Acing Your Java Interview: Questions and Answers Guide
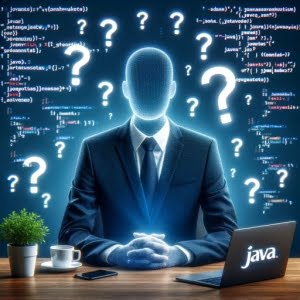
Are you gearing up for a Java interview and feeling a bit lost? You’re not alone. Many developers find themselves in the same boat when it comes to preparing for Java interviews. But just like a well-prepared student acing an exam, you can ace your Java interview with the right preparation.
Think of Java interview questions as a stepping stone – a stepping stone that can lead you to your dream job. These questions are a way for your potential employers to assess your understanding of Java, its syntax, and its real-world applications.
This guide will provide you with a comprehensive list of Java interview questions, categorized by difficulty level, along with detailed answers and explanations. We’ll cover everything from basic Java concepts to more advanced topics, providing you with the tools you need to excel in your Java interview.
So, let’s dive in and start mastering Java interview questions!
TL;DR: What are Some Common Java Interview Questions?
Some common Java interview questions include:
'Explain the concept of object-oriented programming and how it's implemented in Java.'
,'What is a Java Exception and how do you handle it?'
, or'What is the difference between an interface and an abstract class in Java?'
. We will show you how to answer the last question below.
Here’s a simple example of the difference between an interface and an abstract class in Java:
interface Animal {
void eat();
}
abstract class Mammal {
abstract void breathe();
}
# Output:
# These are examples of an interface and an abstract class in Java. An interface, like Animal, is a completely 'abstract class' that is used to group related methods with empty bodies. An abstract class, like Mammal, is a class that cannot be instantiated and is often used as a base class for other classes.
In this example, we’ve defined an interface Animal
with a method eat()
and an abstract class Mammal
with a method breathe()
. This is a basic illustration of the difference between an interface and an abstract class in Java.
But Java’s interview questions can be more complex and wide-ranging. Continue reading for more detailed examples and comprehensive answers.
Table of Contents
- Basic Java Interview Questions: A Beginner’s Guide
- Intermediate Java Interview Questions: Upping Your Game
- Advanced Java Interview Questions: Expert-Level Insights
- Java Interview Tips: Your Path to Success
- Common Mistakes to Avoid
- Understanding Fundamental Java Concepts
- Beyond the Interview: Continuing Your Java Journey
- Wrapping Up: Mastering Java Interview Questions
Basic Java Interview Questions: A Beginner’s Guide
What is the difference between JDK, JRE, and JVM?
// Java Development Kit (JDK) is a software development environment used for developing Java applications and applets. It includes the Java Runtime Environment (JRE), an interpreter/loader (java), a compiler (javac), an archiver (jar), a documentation generator (Javadoc), etc.
// Java Runtime Environment (JRE) is an implementation of the Java Virtual Machine which executes Java programs. It includes class libraries and other supporting files that JVM uses at runtime.
// Java Virtual Machine (JVM) is an abstract machine. It is a specification that provides a runtime environment in which Java bytecode can be executed.
What is a Class in Java?
// A class in Java is a blueprint which includes all your data. A class contains fields (variables) and methods to describe the behavior of an object.
class Car {
String color;
String model;
void start() {
System.out.println("Car started");
}
}
# Output:
# This is a simple class named 'Car' with two fields ('color' and 'model') and a method 'start'.
In the above example, Car
is a class that includes two fields color
and model
, and a method start()
. When the start()
method is called, it prints out ‘Car started’.
What is an Object in Java?
// An object is an instance of a class. You can create any number of objects for a class. Objects have the state and behavior.
Car myCar = new Car();
myCar.color = "Red";
myCar.model = "Mustang";
myCar.start();
# Output:
# 'Car started'
In this example, myCar
is an object of the class Car
. We have assigned the color ‘Red’ and model ‘Mustang’ to it and then we called the start()
method which printed ‘Car started’.
Intermediate Java Interview Questions: Upping Your Game
What is Multithreading in Java?
Multithreading in Java is a feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU.
class MultithreadingDemo extends Thread {
public void run() {
try {
System.out.println("Thread " + Thread.currentThread().getId() + " is running");
}
catch (Exception e) {
System.out.println("Exception is caught");
}
}
}
public class Multithread {
public static void main(String[] args) {
int n = 8; // Number of threads
for (int i = 0; i < n; i++) {
MultithreadingDemo object = new MultithreadingDemo();
object.start();
}
}
}
# Output:
# 'Thread [thread id] is running'
In the above example, we created 8 threads. Each thread starts a new task and runs concurrently, maximizing CPU utilization.
What is Exception Handling in Java?
Exception handling in Java is a powerful mechanism that is used to handle runtime errors, maintain normal flow of the application, and avoid program termination.
public class ExceptionHandlingDemo {
public static void main(String[] args) {
try {
int divideByZero = 5 / 0;
System.out.println("Let's divide!");
}
catch (ArithmeticException e) {
System.out.println("Can't divide by zero!");
}
}
}
# Output:
# 'Can't divide by zero!'
In this example, we try to divide a number by zero, which is not allowed. This would normally terminate the program, but with exception handling, we can catch the error and print a friendly message instead, allowing the program to continue running.
Advanced Java Interview Questions: Expert-Level Insights
What is Garbage Collection in Java?
Java Garbage Collection is a process of looking at heap memory, identifying which objects are in use and which are not, and deleting the unused objects.
public class GarbageCollectionDemo {
public void finalize() {System.out.println("Object is garbage collected");}
public static void main(String args[]) {
GarbageCollectionDemo s1 = new GarbageCollectionDemo();
GarbageCollectionDemo s2 = new GarbageCollectionDemo();
s1 = null;
s2 = null;
System.gc();
}
}
# Output:
# 'Object is garbage collected'
# 'Object is garbage collected'
In this example, we create two objects s1
and s2
. By setting them to null
, they become eligible for garbage collection. The System.gc()
method is used to invoke the garbage collector to perform cleanup processing.
What are Java Annotations?
Java Annotations provide information about the code and they have no direct effect on the code they annotate.
@Override
public String toString() {
return "This is a string.";
}
# Output:
# This is the usage of @Override annotation. It tells the compiler that the following method overrides a method of its superclass.
In the above example, @Override
is an annotation which indicates that the following method overrides a method of its superclass.
What is Reflection in Java?
Java Reflection is an API which is used to examine or modify the behavior of methods, classes, interfaces at runtime.
public class ReflectionDemo {
public static void main(String[] args) throws Exception {
Class<?> c = Class.forName("java.lang.String");
System.out.println("Class Name: " + c.getName());
}
}
# Output:
# 'Class Name: java.lang.String'
In this example, we’re using reflection to get the class name of java.lang.String
at runtime.
Java Interview Tips: Your Path to Success
Understand the Basics
Before delving into complex topics, ensure you have a solid understanding of Java basics. This includes understanding data types, operators, control statements, classes, objects, and methods. Having a strong foundation will make it easier for you to tackle more complex questions.
Practice Coding
Java interview questions often involve writing code. Make sure you’re comfortable writing code by hand, as well as on a computer. This can involve everything from writing a simple ‘Hello, World!’ program to more complex data structures and algorithms.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# 'Hello, World!'
In this simple example, we’re just printing ‘Hello, World!’ to the console. But the more you practice, the more comfortable you’ll get with Java syntax and problem-solving.
Brush Up on Core Concepts
Make sure you’re familiar with core Java concepts like object-oriented programming, exception handling, multithreading, and more. These concepts are often the focus of Java interview questions.
Be Prepared for Tricky Questions
Java interview questions can sometimes be tricky. They might ask you about the nuances of the language or how certain keywords work. Make sure you understand the ins and outs of Java and can handle such questions.
Common Mistakes to Avoid
Not Understanding the Question
Before you start answering, make sure you fully understand the question. Don’t hesitate to ask for clarification if something is unclear.
Rushing Through Your Answer
Take your time when answering. Make sure you explain your thought process and don’t rush through your code. It’s better to be slow and thorough than fast and incorrect.
Ignoring Best Practices
When writing code during an interview, don’t forget about best practices. This includes things like proper indentation, variable naming, and using comments to explain your code.
// Good practice
int numberOfCats = 5;
// Bad practice
int n = 5;
In the above example, numberOfCats
is a much more descriptive variable name than n
. It makes your code easier to understand, which is crucial in an interview setting.
Understanding Fundamental Java Concepts
Java and Object-Oriented Programming
Java is an object-oriented programming language, which means it revolves around the concept of ‘objects’. These objects are instances of classes, which are essentially user-defined data types.
// Here's a simple class definition and object creation
class Dog {
String breed;
String size;
int age;
String color;
}
Dog myDog = new Dog();
# Output:
# This creates a new Dog object named 'myDog'.
In this example, we define a Dog
class with four attributes: breed
, size
, age
, and color
. Then we create an object myDog
of the Dog
class.
The Role of Java Virtual Machine (JVM)
Java’s platform independence is made possible by the Java Virtual Machine (JVM). JVM is a part of the Java Runtime Environment (JRE) that converts Java bytecode into machine language.
The Importance of Java’s Garbage Collection
Java’s garbage collection is a form of automatic memory management. It reclaims the runtime unused memory automatically. This process minimizes the risk of memory leaks, which can cause your application to consume more and more memory – potentially leading to a system slowdown or crash.
Java’s Exception Handling
Exception handling in Java is a powerful mechanism that allows you to handle runtime errors, maintain the normal flow of the application, and avoid program termination. It provides a meaningful message about the error, making it easier to debug the issue.
try {
// code that may raise an exception
} catch (ExceptionClass e) {
// code to handle the exception
}
# Output:
# This is a simple try-catch block which is used to handle exceptions.
In the above example, the code that may raise an exception is placed inside the try
block, and the handling of the exception is done in the catch
block.
These are just a few fundamental Java concepts that are commonly asked about in interviews. Having a strong understanding of these concepts will make it easier for you to answer related interview questions effectively.
Beyond the Interview: Continuing Your Java Journey
Books for Further Reading
Books are a great way to delve deeper into Java. They can provide comprehensive coverage of topics, from basic to advanced levels. Here are a few recommendations:
- ‘Head First Java’ by Kathy Sierra and Bert Bates
- ‘Effective Java’ by Joshua Bloch
- ‘Java: The Complete Reference’ by Herbert Schildt
Online Courses for Skill Enhancement
Online courses can provide structured learning paths and often include hands-on projects. Here are a few platforms offering high-quality Java courses:
- Codecademy’s Learn Java
- Coursera’s Java Programming and Software Engineering Fundamentals
- Udemy’s Java Programming Masterclass
Practice Problems for Mastery
Solving practice problems can help reinforce your understanding of Java concepts. Here are a few websites offering Java practice problems:
Further Resources for Java Interview Mastery
To further your understanding and practice for Java interviews, check out these resources:
- IOFlood’s Article: Exploring Java’s Uses – Explore Java’s role in enterprise-level software solutions.
Java Comments: Usage Guide – Understand the importance of comments in Java for code documentation and readability
Common Core Java Interview Questions tackles questions on classes, objects, inheritance, polymorphism, and abstraction
Java Code Geeks’ Java Interview Questions contains a comprehensive question list.
Educative’s Grokking the Java Interview focuses on coding patterns commonly seen in interviews.
Must-Do Coding Questions for Companies like Amazon, Microsoft, Adobe can help you ace interviews in top tech companies.
Remember, the key to mastering Java interview questions is understanding the concepts and practicing regularly. Keep learning and coding, and you’ll be well-prepared for your next Java interview.
Wrapping Up: Mastering Java Interview Questions
In this comprehensive guide, we’ve explored the vast landscape of Java interview questions, providing a detailed walkthrough to help you ace your next Java interview.
We started with the basics, discussing fundamental Java concepts, and how they are often framed in interview questions. We then went a step further, exploring intermediate and advanced Java topics, providing you with a plethora of examples and explanations to help you understand and answer these complex questions.
Along the way, we provided tips on how to approach these questions, common mistakes to avoid, and how to effectively prepare for a Java interview. We also provided additional resources for further learning and practice, to help you continue your journey beyond the interview.
Here’s a quick recap of the different levels of Java interview questions we’ve discussed:
Level | Examples of Topics Covered |
---|---|
Basic | JDK, JRE, JVM, Classes, Objects |
Intermediate | Multithreading, Exception Handling |
Advanced | Garbage Collection, Annotations, Reflection |
Whether you’re just starting out with Java or you’re an experienced developer preparing for your next interview, we hope this guide has given you a deeper understanding of Java interview questions and how to tackle them.
With the knowledge of Java fundamentals, practice with coding problems, and the understanding of how to approach Java interview questions, you’re now well-equipped to ace your next Java interview. Happy coding!