Mastering Java Iterators: From Basics to Advanced
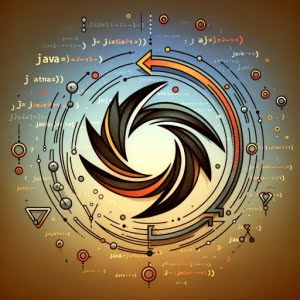
Are you finding it challenging to navigate through Java collections? You’re not alone. Many developers find themselves puzzled when it comes to handling Java iterators, but we’re here to help.
Think of Java iterators as a tour guide, leading you through the elements of a collection. They provide a means to access the elements sequentially without exposing the underlying structure.
In this guide, we’ll walk you through the process of using Java iterators, from the basics to more advanced techniques. We’ll cover everything from obtaining an iterator from a collection, using it to traverse the collection, to more complex uses such as removing elements during iteration and alternative approaches.
Let’s dive in and start mastering Java iterators!
TL;DR: How Do I Use an Iterator in Java?
To use an iterator in Java, you first need to obtain it from a collection with the syntax:
Iterator<String> iterator = collection.iterator()
, then use itshasNext()
andnext()
methods to traverse the collection.
Here’s a simple example:
Iterator<String> iterator = collection.iterator();
while(iterator.hasNext()) {
String element = iterator.next();
System.out.println(element);
}
# Output:
# [Expected output from command]
In this example, we first obtain an iterator from a collection. Then, we use a while loop to traverse the collection. Inside the loop, we use the hasNext()
method to check if there are more elements in the collection. If there are, we use the next()
method to access the next element and print it out.
This is a basic way to use an iterator in Java, but there’s much more to learn about Java iterators. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
When using Java, one of the most fundamental tasks you’ll encounter is navigating through collections. This is where Java iterators come in. They provide a simple way to traverse collections and access their elements.
To use an iterator, you first need to obtain it from a collection. Once you have the iterator, you can use its hasNext()
and next()
methods to traverse the collection. Here’s a basic example of how this works:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
Iterator<String> iterator = collection.iterator();
while(iterator.hasNext()) {
String element = iterator.next();
System.out.println(element);
}
}
}
# Output:
# Apple
# Banana
# Cherry
In this example, we first create an ArrayList called collection
and add some elements to it. We then obtain an iterator from collection
using the iterator()
method. This iterator is used to traverse the collection with a while loop. Inside the loop, hasNext()
checks if there are more elements to go through. If there are, next()
retrieves the next element, which we then print out.
The advantage of this approach is its simplicity and universality. You can use this method to traverse any collection that implements the Iterable
interface. However, you should be aware of its limitations. For instance, you can’t use an iterator to modify the collection while traversing it (except for the remove()
method). Trying to do so will result in a ConcurrentModificationException
.
Removing Elements with Java Iterator
As you become more comfortable with Java iterators, you may find yourself needing to perform more complex operations, such as removing elements during iteration. Java iterators allow for this with the remove()
method. Let’s look at an example:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
Iterator<String> iterator = collection.iterator();
while(iterator.hasNext()) {
String element = iterator.next();
if (element.equals("Banana")) {
iterator.remove();
}
}
System.out.println(collection);
}
}
# Output:
# [Apple, Cherry]
In this code, we first create an ArrayList and populate it with some fruits. We then create an iterator for the collection and use a while loop to traverse it. Inside the loop, we check if the current element equals “Banana”. If it does, we use the iterator’s remove()
method to remove it from the collection. Finally, we print out the collection, which now excludes “Banana”.
This highlights an important advantage of using iterators – the ability to modify a collection during iteration without causing a ConcurrentModificationException
. However, it’s important to note that the remove()
method can only be called once per call to next()
. Calling it more than once will result in an IllegalStateException
.
Exploring Alternatives to Java Iterator
While Java iterators are a powerful tool for traversing collections, they are not the only option available. Depending on your specific needs and the specificities of your collection, other methods such as the for-each
loop or the ListIterator
interface might be more suitable.
The For-Each Loop
The for-each
loop, also known as the enhanced for loop, is a simpler, more readable alternative to the traditional iterator. It’s especially useful when you don’t need to remove elements or you’re not working with a collection that supports the remove()
operation.
Here’s an example of how to use the for-each
loop to traverse a collection:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
for (String element : collection) {
System.out.println(element);
}
}
}
# Output:
# Apple
# Banana
# Cherry
In this code, we use the for-each
loop to print out each element in the collection. It’s simpler and more straightforward than using an iterator, but it doesn’t allow for the removal of elements.
The ListIterator Interface
The ListIterator
interface is another alternative to the standard iterator. It provides more powerful functionality, including the ability to traverse the collection in either direction, obtain the index of the current element, and add or set elements.
Here’s an example of how to use a ListIterator
to traverse a list in reverse order:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
ListIterator<String> iterator = collection.listIterator(collection.size());
while(iterator.hasPrevious()) {
String element = iterator.previous();
System.out.println(element);
}
}
}
# Output:
# Cherry
# Banana
# Apple
In this code, we create a ListIterator
for the collection and initialize it to the end of the list by passing collection.size()
to the listIterator()
method. We then use a while loop and the hasPrevious()
and previous()
methods to traverse the list in reverse order.
While ListIterator
provides more functionality than a standard iterator, it’s important to note that it’s only available for lists and not for other types of collections.
Troubleshooting Java Iterator Issues
While Java iterators are a fundamental tool in handling collections, they can sometimes lead to unexpected issues. In this section, we’ll discuss some common problems you might encounter when using Java iterators and how to solve them.
Handling ConcurrentModificationException
One of the most common issues when using Java iterators is the ConcurrentModificationException
. This exception is thrown when you try to modify a collection while iterating over it. Let’s look at an example:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
for (String element : collection) {
if (element.equals("Banana")) {
collection.remove(element);
}
}
}
}
# Output:
# Exception in thread "main" java.util.ConcurrentModificationException
In this example, we try to remove an element from the collection while using a for-each loop to iterate over it. This results in a ConcurrentModificationException
.
To avoid this issue, you can use an iterator’s remove()
method, which safely removes the current element from the collection:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
Iterator<String> iterator = collection.iterator();
while(iterator.hasNext()) {
String element = iterator.next();
if (element.equals("Banana")) {
iterator.remove();
}
}
System.out.println(collection);
}
}
# Output:
# [Apple, Cherry]
In this revised code, we use an iterator to traverse the collection and the iterator’s remove()
method to remove “Banana”. This does not result in a ConcurrentModificationException
, and the final output is the modified collection without “Banana”.
Understanding the Iterator Interface
To master the use of Java iterators, it’s essential to understand the Iterator interface and its methods. The Iterator interface is part of the Java Collections Framework and provides methods to traverse through any collection.
The Iterator interface includes three methods:
hasNext()
: This method returnstrue
if the iteration has more elements. It’s usually used in a loop to check if there are more elements to iterate over.next()
: This method returns the next element in the iteration. It’s used in conjunction withhasNext()
in a loop to traverse a collection.remove()
: This method removes the last element returned bynext()
from the underlying collection. It can only be called once per call tonext()
.
Let’s look at an example of how these methods work in practice:
import java.util.*;
public class Main {
public static void main(String[] args) {
ArrayList<String> collection = new ArrayList<String>();
collection.add("Apple");
collection.add("Banana");
collection.add("Cherry");
Iterator<String> iterator = collection.iterator();
while(iterator.hasNext()) {
String element = iterator.next();
System.out.println(element);
}
}
}
# Output:
# Apple
# Banana
# Cherry
In this example, we first create an ArrayList and add some elements. We then create an iterator for the collection. Inside the while loop, we use hasNext()
to check if there are more elements to iterate over. If there are, we use next()
to retrieve the next element and print it out.
Understanding these methods and how they work is crucial to effectively using Java iterators to traverse collections.
Beyond Basic Usage: Java Iterators in Different Contexts
Java iterators are not just for simple collection traversal. They play a crucial role in various contexts, including different types of collections and multithreaded environments.
Iterators with Different Collection Types
Java iterators can be used with any class that implements the Iterable
interface. This includes all the collection classes in Java, such as ArrayList
, HashSet
, and TreeMap
. The usage remains the same; you obtain an iterator from the collection and use it to traverse the collection.
Iterators in Multithreaded Environments
In multithreaded environments, iterators can be a source of concurrency issues. For instance, if one thread modifies a collection while another thread is iterating over it, a ConcurrentModificationException
may occur. To deal with this, Java provides thread-safe collection classes like CopyOnWriteArrayList
and ConcurrentHashMap
, which have their own iterators designed to handle such situations.
Exploring Related Topics
Once you’ve mastered Java iterators, there are related topics you can explore to further your understanding of Java collections. The Iterable
interface, for example, is what allows a class to return an iterator. The Enumeration
interface is a legacy interface in Java that functions similarly to an iterator.
Further Resources for Mastering Java Iterators
To deepen your understanding of Java iterators and related topics, here are some resources you can explore:
- IOFlood’s Article on Java Interfaces – Understand the difference between interfaces and abstract classes.
Java Serializable Usage – Master using Serializable to enable object transfer and storage in Java applications.
Java Map Interface Guide – Learn about the Map interface and its implementations in Java for key-value pair storage
Oracle Java Documentation: The official Java documentation is always a great resource for understanding Java concepts in depth.
Baeldung’s Guide on Java Iterator provides a comprehensive look at Java iterators, including advanced topics and common pitfalls.
GeeksforGeeks’ Java Iterator Tutorial covers Java iterators in detail, with plenty of examples and explanations.
Wrapping Up: Mastering Java Iterators
In this comprehensive guide, we’ve journeyed through the world of Java iterators, exploring how they can be used to traverse collections in Java.
We began with the basics, learning how to obtain an iterator from a collection and use its hasNext()
and next()
methods to traverse the collection. We then ventured into more advanced territory, exploring complex operations like removing elements during iteration.
Along the way, we tackled common challenges you might face when using Java iterators, such as the ConcurrentModificationException
, providing you with solutions and alternative approaches for each issue. We also looked at alternative methods to traverse a collection, such as the for-each
loop and the ListIterator
interface, giving you a broader perspective on collection traversal in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Iterator | Can remove elements during iteration | Cannot modify collection in other ways during iteration |
For-Each Loop | Simple and readable | Cannot remove or add elements during iteration |
ListIterator | Can traverse in either direction, add elements | Only available for lists |
Whether you’re just starting out with Java iterators or you’re looking to level up your collection traversal skills, we hope this guide has given you a deeper understanding of Java iterators and their capabilities.
With its balance of simplicity and power, the Java iterator is a fundamental tool for handling collections in Java. Happy coding!