Python Loop Mastery: A Comprehensive Guide
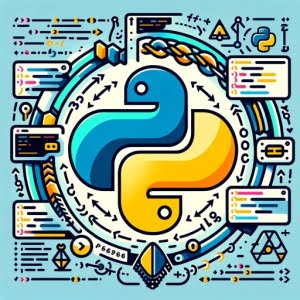
Are you finding it challenging to work with loops in Python? You’re not alone. Many developers, especially beginners, often find loops a bit tricky to grasp. But, think of Python’s loops as a well-choreographed dance routine – once you get the steps right, it’s a smooth and efficient performance.
Whether you’re trying to automate repetitive tasks or traverse through data structures, understanding how to use loops in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using loops in Python, from the basics to more advanced techniques. We’ll cover everything from the for
and while
loops, to even some alternative approaches.
So, whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you. Let’s get started!
TL;DR: How Do I Create a Loop in Python?
In Python, you can create a loop using the ‘for’ or ‘while’ keywords. These loops allow you to execute a block of code multiple times, which can be incredibly useful in many programming scenarios.
Here’s a simple example of a ‘for’ loop:
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, we use the for
keyword to create a loop that iterates over a sequence of numbers generated by the range()
function. The print(i)
statement is executed five times, printing the numbers 0 through 4.
This is a basic way to create a loop in Python, but there’s much more to learn about using loops effectively. Continue reading for more detailed explanations and examples.
Table of Contents
Python Loops for Beginners: ‘For’ and ‘While’ Loops
In Python, the two primary types of loops you’ll encounter are ‘for’ and ‘while’ loops. Let’s delve into how these two types of loops work in Python and how to use them effectively.
‘For’ Loops in Python
A ‘for’ loop in Python iterates over a sequence (like a list, tuple, string, or range) or other iterable objects. Here’s an example of a ‘for’ loop that iterates over a list:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, fruit
is the loop variable that takes the value of the next element in fruits
each time through the loop. The print(fruit)
statement is executed for each item in the list, printing each fruit’s name.
‘While’ Loops in Python
A ‘while’ loop in Python repeatedly executes a target statement as long as a given condition is true. Here’s an example:
count = 0
while count < 5:
print(count)
count += 1
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the print(count)
statement is executed as long as the count
is less than 5. With each iteration, the count
is incremented by 1. When count
reaches 5, the condition becomes false, and the loop terminates.
Understanding these basic loop structures in Python is crucial to writing efficient and effective code. As you continue to learn and grow as a Python programmer, you’ll find these loops invaluable for handling repetitive tasks and iterating over data structures.
Advanced Python Loops: Delving Deeper
As you become more comfortable with basic Python loops, you’ll find that there are more complex, yet powerful, ways to use loops. Let’s explore some of these advanced techniques, including nested loops, loop control statements like ‘break’ and ‘continue’, and list comprehensions.
Nested Loops in Python
A nested loop is a loop inside a loop. It’s a powerful tool that allows you to handle complex tasks efficiently. Here’s an example of a nested ‘for’ loop:
for i in range(3):
for j in range(3):
print(i, j)
# Output:
# 0 0
# 0 1
# 0 2
# 1 0
# 1 1
# 1 2
# 2 0
# 2 1
# 2 2
In this example, for each iteration of the outer loop, the inner loop is executed three times, printing a grid of coordinates.
Loop Control Statements: ‘Break’ and ‘Continue’
‘Break’ and ‘continue’ are two loop control statements that can change your loop’s flow. The ‘break’ statement allows you to exit the loop prematurely when a certain condition is met, while ‘continue’ skips the rest of the current loop iteration and moves on to the next one. Here’s an example:
for num in range(10):
if num == 5:
break
print(num)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the loop breaks as soon as num
equals 5, thus only numbers from 0 to 4 are printed.
List Comprehensions in Python
List comprehensions provide a concise way to create lists based on existing lists. It’s a more Pythonic way to use ‘for’ loops. Here’s an example:
squares = [x**2 for x in range(5)]
print(squares)
# Output:
# [0, 1, 4, 9, 16]
In this example, a new list of squares is generated using a list comprehension. The expression x**2
is calculated for every value of x
in the range from 0 to 4.
These advanced techniques can help you write more efficient and readable Python code. As you continue to develop your Python skills, understanding these concepts will prove invaluable.
Alternative Looping Techniques in Python
As you progress in your Python journey, you’ll discover that ‘for’ and ‘while’ loops aren’t the only ways to loop in Python. There are alternative methods you can use, such as the ‘map’ and ‘filter’ functions, or generator expressions. Let’s explore these methods and compare them with traditional loops.
Python’s ‘Map’ and ‘Filter’ Functions
Python’s built-in ‘map’ and ‘filter’ functions offer a different approach to looping. They apply a function to each item in an iterable and return a new iterable with the results.
Here’s an example of using the ‘map’ function to square all numbers in a list:
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the ‘map’ function applies the lambda function x**2
to each item in numbers
, returning a new list of squares.
The ‘filter’ function, on the other hand, filters the items in an iterable based on a function’s result. Here’s an example:
numbers = [1, 2, 3, 4, 5]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(evens)
# Output:
# [2, 4]
In this example, the ‘filter’ function applies the lambda function x % 2 == 0
to each item in numbers
, returning a new list of even numbers.
Generator Expressions in Python
Generator expressions are a high-performance, memory–efficient generalization of list comprehensions and generators. Here’s an example:
numbers = (x for x in range(5))
for number in numbers:
print(number)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, a generator expression (x for x in range(5))
is used to generate numbers from 0 to 4. This is a more memory-efficient way to generate numbers as they are needed, rather than all at once like in a list.
While ‘for’ and ‘while’ loops are fundamental to Python, understanding these alternative techniques can help you write more efficient and readable code. These methods are particularly useful when working with large data sets, where memory efficiency is crucial.
Troubleshooting Python Loops: Common Pitfalls and Solutions
As you work with loops in Python, you may encounter some common issues. Let’s discuss these problems, such as infinite loops, off-by-one errors, and unexpected behavior with mutable objects, and how to solve them.
Infinite Loops
An infinite loop occurs when the loop’s condition never becomes false. This results in the loop running indefinitely, which can cause your program to become unresponsive. Here’s an example:
# WARNING: This is an example of what NOT to do.
# This is an infinite loop. Do not run this code.
# while True:
# print('This is an infinite loop')
In this example, because the condition True
never changes, the loop will run forever. To avoid this, always ensure that your loop’s condition will eventually become false.
Off-By-One Errors
Off-by-one errors happen when your loop iterates one time too many or one time too few. This is a common issue when using ‘for’ loops with the range()
function.
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, range(5)
generates numbers from 0 to 4, not 1 to 5. To avoid off-by-one errors, remember that range(n)
generates numbers up to n-1
.
Unexpected Behavior with Mutable Objects
Mutable objects like lists can behave unexpectedly when modified inside a loop. Here’s an example:
numbers = [1, 2, 3, 4, 5]
for i in range(len(numbers)):
del numbers[i]
# Output:
# IndexError: list assignment index out of range
In this example, deleting items from numbers
while iterating over it causes an IndexError
. This is because the size of the list decreases with each deletion, but the loop continues to the original length of the list. To avoid this, you can iterate over a copy of the list or use a list comprehension.
Understanding these common issues with Python loops can help you write more robust code. Always remember to test your loops thoroughly and consider edge cases to ensure they work as expected.
Understanding Iteration in Programming
Before we delve deeper into Python loops, it’s crucial to understand the concept of iteration in programming. Iteration, in the context of programming, is the repetition of a block of code until a certain condition is met. It’s the fundamental concept behind loops.
In Python, the ‘for’ and ‘while’ loops are the most common ways to perform iteration. However, the power of Python’s loops comes from the iterable and iterator protocols.
Python’s Iterable and Iterator Protocols
In Python, an iterable is an object capable of returning its elements one at a time. Lists, tuples, strings, and dictionaries are all examples of iterable objects in Python.
An iterator, on the other hand, is an object that implements the iterator protocol, which consists of the methods __iter__()
and __next__()
.
Here’s an example of how these protocols work in a ‘for’ loop:
numbers = [1, 2, 3, 4, 5]
iterator = iter(numbers)
while True:
try:
number = next(iterator)
print(number)
except StopIteration:
break
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we first create an iterator from the numbers
list using the iter()
function. We then use a ‘while’ loop and the next()
function to iterate through the numbers
. When the numbers
are exhausted, the next()
function raises a StopIteration
exception, which breaks the loop.
Understanding these protocols is key to understanding how loops work in Python. They allow Python’s loops to be flexible and powerful, capable of iterating over virtually any sequence or collection of items.
Python Loops in Real-World Projects
Python loops are not just theoretical concepts; they’re practical tools used in real-world projects. Let’s explore how loops are used in data analysis, web scraping, and automation.
Python Loops in Data Analysis
In data analysis, Python loops are often used to iterate over datasets, apply calculations, and generate results. For example, you might use a ‘for’ loop to calculate the average of a list of numbers:
numbers = [1, 2, 3, 4, 5]
sum = 0
for number in numbers:
sum += number
average = sum / len(numbers)
print(average)
# Output:
# 3.0
In this example, the ‘for’ loop iterates over the numbers
list, adding each number to the sum
. The average is then calculated by dividing the sum
by the number of items in numbers
.
Python Loops in Web Scraping
In web scraping, Python loops can be used to iterate over web pages and extract information. For example, you might use a ‘for’ loop to extract all links from a web page using the BeautifulSoup library.
Python Loops in Automation
Python loops are also useful in automation tasks. For example, you might use a ‘for’ loop to automate repetitive tasks such as renaming multiple files in a directory.
Further Learning: Recursion, Functional Programming, and Concurrency
As you continue to develop your Python skills, you might want to explore related topics such as recursion, functional programming, and concurrency. These concepts can provide you with even more tools to write efficient and effective Python code.
Further Resources for Python Loop Mastery
To continue your journey with mastering Python loops, here are a few resources that you might find enlightening:
- Python “do-while” Loop – Explore the do-while loop concept and its usage in Python programming.
Python “for” Loop – Explore the syntax and usage of “for” loops in Python for efficient iteration.
Python.org’s Tutorial on Control Flow Tools covers the basics of how to use loops and other control flow tools in Python.
Real Python’s Guide on Loops provides a more in-depth look at Python’s ‘for’ loop.
Python Course’s Article on Python Iterators explains the concept of iterators and understanding how loops work in Python.
Harness the power of these materials, deepening your understanding and proficiency with Python loops.
Wrapping Up: Mastering Python Loops
In this comprehensive guide, we’ve navigated the world of Python loops, from the basics to more advanced techniques, providing you with the tools to write more efficient and effective Python code.
We started with the basics, learning how to use ‘for’ and ‘while’ loops in Python. We then dived into more advanced territory, exploring nested loops, loop control statements, and list comprehensions. We also discussed alternative looping methods, such as Python’s ‘map’ and ‘filter’ functions and generator expressions, giving you a broader understanding of Python’s looping capabilities.
Along the way, we tackled common issues you might encounter when working with Python loops, such as infinite loops, off-by-one errors, and unexpected behavior with mutable objects. We provided solutions and workarounds for each issue, helping you to write more robust Python code.
Here’s a quick comparison of the looping techniques we’ve discussed:
Looping Technique | Use Case | Complexity |
---|---|---|
‘For’ and ‘While’ Loops | General Purpose | Low |
Nested Loops | Multi-Dimensional Data | Moderate |
Loop Control Statements | Specific Conditions | Moderate |
List Comprehensions | Creating Lists | Low |
‘Map’ and ‘Filter’ Functions | Applying Functions to Iterables | High |
Generator Expressions | Large Data Sets | High |
Whether you’re a beginner just starting out with Python loops or an experienced developer looking to level up your Python skills, we hope this guide has given you a deeper understanding of Python loops and their capabilities.
With the right knowledge and practice, you can master Python loops and write more efficient and effective code. Happy coding!