Python Break: Guide to Controlling Loop Execution
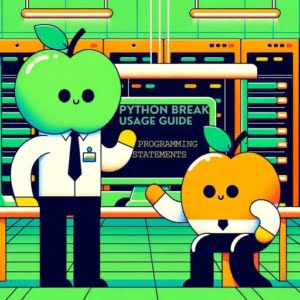
Proper usage of the ‘break’ statement in Python programming is crucial while automating tasks at IOFLOOD, as it enables premature termination of loop execution based on specified conditions. In our experience, the ‘break’ statement enhances code control and simplifies logic implementation, particularly in iterative tasks with exit conditions. Today’s article delves into how to effectively use the ‘break’ statement in Python, providing examples and explanations to empower our bare metal hosting customers with useful scripting techniques.
The ‘break’ statement is a powerful tool that allows you to stop the execution of a loop when a certain condition is met. This article will guide you through the use of the ‘break’ statement, from its basic usage to more advanced techniques. By the end, you’ll have a solid understanding of how to use ‘break’ to effectively control your loops in Python.
Let’s dive in to loop flow control with Python’s break’ statement!
TL;DR: How Do I Use the ‘Break’ Statement in Python?
The
break
statement in Python is used to prematurely exit afor
orwhile
loop when a certain condition is met.
Here’s a simple example to illustrate this:
for i in range(10):
if i == 5:
break
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the loop begins to iterate over the numbers 0 through 9. However, as soon as the variable i
equals 5, the ‘break’ statement is triggered, and the loop is immediately exited, hence you only see the numbers 0 through 4 printed.
Dive deeper into this article for a more comprehensive understanding of the ‘break’ statement, its advanced usage, and scenarios where you might want to use it.
Table of Contents
- Understanding the Basic Use of ‘Break’ in Python
- Tackling Nested Loops with ‘Break’
- Other Python Control Flow Tools: ‘Continue’ and ‘Pass’
- Troubleshooting ‘Break’ Statement Usage in Python
- Python Control Flow: The Role of ‘Break’
- ‘Break’ in Larger Scripts and Projects
- Exploring Related Topics: Exception Handling and Loop Optimization
- Wrapping Up: Python ‘Break’ Statement
Understanding the Basic Use of ‘Break’ in Python
The ‘break’ statement in Python is quite straightforward. It is used within a loop, and as soon as Python encounters this statement, it immediately exits the loop, regardless of whether the loop has fully completed its iterations or not.
Let’s consider a simple example to illustrate this concept:
for number in range(1, 11):
if number == 6:
break
print(number)
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the loop is supposed to iterate over the numbers 1 through 10. However, we’ve included a condition: if the variable number
equals 6, the ‘break’ statement is triggered and the loop is immediately exited. Therefore, only the numbers 1 through 5 are printed.
Advantages and Pitfalls of Using ‘Break’
The ‘break’ statement is a powerful tool that can provide a lot of flexibility in controlling the flow of your loops. It allows you to exit a loop as soon as a certain condition is met, which can be very useful in scenarios where you don’t need to complete all iterations of the loop.
However, it’s important to use the ‘break’ statement wisely. Overuse can lead to code that is difficult to read and debug, as it can become unclear where and why the loop is being exited. It’s also worth noting that the ‘break’ statement only exits the loop it is directly inside of; if used within nested loops, it will not affect the outer loops.
Tackling Nested Loops with ‘Break’
As you progress with Python, you’ll encounter situations where loops are nested within each other. The ‘break’ statement, when used in these scenarios, only exits the loop it is directly inside of. It does not affect the execution of the outer loops. Let’s illustrate this with a code example:
for outer in range(1, 4):
for inner in range(1, 4):
if inner == 2:
break
print('Outer:', outer, 'Inner:', inner)
# Output:
# Outer: 1 Inner: 1
# Outer: 2 Inner: 1
# Outer: 3 Inner: 1
In this example, we have an outer loop and an inner loop, both iterating through the numbers 1 to 3. The ‘break’ statement is placed in the inner loop, so when the inner
variable equals 2, the inner loop is exited. However, the outer loop continues its execution, hence you see the ‘Outer’ value changing in the output.
Analyzing the ‘Break’ Behaviour in Nested Loops
Understanding the behavior of the ‘break’ statement in nested loops is crucial for controlling the flow of complex programs. It’s important to remember that ‘break’ will only exit the loop it’s directly inside of. If you need to exit multiple levels of loop, you’ll need to architect your program in a way that allows this – perhaps by using a flag variable or by structuring your conditions differently.
Other Python Control Flow Tools: ‘Continue’ and ‘Pass’
While the ‘break’ statement is a powerful tool for controlling your loops in Python, it’s not the only one. Python provides other control flow statements such as ‘continue’ and ‘pass’ that you can use depending on your specific needs.
The ‘Continue’ Statement
The ‘continue’ statement, like ‘break’, influences the flow of your loop. However, instead of terminating the loop entirely, ‘continue’ simply skips the rest of the current iteration and moves on to the next one. Let’s illustrate this with a code example:
for number in range(1, 6):
if number == 3:
continue
print(number)
# Output:
# 1
# 2
# 4
# 5
In this example, the ‘continue’ statement is triggered when the variable number
equals 3. Instead of terminating the loop, Python simply skips the print statement for this iteration and moves on to the next number.
The ‘Pass’ Statement
The ‘pass’ statement in Python is a bit different. It’s a placeholder statement that does nothing. It’s commonly used in places where your code will syntactically require something, but you don’t want any operation to be performed. Here’s an example:
for number in range(1, 6):
if number == 3:
pass
else:
print(number)
# Output:
# 1
# 2
# 4
# 5
In this example, when the variable number
equals 3, the ‘pass’ statement does nothing, and Python simply moves on to the next iteration.
When to Use ‘Break’, ‘Continue’, and ‘Pass’
Choosing between ‘break’, ‘continue’, and ‘pass’ depends on what you need your loop to do. If you need to exit the loop entirely when a condition is met, use ‘break’. If you want to skip the rest of the current iteration and move on to the next one, use ‘continue’. And if you need a placeholder statement that does nothing, use ‘pass’.
Troubleshooting ‘Break’ Statement Usage in Python
Like any other programming concept, using the ‘break’ statement in Python can sometimes lead to unexpected results or errors. Understanding these common issues and how to resolve them can save you a lot of debugging time.
Misplaced ‘Break’ Statement
One common mistake is placing the ‘break’ statement outside a loop. Given that ‘break’ is designed to exit loops, using it outside a loop will result in a SyntaxError.
if True:
break
# Output:
# SyntaxError: 'break' outside loop
To resolve this, ensure that your ‘break’ statement is always used within a loop.
‘Break’ in Nested Loops
Another common point of confusion is when ‘break’ is used in nested loops. As we discussed earlier, ‘break’ only exits the loop it is directly inside of. If you need to break out of an outer loop from within an inner loop, you’ll need to use a different strategy, such as using a flag variable.
exit_outer_loop = False
for outer in range(1, 4):
for inner in range(1, 4):
if inner == 2:
exit_outer_loop = True
break
if exit_outer_loop:
break
print('Outer:', outer)
# Output:
# Outer: 1
In this example, we use a flag variable exit_outer_loop
to signal when we want to break out of the outer loop.
Best Practices for Using ‘Break’
While ‘break’ is a powerful tool, it’s best to use it sparingly. Overuse can lead to code that is difficult to understand and debug. Always consider whether there’s a way to structure your loop such that a ‘break’ statement is unnecessary. For example, you might be able to use a conditional statement to control your loop instead.
Python Control Flow: The Role of ‘Break’
To fully understand the ‘break’ statement in Python, we need to delve a bit deeper into the concept of control flow statements and loops.
Control Flow in Python
Control flow is a fundamental concept in programming that determines the order in which your code is executed. Python, like other programming languages, provides several control flow statements including ‘if’, ‘for’, ‘while’, ‘break’, ‘continue’, and ‘pass’. Each of these statements allows you to control your code’s execution in different ways.
The Concept of Loops
Loops are a key part of control flow. They allow you to execute a block of code repeatedly. Python provides ‘for’ and ‘while’ loops. The ‘for’ loop is used to iterate over a sequence (like a list, tuple, dictionary, string, or range) or other iterable objects. The ‘while’ loop, on the other hand, runs as long as a certain condition is True.
# for loop example
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
# while loop example
i = 0
while i < 5:
print(i)
i += 1
# Output:
# 0
# 1
# 2
# 3
# 4
In both examples, the loop prints the numbers 0 through 4. The ‘for’ loop does this by iterating over the sequence generated by range(5)
, while the ‘while’ loop does it by repeatedly checking whether i
is less than 5.
How ‘Break’ Affects Loop Execution
The ‘break’ statement directly influences the execution of loops. When Python encounters a ‘break’ statement within a loop, it immediately terminates that loop, even if the loop hasn’t finished iterating over all items in a sequence (in the case of a ‘for’ loop) or the loop condition is still True (in the case of a ‘while’ loop).
This ability to prematurely terminate a loop makes ‘break’ a powerful tool for controlling your code’s execution. However, it’s important to use it judiciously, as overuse can make your code harder to understand and debug.
‘Break’ in Larger Scripts and Projects
As you delve deeper into Python and start working on larger scripts and projects, you’ll find that the ‘break’ statement can play a significant role in controlling the flow of your code. For instance, you might use ‘break’ in a loop that’s processing user input or data from a file, allowing you to stop the processing as soon as you encounter a certain condition.
with open('large_file.txt', 'r') as file:
for line in file:
if 'ERROR' in line:
print('Error found! Stopping processing.')
break
# Output (example):
# Error found! Stopping processing.
In this example, we’re reading a large file line by line. As soon as we encounter a line that contains the word ‘ERROR’, we print a message and break out of the loop, stopping the processing of the file.
Exploring Related Topics: Exception Handling and Loop Optimization
The ‘break’ statement often comes into play alongside other important Python concepts like exception handling and loop optimization.
Exception handling allows you to deal with unexpected situations that arise during your code’s execution, while loop optimization techniques can help make your loops more efficient. Both of these concepts can be crucial when you’re working with ‘break’ in more complex scenarios.
Further Resources for Python Iterator Mastery
For more in-depth information on the above topics, consider exploring these online resources:
- Mastering Python Loop Structures – A complete guide on Python loops and their various types.
Python “continue” Statement – Explore the “continue” statement in Python and its role in skipping iteration.
Looping with Python “enumerate()” – Learn how to use “enumerate” to enhance loop processing.
Comparing Iterable and Iterator – This GeekforGeeks article explains the differences of Python iterables and iterators.
Iterators and Generators in Python – A Plain English guide that dives into the concept of iterators and generators in Python.
Control Structures in Python – A JavaTpoint tutorial that provides a comprehensive overview of control structures in Python.
These resources can provide you with a deeper understanding of how to write robust and efficient Python code.
Wrapping Up: Python ‘Break’ Statement
The ‘break’ statement is an essential tool in Python that allows you to control the flow of your loops. It enables you to prematurely exit a loop when a certain condition is met, providing flexibility in how your code is executed.
Here’s a quick recap of what we’ve covered:
- Basic Use: The ‘break’ statement is used within a loop. As soon as Python encounters it, the loop is immediately exited.
Advanced Use: In nested loops, ‘break’ only exits the loop it is directly inside of. It does not affect the execution of the outer loops.
Alternative Approaches: Python provides other control flow statements such as ‘continue’ and ‘pass’ that can be used depending on your specific needs.
Troubleshooting: Common issues include placing the ‘break’ statement outside a loop, which will result in a SyntaxError, and confusion when using ‘break’ in nested loops.
In conclusion, mastering the use of the ‘break’ statement will significantly improve your ability to write efficient and effective Python code. By understanding its basic usage and how it behaves in different scenarios, you can take better control of your loops and make your code more robust and readable.