Python Exception Handling Guide (With Examples)
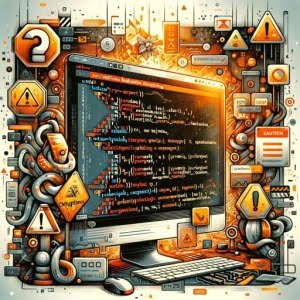
Are you finding Python exceptions a bit tricky to understand? Think of them as traffic signals for your code, guiding you when things take an unexpected turn. Just as a red light in traffic indicates a halt, a Python exception signals an error or an ‘exceptional’ condition in your code.
In this comprehensive guide, we will unravel everything you need to know about Python exceptions. We’ll start from the basics, gradually moving towards more advanced concepts.
So buckle up, and let’s start this exciting journey towards mastering Python exceptions!
TL;DR: What is a Python Exception?
A Python exception is an event that arises when an error occurs during the execution of a program. Python uses exception objects to represent these exceptions, signaling that something has gone awry. Here’s a simple example illustrating how to raise and handle an exception:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print(x)
# Output:
# 0
In this code snippet, we tried to divide 1 by 0, which is mathematically impossible and therefore an error. Python raises a ZeroDivisionError exception, which we catch using the except
clause and then set x
to zero. The program then continues, printing the value of x
, which is now 0.
For a more detailed explanation and advanced usage scenarios, let’s delve deeper into the world of Python exceptions!
Table of Contents
- Understanding Python Exceptions: The Basics
- Advanced Python Exceptions: Else and Finally Clauses
- Exploring Alternative Approaches to Python Exceptions
- Troubleshooting Python Exceptions
- Understanding Python’s Exception Hierarchy
- Python Exceptions in Larger Projects
- Further Resources for Exceptions and Testing
- Python Exceptions: A Recap
Understanding Python Exceptions: The Basics
In Python, when an error occurs during the execution of a program, an exception is raised. These exceptions can be caught and handled using the try/except
block. Let’s break down how this works.
The Try/Except Block: A Simple Example
Consider the following code:
try:
x = 1 / 0
except ZeroDivisionError:
x = 0
print(x)
# Output:
# 0
In this example, we attempt to divide 1 by 0 inside the try
block. Since division by zero is mathematically impossible, Python raises a ZeroDivisionError
exception. We catch this exception in the except
block and set x
to zero. Despite the error, our program continues without crashing and prints the value of x
, which is now 0.
Advantages and Potential Pitfalls
The main advantage of using a try/except
block is that it allows your program to handle errors gracefully instead of crashing. It gives you control over the flow of your program even in the face of exceptions.
However, a common pitfall when using try/except
blocks is catching all exceptions instead of specific ones. This can mask real issues in your code. Remember, it’s best practice to only catch exceptions that you know how to handle and let the rest propagate up.
By understanding the basic use of Python exceptions, you’re now equipped to handle errors in your code more efficiently. But that’s just scratching the surface. Let’s delve deeper into more advanced uses of Python exceptions in the next section.
Advanced Python Exceptions: Else and Finally Clauses
While the try/except
block forms the foundation of Python exceptions, there are additional clauses – else
and finally
– that provide more control and flexibility.
The Else Clause
The else
clause in a try/except
block executes if the try
block does not raise an exception. Here’s an example:
try:
x = 1 / 1
except ZeroDivisionError:
x = 0
else:
x = x * 2
print(x)
# Output:
# 2
In this code, since 1 divided by 1 does not raise a ZeroDivisionError
, the else
clause is executed and x
is doubled. The final value of x
, which is 2, is then printed.
The Finally Clause
The finally
clause executes no matter what, even if an exception is raised and not caught. It’s typically used for cleanup actions that must always be completed.
try:
x = 1 / 0
except ValueError:
x = 0
finally:
print('Cleanup actions here')
# Output:
# Cleanup actions here
# ZeroDivisionError: division by zero
In this example, even though a ZeroDivisionError
is raised (which is not caught as we’re only catching ValueError
), the finally
clause still executes.
Creating Custom Exceptions
Python allows you to define your own exceptions by creating a new exception class. This is useful when you want to raise an exception that describes a specific error condition in your program.
class CustomError(Exception):
pass
try:
raise CustomError('This is a custom exception')
except CustomError as e:
print(e)
# Output:
# This is a custom exception
Here, we’ve defined a new exception class called CustomError
. We then raise this exception in our try
block and catch it in our except
block.
These advanced techniques give you greater control over how Python exceptions are handled in your code, allowing you to manage errors more effectively.
Exploring Alternative Approaches to Python Exceptions
While try/except
blocks are the standard way to handle exceptions in Python, there are alternative methods that can provide more flexibility or simplicity in certain scenarios. Let’s explore two of these methods: the assert
statement and the with
statement.
Asserting Conditions with the Assert Statement
The assert
statement allows you to test if a condition in your code returns true, and if not, the program will raise an AssertionError
exception. Here’s an example:
x = 1
assert x == 0, 'x is not zero'
# Output:
# AssertionError: x is not zero
In this code, the assert
statement checks whether x
is equal to 0. Since x
is 1, the condition fails, and an AssertionError
with the message ‘x is not zero’ is raised.
The assert
statement is a useful tool for debugging and testing, but it should not be used for handling runtime exceptions. This is because assertions can be globally disabled with the -O
and -OO
command line switches, as well as the PYTHONOPTIMIZE
environment variable in Python.
Managing Resources with the With Statement
The with
statement in Python is not a direct method for handling exceptions, but it is often used with file operations or other tasks that require cleanup after completion, which makes it relevant in the context of error handling.
try:
with open('file.txt', 'r') as f:
contents = f.read()
except FileNotFoundError:
contents = None
print(contents)
# Output (if file.txt does not exist):
# None
Here, the with
statement is used to open a file. If the file does not exist, a FileNotFoundError
is raised, which we catch and handle by setting contents
to None
. The advantage of the with
statement is that it automatically closes the file after it’s done, even if an exception is raised within the block.
These alternative methods offer additional ways to handle exceptions and manage errors in your Python code. However, remember that the best method depends on your specific use case and requirements.
Troubleshooting Python Exceptions
Working with Python exceptions can sometimes be a bit tricky. There are common issues and pitfalls that you might encounter. Let’s discuss a few of these problems and their solutions.
Catching the Wrong Exception
One common mistake is catching the wrong exception. This can lead to unexpected behavior in your program. For instance, catching a TypeError
when you should be catching a ValueError
.
try:
x = int('a')
except TypeError:
x = 0
# Output:
# ValueError: invalid literal for int() with base 10: 'a'
In this example, we’re trying to convert a string ‘a’ to an integer, which raises a ValueError
. However, we’re catching a TypeError
, so the exception is not handled, and our program crashes.
Not Handling Exceptions Properly
Another common issue is not handling exceptions properly. This could mean not catching an exception at all, or not taking the appropriate action when an exception is caught.
try:
x = 1 / 0
except ZeroDivisionError:
pass
print(x)
# Output:
# NameError: name 'x' is not defined
In this code, we catch the ZeroDivisionError
but do nothing in the except
block. As a result, x
is never defined, and a NameError
is raised when we try to print x
.
Tips for Troubleshooting Python Exceptions
- Always catch the specific exceptions that you know how to handle.
- Ensure that you take appropriate action in your
except
block to handle the exception. - Use the
else
andfinally
clauses in yourtry/except
block for code that should run whether or not an exception is raised.
By understanding these common issues and their solutions, you can avoid these pitfalls and handle Python exceptions effectively in your code.
Understanding Python’s Exception Hierarchy
To effectively handle Python exceptions, we need to understand the hierarchy of Python’s built-in exceptions. This hierarchy is structured as a tree with BaseException
as the root, which is the base class for all built-in exceptions.
Python’s Built-In Exceptions
Here are some of the more common built-in exceptions you might encounter:
Exception
: This is the base class for most error types.ArithmeticError
: This is the base class for exceptions that occur for numeric calculations.ZeroDivisionError
: This is a subclass ofArithmeticError
and is raised when you’re trying to divide by zero.FileNotFoundError
: This exception is raised when a file or directory is requested but doesn’t exist.
You can view the full list of Python’s built-in exceptions in the Python documentation.
Python Exceptions in Action
Let’s see a simple example of how Python uses exceptions to handle errors.
try:
x = 1 / 0
except ZeroDivisionError as e:
print(f'Caught an exception: {e}')
# Output:
# Caught an exception: division by zero
In this example, we’re trying to divide 1 by 0, which raises a ZeroDivisionError
. We catch this exception and print a message, preventing our program from crashing.
This is the essence of Python exceptions: they provide a way to detect and handle errors or exceptional conditions in your code, allowing you to control the flow of your program and prevent crashes. Understanding this concept and the hierarchy of Python exceptions is crucial to mastering Python exception handling.
Python Exceptions in Larger Projects
As your Python projects grow in size and complexity, the proper handling of exceptions becomes increasingly important. In large codebases, unhandled exceptions can cause your program to crash unexpectedly or behave unpredictably.
Best Practices for Exception Handling
Here are some best practices for handling exceptions in Python projects:
- Always catch exceptions that you know how to handle and let the rest propagate up.
- Use the most specific exceptions possible in your
except
clauses. - Avoid catching the base
Exception
class, as this can mask real issues in your code. - Use the
finally
clause for cleanup actions that must always be completed.
Exploring Related Concepts
Beyond exceptions, there are other related concepts in Python that can help you write more robust and error-free code. Logging and error reporting are two such concepts.
- Logging: This is the process of recording events in your program, such as user actions, system events, or errors. Python’s built-in
logging
module provides a flexible framework for emitting log messages from your code. Error Reporting: This is the process of automatically reporting unhandled exceptions to a bug tracking system. Python’s
traceback
module can be used to extract, format, and print exceptions and their accompanying stack traces.
For a deeper understanding of Python exceptions and related concepts, you can explore the Python documentation or the other online resources listed below.
Further Resources for Exceptions and Testing
To deepen your understanding of Python exception handling, here are some carefully curated resources that will prove helpful:
- Pytest Best Practices: Writing Clean and Effective Tests: Discover how Pytest simplifies test discovery, making it easy to find and run your tests.
Dealing with KeyError Exceptions in Python: Best Practices: Master the art of handling KeyError exceptions in Python for reliable dictionary operations.
Dealing with Value Errors in Python: Practical Tips and Examples: Master the art of gracefully handling value-related errors in Python to improve code robustness.
Troubleshooting Python Scripts: This PDF by Esri shares tips and techniques for troubleshooting Python scripts.
Python Debugging Tools: The Python Wiki provides a comprehensive list and description of the various debugging tools available for Python.
W3Schools: Python Raise: This glossary entry from W3Schools explains the use of the “raise” keyword in Python for generating exceptions.
Remember, mastering exceptions is a crucial step towards becoming a proficient Python programmer.
Python Exceptions: A Recap
We’ve taken a deep dive into the world of Python exceptions, exploring their usage, common issues, and solutions. We’ve seen how Python exceptions act as traffic signals for your code, signaling when something goes wrong and providing a way to handle these errors gracefully.
Key Takeaways
- Python exceptions are raised when an error occurs during the execution of a program.
- The
try/except
block is the cornerstone of Python exception handling. - The
else
andfinally
clauses provide additional control over the flow of your program. - Python allows you to define your own exceptions for specific error conditions.
- Alternative methods like the
assert
statement and thewith
statement offer additional ways to handle exceptions.
Common Issues and Solutions
- Catching the wrong exception can lead to unexpected behavior. Always catch the specific exceptions that you know how to handle.
- Not handling exceptions properly can cause your program to crash or behave unpredictably. Take appropriate action in your
except
block to handle the exception.
Comparison of Exception Handling Methods
Method | Use Case | Pros | Cons |
---|---|---|---|
try/except | General error handling | Full control over error handling | Can mask real issues if not used properly |
assert | Debugging and testing | Simple syntax, useful for catching programming errors | Not suitable for handling runtime exceptions |
with | Resource management | Automatically manages resources, even if an exception is raised | Not a direct method for handling exceptions |
By understanding these concepts and best practices, you’re now equipped to handle Python exceptions effectively in your code. As a result, you can write more robust and error-free Python programs.