Deque Java: Add and Remove Elements with Ease
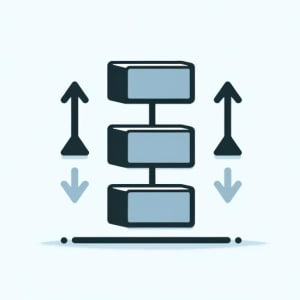
Have you ever found yourself needing to manage elements in Java with flexibility at both ends? Like a two-way street, Deque in Java allows you to add or remove elements from both ends. It’s a versatile tool that can be a game-changer in your Java coding journey.
This guide will walk you through the basics of using Deque, an interface that extends the Queue interface in Java. We’ll explore everything from the basic use of Deque to more advanced techniques, as well as alternative approaches. So, let’s dive in and start mastering Deque in Java!
TL;DR: What is Deque in Java and How Do I Use It?
Deque
in Java is a double-ended queue that allows you to add, with syntaxdeque.addFirst("");
ordeque.addLast("");
, or remove elements, with syntax,deque.removeFirst();
ordeque.removeLast();
from both ends. It’s a part of the Java Collections Framework and extends the Queue interface.
Here’s a simple example:
Deque<String> deque = new ArrayDeque<>();
deque.addFirst("First");
deque.addLast("Last");
// Output:
// [First, Last]
In this example, we create a Deque of Strings using the ArrayDeque implementation. We then add ‘First’ to the beginning of the Deque using the addFirst()
method and ‘Last’ to the end of the Deque using the addLast()
method. The output shows that ‘First’ is at the beginning and ‘Last’ is at the end of the Deque.
This is just a basic way to use Deque in Java, but there’s much more to learn about managing elements in a double-ended queue. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Deque in Java: Basic Use
Deque in Java provides several methods that allow you to manipulate elements at both ends of the queue. Let’s dive into some of the basic methods you’ll likely use when working with Deque.
Adding Elements: addFirst()
and addLast()
These methods allow you to add elements to the beginning and end of the Deque. Here’s how it works:
Deque<String> deque = new ArrayDeque<>();
deque.addFirst("Element 1");
deque.addLast("Element 2");
// Output:
// [Element 1, Element 2]
In this example, we’re adding ‘Element 1’ to the beginning of the Deque and ‘Element 2’ to the end. The output shows that ‘Element 1’ is at the beginning and ‘Element 2’ is at the end of the Deque.
Removing Elements: removeFirst()
and removeLast()
Similarly, Deque provides methods to remove elements from both ends. Here’s an example:
Deque<String> deque = new ArrayDeque<>();
deque.addFirst("Element 1");
deque.addLast("Element 2");
deque.removeFirst();
deque.removeLast();
// Output:
// []
In this case, we’re removing the first and last elements from the Deque. The output shows an empty Deque, as all elements have been removed.
It’s important to note that these remove
methods throw a NoSuchElementException
if the Deque is empty. So, always ensure that your Deque has elements before trying to remove them. We’ll discuss more about handling such issues in the ‘Troubleshooting and Considerations’ section.
Deque in Java: Advanced Use
Let’s delve deeper into the Deque interface and explore some more complex methods and their uses.
Accessing Elements: getFirst()
and getLast()
These methods allow you to access the first and last element of the Deque without removing them. Here’s an example:
Deque<String> deque = new ArrayDeque<>();
deque.addFirst("Element 1");
deque.addLast("Element 2");
String firstElement = deque.getFirst();
String lastElement = deque.getLast();
// Output:
// First Element: Element 1
// Last Element: Element 2
In this example, we’re accessing the first and last elements using getFirst()
and getLast()
. The output shows ‘Element 1’ as the first element and ‘Element 2’ as the last element. These methods are useful when you need to peek at the elements without altering the Deque.
Adding Elements Safely: offerFirst()
and offerLast()
These methods add elements to the Deque just like addFirst()
and addLast()
, but they return a boolean indicating success or failure instead of throwing an exception if the Deque is full. Let’s see it in action:
Deque<String> deque = new ArrayDeque<>();
boolean isFirstAdded = deque.offerFirst("Element 1");
boolean isLastAdded = deque.offerLast("Element 2");
// Output:
// First Element Added: true
// Last Element Added: true
In this example, we’re adding ‘Element 1’ to the beginning and ‘Element 2’ to the end of the Deque. The output shows that both elements are successfully added.
Removing Elements Safely: pollFirst()
and pollLast()
Similar to offerFirst()
and offerLast()
, these methods remove elements from the Deque and return the removed element, or null if the Deque is empty. Here’s an example:
Deque<String> deque = new ArrayDeque<>();
deque.addFirst("Element 1");
deque.addLast("Element 2");
String removedFirst = deque.pollFirst();
String removedLast = deque.pollLast();
// Output:
// Removed First Element: Element 1
// Removed Last Element: Element 2
In this case, we’re removing the first and last elements from the Deque. The output shows ‘Element 1’ as the removed first element and ‘Element 2’ as the removed last element. These methods are handy when you need to remove elements without risking an exception if the Deque is empty.
Exploring Alternatives to Deque in Java
While Deque is a powerful tool, Java provides other data structures that can be used as alternatives. Let’s take a look at two of these alternatives: Stack and LinkedList.
Java Stack: A LIFO Data Structure
Stack is a Last-In-First-Out (LIFO) data structure. Here’s a simple example of how to use Stack in Java:
Stack<String> stack = new Stack<>();
stack.push("Element 1");
stack.push("Element 2");
String poppedElement = stack.pop();
// Output:
// Popped Element: Element 2
In this example, we’re adding ‘Element 1’ and ‘Element 2’ to the Stack. The pop()
method removes and returns the last added element. So, the output shows ‘Element 2’ as the popped element.
While a stack can be a good alternative for certain use cases, it only allows element manipulation at one end. This limitation can be a disadvantage when compared to a Deque.
Java LinkedList: A List and Deque
LinkedList in Java is a doubly-linked list implementation of the List and Deque interfaces. It allows element manipulation at both ends, similar to a Deque. Here’s an example:
LinkedList<String> linkedList = new LinkedList<>();
linkedList.addFirst("Element 1");
linkedList.addLast("Element 2");
String firstElement = linkedList.getFirst();
String lastElement = linkedList.getLast();
// Output:
// First Element: Element 1
// Last Element: Element 2
In this example, we’re adding ‘Element 1’ to the beginning and ‘Element 2’ to the end of the LinkedList. The getFirst()
and getLast()
methods return the first and last elements. So, the output shows ‘Element 1’ as the first element and ‘Element 2’ as the last element.
LinkedList can be a great alternative to Deque as it provides similar functionality. However, it can be slower than ArrayDeque (an implementation of Deque) for queue operations. So, your choice between LinkedList and Deque depends on your specific requirements and the trade-offs you’re willing to make.
Dealing with Deque in Java: Troubleshooting and Considerations
Working with Deque in Java is generally smooth sailing, but there are a few common issues that you might encounter. Let’s discuss these potential problems and how to solve them.
NoSuchElementException
One common issue is the NoSuchElementException
. This exception is thrown by methods like getFirst()
, getLast()
, removeFirst()
, and removeLast()
when trying to retrieve or remove an element from an empty Deque. Here’s an example:
Deque<String> deque = new ArrayDeque<>();
try {
deque.removeFirst();
} catch (NoSuchElementException e) {
System.out.println("Caught NoSuchElementException");
}
// Output:
// Caught NoSuchElementException
In this example, we’re trying to remove the first element from an empty Deque, which results in a NoSuchElementException
. The output shows the exception being caught and handled.
To avoid this issue, always check if the Deque is empty before trying to retrieve or remove an element. You can do this using the isEmpty()
method, like so:
Deque<String> deque = new ArrayDeque<>();
if (!deque.isEmpty()) {
deque.removeFirst();
}
In this case, the removeFirst()
method is only called if the Deque is not empty, preventing the NoSuchElementException
.
Remember, understanding the potential pitfalls and how to handle them is key to mastering the use of Deque in Java. Happy coding!
Understanding Queue Interface and Deque in Java
Before we delve further into the Deque interface, it’s essential to understand the Queue interface and the concept of double-ended queues.
Java Queue Interface: A Brief Overview
The Queue interface in Java is a part of the Java Collections Framework and provides the functionality of a typical queue data structure – First-In-First-Out (FIFO). Here’s a simple example of a Queue in Java:
Queue<String> queue = new LinkedList<>();
queue.add("Element 1");
queue.add("Element 2");
String head = queue.peek();
// Output:
// Head: Element 1
In this example, we’re adding ‘Element 1’ and ‘Element 2’ to the Queue. The peek()
method returns the head of the queue without removing it. So, the output shows ‘Element 1’ as the head of the queue.
Deque: Extending the Queue Interface
Deque in Java extends the Queue interface to provide additional functionality. The term ‘Deque’ stands for ‘Double Ended Queue’, which means it allows you to add or remove elements from both ends.
This flexibility makes Deque a powerful tool for a variety of applications, from implementing stacks and queues to other more complex data structures.
In the following sections, we’ll explore how to leverage the power of Deque in Java, from basic use to more advanced techniques, and how to handle common issues you might encounter along the way.
Deque in Java: Beyond the Basics
The Deque interface in Java is not just a theoretical concept, but a practical tool used in real-world applications. Its flexibility and efficiency make it a go-to choice for a variety of scenarios.
Deque in Algorithm Implementation
Deque is often used in the implementation of certain algorithms. For instance, in graph algorithms like Breadth-First Search (BFS), a Deque can be used to hold the nodes to be processed. Its ability to add and remove elements from both ends can be leveraged to optimize the algorithm.
Deque in Data Streaming Applications
In data streaming applications where you need to maintain a ‘window’ of the last ‘n’ elements, Deque can be a perfect fit. You can easily add new elements to one end and remove old elements from the other end, keeping the ‘window’ at a fixed size.
Further Resources for Deque in Java
To deepen your understanding of Deque in Java, here are some resources worth exploring:
- Best Practices for Using Data Structures in Java – Discover common data structure algorithms and implementations in Java.
Introduction to Java Generics – Master using generics for creating reusable and data structures and algorithms in Java.
Java Set Overview – Learn about Set in Java, representing a collection of unique elements with no duplicates.
Java Collections Framework Tutorial by Oracle provides a comprehensive overview of the Java Collections Framework.
Java Deque Interface by GeeksforGeeks offers a detailed guide on the Deque interface.
Java Programming Masterclass for Software Developers covers the fundamentals of Java programming, including the use of Deque.
Remember, mastering Deque in Java is not just about understanding its methods, but also about knowing how and when to use them effectively in your code.
Wrapping Up: Deque in Java
In this comprehensive guide, we’ve explored the Deque interface in Java, its usage, and its relevance in real-world applications. We’ve delved into the depths of Deque, from the basic methods to more advanced techniques, providing you with a robust understanding of this powerful tool.
We began with the basics, understanding how to add and remove elements using methods like addFirst()
, addLast()
, removeFirst()
, and removeLast()
. We then moved to more advanced usage, exploring methods like getFirst()
, getLast()
, offerFirst()
, offerLast()
, pollFirst()
, and pollLast()
. Each step of the way, we provided practical examples to help you understand how these methods work in real code.
We also discussed common issues you might encounter when using Deque, such as the NoSuchElementException
, and provided solutions to help you troubleshoot these problems. Additionally, we explored alternative approaches to Deque, taking a look at Stack and LinkedList, and compared their usage and effectiveness.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Deque | Flexible, allows manipulation at both ends | May throw exceptions if not used carefully |
Stack | Simple, easy to use | Only allows manipulation at one end |
LinkedList | Flexible, allows manipulation at both ends | Can be slower than Deque for queue operations |
Whether you’re just starting out with Deque in Java or you’re looking to level up your skills, we hope this guide has been a valuable resource. With its flexibility and efficiency, Deque is a powerful tool in your Java coding arsenal. Happy coding!