Python enumerate() | Function Guide (With Examples)
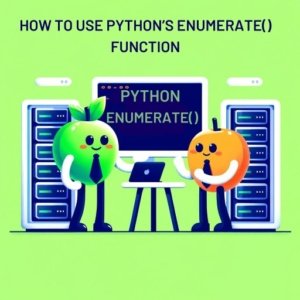
Understanding the enumerate function in Python is crucial while programming scripts for use at IOFLOOD, enabling iteration over iterable objects with index and value pairs. In our experience, the enumerate function simplifies code logic and enhances readability, particularly in scenarios requiring indexing and iteration simultaneously. Today’s article delves into how to effectively use the enumerate function in Python, providing examples and explanations to empower our dedicated server customers with commonly used scripting techniques for their bare metal servers.
In this comprehensive guide, we will traverse the landscape of the Python enumerate function, exploring its basic use and even delving into some advanced techniques. Once again, our aim is to equip you with the knowledge and skills to utilize this function effectively in your Python programming journey.
So, buckle up and let’s get started on this exciting tour of the Python enumerate function!
TL;DR: How Do I Use the Enumerate Function in Python?
The
enumerate
function in Python is a built-in function that adds a counter to aniterable
and returns it as anenumerate
object, commonly used within afor
loop. This counter can be used to keep track of the index position while iterating over the iterable.
Here’s a simple example:
for i, value in enumerate(['apple', 'banana', 'cherry']):
print(i, value)
# Output:
# 0 apple
# 1 banana
# 2 cherry
In this example, we have a list of fruits. We use the enumerate function in a for loop to iterate over this list. The enumerate function returns a tuple for each item in the list. This tuple contains the index of the item and the item itself, which we unpack into i
and value
. We then print out the index and value, which gives us the output shown.
Intrigued? Keep reading for a deeper dive into the Python enumerate function, including more detailed explanations and advanced usage scenarios.
Table of Contents
- Python Enumerate: Your Iteration Companion
- Level Up: Advanced Python Enumerate
- Exploring Alternatives to Python Enumerate
- Troubleshooting Python Enumerate: Common Pitfalls
- Python Iterables: The Building Blocks of Enumeration
- Enumerate in the Wild: Real-World Applications
- Further Resources for Python Iterator Mastery
- Wrapping Up: Python Enumerate Uncovered
Python Enumerate: Your Iteration Companion
The basic use of the Python enumerate function involves adding a counter to an iterable. An iterable in Python is any object capable of returning its elements one at a time, such as a list, tuple, or string. The enumerate function enhances the iteration process by providing a built-in counter that tracks the current iteration number.
Let’s see this in action with a simple code example:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f'At index {i}, the fruit is {fruit}.')
# Output:
# At index 0, the fruit is apple.
# At index 1, the fruit is banana.
# At index 2, the fruit is cherry.
In this example, we have a list of fruits. We use the enumerate function in a for loop to iterate over this list. The enumerate function returns a tuple for each item in the list. This tuple contains the index of the item (starting from 0) and the item itself, which we unpack into i
and fruit
. We then print out the index and fruit in a formatted string, which gives us the output shown.
The Python enumerate function is a powerful tool for iterating over sequences, but it’s not without its quirks. One potential pitfall to be aware of is that the counter starts at 0 by default. This is standard in Python, but it can cause confusion if you’re expecting the counter to start at 1. Stay tuned for our discussion on advanced use where we’ll show you how to customize the start of the counter.
Level Up: Advanced Python Enumerate
As you become more proficient in Python, you’ll find that the enumerate function has more to offer than just basic iteration. One of its advanced features is the optional start
parameter. This parameter allows you to customize the initial count of the enumeration.
Let’s take a look at how this works in the following code snippet:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits, start=1):
print(f'Fruit number {i} is {fruit}.')
# Output:
# Fruit number 1 is apple.
# Fruit number 2 is banana.
# Fruit number 3 is cherry.
In this example, we’ve added a start
parameter to the enumerate function. This parameter sets the initial count to 1 instead of the default 0. So when we print out the fruit number and fruit, the output starts from 1.
This feature is particularly useful when you want your enumeration to align with a more human-friendly counting system (i.e., starting from 1 instead of 0). It adds an extra layer of flexibility to the Python enumerate function, making it an even more powerful tool in your Python arsenal.
Exploring Alternatives to Python Enumerate
While the Python enumerate function is a powerful tool, it’s not the only way to keep track of your position in an iterable. An alternative approach is using a counter variable in a for loop.
Let’s see how this works in practice:
fruits = ['apple', 'banana', 'cherry']
index = 1
for fruit in fruits:
print(f'Fruit number {index} is {fruit}.')
index += 1
# Output:
# Fruit number 1 is apple.
# Fruit number 2 is banana.
# Fruit number 3 is cherry.
In this example, we manually create a counter variable index
and increment it by 1 in each iteration of the loop. This gives us the same output as the previous example where we used the start
parameter of the enumerate function.
While this method can be useful in some cases, it does have its drawbacks. The main one is that it requires more lines of code, which can make your script longer and more difficult to read. Moreover, you need to remember to increment the counter in each iteration, which can be a source of bugs if forgotten.
In contrast, the Python enumerate function handles the counter automatically, making your code cleaner and more efficient. It’s generally best to use the enumerate function when you need to track the index in an iterable, but it’s good to know that you have alternatives in case you need them.
Troubleshooting Python Enumerate: Common Pitfalls
As you journey through the world of Python programming, you’re bound to encounter some obstacles. The enumerate function, despite its usefulness, is no exception. Let’s discuss some common errors and their solutions, along with best practices for using the Python enumerate function.
Error: Forgetting to Unpack the Tuple
One common mistake when using the Python enumerate function is forgetting to unpack the tuple it returns. Let’s see what happens when we do this:
fruits = ['apple', 'banana', 'cherry']
for item in enumerate(fruits):
print(item)
# Output:
# (0, 'apple')
# (1, 'banana')
# (2, 'cherry')
In this example, we didn’t unpack the tuple returned by the enumerate function. As a result, the entire tuple is printed in each iteration.
To fix this, we need to unpack the tuple into two variables, like so:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(i, fruit)
# Output:
# 0 apple
# 1 banana
# 2 cherry
Best Practice: Use the Start Parameter Sparingly
While the start
parameter of the enumerate function can be useful, it’s best to use it sparingly. This is because Python, like most programming languages, uses zero-based indexing. This means that the first item in a list is at index 0, not 1. If you use the start
parameter to change this, it could confuse other developers who are used to zero-based indexing.
In conclusion, the Python enumerate function is a powerful tool, but it’s not without its quirks. By being aware of these common errors and best practices, you can use the enumerate function effectively and efficiently in your Python programming journey.
Python Iterables: The Building Blocks of Enumeration
Before we delve further into the Python enumerate function, it’s crucial to understand the concept of iterables in Python. An iterable is any Python object capable of returning its members one at a time. This includes common data types like lists, tuples, strings, and dictionaries.
Here’s a simple example of an iterable:
# A list is an iterable
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, fruits
is a list, which is an iterable. We use a for loop to iterate over the list and print each fruit. The output is the name of each fruit on a new line.
The Python enumerate function works with any iterable. It takes an iterable as input and returns an enumerate object, which is itself an iterable. This enumerate object contains tuples, where the first item is the index and the second item is the corresponding item from the input iterable.
Understanding iterables is the first step to mastering the Python enumerate function. With this knowledge in hand, you’re well on your way to becoming a Python enumerate expert.
Enumerate in the Wild: Real-World Applications
The Python enumerate function is not limited to simple iterations over lists or tuples. Its utility extends to larger scripts and projects, where it can help track indices in more complex data structures, like nested lists or dictionaries.
For instance, consider a scenario where you’re working with a list of dictionaries, each representing a user with a ‘name’ and ’email’ key. Using enumerate, you can easily track the index of each user in the list:
users = [
{'name': 'Alice', 'email': '[email protected]'},
{'name': 'Bob', 'email': '[email protected]'},
{'name': 'Charlie', 'email': '[email protected]'},
]
for i, user in enumerate(users):
print(f'User {i+1}: {user['name']} ({user['email']})')
# Output:
# User 1: Alice ([email protected])
# User 2: Bob ([email protected])
# User 3: Charlie ([email protected])
In this example, enumerate allows us to print out each user’s position in the list along with their name and email.
In addition to its standalone utility, the Python enumerate function often finds itself in the company of other Python functions and constructs. For example, it pairs well with the zip
function when you need to iterate over multiple iterables in parallel.
Stay tuned for more guides that delve into these related topics, offering a more detailed exploration of Python’s powerful and versatile built-in functions.
Further Resources for Python Iterator Mastery
To help expand your skills and comprehension of Python control structures, here are a selection of insightful resources:
- Python Loop Basics Covered – A quick tutorial on Python loops and data processing.
Breaking Out of Loops with Python “break” – Explore how “break” exits loops based on specific conditions.
Iteration with Python “for” Loop – Learn how to create and customize “for” loops for various programming tasks.
Python ‘While’ Loop Guide on Real Python provides an in-depth exploration of Python’s ‘while’ loop.
Python Generators by Tutorialspoint discusses Python generators in detail.
Control Statements in Python – An article from Python Tricks detailing the usage of control statements in Python.
Leverage these materials and continue your journey towards mastering these crucial Python concepts.
Wrapping Up: Python Enumerate Uncovered
In this comprehensive guide, we’ve explored the Python enumerate function in depth. From basic usage to advanced techniques, we’ve covered how this function can simplify your iteration tasks and make your Python code cleaner and more efficient.
Here’s a quick recap of the key points we’ve covered:
- Basic Use: The Python enumerate function adds a counter to an iterable, making it easier to track your position in the iterable. For example:
for i, value in enumerate(['apple', 'banana', 'cherry']): print(i, value) # Outputs: 0 apple, 1 banana, 2 cherry
- Advanced Use: The enumerate function offers an optional
start
parameter that allows you to customize the initial count of the enumeration.for i, value in enumerate(['apple', 'banana', 'cherry'], start=1): print(i, value) # Outputs: 1 apple, 2 banana, 3 cherry
- Alternative Approaches: While the enumerate function is a powerful tool, you can also use a counter variable in a for loop as an alternative approach.
Troubleshooting and Considerations: Be aware of common errors when using the enumerate function, such as forgetting to unpack the tuple it returns. Also, remember that the
start
parameter should be used sparingly to avoid confusion.Beyond: The Python enumerate function can be used in larger scripts and projects, and often pairs well with other Python functions and constructs.
With this knowledge, you’re now well-equipped to use the Python enumerate function in your coding journey. Happy coding!