Java Set: Guide to Managing Collections of Elements
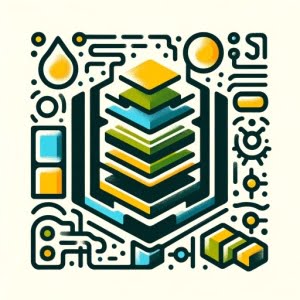
Are you finding it challenging to work with Java Set? You’re not alone. Many developers find themselves puzzled when it comes to handling Sets in Java, but we’re here to help.
Think of Java’s Set as a unique container – a container that holds non-duplicate elements, providing a versatile and handy tool for various tasks.
This guide will walk you through the ins and outs of using Set in Java, from basic operations to advanced techniques. We’ll cover everything from the creation, manipulation, and usage of Sets to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Java Set!
TL;DR: How Do I Use a Set in Java?
To use a Set in Java, you need to create an instance of it,
Set<String> set = new 'setType'<>();
then use its methods to add or remove elements. It’s a simple yet powerful tool for handling non-duplicate elements.
Here’s a simple example:
Set<String> set = new HashSet<>();
set.add('Hello');
System.out.println(set);
// Output:
// ['Hello']
In this example, we create a new instance of a HashSet, which is a type of Set. We then add the string ‘Hello’ to the Set. When we print the Set, we see that it contains the element we added.
This is just a basic way to use a Set in Java, but there’s so much more to explore. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Java Set Basics: Creation, Addition, and Iteration
Starting with the basics, let’s dive into how to create a Set, add elements, and iterate over it.
Creating a Set in Java
In Java, we can create a Set using various classes like HashSet, TreeSet, and LinkedHashSet. All these classes implement the Set interface. Here’s how to create a Set:
Set<String> set = new HashSet<>();
In this code block, we’re creating a new HashSet and assigning it to a Set reference. This Set is designed to hold String values.
Adding Elements to a Set
Once you have a Set, you can add elements using the add() method. Remember, Sets only store unique elements. Here’s how to add elements:
set.add('Hello');
set.add('World');
System.out.println(set);
// Output:
// ['Hello', 'World']
In this example, we’re adding ‘Hello’ and ‘World’ to our Set. When we print the Set, we see both elements.
Iterating Over a Set
To go through each element in a Set, you can use a for-each loop. Here’s an example:
for (String element : set) {
System.out.println(element);
}
// Output:
// 'Hello'
// 'World'
In this code block, we’re using a for-each loop to iterate over the Set and print each element.
Understanding these basic operations is the first step to mastering Java Set. But as with any tool, there are potential pitfalls to avoid and best practices to follow, which we’ll explore in the following sections.
Advanced Java Set Operations: Duplicates, Checks, and Comparisons
As you become more familiar with Java Set, you’ll encounter situations that require more complex operations. Let’s discuss some of these scenarios.
Removing Duplicates
One of the main benefits of a Set is its inherent ability to remove duplicate elements. Here’s an example:
Set<String> set = new HashSet<>();
set.add('Java');
set.add('Java');
System.out.println(set);
// Output:
// ['Java']
In this code, we’re trying to add ‘Java’ to our Set twice. But when we print the Set, we see that ‘Java’ only appears once. This is because Sets automatically remove duplicate elements.
Checking if an Element Exists
You can check if a Set contains a specific element using the contains() method. Here’s how:
boolean exists = set.contains('Java');
System.out.println(exists);
// Output:
// true
In this example, we’re checking if ‘Java’ exists in our Set. The contains() method returns true because ‘Java’ is indeed in our Set.
Comparing Two Sets
To compare two Sets for equality, you can use the equals() method. Here’s an example:
Set<String> set1 = new HashSet<>();
set1.add('Java');
Set<String> set2 = new HashSet<>();
set2.add('Java');
boolean isEqual = set1.equals(set2);
System.out.println(isEqual);
// Output:
// true
In this code, we’re creating two Sets and adding ‘Java’ to both. When we compare them using the equals() method, it returns true because both Sets contain the same elements.
Mastering these advanced operations will help you make the most out of Java Set. Remember, best practices involve understanding when and how to use these operations effectively.
Exploring Alternatives to Java Set
While Sets are powerful, it’s equally important to understand alternative data structures in Java, such as List and Map, and when to use each.
When to Use List
List is an ordered collection and can contain duplicate elements. You can use it when you need to maintain the insertion order or when duplicates are important.
Here’s how to use a List:
List<String> list = new ArrayList<>();
list.add('Java');
list.add('Java');
System.out.println(list);
// Output:
// ['Java', 'Java']
In this example, we’re adding ‘Java’ twice to our List. When we print the List, we see ‘Java’ twice because Lists allow duplicates.
When to Use Map
Map is an object that maps keys to values. A map cannot contain duplicate keys; each key can map to at most one value. Use it when you need to associate specific keys with values.
Here’s how to use a Map:
Map<String, Integer> map = new HashMap<>();
map.put('Java', 1);
map.put('Python', 2);
System.out.println(map);
// Output:
// {'Java'=1, 'Python'=2}
In this code, we’re associating ‘Java’ with 1 and ‘Python’ with 2. When we print the Map, we see each key paired with its value.
While Set, List, and Map each have their uses, it’s crucial to choose the right data structure for your specific needs. Understanding their differences and advantages will help you make an informed decision and write more effective code.
Troubleshooting Java Set Issues
Working with Java Set isn’t always smooth sailing. You may encounter some common issues, such as handling null values and concurrency problems. Let’s discuss these issues and how to overcome them.
Handling Null Values
While a Java Set can contain a null value, it can only contain one. Adding a null value more than once won’t cause an error, but it won’t increase the size of the Set either. Here’s an example:
Set<String> set = new HashSet<>();
set.add(null);
set.add(null);
System.out.println(set.size());
// Output:
// 1
In this code, we’re adding null to our Set twice. However, when we print the size of the Set, it only counts as one element.
Addressing Concurrency Issues
If multiple threads access and modify a Set concurrently, it can lead to data inconsistency. To solve this, you can use synchronized Sets or concurrent Set implementations like CopyOnWriteArraySet. Here’s an example of a synchronized Set:
Set<String> set = Collections.synchronizedSet(new HashSet<>());
In this code, we’re creating a synchronized Set using the Collections.synchronizedSet() method. This Set is safe to use across multiple threads.
Understanding these common issues and their solutions will help you work more effectively with Java Set. Remember, the key to successful programming is not just knowing how to write code, but also how to debug it and handle potential pitfalls.
Understanding Java Set Fundamentals
To fully appreciate Java Set and its capabilities, we need to delve into its inner workings. This includes understanding its implementation and the role of crucial methods like equals() and hashCode().
Java Set Implementation
In Java, Set is an interface in the Java Collections Framework. It has various implementations, including HashSet, TreeSet, and LinkedHashSet. Each implementation has its characteristics and use cases:
- HashSet: This class implements the Set interface, backed by a hash table (actually a HashMap instance). It makes no guarantees as to the iteration order of the set; in particular, it does not guarantee that the order will remain constant over time.
TreeSet: This class implements the Set interface that uses a tree for storage. Objects are stored in a sorted and ascending order. Access and retrieval times are quite fast, which makes TreeSet an excellent choice when storing large amounts of sorted information that must be found quickly.
LinkedHashSet: This class is a Hash table and linked list implementation of the Set interface, with predictable iteration order. It maintains a doubly-linked list running through all of its entries.
The Role of equals() and hashCode()
Java Set uses the equals() and hashCode() methods to ensure that no duplicate values are stored.
- equals(): This method checks if two objects are equal. In the context of a Set, it’s used to check if an element already exists before adding a new one.
hashCode(): This method returns an integer hash code representing the object. Java Set uses this value for faster lookups and operations like add() or remove().
Here’s an example of how equals() and hashCode() work:
Set<String> set = new HashSet<>();
set.add('Java');
boolean isEqual = 'Java'.equals('Java');
int hashCode = 'Java'.hashCode();
System.out.println(isEqual);
System.out.println(hashCode);
// Output:
// true
// 3254818
In this code, we’re adding ‘Java’ to our Set. We then check if ‘Java’ equals ‘Java’ using the equals() method, which returns true. We also get the hash code of ‘Java’ using the hashCode() method.
Understanding these fundamentals will give you a solid foundation to effectively use and navigate Java Set.
The Relevance of Java Set in Larger Projects
Java Set isn’t just a tool for handling non-duplicate elements; it’s an integral part of larger projects, including data processing and web development.
Java Set in Data Processing
In data processing, Sets are often used to identify unique data points. For example, if you’re processing a large dataset and want to eliminate any duplicate entries, a Set can do that efficiently.
Set<DataPoint> uniqueDataPoints = new HashSet<>(dataPoints);
System.out.println('Number of unique data points: ' + uniqueDataPoints.size());
In this code, we’re creating a Set from a list of data points. The Set automatically removes any duplicates, leaving us with only the unique data points.
Java Set in Web Development
In web development, Sets can be used for tasks like session management or tracking unique user visits. Here’s an example:
Set<String> uniqueVisitors = new HashSet<>(visitorIds);
System.out.println('Number of unique visitors: ' + uniqueVisitors.size());
In this code, we’re tracking unique visitors to a website by their IDs. By storing the IDs in a Set, we can quickly and easily get the number of unique visitors.
Exploring Related Concepts
To further your understanding of Java Set and its applications, we recommend exploring related concepts like the Java Collections Framework, Generics, and Multithreading. These topics will give you a broader view of Java and its capabilities.
Further Resources for Mastering Java Set
To deepen your knowledge and proficiency with Java Set, consider exploring the following resources:
- IOFlood’s Java Data Structures Article – Learn the benefits and trade-offs of the data structures in Java programming.
Understanding Java TreeMap – Learn about TreeMap’s features like sorted key-value pairs and search operations.
Exploring Deque in Java – Learn about Deque implementations like ArrayDeque and LinkedList.
Oracle’s Java Collections Tutorial – The official Oracle tutorial on Java Collections.
Baeldung’s Guide on Java Set explains how to operate on Java Set.
Java Code Geeks’ Java Set Examples contains practical examples of Java Set usage.
These resources provide in-depth tutorials and examples that can help you become more proficient with Java Set.
Wrapping Up: Java Set
In this comprehensive guide, we’ve journeyed through the intricacies of Java Set, a powerful tool for storing unique elements in Java.
We began with the basics, learning how to create a Set, add elements to it, and iterate over it. We then ventured into more advanced territory, exploring complex operations like removing duplicates, checking if an element exists, and comparing two Sets. Along the way, we tackled common challenges you might face when using Java Set, such as handling null values and concurrency issues, and provided solutions to help you overcome these hurdles.
We also looked at alternative data structures in Java, such as List and Map, giving you a sense of the broader landscape of tools for handling collections of elements. Here’s a quick comparison of these data structures:
Data Structure | Allows Duplicates | Maintains Insertion Order |
---|---|---|
Set | No | No |
List | Yes | Yes |
Map | No (for keys) | No |
Whether you’re just starting out with Java Set or you needed a handy reference, we hope this guide has helped you understand how to use Java Set effectively.
Mastering Java Set is a valuable skill for any Java developer, and with this guide, you’re well on your way to becoming proficient in handling Sets. Happy coding!