Java Comparator Interface: Sorting with Comparator
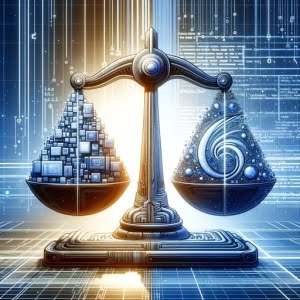
Ever felt like you’re wrestling with sorting collections in Java? You’re not alone. Many developers find Java’s Comparator interface a bit daunting. Think of Java’s Comparator as a librarian – a librarian that helps you sort collections of objects, just like a librarian organizes books.
Comparators are a powerful tool to extend the functionality of your Java code, making them extremely popular for sorting collections of objects.
In this guide, we’ll walk you through the process of using Java Comparators, from the basics to more advanced techniques. We’ll cover everything from implementing the compare method, handling different types of sorting requirements, to dealing with common issues and even troubleshooting.
Let’s dive in and start mastering Java Comparators!
TL;DR: How Do I Use a Comparator in Java?
In Java you can use the Comparator interface and its
compare
method to sort collections, with the syntaxComparator<Integer> comparator = Integer::compare;
. The Comparator interface provides a powerful way to define custom sorting rules for collections in Java.
Here’s a simple example:
Comparator<Integer> comparator = Integer::compare;
List<Integer> list = Arrays.asList(3, 1, 4, 1, 5, 9);
Collections.sort(list, comparator);
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, we’ve created a Comparator for Integers using a method reference to the Integer class’s compare method. We then use this Comparator to sort a list of integers in ascending order using the Collections.sort method.
This is just a basic way to use a Comparator in Java, but there’s much more to learn about sorting collections in Java. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
Java Comparator: Sorting Basics
The Comparator interface in Java is used primarily for sorting collections of objects. It provides a powerful and flexible way to define custom sorting rules.
Here’s how it works:
A Comparator is simply an object that represents an ordering of objects of a specific type. It has a single method, compare
, which takes two objects and returns a negative integer, zero, or a positive integer if the first object is less than, equal to, or greater than the second, respectively.
Let’s look at a simple example of using a Comparator to sort a list of integers in ascending order:
Comparator<Integer> comparator = new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o1 - o2;
}
};
List<Integer> list = Arrays.asList(3, 1, 4, 1, 5, 9);
Collections.sort(list, comparator);
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, we’ve created an anonymous class that implements the Comparator interface for Integers. The compare
method is implemented to subtract the second integer from the first. This results in a negative number if the first integer is less than the second, zero if they’re equal, and a positive number if the first is greater than the second. This ordering results in the list being sorted in ascending order.
The advantage of using a Comparator is that it allows you to define custom sorting rules, such as sorting in descending order, or sorting by multiple fields in an object. However, one potential pitfall is that the compare
method must be implemented correctly to avoid unexpected results. For example, subtracting two integers can result in an overflow if the integers are very large, which can lead to incorrect sorting.
Chaining Comparators and Stream Sorting
As you delve deeper into the world of Java Comparators, you’ll find that they offer more advanced features that can add flexibility and efficiency to your code. Two such features are chaining comparators for multi-field sorting and using comparators with streams.
Multi-field Sorting with Comparator Chaining
Java Comparators can be chained together to perform complex, multi-field sorting. This is particularly useful when you want to sort by one field, and then by another if the first fields are equal.
Here’s an example where we sort a list of students first by grade, and then by name:
class Student {
String name;
int grade;
// Constructor, getters and setters omitted for brevity
}
List<Student> students = Arrays.asList(
new Student("Alice", 90),
new Student("Bob", 90),
new Student("Charlie", 85)
);
Comparator<Student> comparator = Comparator
.comparingInt(Student::getGrade)
.reversed()
.thenComparing(Student::getName);
students.sort(comparator);
# Output:
# Bob, 90
# Alice, 90
# Charlie, 85
In this example, we first create a Comparator that compares students based on their grade. We then reverse this comparator to sort in descending order of grade. Finally, we chain another comparator that compares students by their name. This results in the students being sorted first by grade (in descending order), and then by name (in ascending order) if the grades are equal.
Sorting Streams with Comparators
Java 8 introduced streams, which provide a powerful way to process collections of data. One of the features of streams is the ability to sort them using a Comparator.
Here’s an example where we sort a stream of integers in descending order:
List<Integer> list = Arrays.asList(3, 1, 4, 1, 5, 9);
List<Integer> sortedList = list.stream()
.sorted(Comparator.reverseOrder())
.collect(Collectors.toList());
# Output:
# [9, 5, 4, 3, 1, 1]
In this example, we create a stream from the list of integers, sort it in descending order using a reversed Comparator, and then collect the results into a new list.
Using Comparators with streams can provide a more declarative and often more readable way to sort collections, especially when combined with other stream operations.
Exploring Alternatives to Java Comparators
While Java Comparators are a powerful tool for sorting collections, they’re not the only game in town. There are other methods you can use to sort collections in Java, such as the Comparable interface and third-party libraries.
Sorting with the Comparable Interface
The Comparable interface is another way to define custom sorting rules for collections in Java. Unlike Comparators, which allow you to define multiple different orderings for a type, the Comparable interface is used to define a natural ordering for a type.
Here’s an example of sorting a list of strings in reverse natural order using the Comparable interface:
List<String> list = Arrays.asList("Alice", "Bob", "Charlie");
Collections.sort(list, Collections.reverseOrder());
# Output:
# ["Charlie", "Bob", "Alice"]
In this example, we use the Collections.sort
method with Collections.reverseOrder
to sort the list in reverse natural order. The reverseOrder
method returns a Comparator that imposes the reverse of the natural ordering on a collection of objects that implement the Comparable interface.
Sorting with Third-Party Libraries
There are also several third-party libraries available that provide powerful and flexible sorting capabilities. One popular library is Apache Commons Lang, which provides a builder-style API for creating complex Comparator instances.
Here’s an example of sorting a list of strings by length using Apache Commons Lang:
List<String> list = Arrays.asList("Alice", "Bob", "Charlie");
Comparator<String> comparator = new CompareToBuilder()
.appendSuper(super.compareTo(o))
.append(this.lastName, rhs.lastName)
.append(this.firstName, rhs.firstName)
.toComparison();
Collections.sort(list, comparator);
# Output:
# ["Bob", "Alice", "Charlie"]
In this example, we use the CompareToBuilder
class from Apache Commons Lang to create a Comparator that sorts strings by their length. We then use this Comparator to sort the list.
While these alternative approaches to sorting collections in Java can be useful in certain situations, they each have their own trade-offs. Using the Comparable interface can be simpler for basic sorting needs, but it lacks the flexibility of Comparators. On the other hand, using third-party libraries can provide additional features and a more fluent API, but they also add an extra dependency to your project.
Troubleshooting Java Comparators
While Java Comparators are a powerful tool for sorting collections, you may run into some common issues when using them. In this section, we’ll discuss these issues and provide solutions and workarounds.
Handling Null Values
One common issue when using Comparators is dealing with null values. If the compare
method is called with a null argument, it will throw a NullPointerException
. To handle this, you can create a custom Comparator that treats null values as being less than any non-null value:
Comparator<String> nullSafeComparator = Comparator.nullsFirst(Comparator.naturalOrder());
List<String> list = Arrays.asList("Alice", null, "Bob");
Collections.sort(list, nullSafeComparator);
# Output:
# [null, "Alice", "Bob"]
In this example, we use the Comparator.nullsFirst
method to create a null-safe Comparator. This Comparator treats null values as being less than any non-null value, so when we sort the list, the null value ends up at the beginning.
Sorting in Reverse Order
Another common issue is sorting in reverse order. While you can reverse a Comparator using the Comparator.reversed
method, this can be confusing if you’re not familiar with it. Here’s an example of sorting a list of integers in descending order:
Comparator<Integer> comparator = Comparator.reverseOrder();
List<Integer> list = Arrays.asList(3, 1, 4, 1, 5, 9);
Collections.sort(list, comparator);
# Output:
# [9, 5, 4, 3, 1, 1]
In this example, we use the Comparator.reverseOrder
method to create a Comparator that sorts in reverse of the natural ordering. We then use this Comparator to sort the list in descending order.
By understanding these common issues and how to handle them, you can use Java Comparators more effectively and avoid potential pitfalls.
Understanding Java Comparators: Background and Fundamentals
Before we dive further into the practical applications of Java Comparators, it’s essential to understand the fundamental concepts that underpin this powerful feature of the Java programming language.
The Role of the Comparator Interface
At its core, the Comparator interface is a critical part of Java’s object-oriented programming model. It’s a functional interface that represents an ordering of objects of a specific type. The Comparator interface declares a single method, compare
, which compares its two arguments for order and returns a negative integer, zero, or a positive integer depending on whether the first argument is less than, equal to, or greater than the second.
Comparators vs. Comparable
The Comparator interface is often compared to the Comparable interface, another Java interface used to order objects. While they serve similar purposes, there’s a crucial difference: the Comparable interface is used to define a ‘natural’ ordering for a class, while the Comparator interface allows you to define multiple possible orderings for a class.
For instance, consider a Person
class with a name
and age
field. The ‘natural’ ordering might be alphabetical by name. However, you might also want to sort Person
objects by age, which is where a Comparator comes in handy.
Lambda Expressions and Comparators
With the introduction of lambda expressions in Java 8, creating Comparators has become even more straightforward. A lambda expression is a short block of code that takes in parameters and returns a value. Lambda expressions are often used to create anonymous methods.
For instance, here’s how you might create a Comparator to sort integers in ascending order using a lambda expression:
Comparator<Integer> comparator = (a, b) -> a - b;
List<Integer> list = Arrays.asList(3, 1, 4, 1, 5, 9);
Collections.sort(list, comparator);
# Output:
# [1, 1, 3, 4, 5, 9]
In this example, (a, b) -> a - b
is the lambda expression. It takes in two parameters, a
and b
, and returns the result of a - b
. This lambda expression effectively implements the compare
method of the Comparator interface, providing a concise way to define custom sorting rules.
By understanding these fundamental concepts, you can better appreciate the power and flexibility of Java Comparators and how they fit into the larger context of Java’s object-oriented programming model.
Java Comparators: Real-World Applications and Beyond
Java Comparators are not just theoretical constructs or tools for academic exercises. They have practical applications in real-world Java development scenarios, particularly when it comes to sorting data in web applications or databases.
Sorting Data in Web Applications
In web applications, data sorting is a common requirement. For instance, you may need to sort user profiles based on their creation date, or sort products by price in an e-commerce application. A Java Comparator can be used to define the sorting rules for these objects, providing a flexible and efficient way to sort collections of data.
Sorting Data in Databases
Java Comparators can also be used to sort data retrieved from databases. For instance, you might retrieve a list of records from a database, and then use a Comparator to sort these records based on a specific field before displaying them to the user. This can provide a more user-friendly display of data and can also improve the efficiency of your application by reducing the amount of data that needs to be transferred from the database.
Exploring Related Topics
If you’ve enjoyed learning about Java Comparators and want to explore related topics, consider looking into Java streams and generics. Streams provide a powerful way to process collections of data, and can be used in conjunction with Comparators to sort data in a more declarative and often more readable way. Generics, on the other hand, allow you to write more generic and reusable code, and can be used to create more flexible Comparators that can compare objects of any type.
Further Resources for Mastering Java Comparators
For those who wish to dive deeper into the world of Java Comparators, here are some valuable resources to explore:
- Java Interface Tutorial: Getting Started – Explore the use of interfaces for achieving abstraction.
Exploring Iterable in Java – Learn how to implement Iterable and create custom iterable collections.
Map Usage in Java – Master using maps for efficient data storage and retrieval in Java applications.
Java Comparator tutorial by Baeldung covers everything from basic usage to advanced features like Comparator chaining and combining.
Java Sorting Guide by GeeksforGeeks provides an in-depth look at sorting in Java, including the use of both Comparable and Comparator.
Java Collections tutorial by Oracle includes a section on object ordering with Comparators.
Wrapping Up: Comparator Interface Usage
In this comprehensive guide, we’ve explored the ins and outs of using Java Comparators to sort collections. From the basics of implementing the compare method to advanced uses such as chaining comparators for multi-field sorting and using comparators with streams, we’ve covered a wide range of topics to help you master Java Comparators.
We began with the basics, learning how to use the Comparator interface to sort collections in Java. We then delved into more advanced usage, exploring how to chain comparators for multi-field sorting and how to use comparators with streams.
Along the way, we tackled common issues you might encounter when using Java Comparators, such as handling null values and sorting in reverse order, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to sorting collections in Java, comparing the use of Java Comparators with the Comparable interface and third-party libraries. Here’s a quick comparison of these methods:
Method | Flexibility | Ease of Use | Additional Features |
---|---|---|---|
Java Comparator | High | Moderate | Chaining, Reverse sorting |
Comparable Interface | Low | High | Natural ordering |
Third-Party Libraries | High | High | Builder-style API, Additional features |
Whether you’re just starting out with Java Comparators or you’re looking to level up your sorting skills, we hope this guide has given you a deeper understanding of Java Comparators and their capabilities.
With its balance of flexibility, ease of use, and powerful features, the Java Comparator interface is a key tool for sorting collections in Java. Happy coding!