The Pipe ‘|’ Operator in Bash | Command Chaining Guide
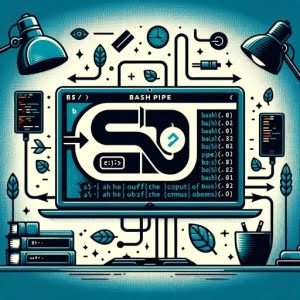
Are you finding it hard to grasp the concept of bash pipes? You’re not alone. Many developers find bash pipes a bit elusive, but we’re here to help.
Think of bash pipes as a relay race – passing the output of one command to another for further processing. It’s a powerful tool that can make your bash scripting more efficient and versatile.
In this guide, we’ll walk you through the process of using bash pipes, from the basics to more advanced techniques. We’ll cover everything from simple command chaining to complex multi-pipe scenarios, as well as alternative approaches.
Let’s dive in and start mastering bash pipes!
TL;DR: What is a Bash Pipe Used For?
A bash pipe, symbolized by
'|'
, is a powerful tool used to pass the output of one command as input to another, with the syntax[command1] | [command2]
. This allows for efficient chaining of commands in bash scripting.
Here’s a simple example:
ls | grep '.txt'
# Output:
# file1.txt
# file2.txt
# ...
In this example, the ls
command lists all files in the current directory. The output of ls
is then passed as input to the grep
command, which filters and displays only the ‘.txt’ files. This is a basic use of a bash pipe, but there’s so much more you can do with it.
Want to learn more about bash pipes and how to use them in more complex scenarios? Keep reading for a comprehensive guide on mastering bash pipes!
Table of Contents
Getting Started with Bash Pipes
Bash pipes are an essential tool in shell scripting, allowing you to chain commands together for more efficient and powerful operations. Let’s start with a simple example of a bash pipe in action.
echo 'Hello, bash pipes!' | wc -w
# Output:
# 3
In this example, we’re using the echo
command to output ‘Hello, bash pipes!’, and then piping that output into the wc -w
command, which counts the number of words in the input it receives. The result is ‘3’, which is the number of words in our initial string.
This is a simple yet powerful demonstration of how bash pipes can be used to process and manipulate data in bash scripting.
Advantages and Pitfalls of Using Bash Pipes
Bash pipes offer several key advantages. Firstly, they allow for efficient command chaining, enabling complex operations to be performed in a single line of code. This can significantly reduce the complexity and length of your scripts.
However, it’s essential to be aware of potential pitfalls. One common issue is that each command in the pipe is run in a subshell. This means that any variable assignments or other changes made within a command will not persist after the command finishes executing. This can lead to unexpected results if you’re not careful.
Despite these potential pitfalls, mastering bash pipes is a critical skill for any developer working with bash scripts. With practice, you’ll be able to leverage the power of bash pipes to streamline your code and perform complex operations with ease.
Delving Deeper: Multiple Bash Pipes
As you become more comfortable with bash pipes, you can start to chain multiple commands together. This allows you to perform more complex operations in a single line of code.
Let’s take a look at a more advanced example:
cat file.txt | grep 'bash' | wc -l
# Output:
# 10
In this example, we’re using the cat
command to read the contents of ‘file.txt’. The output of cat
is then piped into the grep
command, which filters and displays only the lines containing the word ‘bash’. The output of grep
is subsequently piped into the wc -l
command, which counts the number of lines in its input.
The result is ’10’, indicating that there are 10 lines in ‘file.txt’ that contain the word ‘bash’. This is a powerful demonstration of how multiple bash pipes can be used to perform complex data processing tasks in a single line of code.
Mastering the use of multiple bash pipes can greatly enhance your ability to perform complex operations in bash scripting. It allows you to write more efficient and powerful scripts, and is a key skill for any developer working with bash.
Exploring Alternatives: Redirection and More
While bash pipes are incredibly powerful, there are other tools and techniques available that can achieve similar results. One such tool is redirection.
Redirection: A Viable Alternative
Redirection in bash scripting allows you to control where the output of a command is sent. It can be used as an alternative to piping in certain scenarios. Here’s an example:
grep 'bash' file.txt > output.txt
cat output.txt
# Output:
# bash line 1
# bash line 2
# ...
In this example, we’re using the grep
command to filter lines containing the word ‘bash’ from ‘file.txt’. Instead of piping the output to another command, we’re redirecting it to a new file ‘output.txt’. The cat
command is then used to display the contents of ‘output.txt’.
Weighing the Pros and Cons
Redirection has its benefits. It can be more efficient than piping in scenarios where you need to store the output for later use. However, it also has drawbacks. For example, it requires additional disk space to store the output files, and it can be slower than piping if you’re working with large volumes of data.
In conclusion, while bash pipes are a powerful tool for chaining commands and processing data, they’re not the only tool at your disposal. By understanding and mastering alternative approaches like redirection, you can choose the best tool for each task and write more efficient and versatile bash scripts.
Troubleshooting Bash Pipes: Common Errors and Solutions
As with any coding tool, you might encounter obstacles or errors when using bash pipes. Let’s explore some common issues and how to solve them.
Problem: Unintended Word Splitting
One common issue is unintended word splitting. This happens when a command’s output contains spaces or other special characters that bash interprets as delimiters. Here’s an example:
echo 'Hello, bash pipes!' | wc
# Output:
# 1 3 18
In this example, the wc
command splits the input into words, leading to an unexpected output. The output ‘1 3 18’ represents the number of lines, words, and characters respectively.
Solution: Quoting and Escaping Special Characters
To prevent unintended word splitting, you can use quotes or escape special characters. Here’s the corrected code:
echo 'Hello, bash pipes!' | wc -m
# Output:
# 18
In this example, the -m
option tells wc
to count characters, not words. As a result, the output is ’18’, which is the number of characters in the input string.
Best Practices and Optimization Tips
When using bash pipes, keep these tips in mind for optimal performance and fewer errors:
- Always double-check your commands before piping their output to avoid unintended results.
- Use quotes or escape special characters to prevent unintended word splitting.
- Remember that each command in a pipe is run in a subshell, so changes made within a command (like variable assignments) won’t persist after the command finishes executing.
By understanding these common issues and how to solve them, you can use bash pipes more effectively and avoid common pitfalls.
Bash Pipes: The Inner Workings
To fully leverage the power of bash pipes, it’s crucial to understand their underlying principles and how they interact with the bash shell’s input and output mechanisms.
Bash I/O: The Basics
Bash handles input and output through something called file descriptors. By default, there are three file descriptors:
- Standard Input (stdin): File descriptor 0, typically the keyboard.
- Standard Output (stdout): File descriptor 1, typically the terminal screen.
- Standard Error (stderr): File descriptor 2, also typically the terminal screen.
These file descriptors form the basis of bash’s I/O operations.
The Role of Pipes
A pipe in bash takes the stdout of the command to its left and passes it as the stdin to the command on its right. Here’s a simple example:
echo 'Hello, bash pipes!' | wc -w
# Output:
# 3
In this example, the echo
command outputs ‘Hello, bash pipes!’, and the pipe (|
) takes this output and feeds it as input to the wc -w
command. The wc -w
command then counts the number of words in its input, resulting in the output ‘3’.
This is the fundamental principle behind bash pipes. By understanding this, you can start to see why bash pipes are so powerful: they allow you to chain commands together, with the output of one command serving as the input to the next. This enables complex, multi-step operations to be performed in a single line of code.
Expanding Horizons: Bash Pipes in Larger Projects
Bash pipes aren’t just for small, one-off scripts. They can play a vital role in larger projects and complex scripts, enabling you to streamline your code and perform intricate operations efficiently.
Leveraging Bash Pipes in Complex Scripts
Imagine you’re working on a script that needs to process large amounts of data. Instead of writing lengthy, convoluted code, you could use bash pipes to chain commands together and process the data in stages. This can make your script more efficient and easier to read and maintain.
Here’s an example of how you might use a bash pipe in a larger script:
# Generate a list of log files
ls /var/log | grep '.log'
# Output:
# syslog.log
# kern.log
# ...
In this example, we’re using a bash pipe to generate a list of all ‘.log’ files in the ‘/var/log’ directory. This could be the first step in a larger script that processes these log files in some way.
Related Commands and Concepts
When working with bash pipes, you’ll often find yourself using related commands and concepts. These might include redirection (>
and <
), command substitution ($(command)
), and process substitution ((command)
).
These tools can complement the use of bash pipes, allowing you to perform more complex operations and write more powerful scripts.
Further Resources for Bash Pipe Proficiency
If you’re looking to delve deeper into the world of bash scripting and bash pipes, here are some additional resources you might find useful:
- GNU Bash Manual: The official manual for the GNU Bash shell, including a comprehensive section on bash pipes.
- Advanced Bash-Scripting Guide: A detailed guide to advanced bash scripting techniques, including the use of pipes.
- TecAdmin’s Guide on Bash Pipe Operator – This guide provides an explanation of the pipe operator (|) in Bash scripting.
Wrapping Up: Bash Command Chaining with |
In this comprehensive guide, we’ve taken a deep dive into the world of bash pipes, a powerful tool for chaining commands and processing data in bash scripting.
We started with the basics, demonstrating how to use a bash pipe to pass the output of one command as input to another. We then progressed to more complex scenarios, exploring how to chain multiple commands together using multiple bash pipes.
We also delved into alternative approaches, such as redirection, giving you a wider toolkit for handling command output in bash scripting. Along the way, we discussed common challenges you might face when using bash pipes, such as unintended word splitting, and provided solutions to help you overcome these hurdles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Pitfalls |
---|---|---|
Bash Pipes | Efficient command chaining, powerful data processing | Each command runs in a subshell, changes don’t persist |
Redirection | Efficient for storing output for later use, avoids subshell issues | Requires additional disk space, can be slower with large data |
Whether you’re just starting out with bash pipes or looking to refine your skills, we hope this guide has given you a deeper understanding of bash pipes and their capabilities.
With their ability to streamline code and perform complex operations, bash pipes are a powerful tool in bash scripting. Now, you’re well equipped to leverage their benefits. Happy scripting!