Map Java: Your Ultimate Guide to Map Interface in Java
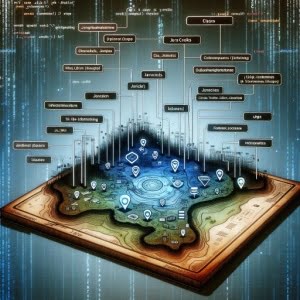
Ever found yourself struggling with Map in Java? You’re not alone. Many developers find it challenging to grasp the concept and usage of Map in Java. But think of it as a dictionary, where you can store and retrieve information based on unique keys. It’s a powerful tool that can significantly simplify your data management tasks.
This guide will walk you through the basics to advanced usage of Map in Java. We’ll cover everything from creating a Map, adding elements to it, retrieving them, to handling more complex scenarios like dealing with different types of Maps, null values, and more.
So, let’s dive in and start mastering Map in Java!
TL;DR: How Do I Use Map in Java?
In Java, you can create a Map and use it to store key-value pairs with the syntax
Map<String, Integer> map = new HashMap<>()
. To achieve this you will use theMap
interface and one of its implementing classes, such asHashMap
.
Here’s a simple example:
Map<String, Integer> map = new HashMap<>();
map.put('apple', 10);
System.out.println(map.get('apple'));
# Output:
# 10
In this example, we create a HashMap
where the keys are strings and the values are integers. We then add a key-value pair (‘apple’, 10) to the map using the put
method. Finally, we retrieve the value associated with the key ‘apple’ using the get
method, which outputs ’10’.
This is a basic way to use Map in Java, but there’s much more to learn about handling key-value pairs in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of Map in Java
- Exploring Different Types of Maps
- Iterating Over a Map
- Handling Null Values
- Exploring Alternative Approaches for Key-Value Pairs
- Troubleshooting Common Map Issues in Java
- Understanding the Map Interface in Java
- The Role of Hashing in HashMap
- Map Java: Beyond Basic Usage
- Further Resources for Mastering Map in Java
- Wrapping Up: Mastering Key-Value Pairs with Java Map
Basic Use of Map in Java
In Java, a Map is an object that stores associations between keys and values, or key/value pairs. Both keys and values are objects. The keys must be unique, but the values may be duplicated.
Creating a Map
Creating a Map in Java involves two steps: declaring a Map variable, and instantiating a new Map object.
Map<String, Integer> map;
map = new HashMap<>();
In this example, we declare a Map variable map
that will hold String keys and Integer values. We then instantiate a new HashMap
object and assign it to map
.
Adding Elements to a Map
After creating a Map, you can add elements to it using the put
method. The put
method takes a key and a value, and adds the key-value pair to the Map.
map.put('apple', 10);
map.put('banana', 20);
In this code, we add two key-value pairs to the Map: ‘apple’ with a value of 10, and ‘banana’ with a value of 20.
Retrieving Elements from a Map
You can retrieve values from a Map using the get
method. The get
method takes a key and returns the corresponding value.
System.out.println(map.get('apple'));
System.out.println(map.get('banana'));
# Output:
# 10
# 20
In this code, we retrieve the values associated with the keys ‘apple’ and ‘banana’, and print them to the console.
Advantages and Potential Pitfalls
The Map interface provides a powerful way to manage key-value pairs. It allows for fast retrieval of values based on keys, which can greatly simplify your code. However, it’s important to remember that keys in a Map must be unique. If you try to add a key-value pair with a key that’s already in the Map, the old value will be replaced with the new one. This can lead to unexpected results if you’re not careful.
map.put('apple', 30);
System.out.println(map.get('apple'));
# Output:
# 30
In this code, we add a key-value pair with the key ‘apple’ and a value of 30. Since ‘apple’ is already in the Map, the old value (10) is replaced with the new value (30).
Exploring Different Types of Maps
Java provides several implementations of the Map interface, each with its own characteristics and benefits. Let’s take a closer look at three commonly used Map types: HashMap
, TreeMap
, and LinkedHashMap
.
HashMap
HashMap
is the most commonly used implementation of the Map interface. It stores key-value pairs in an array-based data structure, which provides constant-time performance for basic operations like get
and put
.
Map<String, Integer> hashMap = new HashMap<>();
hashMap.put('apple', 10);
hashMap.put('banana', 20);
System.out.println(hashMap.get('apple'));
System.out.println(hashMap.get('banana'));
# Output:
# 10
# 20
TreeMap
TreeMap
is a sorted map implementation that maintains its elements in ascending order. This can be particularly useful when you need to retrieve keys or values in a sorted manner.
Map<String, Integer> treeMap = new TreeMap<>();
treeMap.put('apple', 10);
treeMap.put('banana', 20);
System.out.println(treeMap.get('apple'));
System.out.println(treeMap.get('banana'));
# Output:
# 10
# 20
LinkedHashMap
LinkedHashMap
is a hash table and linked list implementation of the Map interface. It maintains a doubly-linked list running through all of its entries, which allows it to maintain the order in which keys were inserted into the Map.
Map<String, Integer> linkedHashMap = new LinkedHashMap<>();
linkedHashMap.put('apple', 10);
linkedHashMap.put('banana', 20);
System.out.println(linkedHashMap.get('apple'));
System.out.println(linkedHashMap.get('banana'));
# Output:
# 10
# 20
Iterating Over a Map
There are several ways to iterate over a Map in Java. One common approach is to use the entrySet
method, which returns a Set
view of the Map’s entries.
for (Map.Entry<String, Integer> entry : hashMap.entrySet()) {
System.out.println(entry.getKey() + ': ' + entry.getValue());
}
# Output:
# apple: 10
# banana: 20
Handling Null Values
In Java, Map implementations like HashMap
and LinkedHashMap
allow null keys and null values, while TreeMap
does not. When working with Maps, it’s important to be aware of this, as trying to use a null key or value where it’s not allowed will result in a NullPointerException
.
hashMap.put(null, 30);
System.out.println(hashMap.get(null));
# Output:
# 30
In this example, we add a key-value pair with a null key to a HashMap
, which is allowed. When we retrieve the value associated with the null key, it correctly returns ’30’.
Exploring Alternative Approaches for Key-Value Pairs
While the Map interface and its standard implementations provide a powerful way to handle key-value pairs in Java, there are alternative methods that can offer additional features or better performance in certain situations.
Using the Properties Class
The Properties
class is a special type of Map that’s designed to store configuration data as key-value pairs. It provides methods for reading and writing data from and to a stream, which makes it suitable for managing configuration files.
Properties properties = new Properties();
properties.setProperty('apple', '10');
properties.setProperty('banana', '20');
System.out.println(properties.getProperty('apple'));
System.out.println(properties.getProperty('banana'));
# Output:
# 10
# 20
In this example, we create a Properties
object and add two key-value pairs to it. We then retrieve the values associated with the keys ‘apple’ and ‘banana’. Note that both keys and values are stored as strings.
Multimap from Google Guava
Google Guava’s Multimap
is an advanced tool that allows you to map keys to multiple values. This can be particularly useful when you need to associate a key with more than one value.
Multimap<String, Integer> multimap = ArrayListMultimap.create();
multimap.put('apple', 10);
multimap.put('apple', 20);
System.out.println(multimap.get('apple'));
# Output:
# [10, 20]
In this example, we create an ArrayListMultimap
and add two key-value pairs with the same key ‘apple’. When we retrieve the values associated with ‘apple’, it returns a list containing both values.
While these alternative methods can offer additional features, they also have their own limitations. For example, the Properties
class only supports string keys and values, and Multimap
requires an external library. Therefore, it’s important to choose the right tool based on your specific needs.
Troubleshooting Common Map Issues in Java
While using Map in Java, you might encounter several common issues. Let’s discuss these problems and provide solutions and workarounds.
Handling Duplicate Keys
In a Map, keys are unique. If you try to insert a duplicate key with a different value, the old value will be replaced.
Map<String, Integer> map = new HashMap<>();
map.put('apple', 10);
map.put('apple', 20);
System.out.println(map.get('apple'));
# Output:
# 20
In this example, the value associated with the key ‘apple’ is replaced from 10 to 20. To prevent this, you can check if a key is already present using the containsKey
method.
Dealing with Null Values
Some Map implementations allow null keys and values, while others do not. For example, HashMap allows one null key and any number of null values, while TreeMap doesn’t allow any null keys or values.
Map<String, Integer> map = new TreeMap<>();
map.put(null, 10);
# Output:
# NullPointerException
This example throws a NullPointerException
because TreeMap doesn’t allow null keys. Always check the documentation of the Map implementation you’re using to understand how it handles null keys and values.
Concurrent Modification Exceptions
If a Map is modified while iterating over it, a ConcurrentModificationException
is thrown.
Map<String, Integer> map = new HashMap<>();
map.put('apple', 10);
map.put('banana', 20);
for (String key : map.keySet()) {
map.remove(key);
}
# Output:
# ConcurrentModificationException
In this example, we try to remove an entry from the Map while iterating over it, which results in a ConcurrentModificationException
. To avoid this, you can use an Iterator
to safely remove entries during iteration.
Remember, understanding these common issues and their solutions can help you avoid bugs and write more robust code when working with Map in Java.
Understanding the Map Interface in Java
The Map interface is a part of the Java Collections Framework, which provides a unified architecture for representing and manipulating collections. The Map interface defines an object that maps keys to values. It’s not a subtype of the Collection interface, but it’s fully integrated into the framework.
A Map cannot contain duplicate keys; each key can map to at most one value. It models the mathematical function abstraction. The Map interface includes methods for basic operations (such as put
, get
, and remove
), bulk operations (such as putAll
and clear
), and collection views (such as keySet
, entrySet
, and values
).
Java provides several Map implementations: HashMap
, TreeMap
, LinkedHashMap
, and Hashtable
. Each of these classes has its own set of characteristics and benefits.
The Role of Hashing in HashMap
Understanding the concept of hashing is fundamental to understanding how a HashMap
works. Hashing is the process of converting an input of any size into a fixed-size string of text, using a mathematical algorithm.
In Java, when you put an object into a HashMap
, the hashCode
method of the key object is called, and the returned hash code is used to find a bucket location where the value is stored. When you try to retrieve an object from the HashMap
, the hashCode
method is called again and uses the returned hash code to find the bucket where the value is stored.
Here’s a simple example:
Map<String, Integer> map = new HashMap<>();
map.put('apple', 10);
System.out.println(map.get('apple'));
# Output:
# 10
In this example, when we put the key-value pair into the HashMap
, the hashCode
method of the string ‘apple’ is called to find a bucket location to store the value 10. When we retrieve the value with the key ‘apple’, the hashCode
method is called again to find the bucket where the value 10 is stored.
Map Java: Beyond Basic Usage
The Map interface in Java is not just a tool for storing key-value pairs. It’s a powerful data structure that can solve a wide range of problems in fields such as data analysis, web development, and more.
Map in Data Structure Problems
In data structure problems, Map is often used to count occurrences, track unique elements, or build frequency tables. Its ability to store and retrieve values based on unique keys makes it an excellent tool for these tasks.
Map in Web Applications
In web applications, Map can be used to store session data, cache results, or manage user preferences. The flexibility and efficiency of Map make it ideal for these use cases.
Exploring Related Concepts
If you’re interested in Map, you might also want to explore related concepts in the Java Collections Framework, such as Set and List. The Set interface is a collection that contains no duplicate elements, while the List interface is an ordered collection that can contain duplicate elements. Both interfaces provide a different set of capabilities and can be used in conjunction with Map to solve more complex problems.
Further Resources for Mastering Map in Java
Map is one of the fundamental interfaces of Java programming. To find out more relevant info on Java Interfaces, Click Here!
And to deepen your understanding of Map and related concepts in Java, consider exploring these resources:
- Java Comparator Interface: Guide – Explore Java Comparator for custom object sorting and comparison.
Iterator Usage in Java – Master Iterator usage for sequential access to collection elements in Java.
Java Collections Tutorial from Oracle provides an in-depth look at the Java Collections Framework, including Map, Set, and List.
Java Map Documentation provides a detailed explanation of the methods provided by Map and its standard implementations.
Java Programming Masterclass for Software Developers covers a wide range of Java topics, including the Map interface and the Java Collections Framework.
Wrapping Up: Mastering Key-Value Pairs with Java Map
In this comprehensive guide, we’ve journeyed through the world of Map in Java, a fundamental part of the Java Collections Framework that provides a powerful way to handle key-value pairs.
We began with the basics, learning how to create a Map, add elements to it, and retrieve them. We then ventured into more advanced territory, exploring different types of Maps, such as HashMap
, TreeMap
, and LinkedHashMap
, and how to handle more complex scenarios like dealing with null values and iterating over a Map.
Along the way, we tackled common issues you might encounter when using Map in Java, such as handling duplicate keys and concurrent modification exceptions, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handle key-value pairs in Java, such as using the Properties
class or Multimap
from Google Guava. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Map Interface | Handles unique key-value pairs, Fast retrieval based on keys | Keys must be unique |
Properties Class | Designed for configuration data, Supports reading and writing from/to a stream | Only supports string keys and values |
Google Guava’s Multimap | Allows keys to map to multiple values | Requires an external library |
Whether you’re just starting out with Map in Java or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of Map and its capabilities.
With its balance of flexibility, efficiency, and ease of use, Map is a powerful tool for managing key-value pairs in Java. Now, you’re well equipped to enjoy those benefits. Happy coding!