Java Iterable Interface: Guide and Examples
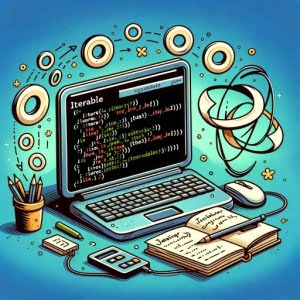
Do you find the Iterable interface in Java puzzling? You’re not alone. Many developers find themselves in a maze when it comes to understanding and implementing the Iterable interface in Java. Think of the Iterable interface as a tour guide, leading you through each element of a collection in a systematic manner.
In this guide, we’ll navigate through the Iterable interface in Java, from its basic usage to more advanced techniques. We’ll cover everything from implementing the Iterable interface in a class, discussing its advantages and potential pitfalls, to exploring alternative approaches for iterating over collections.
So, let’s embark on this journey and master the Java Iterable interface!
TL;DR: How Do I Use the Iterable Interface in Java?
The Iterable interface in Java is used to allow an object to be the target of the ‘for-each loop’ statement. Once the class interface is defined, you can create a new instance with the syntax,
MyIterable iterable = new MyIterable();
and use it in a loop with the line,for (String s : iterable) {}
. It provides a way to iterate over a collection of items.
Here’s a simple example:
class MyIterable implements Iterable<String> {
//...implementation details
}
MyIterable iterable = new MyIterable();
for (String s : iterable) {
System.out.println(s);
}
# Output:
# Depends on the implementation of MyIterable
In this example, we’ve created a class MyIterable
that implements the Iterable
interface. We then create an instance of MyIterable
and use a for-each loop to iterate over each element, printing each string to the console.
This is just a basic way to use the Iterable interface in Java, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Java Iterable Interface
- Iterable Interface with Custom Data Structures
- Exploring Alternatives: Iterators and Streams
- Common Issues with Java Iterable Interface
- Unraveling the Java Iterable Interface
- Iterable Interface in Real-World Applications
- Expanding Your Java Knowledge
- Wrapping Up: Java Iterable Interface
Getting Started with Java Iterable Interface
The Iterable interface is a core part of Java and is used for enabling objects to be the target of the ‘for-each loop’ statement. It is present in the java.lang
package, and any class implementing this interface allows its objects to be iterated using the enhanced for loop.
Let’s start with a simple example of how to implement the Iterable interface in a class:
import java.util.Iterator;
import java.util.Arrays;
class MyIterable implements Iterable<String> {
private final String[] array = {"Apple", "Banana", "Cherry"};
public Iterator<String> iterator() {
return Arrays.asList(array).iterator();
}
}
public class Main {
public static void main(String[] args) {
MyIterable iterable = new MyIterable();
for (String s : iterable) {
System.out.println(s);
}
}
}
# Output:
# Apple
# Banana
# Cherry
In this example, we’ve created a class MyIterable
that implements the Iterable
interface. We’ve defined an array of strings and the iterator()
method returns an Iterator for this array. In the main
method, we create an instance of MyIterable
and use a for-each loop to iterate over each element, printing each string to the console.
Advantages of Using Iterable Interface
Implementing the Iterable interface has several advantages. It provides a simple and consistent way to iterate over collections, and it allows your class to be used with the enhanced for loop, making your code more readable and less prone to errors.
Potential Pitfalls of Iterable Interface
However, there are potential pitfalls when using the Iterable interface. One common mistake is failing to implement the iterator()
method correctly. If the method doesn’t return a proper Iterator, it can lead to unexpected behavior or runtime errors. Always ensure that your iterator()
method is correctly implemented.
Iterable Interface with Custom Data Structures
As you become more comfortable with the Iterable interface, you can start to explore more complex uses. A common scenario is using the Iterable interface with custom data structures. Let’s dive into an example where we use the Iterable interface with a custom LinkedList data structure.
import java.util.Iterator;
import java.util.NoSuchElementException;
class Node {
int data;
Node next;
}
class LinkedList implements Iterable<Node> {
Node head;
public Iterator<Node> iterator() {
return new Iterator<Node>() {
private Node current = head;
public boolean hasNext() {
return current != null;
}
public Node next() {
if (current == null) throw new NoSuchElementException();
Node node = current;
current = current.next;
return node;
}
};
}
}
public class Main {
public static void main(String[] args) {
LinkedList list = new LinkedList();
//...Add nodes to the list
for (Node node : list) {
System.out.println(node.data);
}
}
}
# Output:
# Depends on the nodes added to the list
In this example, we’ve created a custom LinkedList data structure. Each Node in the LinkedList contains an integer data and a reference to the next Node. The LinkedList class implements the Iterable interface, and the iterator() method returns an anonymous inner class that defines how to iterate through the Nodes in the LinkedList.
Pros and Cons of Using Iterable with Custom Data Structures
Using the Iterable interface with custom data structures allows you to use the enhanced for loop with your data structure, which can simplify your code and make it more readable. However, it requires a correct implementation of the iterator() method, which can be complex for custom data structures. If the iterator() method is not correctly implemented, it can lead to unexpected behavior or runtime errors.
Exploring Alternatives: Iterators and Streams
While the Iterable interface is a powerful tool for iterating over collections, Java provides other ways to achieve the same goal. Two such alternatives are using Iterators directly and using Streams.
Direct Use of Iterators
Iterators are the backbone of the Iterable interface. They provide the actual mechanism for traversing through a collection. You can use an Iterator directly without implementing the Iterable interface.
import java.util.ArrayList;
import java.util.Iterator;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String s = iterator.next();
System.out.println(s);
}
}
}
# Output:
# Apple
# Banana
# Cherry
In this example, we create an ArrayList and directly use its iterator to traverse through its elements. This approach gives you more control over the iteration process but makes the code slightly more verbose.
Using Streams
Java 8 introduced the concept of Streams, which provide a more declarative approach to working with collections.
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream.of("Apple", "Banana", "Cherry").forEach(System.out::println);
}
}
# Output:
# Apple
# Banana
# Cherry
In this example, we create a Stream of strings and use the forEach
method to print each element. Streams provide a rich set of operations like filter
, map
, and reduce
that can make your code more expressive and easier to read.
Comparison of Methods
Method | Advantages | Disadvantages |
---|---|---|
Iterable | Simple, consistent, works with for-each loop | Requires correct implementation of iterator() |
Iterator | More control over iteration | More verbose, risk of ConcurrentModificationException |
Stream | Declarative, expressive, rich set of operations | Overkill for simple iterations, performance overhead for small collections |
While the Iterable interface is usually the go-to method for iterating over collections, consider using Iterators or Streams when they better fit your needs.
Common Issues with Java Iterable Interface
While working with the Iterable interface, you might encounter some common issues. Understanding these issues and knowing how to handle them can save you a lot of debugging time.
Dealing with ConcurrentModificationException
One common issue when using the Iterable interface is the ConcurrentModificationException
. This exception is thrown when a collection is modified while it is being iterated over.
import java.util.ArrayList;
import java.util.Iterator;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String s = iterator.next();
if ("Banana".equals(s)) {
list.remove(s);
}
}
}
}
# Output:
# Throws ConcurrentModificationException
In this example, we try to remove an element from the list while iterating over it, which leads to ConcurrentModificationException
.
To avoid this, you can use the remove()
method of the Iterator. This method safely removes the current element from the underlying collection.
while (iterator.hasNext()) {
String s = iterator.next();
if ("Banana".equals(s)) {
iterator.remove();
}
}
This code will remove the “Banana” element from the list without throwing an exception.
Other Considerations
When using the Iterable interface, keep in mind that the iterator()
method should return a new Iterator each time it is called. Returning the same Iterator can lead to unexpected behavior.
Also, be aware that the Iterator does not guarantee any specific order of elements unless the underlying collection has a defined order, like ArrayList
or LinkedList
.
Unraveling the Java Iterable Interface
To fully grasp the use of the Iterable interface in Java, it’s crucial to understand the underlying concepts and how they relate to each other. The Iterable interface and the for-each loop are tightly connected, with the Iterator interface serving as the bridge between them.
The Iterable Interface
The Iterable interface is part of the java.lang
package. It is designed to provide a standard way to iterate over collections of objects. The interface itself is quite simple, with just one method to implement: iterator()
. This method returns an Iterator over the elements in the collection.
public interface Iterable<T> {
Iterator<T> iterator();
}
The Iterator Interface
The Iterator interface is the workhorse behind the Iterable interface. It provides the mechanism for iterating over a collection. The Iterator interface has three methods: hasNext()
, next()
, and remove()
.
public interface Iterator<E> {
boolean hasNext();
E next();
void remove(); //optional
}
The hasNext()
method returns true if the iteration has more elements. The next()
method returns the next element in the iteration. The remove()
method removes the last element returned by the iterator from the underlying collection.
The For-Each Loop
The for-each loop, also known as the enhanced for loop, is a simplified syntax for iterating over collections of objects. It works with any object that implements the Iterable interface.
for (String s : iterable) {
System.out.println(s);
}
In this example, iterable
is an object that implements the Iterable interface. The for-each loop internally uses the Iterator returned by the iterator()
method to iterate over the elements.
Understanding these fundamental concepts and how they interrelate is key to effectively using the Iterable interface in Java.
Iterable Interface in Real-World Applications
The Iterable interface is not just a theoretical concept; it has practical relevance in real-world applications. For instance, it plays a crucial role in database access and file I/O operations in Java.
Iterable in Database Access
In database operations, the Iterable interface can be used to iterate over a result set from a database query. This allows you to process each row of the result set one at a time, reducing memory usage and improving performance when dealing with large result sets.
Iterable in File I/O
When dealing with file I/O, the Iterable interface can be used to read or write to a file line by line. This can be particularly useful when working with large files that cannot be loaded into memory all at once.
Expanding Your Java Knowledge
While understanding the Iterable interface is a significant step, there are other related concepts that are worth exploring to deepen your Java knowledge. The Java Collections Framework, for example, offers a unified architecture for representing and manipulating collections. Generics, on the other hand, provide type safety and can be used with classes, interfaces, and methods.
Further Resources for Mastering Java Iterable Interface
Here are some additional resources to help you delve deeper into the world of Java Iterable and related concepts:
- Beginner’s Guide to Java Interfaces – Discover how Java interfaces enable multiple inheritance.
Exploring Predicates in Java – Learn how to use Predicate interface and its test method for evaluating conditions.
Comparator Usage in Java – Master Comparator usage for flexible and customizable sorting in Java collections.
Java Collections Framework Tutorial by Oracle covers the Java Collections Framework, including the Iterable interface.
Generics in Java by Baeldung dives deep into the concept of Generics in Java, a feature that works hand-in-hand with collections.
Java I/O Tutorial by Jenkov covers Java’s File I/O APIs, where the Iterable interface is commonly used.
Wrapping Up: Java Iterable Interface
In this comprehensive guide, we’ve journeyed through the world of the Iterable interface in Java, a fundamental tool for efficient iteration over collections.
We began with the basics, learning how to implement and use the Iterable interface in a class. We then ventured into more advanced territory, discussing its usage with custom data structures, and exploring common issues and their solutions. Along the way, we’ve also touched on the underlying concepts of the Iterator interface and the for-each loop, providing you with a solid foundation for understanding and using the Iterable interface.
We also explored alternative approaches for iterating over collections. We looked at the direct use of Iterators and the use of Streams, each with its own advantages and potential pitfalls. Here’s a quick comparison of these methods:
Method | Advantages | Disadvantages |
---|---|---|
Iterable | Simple, consistent, works with for-each loop | Requires correct implementation of iterator() |
Iterator | More control over iteration | More verbose, risk of ConcurrentModificationException |
Stream | Declarative, expressive, rich set of operations | Overkill for simple iterations, performance overhead for small collections |
Whether you’re just starting out with the Iterable interface in Java or you’re looking to level up your iteration skills, we hope this guide has given you a deeper understanding of the Iterable interface and its capabilities.
With its balance of simplicity, consistency, and flexibility, the Iterable interface is a powerful tool for handling iteration in Java. Now, you’re well equipped to enjoy those benefits. Happy coding!