Java UUID: Your Ultimate Guide to Unique Identifiers
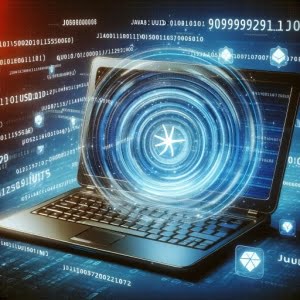
Ever found yourself grappling with UUIDs in Java? You’re not alone. Many developers find the concept of UUIDs a bit challenging. Think of Java’s UUID as a unique fingerprint – a tool that helps identify objects in your code, much like a detective identifies a suspect using their unique fingerprint.
UUIDs are a powerful way to ensure the uniqueness of objects in your Java code, making them extremely popular for a variety of tasks in Java development.
In this guide, we’ll walk you through the process of using UUIDs in Java, from the basics to more advanced techniques. We’ll cover everything from generating simple UUIDs, understanding different versions of UUIDs, to dealing with common issues and even exploring alternative approaches.
Let’s dive in and start mastering UUIDs in Java!
TL;DR: How Do I Generate a UUID in Java?
There are various methods to Generate a UUID in Java, however the
UUID.randomUUID()
method is the most common. Used with the syntax,UUID uuid = UUID.randomUUID();
a unique UUID is generated and returned each time it is ran.
Here’s a simple example:
UUID uuidA = UUID.randomUUID();
UUID uuidB = UUID.randomUUID();
System.out.println(uuidA.equals(uuidB));
# Output:
# false
In this example, we’re using the randomUUID()
method from the UUID
class to generate a random UUID. We then print this UUID to the console. Each time you run this code, a unique UUID will be printed.
This is a basic way to generate a UUID in Java, but there’s much more to learn about UUIDs, their uses, and how to handle them in more complex scenarios. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Generating and Using UUIDs in Java
UUIDs in Java are generated using the UUID
class. This class comes with a variety of methods that can be used to create and manipulate UUIDs. Let’s start with the basics.
Generating a UUID
The most straightforward way to generate a UUID in Java is by using the randomUUID()
method from the UUID
class. This method generates a random UUID, which can then be used as per your requirements. Here’s how you do it:
UUID uuid = UUID.randomUUID();
System.out.println(uuid);
# Output:
# Random UUID each time you run it
In this code block, we’re creating a new UUID using the randomUUID()
method and storing it in the uuid
variable. We then print this UUID to the console. Each time you run this code, a unique UUID will be printed.
Understanding the UUID
Class
The UUID
class in Java is used to represent a UUID. This class comes with a variety of methods that can be used to create and manipulate UUIDs. Some of the most commonly used methods include randomUUID()
, toString()
, version()
, and variant()
.
Advantages and Potential Pitfalls
One of the main advantages of using UUIDs is that they are globally unique identifiers. This means that you can use them to uniquely identify objects, even across different systems.
However, there are also potential pitfalls to be aware of. For instance, generating a UUID is a relatively expensive operation in terms of processing power. Also, UUIDs take up more space than simpler identifiers like integers. Therefore, while they are extremely useful, they should be used judiciously.
Advanced UUID Operations in Java
After mastering the basics of generating UUIDs in Java, it’s time to dive into some more complex operations. In this section, we’ll discuss creating a UUID from a string, comparing UUIDs, and using different versions of UUID.
Creating a UUID from a String
In Java, you can create a UUID from a string using the UUID.fromString()
method. This method accepts a string representation of a UUID and returns a UUID object. Here’s an example:
String uuidString = "123e4567-e89b-12d3-a456-556642440000";
UUID uuid = UUID.fromString(uuidString);
System.out.println(uuid);
# Output:
# 123e4567-e89b-12d3-a456-556642440000
In this code snippet, we’re creating a UUID from a string. The string uuidString
is passed to the fromString()
method, which returns a UUID object. We then print this UUID to the console.
Comparing UUIDs
UUIDs can be compared using the equals()
method. This method compares the calling UUID with the specified UUID for equality. Here’s how you can do it:
UUID uuid1 = UUID.randomUUID();
UUID uuid2 = UUID.randomUUID();
System.out.println(uuid1.equals(uuid2));
# Output:
# false
In this example, we’re generating two random UUIDs and comparing them using the equals()
method. The method returns false
since the two UUIDs are different.
Different Versions of UUID
UUIDs have different versions, each with its own use case. In Java, you can use the version()
method to get the version of a UUID. Here’s an example:
UUID uuid = UUID.randomUUID();
int version = uuid.version();
System.out.println(version);
# Output:
# 4
In this code block, we’re generating a random UUID and retrieving its version using the version()
method. The method returns 4
, which represents a randomly generated UUID.
Exploring Alternative Methods for Unique Identifiers
While UUIDs are a popular choice for generating unique identifiers in Java, they’re not the only option. Let’s explore some alternative approaches you can use to generate unique identifiers in Java, such as using SecureRandom
or third-party libraries.
Using SecureRandom
Java’s SecureRandom
class is another way to generate unique identifiers. This class provides a cryptographically strong random number generator. Here’s an example of how you can use SecureRandom
to generate a unique identifier:
import java.security.SecureRandom;
SecureRandom random = new SecureRandom();
byte[] bytes = new byte[20];
random.nextBytes(bytes);
# Output:
# A unique byte array each time you run it
In this code snippet, we’re creating a new SecureRandom
object and generating a unique byte array. This byte array can then be used as a unique identifier.
Using Third-Party Libraries
There are also third-party libraries available that can generate unique identifiers. For example, the Apache Commons Lang library provides the RandomStringUtils
class, which can be used to generate random strings of any length. Here’s an example:
import org.apache.commons.lang3.RandomStringUtils;
String uniqueID = RandomStringUtils.randomAlphanumeric(20);
System.out.println(uniqueID);
# Output:
# A unique alphanumeric string each time you run it
In this example, we’re using the randomAlphanumeric()
method from the RandomStringUtils
class to generate a unique alphanumeric string. This string can then be used as a unique identifier.
Weighing the Options
Each of these methods has its benefits and drawbacks. While SecureRandom
and third-party libraries may offer more flexibility, they can also be more complex to use and may not provide the same level of uniqueness as UUIDs. Ultimately, the best method to use will depend on your specific use case and requirements.
Troubleshooting Common UUID Issues
While UUIDs are a powerful tool in Java, like all tools, they can present their own challenges. Let’s discuss some common issues you may encounter when using UUIDs in Java, such as performance considerations and collision risks, and how to overcome them.
Performance Considerations
Generating a UUID is a somewhat expensive operation in terms of processing power. If your application needs to generate a large number of UUIDs, this could potentially slow down your application. In such cases, you might want to consider using simpler identifiers or caching UUIDs.
Collision Risks
One of the main advantages of UUIDs is that they are globally unique identifiers. However, there is a very small chance of collision, especially if you are generating a large number of UUIDs. While this risk is extremely small (the chances are 1 in 2^122 for version 4 UUIDs), it’s something to be aware of.
Here’s a simple way to check for collision:
Set<UUID> uuids = new HashSet<>();
for (int i = 0; i < 10000000; i++) {
UUID uuid = UUID.randomUUID();
if (!uuids.add(uuid)) {
System.out.println("Collision detected: " + uuid);
}
}
# Output:
# No output unless a collision is detected
In this code block, we’re generating 10 million UUIDs and storing them in a HashSet
. If a collision occurs, the add()
method will return false
, and we print the colliding UUID.
While UUIDs are an excellent choice for many use cases, it’s essential to understand their potential challenges and how to address them to use them effectively in your Java applications.
Unraveling the Concept of UUID
UUID, short for Universally Unique Identifier, is a 128-bit value used to uniquely identify information in computer systems. The term UUID is often used interchangeably with GUID (Globally Unique Identifier), a term used by Microsoft.
The History of UUID
The concept of UUID was developed as part of the Network Computing System (NCS), and later formalized as part of the Distributed Computing Environment (DCE). The goal was to create identifiers that are unique across space and time, even in the face of systems being created independently without central coordination.
UUIDs in Java and Other Languages
In Java, UUIDs are represented as objects of the UUID
class. This class provides a number of methods for creating and manipulating UUIDs. Java’s implementation of UUIDs adheres to the UUID specification in RFC 4122, which is the standard used by most systems today.
UUID uuid = UUID.randomUUID();
System.out.println(uuid);
# Output:
# A unique UUID each time you run it
In this example, we’re generating a random UUID using the randomUUID()
method of the UUID
class. This method adheres to the version 4 of the UUID standard, which specifies that the UUID should be generated using a random or pseudo-random number.
UUIDs are also used in many other programming languages, including Python, C#, JavaScript, and more. While the specifics of how UUIDs are generated and used can vary from language to language, the underlying concept remains the same.
Understanding the fundamentals of UUIDs can help you appreciate their power and versatility, and enable you to use them more effectively in your Java applications.
UUIDs in Larger Java Projects: Distributed Systems and Databases
UUIDs play a crucial role in larger Java projects, particularly in distributed systems and databases. Their unique nature makes them a perfect fit for identifying records in a database or nodes in a distributed system.
UUIDs in Distributed Systems
In distributed systems, UUIDs can be used to uniquely identify nodes or data. This is particularly useful in situations where you can’t rely on IP addresses or hostnames, which might change over time. Additionally, since UUIDs can be generated independently on each node without any central coordination, they’re a natural choice for distributed systems.
UUIDs in Databases
In databases, UUIDs are often used as primary keys. This can be particularly useful in distributed databases, where it might not be possible to use a simple integer as a primary key. Using UUIDs as primary keys allows records to be uniquely identified, even across different databases or systems.
Related Topics for Further Exploration
If you’re interested in delving deeper into the world of UUIDs and related topics, you might want to explore hashing, encryption, and data structures. Each of these topics can provide further insights into how data can be manipulated and managed in Java.
Further Resources for Mastering UUIDs in Java
- Understanding Java Classes: Step-by-Step Tutorial – Dive deep into Java classes for effective programming.
Exploring Java Random Class – Explore Java’s random class for generating random numbers and sequences.
JSONObject in Java Explained – Understand the role of JsonObject in Java for parsing and manipulating JSON data.
Oracle’s Official Java UUID Documentation provides a comprehensive overview of the
UUID
class and its methods.Java UUID Guide provides a hands-on approach to using Universal Unique Identifiers in Java.
Java UUID Tutorial by javatpoint provides a guide on using UUID in Java.
Wrapping Up: Mastering UUID in Java
In this comprehensive guide, we’ve delved deep into the world of UUIDs in Java, exploring the ins and outs of this powerful tool for generating unique identifiers.
We began with the basics, learning how to generate and use UUIDs in Java. We then progressed to more advanced techniques, such as creating a UUID from a string, comparing UUIDs, and understanding different versions of UUID. Along the way, we tackled common issues that you might encounter when using UUIDs, providing practical solutions to these challenges.
We also explored alternative approaches to generating unique identifiers in Java, such as using SecureRandom
and third-party libraries. Each method has its own advantages and potential pitfalls, and the best choice depends on your specific requirements and use case.
Here’s a quick comparison of the methods we’ve discussed:
Method | Uniqueness | Complexity | Performance |
---|---|---|---|
UUID | High | Moderate | Moderate |
SecureRandom | High | High | High |
Third-Party Libraries | Varies | Varies | Varies |
Whether you’re just starting out with UUIDs in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. Understanding and effectively using UUIDs is a vital skill for any Java developer, and you’re now well-equipped to generate and manipulate these unique identifiers in your own projects.
With the knowledge you’ve gained from this guide, you’re ready to tackle any challenge that involves UUIDs in Java. Happy coding!