SQL “Join” | Learn to use the Types of Joins in SQL
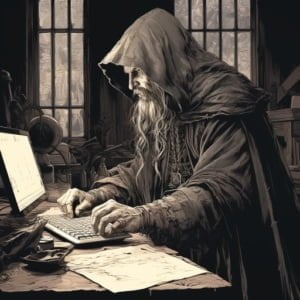
Are you intrigued by the process of merging data from multiple tables in a database? Or have you ever been puzzled by a complex data extraction task? If your answer is yes, then it’s high time you learn about the power of SQL Join statements. SQL Joins are more than just a tool; they are the secret sauce in managing and manipulating relational databases.
There are several types of SQL Joins available, each with their unique functions. They allow you to merge data from different tables based on common fields with ease. But the question arises, how do you determine which SQL Join to use? When is it appropriate to use one type of join over another?
This blog post aims to unravel the mystery of SQL Joins, providing you with a comprehensive understanding of different join types and their practical usage. So, whether you’re an experienced SQL user or a novice, get ready for an enlightening journey into the world of SQL Joins.
TL;DR: What is an SQL Join?
An SQL Join is a powerful statement in SQL that allows you to combine rows from two or more tables based on a related column between them. See example:
SELECT Orders.OrderNumber, Customers.CustomerName
FROM Orders
JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
Table of Contents
Introduction to SQL Join
To begin, let’s define what an SQL Join is and why it’s an essential tool for anyone working with databases. In simple terms, an SQL Join is a command that merges rows from two or more tables based on a related column between them, often known as a ‘common field’. This common field acts as the binding factor for your data, enabling you to manipulate and retrieve data from multiple tables as if they were a single entity.
There are several types of SQL Joins, each with its unique purpose and usage. These include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN. For example, an INNER JOIN fetches only the records with matching values in both tables, while a LEFT JOIN returns all the records from the left table, and the matched records from the right table.
But why exactly are SQL Joins so crucial? To understand this, consider the concept of table relations in databases. Relational databases are designed to distribute and store data across different tables to prevent data redundancy. SQL Joins, therefore, form the backbone of data retrieval in these databases, enabling you to efficiently gather this dispersed data whenever required.
Comparison of Different Types of SQL Joins:
SQL Join Type | Description |
---|---|
INNER JOIN | Returns records that have matching values in both tables. |
LEFT JOIN | Returns all records from the left table, and the matched records from the right table. |
RIGHT JOIN | Returns all records from the right table, and the matched records from the left table. |
FULL JOIN | Returns all records when there is a match in either left or right table. |
Mastering SQL Joins is like having a powerful wizard at your disposal, one that can magically unify disparate pieces of data into a coherent whole. This capability can significantly enhance your data management tasks.
SQL Joins: The Magic Wand in Data Management
Think of SQL Joins as puzzle pieces. Just as you need the correct pieces to complete a puzzle and reveal the full picture, SQL Joins help you bring together relevant data from different tables to create meaningful information. This analogy makes the concept of SQL Joins more relatable, especially for beginners.
In-depth Look at INNER JOIN
Now that we have a basic understanding of SQL Joins and their significance, it’s time to delve deeper into one of the most frequently used types of SQL Joins – the INNER JOIN.
An INNER JOIN is a specific type of SQL Join that fetches only the records with matching values in both tables. In simpler terms, it retrieves the intersection of two tables based on the common field. This concept can be likened to finding mutual friends between two individuals – you’re only interested in the friends that both people have in common.
Syntax of INNER JOIN
The syntax for an INNER JOIN is as follows:
SELECT column_name(s)
FROM table1
INNER JOIN table2
ON table1.column_name = table2.column_name;
Practical Example
Let’s illustrate the use of INNER JOIN with a practical example. Assume we have two tables – Orders
and Customers
– and we want to identify all the orders made by a specific customer. An INNER JOIN would be ideal for this task:
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers
ON Orders.CustomerID = Customers.CustomerID;
The above SQL statement will yield a list of OrderIDs along with the names of the customers who placed those orders.
Example of expected output:
OrderID | CustomerName |
---|---|
1 | John Doe |
2 | Jane Doe |
But what if you’ve encountered SQL statements where the keyword INNER is omitted, and it just states JOIN? Is there a difference? The answer is no. The term ‘JOIN’ is often used interchangeably with ‘INNER JOIN’. It’s simply a shorthand way of writing it. So, whether you see ‘JOIN’ or ‘INNER JOIN’, understand that they’re identical.
And here’s an interesting fact – you’re not confined to joining just two tables. SQL JOIN operations can be extended to three, four, or even more tables, providing you with even greater versatility in data manipulation.
In conclusion, INNER JOIN is a potent tool in your SQL arsenal, one that is fundamental to merging data in relational databases. Mastering it opens up a world of possibilities in managing and retrieving your data.
Understanding LEFT JOIN and RIGHT JOIN
Having explored the realm of INNER JOIN, it’s time to shift our focus to two other significant types of SQL Joins – LEFT JOIN and RIGHT JOIN.
What is LEFT JOIN?
A LEFT JOIN returns all the records from the left table, and the matched records from the right table. In cases where there’s no match, the result is NULL on the right side. Think of it as asking for all the friends of person A and, if person B happens to be a friend, including their details as well. If person B isn’t a friend, their details would be NULL.
The syntax for a LEFT JOIN is as follows:
SELECT column_name(s)
FROM table1
LEFT JOIN table2
ON table1.column_name = table2.column_name;
What is RIGHT JOIN?
On the other hand, RIGHT JOIN returns all the records from the right table, and the matched records from the left table. If there’s no match, the result is NULL on the left side. It’s essentially the mirror image of a LEFT JOIN.
The syntax for a RIGHT JOIN is:
SELECT column_name(s)
FROM table1
RIGHT JOIN table2
ON table1.column_name = table2.column_name;
Practical Example
Let’s consider a practical example. Suppose we have two tables – Orders
and Customers
– and we want to find out all customers and any orders they might have made. A LEFT JOIN would be the perfect tool for this task:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
LEFT JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
The above SQL statement will return a list of all customers and any orders they have made. If a customer hasn’t made any orders, the OrderID will be NULL.
Example of expected output:
CustomerName | OrderID |
---|---|
John Doe | 1 |
Jane Doe | NULL |
OUTER JOIN
You might have come across OUTER JOIN and wondered how it relates to LEFT JOIN and RIGHT JOIN. An OUTER JOIN returns all records when there is a match in either the left or the right table records. A FULL OUTER JOIN combines the results of both left and right outer joins and returns all (matched or unmatched) records from both tables. LEFT JOIN and RIGHT JOIN are essentially types of OUTER JOINS.
In the broader context of data management, LEFT JOIN and RIGHT JOIN are strategic tools that allow you to retrieve not just matching data, but also unmatched data from one or both tables. They provide a more comprehensive view of your data, making them indispensable tools in your SQL toolkit.
Efficient Use of Table Aliases
As we delve further into the world of SQL Joins, it’s time to introduce a useful companion that can make your SQL queries more manageable and legible – table aliases.
A table alias is a temporary name assigned to a table in a SQL statement. Think of it as a short-term nickname for your table, which exists only for the duration of your query. But why would you want to assign nicknames to your tables? The answer lies in the two primary functions of table aliases – simplifying queries and enhancing readability.
Consider the scenario where you’re working with tables that have lengthy or complicated names. Repeatedly writing out these names every time you reference them in your SQL statement can be tedious and can clutter your query. This is where table aliases come to the rescue. By assigning a concise, intuitive alias to your table, you can maintain a tidy and succinct query.
The process of using table aliases in SQL Joins is straightforward. You simply introduce the alias right after the table name, as shown below:
SELECT column_name(s)
FROM table1 AS alias1
JOIN table2 AS alias2
ON alias1.column_name = alias2.column_name;
Let’s put this into practice with a tangible example. Assume we have two tables – Orders
and Customers
– and we want to identify all the orders placed by a specific customer. Using table aliases, our SQL statement would look like this:
SELECT o.OrderID, c.CustomerName
FROM Orders AS o
INNER JOIN Customers AS c
ON o.CustomerID = c.CustomerID;
In the above SQL statement, ‘o’ is the alias for the Orders
table and ‘c’ is the alias for the Customers
table. This simplifies our query, making it much easier to read and comprehend.
The advantages of using table aliases in SQL Joins are clear. They not only simplify your queries and enhance readability, but they also make your SQL statements easier to maintain and debug. So, the next time you’re working with SQL Joins, don’t forget to bring along your new ally – the table alias!
Conclusion
We’ve embarked on a comprehensive journey through the world of SQL Joins, exploring their potency, versatility, and crucial role in managing and manipulating relational databases. We’ve unraveled the mystery of INNER JOIN, the most frequently used SQL Join that fetches only the records with matching values in both tables.
We’ve also navigated the landscapes of LEFT JOIN and RIGHT JOIN, which provide a more holistic view of our data by returning not only matching records but also unmatched ones from one or both tables. Furthermore, we’ve welcomed a valuable companion into our SQL toolkit – the table alias, a temporary nickname for our tables that enhances readability and simplifies our queries.
SQL Joins, with their diverse types and functions, are the secret ingredient in merging disparate pieces of data into a coherent whole. They form the backbone of data retrieval in relational databases, and mastering them paves the way for a myriad of possibilities in managing and retrieving your data.
Remember, SQL Joins are more than just a tool. They are your powerful wizard in the realm of data management. Whether you’re a seasoned SQL user or a beginner, the knowledge of SQL Joins can transform your data handling skills, enabling you to conjure up meaningful information from a sea of data.
So, keep exploring, keep learning, and let the power of SQL Joins guide you in your data management tasks.