Core Java Interview Questions: Concepts You Should Know
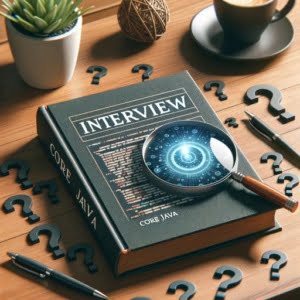
Are you gearing up for a job interview that involves core Java? You’re not alone. Many developers find themselves in your shoes, but we’re here to help.
Think of core Java as the fundamental building blocks of Java programming – it’s the core of the language, and understanding it is crucial for any Java developer.
This guide will provide you with a comprehensive list of core Java interview questions and their answers. We’ll start by understanding what core Java is and why it’s so important in interviews. We’ll cover everything from basic to advanced questions, and provide you with model answers and explanations.
So, let’s dive in and start mastering these core Java interview questions!
TL;DR: What are Some Common Core Java Interview Questions?
Here are a few examples of core Java interview questions:
- What is the difference between an abstract class and an interface in Java?
- Can you explain the concept of inheritance in Java?
- How does exception handling work in Java?
Let’s take a quick look at how you might answer one of these questions:
public abstract class AbstractClass {
public abstract void abstractMethod();
}
public interface Interface {
void interfaceMethod();
}
In this example, we have an abstract class and an interface. The key difference between them is that an abstract class can have both abstract (method without a body) and non-abstract methods (method with a body), while an interface can only have abstract methods.
This is just a sneak peek. We’ll delve into these questions and many more in the following sections. So, buckle up and read on to prepare for your interview.
Table of Contents
Core Java Basics: Beginner Level Questions
When starting with core Java interview questions, it’s essential to have a firm grasp on the basics. Here, we’ll discuss questions related to Java syntax, data types, and control structures. For each question, we’ll provide a model answer and an explanation.
Question 1: What are the main data types in Java?
public class Main {
public static void main(String[] args) {
int myNum = 5; // Integer (whole number)
float myFloatNum = 5.99f; // Floating point number
char myLetter = 'D'; // Character
boolean myBool = true; // Boolean
String myText = "Hello"; // String
System.out.println(myNum);
System.out.println(myFloatNum);
System.out.println(myLetter);
System.out.println(myBool);
System.out.println(myText);
}
}
/* Output:
5
5.99
D
true
Hello
*/
In Java, there are eight main data types: byte, short, int, long, float, double, boolean, and char. The code snippet above demonstrates how you can declare and initialize some of these data types. It also prints out their values.
Question 2: What is the difference between '=='
and ‘equals()’ in Java?
public class Main {
public static void main(String[] args) {
String str1 = new String("Hello");
String str2 = new String("Hello");
System.out.println(str1 == str2);
System.out.println(str1.equals(str2));
}
}
/* Output:
false
true
*/
In Java, '=='
checks if two references point to the same object, while ‘equals()’ checks if the content of two objects are equal. In the example above, str1 and str2 point to two different objects, so '=='
returns false. However, the content of both objects is the same (“Hello”), so ‘equals()’ returns true.
Core Java: Intermediate Level Questions
As we delve deeper into core Java, we encounter more complex concepts like object-oriented programming, exception handling, and multithreading. Let’s explore some intermediate level interview questions on these topics.
Question 1: Explain the Concept of Inheritance in Java
class Animal {
public void eat() {
System.out.println("This animal eats");
}
}
class Dog extends Animal {
public void bark() {
System.out.println("The dog barks");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat();
dog.bark();
}
}
/* Output:
This animal eats
The dog barks
*/
In Java, inheritance is a mechanism where you can to derive a class from another class for a hierarchy of classes that share a set of attributes and methods. In the example above, the Dog
class inherits the eat
method from the Animal
class.
Question 2: How Does Exception Handling Work in Java?
public class Main {
public static void main(String[] args) {
try {
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (Exception e) {
System.out.println("Something went wrong.");
} finally {
System.out.println("The 'try catch' is finished.");
}
}
}
/* Output:
Something went wrong.
The 'try catch' is finished.
*/
Exception handling in Java is managed by five keywords: try, catch, finally, throw, and throws. In the example above, the try block contains a section of code that might generate an exception. The catch block contains the process of handling the exception. Finally, the finally block will execute whether an exception is thrown or not.
Question 3: What is Multithreading in Java?
class RunnableDemo implements Runnable {
private Thread t;
private String threadName;
RunnableDemo(String name) {
threadName = name;
System.out.println("Creating " + threadName);
}
public void run() {
System.out.println("Running " + threadName);
}
public void start() {
System.out.println("Starting " + threadName);
if (t == null) {
t = new Thread(this, threadName);
t.start();
}
}
}
public class TestThread {
public static void main(String args[]) {
RunnableDemo R1 = new RunnableDemo("Thread-1");
R1.start();
RunnableDemo R2 = new RunnableDemo("Thread-2");
R2.start();
}
}
/* Output:
Creating Thread-1
Starting Thread-1
Running Thread-1
Creating Thread-2
Starting Thread-2
Running Thread-2
*/
Multithreading in Java is a feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU. In the example above, two threads (Thread-1
and Thread-2
) are created and executed concurrently.
Advanced Core Java: Expert Level Questions
Now, let’s tackle some advanced core Java interview questions. These questions will cover topics such as Java libraries, frameworks, and performance optimization. As before, we’ll provide a model answer and an explanation for each question.
Question 1: What are Java Libraries and How are They Used?
Java libraries are a collection of precompiled routines that a program can use. The routines, sometimes called modules, are stored in object format. Libraries are particularly useful for storing frequently used routines because you do not need to explicitly link them to every program that uses them.
import java.util.*;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(5);
list.add(10);
list.add(15);
System.out.println(list);
}
}
/* Output:
[5, 10, 15]
*/
In the example above, we use the ArrayList
class from the java.util
library to create a list of integers.
Question 2: What is the Spring Framework in Java?
The Spring Framework is a powerful lightweight application development framework used for Enterprise Java (JEE). The core features of the Spring Framework can be used in developing any Java application.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In the example above, we have a basic Spring Boot application. The @SpringBootApplication
annotation is often placed on your main class, and it implicitly defines a base “search package” for certain items.
Question 3: How Can You Optimize Java Performance?
Performance optimization in Java is a wide topic. Some common strategies include using the right data structures, optimizing memory usage, and using multithreading.
public class Main {
public static void main(String[] args) {
long startTime = System.nanoTime();
// Code to be timed here
long endTime = System.nanoTime();
long duration = (endTime - startTime);
System.out.println("Execution time in nanoseconds: " + duration);
}
}
In the example above, we measure the execution time of a code snippet. This is a simple yet effective way to identify bottlenecks and optimize performance.
Core Java interviews can often present some difficult questions. Let’s discuss how to handle these, along with common mistakes to avoid, and tips for explaining your thought process during the interview.
Handling Tricky Questions
public class Main {
public static void main(String[] args) {
try {
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (Exception e) {
System.out.println("Caught an exception: " + e);
}
}
}
/* Output:
Caught an exception: java.lang.ArrayIndexOutOfBoundsException: Index 10 out of bounds for length 3
*/
In the example above, the code is trying to access an array element that does not exist. This could be a tricky interview question, but the key is to handle it calmly. You can explain that the code is trying to access an index outside the array’s bounds, which throws an ArrayIndexOutOfBoundsException
. This exception is then caught and handled in the catch block.
Common Mistakes to Avoid
public class Main {
public static void main(String[] args) {
int x = 10;
int y = 0;
try {
int z = x / y;
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e);
}
}
}
/* Output:
Caught an exception: java.lang.ArithmeticException: / by zero
*/
In the example above, the code is trying to divide a number by zero, which is a common mistake made by many developers. This will throw an ArithmeticException
. The exception is caught and handled in the catch block.
Explaining Your Thought Process
During an interview, it’s important to explain your thought process while solving problems. This helps the interviewer understand how you approach problems, your analytical skills, and your communication ability. Be sure to explain your code, why you wrote it that way, and discuss any trade-offs or alternative solutions you considered.
Understanding Core Java Fundamentals
To fully grasp the core Java interview questions, it’s crucial to understand the fundamentals of core Java. Here, we’ll dive into details about object-oriented programming, Java syntax, and the Java runtime environment.
Object-Oriented Programming in Java
Java is an object-oriented programming language. This means it revolves around the concept of ‘objects’, which are essentially instances of classes. These objects have states and behaviors, defined by their class.
public class Dog {
String breed;
int age;
String color;
void barking() {
// code here
}
void hungry() {
// code here
}
void sleeping() {
// code here
}
}
In the example above, Dog
is a class that has three fields (breed, age, and color) and three methods (barking, hungry, and sleeping). An object of this Dog
class can be created with specific states and behaviors.
Java Syntax
Java syntax is the set of rules defining how a Java program is written and interpreted.
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
/* Output:
Hello, World!
*/
In the example above, public class Main
defines a public class named Main. public static void main(String[] args)
is the main method where the program starts. System.out.println("Hello, World!");
prints the string “Hello, World!” to the console.
The Java Runtime Environment
The Java Runtime Environment (JRE) is a software layer that runs Java programs. It includes the Java Virtual Machine (JVM), the Java Core Classes, and supporting files.
java Main
In the example above, we use the java
command (part of the JRE) to run the Main class. The java
command loads the JVM, loads the Main class into the JVM, and starts executing the main method of the Main class.
Core Java within the Larger Java Landscape
Understanding core Java is just the beginning; it’s the foundation upon which the rest of your Java knowledge is built. So, let’s discuss the relevance of core Java in the broader context of Java programming and software development.
Core Java and Its Role in Software Development
Core Java forms the base of most Java programming. It includes fundamental concepts like loops, data types, variables, and arrays. These basics are essential in creating more complex applications, whether you’re developing enterprise software or Android apps.
Preparing for a Java Interview
When preparing for a Java interview, it’s crucial to practice coding, study effectively, and know how to present yourself during the interview. Here are some tips:
- Practice coding: Use online platforms to solve problems using Java. This helps you understand the language’s nuances and improves your problem-solving skills.
Study effectively: Don’t just memorize concepts; understand them. Be able to explain why a particular solution works and how you arrived at it.
Present yourself: During the interview, communicate your thought process clearly. Even if you don’t arrive at the correct solution, showing how you approach problems can impress interviewers.
Further Resources for Mastering Core Java
To continue your journey in mastering core Java, here are some resources that can help:
- Beginner’s Guide: What Java Is Used For – Understand the versatility of Java across different industries.
Java Interview Prep Tips – Master preparing for Java interviews with comprehensive knowledge and practice.
Essential Java Concepts – Master core Java skills for building robust and scalable applications.
Oracle’s Java Tutorials cover many aspects of Java programming.
Java Code Geeks offers a vast collection of articles, tutorials, and code examples about Java.
Baeldung’s Guide to Java offers a well-structured approach to learning Java, from beginner to advanced topics.
Wrapping Up: Core Java Interview Questions
In this comprehensive guide, we’ve journeyed through the world of core Java, providing you with a robust list of interview questions, complete with model answers and explanations. From the basics to advanced topics, we’ve covered a wide range of concepts integral to any Java developer preparing for an interview.
We began with a look at basic concepts, such as Java syntax, data types, and control structures. Then, we moved on to intermediate-level topics, including object-oriented programming, exception handling, and multithreading. Finally, we delved into advanced topics, such as Java libraries, frameworks, and performance optimization.
Along the way, we provided practical advice on handling tricky questions, avoiding common mistakes, and effectively explaining your thought process during an interview. We also discussed the fundamentals of core Java and its relevance in the broader context of Java programming and software development.
Whether you’re a beginner just starting out with core Java or an experienced developer looking to level up your interview skills, we hope this guide has given you a deeper understanding of core Java and its applications.
With its balance of detailed explanations, practical examples, and helpful tips, this guide is a powerful tool for any aspiring Java developer. Now, you’re well-equipped to ace your next core Java interview. Happy coding!