Bash Function Arguments | Shell Scripting Reference Guide
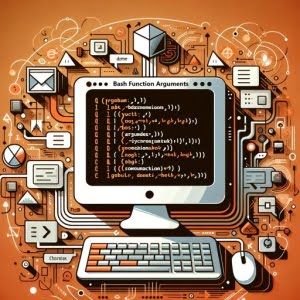
Are you finding it challenging to work with bash function arguments? You’re not alone. Many developers encounter difficulties when trying to understand and use arguments in bash functions.
Think of bash function arguments as the secret ingredients that can make your bash functions more flexible and powerful. They’re like the spices in a recipe, adding flavor and complexity to your scripts.
This guide will walk you through the basics of using arguments in bash functions, all the way to advanced techniques. We’ll cover everything from how to pass arguments to a bash function, how to access them inside the function, and how to use them in your scripts. We’ll also delve into more complex uses of arguments, such as passing arrays, using special variables, and handling optional arguments.
So, let’s dive in and start mastering bash function arguments!
TL;DR: How Do I Use Arguments in Bash Functions?
You can pass arguments to a bash function by including them after the function’s name when calling,
sample_function 'argument1' 'argument2'
.
Here’s a quick example:
function my_function {
echo $1
echo $2
}
my_function 'Hello' 'World'
# Output:
# 'Hello'
# 'World'
In this example, ‘Hello’ and ‘World’ are passed as arguments to the my_function
. Inside the function, $1
and $2
are used to access these arguments, resulting in them being echoed back.
This is a basic way to use arguments in bash functions, but there’s much more to learn about making your bash functions flexible and powerful with arguments. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Getting Started with Bash Function Arguments
- Exploring Advanced Bash Function Arguments
- Diving Deeper: Alternative Ways to Handle Bash Function Arguments
- Troubleshooting Bash Function Arguments
- Understanding Bash Functions and Arguments
- Applying Bash Function Arguments in Real-World Scenarios
- Wrapping Up: Harnessing the Power of Bash Function Arguments
Getting Started with Bash Function Arguments
When beginning to work with bash function arguments, it’s essential to understand how to pass arguments to a bash function and how to access them within the function itself. This knowledge forms the foundation of using arguments in your bash scripts.
Passing Arguments to a Bash Function
To pass arguments to a bash function, you include them after the function’s name when calling it, separated by spaces. Here’s a simple example:
function greet {
echo "Hello, $1"
}
greet 'Alice'
# Output:
# 'Hello, Alice'
In this example, ‘Alice’ is passed as an argument to the greet
function. The argument is accessed inside the function using $1
, which represents the first argument passed to the function.
Accessing Arguments Inside a Bash Function
Inside a bash function, arguments are accessed using $1
, $2
, $3
, etc., where the number represents the position of the argument. $1
is the first argument, $2
is the second, and so on. Let’s expand our previous example to greet two people:
function greet_two {
echo "Hello, $1 and $2"
}
greet_two 'Alice' 'Bob'
# Output:
# 'Hello, Alice and Bob'
In this example, ‘Alice’ and ‘Bob’ are passed as arguments to the greet_two
function. Inside the function, $1
is used to access ‘Alice’, and $2
is used to access ‘Bob’.
Using Arguments in Your Bash Scripts
Arguments can be used in your bash scripts to make them more flexible and reusable. For example, you could create a function to copy files from one directory to another, with the source and destination directories passed as arguments:
function copy_files {
cp $1/* $2/
}
copy_files '/path/to/source' '/path/to/destination'
# Output:
# (Assuming successful operation, no output is produced)
In this script, the copy_files
function takes two arguments: the source directory and the destination directory. The function uses these arguments in the cp
command to copy all files from the source directory to the destination directory.
These examples illustrate the basic use of arguments in bash functions. By understanding how to pass and access arguments, you can begin to create more flexible and powerful bash scripts.
Exploring Advanced Bash Function Arguments
After mastering the basics of bash function arguments, you can start exploring more complex uses. This includes passing arrays, using special variables like $@
and $*
, and handling optional arguments. Let’s dive into these topics.
Passing Arrays to Bash Functions
Bash doesn’t support passing arrays as arguments directly, but there’s a workaround using the declare
command. Here’s an example:
function print_array {
declare -a arr=($@)
for i in ${arr[@]}; do
echo $i
done
}
array=( 'Alice' 'Bob' 'Charlie' )
print_array ${array[@]}
# Output:
# 'Alice'
# 'Bob'
# 'Charlie'
In this example, an array of names is passed to the print_array
function. Inside the function, the $@
variable is used to capture all arguments and store them in a new array. The function then loops over this array and echoes each name.
Special Variables $@
and $*
In bash, $@
and $*
are special variables that hold all arguments passed to a function. While they may seem similar, their behavior differs when quoted. Let’s look at an example:
function print_args {
echo "Using \$@:"
for arg in "$@"; do
echo $arg
done
echo "Using \$*":
for arg in "$*"; do
echo $arg
done
}
print_args 'Alice' 'Bob' 'Charlie'
# Output:
# 'Using $@:'
# 'Alice'
# 'Bob'
# 'Charlie'
# 'Using $*:'
# 'Alice Bob Charlie'
In this example, $@
treats each argument as separate, while $*
treats all arguments as a single string. The difference between these two can be crucial depending on your use case.
Handling Optional Arguments
Optional arguments can make your bash functions more flexible. Here’s an example of a greeting function with an optional language argument:
function greet {
local name=$1
local lang=${2:-'en'}
if [[ $lang == 'es' ]]; then
echo "Hola, $name"
else
echo "Hello, $name"
fi
}
greet 'Alice' 'es'
greet 'Bob'
# Output:
# 'Hola, Alice'
# 'Hello, Bob'
In this function, the second argument is optional and defaults to ‘en’. If ‘es’ is passed as the second argument, the function greets in Spanish. Otherwise, it greets in English.
These examples illustrate more advanced uses of bash function arguments. By understanding these concepts, you can create more complex and flexible bash scripts.
Diving Deeper: Alternative Ways to Handle Bash Function Arguments
While the methods we’ve explored so far are quite powerful, there are other techniques for managing arguments in bash functions. These include using getopts
for option parsing and passing associative arrays. These methods provide more control and flexibility but come with their own complexities. Let’s explore these alternative approaches.
Option Parsing with getopts
getopts
is a built-in bash command used to parse command line options and arguments. It’s especially useful when you want to accept named arguments in your functions. Here’s an example:
function greet {
local name=''
local lang='en'
while getopts 'n:l:' opt; do
case $opt in
n) name=$OPTARG ;;
l) lang=$OPTARG ;;
esac
done
if [[ $lang == 'es' ]]; then
echo "Hola, $name"
else
echo "Hello, $name"
fi
}
greet -n 'Alice' -l 'es'
greet -n 'Bob'
# Output:
# 'Hola, Alice'
# 'Hello, Bob'
In this function, getopts
is used to parse named -n
and -l
options for the name and language arguments. This makes the function call more explicit and easier to understand.
Passing Associative Arrays
Bash 4.0 introduced associative arrays, which can be useful for passing complex data structures as arguments. However, like regular arrays, associative arrays can’t be passed directly. Here’s a workaround using declare
:
function print_person {
declare -A person=($@)
echo "Name: ${person[name]}, Age: ${person[age]}"
}
declare -A alice=( ['name']='Alice' ['age']=30 )
print_person ${alice[@]}
# Output:
# 'Name: Alice, Age: 30'
In this example, an associative array of a person’s name and age is passed to the print_person
function. Inside the function, the $@
variable is used to capture all arguments and store them in a new associative array.
These alternative approaches offer more advanced control over bash function arguments. However, they also introduce additional complexity and may not be necessary for simpler scripts. Always consider your specific use case and choose the most appropriate method.
Troubleshooting Bash Function Arguments
As with any programming task, working with bash function arguments can present its own set of challenges. Some common issues include dealing with spaces in arguments and handling an excessive number of arguments. Let’s look at these problems in more detail and explore possible solutions.
Dealing with Spaces in Arguments
Spaces can cause unexpected behavior when passing arguments to bash functions. For example, consider this function:
function greet {
echo "Hello, $1"
}
greet 'Alice Bob'
# Output:
# 'Hello, Alice Bob'
While this might seem correct, what if ‘Alice Bob’ is supposed to be a single argument representing a full name? The function treats it as such, but this might not always be the case in more complex scripts.
To ensure an argument with spaces is treated as a single argument, you should always quote your variables:
function greet {
echo "Hello, "$1""
}
greet 'Alice Bob'
# Output:
# 'Hello, Alice Bob'
In this revised function, $1
is quoted, ensuring it’s treated as a single argument even if it contains spaces.
Handling Too Many Arguments
If your function expects a certain number of arguments, passing too many can cause unexpected behavior. Here’s a function that expects two arguments:
function greet_two {
echo "Hello, $1 and $2"
}
greet_two 'Alice' 'Bob' 'Charlie'
# Output:
# 'Hello, Alice and Bob'
‘Charlie’ is ignored because the function only uses the first two arguments. To prevent this, you could add a check at the start of your function to ensure the correct number of arguments are passed:
function greet_two {
if [ $# -ne 2 ]; then
echo "Error: Exactly 2 arguments required"
return 1
fi
echo "Hello, $1 and $2"
}
greet_two 'Alice' 'Bob' 'Charlie'
# Output:
# 'Error: Exactly 2 arguments required'
In this revised function, the special variable $#
is used to check the number of arguments. If it’s not 2, the function prints an error message and returns 1 to indicate an error.
These examples illustrate some common issues when using bash function arguments and how to solve them. By understanding these problems and their solutions, you can write more robust and reliable bash scripts.
Understanding Bash Functions and Arguments
To fully grasp the concept of bash function arguments, it’s crucial to understand how bash functions work and how arguments are passed and processed. This also includes related topics like command line arguments, variable scope, and exit status.
How Bash Functions Work
A bash function is a block of reusable code designed to perform a particular task. You can think of it as a mini-script within your script. Here’s an example of a simple bash function:
function greet {
echo "Hello, $1"
}
greet 'Alice'
# Output:
# 'Hello, Alice'
In this example, the greet
function takes one argument and echoes a greeting message. The $1
inside the function is a special variable that holds the value of the first argument passed to the function.
Passing and Processing Arguments
When you call a bash function, you can pass arguments to it, which are then available inside the function. These arguments are processed in the order they’re passed, and you can access them using special variables $1
, $2
, $3
, and so on:
function greet_two {
echo "Hello, $1 and $2"
}
greet_two 'Alice' 'Bob'
# Output:
# 'Hello, Alice and Bob'
In this example, ‘Alice’ and ‘Bob’ are passed as arguments to the greet_two
function. Inside the function, $1
holds ‘Alice’, and $2
holds ‘Bob’.
Command Line Arguments and Variable Scope
Command line arguments work similarly to function arguments. They’re passed to the script when it’s called and can be accessed using the same special variables. However, it’s important to note that these variables are scoped to the function. This means that $1
inside a function is separate from $1
outside a function:
function print_arg {
echo "Inside function: $1"
}
print_arg 'Function Argument'
echo "Outside function: $1"
# Output:
# 'Inside function: Function Argument'
# 'Outside function: '
In this example, ‘Function Argument’ is passed to the print_arg
function and printed inside the function. However, outside the function, $1
is empty because no command line arguments were passed to the script.
Understanding Exit Status
Every bash command returns an exit status, which is a numeric value indicating whether the command succeeded or failed. This is especially important when calling functions because you often want to check whether the function executed successfully:
function fail {
return 1
}
fail
echo $?
# Output:
# '1'
In this example, the fail
function returns 1, indicating an error. After calling the function, $?
is used to print the exit status, which is 1.
Understanding these fundamental concepts is key to mastering bash function arguments. With this knowledge, you can write more effective and reliable bash scripts.
Applying Bash Function Arguments in Real-World Scenarios
Understanding bash function arguments is one thing, applying them in real-world scenarios is another. In larger scripts or projects, the use of function arguments becomes crucial. They can help in making your scripts more modular, maintainable, and reusable.
Bash Scripting Best Practices
When writing bash scripts, it’s important to follow best practices. This includes using clear variable names, commenting your code, and handling errors appropriately. For example, when using function arguments, always check if the correct number of arguments has been passed to your function:
function greet_two {
if [ $# -ne 2 ]; then
echo "Error: Exactly 2 arguments required"
return 1
fi
echo "Hello, $1 and $2"
}
greet_two 'Alice'
# Output:
# 'Error: Exactly 2 arguments required'
In this example, the function checks if exactly two arguments were passed. If not, it prints an error message and returns 1, indicating an error.
Error Handling and Debugging
Error handling and debugging are important aspects of bash scripting. Functions can return an exit status to indicate success or failure, which can be used for error handling:
function fail {
return 1
}
if ! fail; then
echo "Function failed"
fi
# Output:
# 'Function failed'
In this example, the function always fails. The script checks the function’s exit status and prints a message if the function failed.
Further Resources for Mastering Bash Function Arguments
To deepen your understanding of bash function arguments and related concepts, here are some additional resources you can explore:
- GNU Bash Manual: This is the official manual for bash. It covers everything from basic to advanced topics, including function arguments.
- Advanced Bash-Scripting Guide: This guide goes into detail about advanced topics in bash scripting, including functions and arguments.
- Bash Pass Function Arg: This tutorial from Baeldung explores how to pass arguments to a function in Bash.
By understanding and applying bash function arguments in your scripts, you can write more efficient and powerful bash scripts. So, keep exploring and happy scripting!
Wrapping Up: Harnessing the Power of Bash Function Arguments
In this comprehensive guide, we’ve delved into the intricacies of bash function arguments. We’ve explored how to use them to make your bash functions more flexible and powerful, transforming your bash scripting skills.
We started with the basics, learning how to pass arguments to a bash function and access them inside the function. We then progressed to more advanced techniques, such as passing arrays, using special variables like $@
and $*
, and handling optional arguments. We also touched on alternative approaches for managing arguments in bash functions, such as using getopts
for option parsing and passing associative arrays.
Along our journey, we tackled common challenges that you might encounter when working with bash function arguments, such as dealing with spaces in arguments and handling too many arguments. We provided solutions and workarounds for these issues, helping you write more robust and reliable bash scripts.
We also compared the different methods of handling bash function arguments, highlighting their strengths and weaknesses:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Basic Use | Low | Low | Simple scripts |
Advanced Use | High | Medium | Complex scripts with multiple arguments |
Alternative Approaches | Very High | High | Complex scripts with specific needs |
Whether you’re new to bash scripting or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of bash function arguments and how to use them effectively.
Mastering bash function arguments is a powerful tool in your bash scripting arsenal. It can make your scripts more flexible, reusable, and maintainable. Now, you’re well equipped to tackle any bash scripting challenge that comes your way. Happy scripting!