Java’s JsonObject Class: A Detailed Exploration
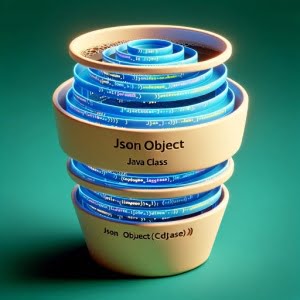
Ever felt overwhelmed by the JsonObject Java Class? You’re not alone. Many developers find themselves puzzled when it comes to handling JSON data in Java. Think of the JsonObject class as a digital librarian – it helps you organize and access data in your Java applications.
The JsonObject class is a part of the javax.json package in Java, and it’s a powerful tool for creating, reading, and manipulating JSON data. It’s like a key that unlocks the power of JSON in your Java applications.
In this guide, we’ll walk you through the process of mastering the JsonObject Java Class, from basic usage to advanced techniques. We’ll cover everything from creating and reading JSON data, handling complex JSON structures, to troubleshooting common issues and exploring alternative approaches.
So, let’s dive in and start mastering the JsonObject Java Class!
TL;DR: What is the JsonObject Java Class?
The
JsonObject Java Class
is a class in Java that represents a JSON object, instantiated with the simple syntax,JsonObject jsonObject = Json.createObjectBuilder()
. It’s part of thejavax.json
package and is used to create, read, and manipulate JSON data in Java. For example:
JsonObject jsonObject = Json.createObjectBuilder().add('name', 'John').build();
In this example, we create a JsonObject instance named jsonObject
using the createObjectBuilder
method from the Json
class. We then add a key-value pair to this object with the key ‘name’ and the value ‘John’. The build
method finalizes the creation of the JsonObject.
This is just a basic way to use the JsonObject Java Class, but there’s much more to learn about creating and manipulating JSON data in Java. Continue reading for more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with JsonObject Java Class
- Handling Complex JSON with JsonObject
- Exploring Alternatives: Gson and Jackson Libraries
- Troubleshooting JsonObject: Common Issues and Solutions
- Understanding JSON and the javax.json Package
- Expanding Your Knowledge: JsonObject in Web Development and RESTful APIs
- Wrapping Up: JsonObject Java Class
Getting Started with JsonObject Java Class
Creating a JsonObject
The JsonObject Java Class allows you to create JSON objects in a straightforward manner. Here’s a simple example:
import javax.json.Json;
import javax.json.JsonObject;
public class Main {
public static void main(String[] args) {
JsonObject jsonObject = Json.createObjectBuilder()
.add("name", "John")
.add("age", 30)
.build();
System.out.println(jsonObject);
}
}
# Output:
# {"name":"John","age":30}
In this example, we first import the necessary classes from the javax.json package. We then use the createObjectBuilder
method from the Json
class to start creating a JsonObject. We add two key-value pairs: ‘name’ with the value ‘John’ and ‘age’ with the value 30. Finally, we build the JsonObject and print it.
Reading a JsonObject
Reading data from a JsonObject is just as simple. Let’s use the JsonObject we created in the previous example:
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
System.out.println("Name: " + name + ", Age: " + age);
# Output:
# Name: John, Age: 30
We use the getString
and getInt
methods from the JsonObject class to read the ‘name’ and ‘age’ values from the JsonObject. We then print these values.
Manipulating a JsonObject
Unfortunately, JsonObject instances are immutable, meaning you cannot change them once they are created. However, you can create a new JsonObject based on an existing one with some modifications. Here’s how you can do it:
JsonObject updatedJsonObject = Json.createObjectBuilder(jsonObject)
.add("name", "Jane")
.build();
System.out.println(updatedJsonObject);
# Output:
# {"name":"Jane","age":30}
In this example, we create a new JsonObject based on the existing one but with the ‘name’ value changed to ‘Jane’. We then print the updated JsonObject.
The JsonObject Java Class provides a simple and intuitive way to handle JSON data in Java. However, it’s important to note that JsonObject instances are immutable. This means you cannot change them once they are created, which can be a potential pitfall if you need to modify JSON data frequently.
Handling Complex JSON with JsonObject
Working with Nested JSON Objects
As your data grows more complex, you may find yourself dealing with nested JSON objects. The JsonObject class can handle this with ease. Let’s consider an example where a person has an address which is represented as a nested JSON object:
import javax.json.Json;
import javax.json.JsonObject;
public class Main {
public static void main(String[] args) {
JsonObject address = Json.createObjectBuilder()
.add("street", "123 Main St")
.add("city", "Springfield")
.add("zip", "12345")
.build();
JsonObject person = Json.createObjectBuilder()
.add("name", "John")
.add("age", 30)
.add("address", address)
.build();
System.out.println(person);
}
}
# Output:
# {"name":"John","age":30,"address":{"street":"123 Main St","city":"Springfield","zip":"12345"}}
In this example, we first create a JsonObject for the address. We then include this address object in the JsonObject for the person. The result is a JsonObject with a nested JsonObject.
Dealing with JSON Arrays
The JsonObject class also allows you to work with JSON arrays. Here’s an example where a person has multiple phone numbers, represented as a JSON array:
import javax.json.Json;
import javax.json.JsonArray;
import javax.json.JsonObject;
public class Main {
public static void main(String[] args) {
JsonArray phoneNumbers = Json.createArrayBuilder()
.add("123-456-7890")
.add("098-765-4321")
.build();
JsonObject person = Json.createObjectBuilder()
.add("name", "John")
.add("age", 30)
.add("phoneNumbers", phoneNumbers)
.build();
System.out.println(person);
}
}
# Output:
# {"name":"John","age":30,"phoneNumbers":["123-456-7890","098-765-4321"]}
In this example, we first create a JsonArray for the phone numbers using the createArrayBuilder
method from the Json
class. We then include this array in the JsonObject for the person.
The JsonObject class provides a powerful way to handle complex JSON data structures in Java. However, it’s important to understand the differences between JSON objects and arrays, and to use the appropriate methods for each. By mastering these techniques, you can handle any JSON data that comes your way.
Exploring Alternatives: Gson and Jackson Libraries
While the JsonObject Java Class is a powerful tool for handling JSON data in Java, there are alternative approaches that offer additional functionality. Two popular alternatives are the Gson library by Google and the Jackson library.
The Gson Approach
The Gson library offers a comprehensive and intuitive framework for converting Java objects to JSON and vice versa. Here’s an example of how you can use Gson to create a JSON object:
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
Person person = new Person();
person.setName("John");
person.setAge(30);
String json = gson.toJson(person);
System.out.println(json);
}
}
# Output:
# {"name":"John","age":30}
In this example, we first create a Gson object. We then create a Person object and set its properties. Finally, we use the toJson
method from the Gson class to convert the Person object to a JSON string.
The Jackson Approach
The Jackson library is another powerful tool for handling JSON data in Java. It offers extensive features and is known for its performance and flexibility. Here’s an example of how you can use Jackson to create a JSON object:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws Exception {
ObjectMapper mapper = new ObjectMapper();
Person person = new Person();
person.setName("John");
person.setAge(30);
String json = mapper.writeValueAsString(person);
System.out.println(json);
}
}
# Output:
# {"name":"John","age":30}
In this example, we first create an ObjectMapper object. We then create a Person object and set its properties. Finally, we use the writeValueAsString
method from the ObjectMapper class to convert the Person object to a JSON string.
Both Gson and Jackson offer powerful features for handling JSON data in Java. However, they each have their strengths and weaknesses. Gson is known for its simplicity and ease of use, while Jackson is known for its performance and flexibility. Your choice between the two will depend on your specific needs and preferences.
Library | Advantages | Disadvantages |
---|---|---|
Gson | Simplicity, ease of use | Less features compared to Jackson |
Jackson | Performance, flexibility | Higher learning curve |
In addition to these libraries, there are many other tools and libraries available for handling JSON data in Java. We recommend exploring these options and choosing the one that best fits your needs.
Troubleshooting JsonObject: Common Issues and Solutions
Handling Null Values
One of the common issues when using the JsonObject Java Class is handling null values. If you try to retrieve a value that is not present in the JsonObject, it will throw a NullPointerException
. Here’s how you can handle this situation:
String value = jsonObject.getString("key", "default value");
System.out.println(value);
# Output:
# default value
In this example, we use the getString
method with two parameters: the key and a default value. If the key is not present in the JsonObject, it will return the default value instead of throwing a NullPointerException
.
Dealing with Type Casting Issues
Another common issue is dealing with type casting. If you try to retrieve a value with the wrong type, it will throw a ClassCastException
. To avoid this, you should use the appropriate method for the type of value you are retrieving. For example, if the value is an integer, you should use the getInt
method:
int value = jsonObject.getInt("key");
System.out.println(value);
# Output:
# 123
In this example, we use the getInt
method to retrieve an integer value. If the value was not an integer, it would throw a ClassCastException
.
Using JsonObject in Multithreaded Environments
JsonObject instances are immutable and thread-safe, meaning they can be used safely in multithreaded environments. However, if you need to create or manipulate JSON data frequently, you should consider using a library like Gson or Jackson that offers mutable JSON objects.
Navigating the JsonObject Java Class can be tricky at times, but with these tips and considerations in mind, you’ll be well-equipped to handle any issues that come your way.
Understanding JSON and the javax.json Package
The Role of JSON in Data Exchange
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It’s based on a subset of JavaScript, but it’s language-independent, with parsers available for virtually every programming language, including Java.
JSON is a text format that’s completely language-independent but uses conventions familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
Here’s a simple example of a JSON object:
{
"name": "John",
"age": 30
}
This JSON object represents a person with a name and an age. It’s easy to understand and can be parsed by a machine easily.
The javax.json Package in Java
Java provides a native API for creating, parsing, and manipulating JSON data using the javax.json package. This package contains several key classes and interfaces that you can use to work with JSON data, including:
JsonObject
: Represents a JSON object, a collection of name-value pairs.JsonArray
: Represents a JSON array, an ordered sequence of values.JsonString
,JsonNumber
,JsonBoolean
: Represent JSON values of string, number, and boolean types respectively.Json
: Provides static methods to create JSON objects, arrays, and values.JsonReader
,JsonWriter
: Provide methods to read and write JSON data.
The JsonObject class is a key part of this package, providing a powerful and flexible way to handle JSON objects in Java. By understanding the basics of JSON and the javax.json package, you’ll be well-prepared to handle JSON data in your Java applications.
Expanding Your Knowledge: JsonObject in Web Development and RESTful APIs
JsonObject in Web Development
In the realm of web development, the JsonObject Java Class plays a crucial role. In the client-server architecture, JSON has become the de facto standard for data interchange between the client (browser) and the server. The JsonObject class provides a convenient way for Java web applications to create, read, and manipulate JSON data, which can then be easily sent to or received from a web client.
JsonObject and RESTful APIs
RESTful APIs, which stand for Representational State Transfer APIs, have become increasingly popular in web development. These APIs use HTTP methods to handle data, and they often use JSON as the data format. The JsonObject class is particularly useful when you’re developing or consuming RESTful APIs in Java. It allows you to easily create and parse the JSON data that you send or receive through the API.
Exploring Related Concepts: JSON Parsing and Serialization
Understanding the JsonObject Java Class is just the beginning. There are many related concepts that you may find useful to explore, such as JSON parsing and serialization.
- JSON parsing refers to converting a JSON string into a JsonObject or JsonArray that you can work with in your Java code.
JSON serialization, on the other hand, refers to converting a JsonObject or JsonArray back into a JSON string that can be sent over the network or stored in a file.
By mastering these concepts, you can take your JSON handling skills to the next level.
Further Resources for Mastering JsonObject
To continue your journey in mastering the JsonObject Java Class and related concepts, here are some valuable resources:
- Enhance Your Java Skills with Classes – Java classes demystified for novice developers.
Generating UUIDs in Java – Explore Java’s UUID class for creating universally unique identifiers.
Exploring ObjectMapper Features – Explore Java’s ObjectMapper for converting Java objects to JSON and vice versa seamlessly.
Oracle’s Official Java EE Tutorial provides a comprehensive guide to the JSON Processing API in Java EE.
Baeldung’s Guide to JSON Processing with Jsonp provides details on the Jackson and Jsonp library in Java.
The JSON Processing GitHub Repository provides a wealth of information, including the source code and examples.
Wrapping Up: JsonObject Java Class
In this in-depth guide, we’ve explored the ins and outs of the JsonObject Java Class, a key tool for handling JSON data in Java.
We began with the basics, learning how to create, read, and manipulate JSON data using the JsonObject class. We then delved into more advanced usage scenarios, such as handling complex JSON data structures and dealing with common issues like null values and type casting. Along the way, we provided practical code examples to help you understand and apply these concepts in your own projects.
We also explored alternative approaches to handle JSON data in Java, such as using the Gson and Jackson libraries. These alternatives offer additional features and can be a good fit for certain use cases.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
JsonObject | Part of Java’s standard library, easy to use | Immutable, may require workarounds for complex use cases |
Gson | Comprehensive and intuitive, mutable JSON objects | Less features compared to Jackson |
Jackson | High performance and flexibility, mutable JSON objects | Higher learning curve |
Whether you’re just starting out with the JsonObject Java Class or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. The ability to handle JSON data effectively is a crucial skill in today’s data-driven world, and you’re now well-equipped to do just that. Keep exploring, keep learning, and happy coding!