occurs when Django is unable to find the specified template.
To resolve this, ensure that your templates are in the correct directory and that the TEMPLATES
setting in your settings.py
file is correctly configured.
Managing Static Files
Django’s static file handling can sometimes be a source of confusion. If your static files are not being served, ensure that STATIC_URL
and STATIC_ROOT
are correctly set in your settings.py
file. Also, remember to run collectstatic
command when deploying your application:
python manage.py collectstatic
This command tells Django to collect all static files in your application in one place so they can be served efficiently.
Handling Form Errors
Django’s form system is a powerful tool for handling form data and validation. However, it can sometimes be a source of confusion, especially when dealing with form errors. If you’re having trouble with form errors, make sure to call form.is_valid()
before accessing form.cleaned_data
.
These are just a few common issues you might encounter when working with Django. Remember, when in doubt, Django’s extensive documentation and the broader Django community are excellent resources for troubleshooting and learning.
Understanding MVC Architecture in Django
Before diving deeper into Django, it’s crucial to understand the architectural pattern it follows – the Model-View-Controller (MVC) architecture. This pattern is a staple in web development, with Django providing a slightly modified version of it, often referred to as the Model-View-Template (MVT) pattern.
What is MVC Architecture?
MVC stands for Model-View-Controller. It’s a design pattern that separates an application into three interconnected components:
- Model: The Model represents the application’s data and the rules to manipulate that data.
- View: The View is what’s presented to the users. It’s a representation of the data, such as a chart, diagram, or table.
- Controller: The Controller is the link between the Model and the View. It processes all the business logic and incoming requests, manipulates data using the Model, and interacts with the Views to render the final output.
Django’s Take on MVC: The MVT Pattern
In Django, this pattern is slightly modified. Django handles the Controller part itself and leaves us with the Model and View, which is why it’s often referred to as the Model-View-Template (MVT) pattern. The Template here is Django’s presentation layer, which handles the part of the View in MVC.
Why Web Frameworks like Django Matter
Web frameworks like Django play a crucial role in web development. They provide a structured way for developers to build web applications without worrying about low-level details such as protocol management or thread management. Django, in particular, is designed to help developers take applications from concept to completion as quickly as possible.
It provides high-level abstractions of common web development patterns, shortcuts for frequent programming tasks, and clear conventions for how to solve problems. This allows you to focus on writing your app without needing to reinvent the wheel. It’s also flexible, scalable, and versatile, making it a great choice for developers of all skill levels.
In the upcoming sections, we’ll delve into practical examples of using Django to build web applications, leveraging its MVT architecture.
Django in Real-World Projects
Having explored the fundamentals and advanced topics of Django, you might be wondering about its application in real-world projects. Django’s versatility makes it a suitable choice for a variety of applications, from small-scale projects to complex, high-traffic sites like Instagram and Disqus.
Django’s Support for AJAX
Django supports AJAX out of the box. AJAX, short for Asynchronous JavaScript and XML, allows for the creation of interactive applications that can update and retrieve data from the server asynchronously, without interfering with the display and behavior of the existing page. It’s widely used in Django projects to enhance user experience.
Django Channels for Real-Time Applications
Django Channels extends Django to handle WebSockets, HTTP2, and other protocols. It’s a project that takes Django and extends its abilities beyond HTTP, to handle WebSockets, chat protocols, IoT protocols, and more. It’s particularly useful for building real-time applications, which are becoming increasingly prevalent in today’s web landscape.
# An example of a basic consumer that accepts WebSocket connections
from channels.generic.websocket import AsyncWebsocketConsumer
import json
class ChatConsumer(AsyncWebsocketConsumer):
async def connect(self):
await self.accept()
async def disconnect(self, close_code):
pass
async def receive(self, text_data):
text_data_json = json.loads(text_data)
message = text_data_json['message']
await self.send(text_data=json.dumps({
'message': message
}))
# Output:
# A WebSocket connection that sends back all received data.
The above example is a simple Django Channels consumer that accepts WebSocket connections and sends back the received data. It’s a basic example of how Django Channels can be used to handle WebSocket connections for real-time applications.
Further Resources for Python Django
Moving forward with Python Django, it’s important to have access to comprehensive resources that will fuel your understanding and ease your development processes. These materials will offer insights, best practices, and tutorials on Django and other Python tools:
- IOFlood’s Python GUI Article – Explore PyQt, Tkinter, and other popular Python GUI libraries.
Flask: Building Web Applications in Python – Explore Flask for web development in Python.
Python GUI Development with Tkinter: Quick Guide – Explore tkinter for creating Python GUIs.
Learn Django in Visual Studio – Enhance your Django skills inside the Visual Studio environment.
Django Tutorial on GeeksForGeeks – A comprehensive tutorial covering the core aspects of Django.
Official Django Documentation – The ultimate guide to Django provided by the creators themselves.
In conclusion, building upon your Django knowledge and knowing where to find solutions can boost your efficiency as a developer. Django is a powerful tool for web development, and these resources are your aid to master it successfully.
Wrapping Up Your Django Framework Python Tutorial
Throughout this comprehensive Django tutorial, we’ve covered a vast range of topics, from basic setup to advanced features.
We started by installing Django and creating a new project using django-admin startproject
. We then explored the structure of a Django project, including its applications, settings, and URL configurations.
We dove into the database aspect of Django, discussing how Django’s ORM allows us to interact with our database using Python. We also covered views and URL routing, two crucial components of any Django application. We then discussed Django’s templating engine and how it allows us to create dynamic HTML templates.
We ventured into advanced topics, like Django’s REST Framework for building APIs, authentication and authorization, testing, and deployment. We also discussed some common issues that might come up when working with Django and how to troubleshoot them.
Finally, we looked at the real-world applications of Django, including its support for AJAX, Django Channels for real-time applications, and more. We hope this tutorial has provided a solid foundation for your Django journey and has equipped you with the knowledge and skills to start building your own Django applications.
Here’s a quick comparison of the methods we discussed:
Method | Use |
---|---|
django-admin startproject | To create a new Django project |
python manage.py startapp | To create a new Django application |
python manage.py runserver | To start the Django development server |
python manage.py makemigrations --merge | To resolve migration conflicts |
python manage.py collectstatic | To collect all static files in one place for deployment |
Remember, Django’s versatility and robust set of features make it a powerful tool for web development. Keep exploring, keep learning, and happy coding!