Converting a List to Array in Java: A Detailed Walkthrough
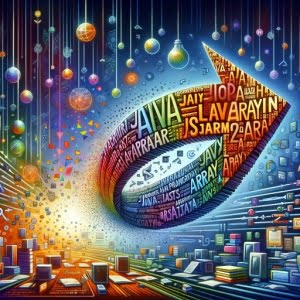
Are you grappling with the task of converting a List to an Array in Java? You’re not alone. This is a common challenge faced by many Java developers, but consider Java as a skilled craftsman, capable of reshaping a List into an Array.
This guide will walk you through the process of converting a List to an Array in Java, from basic to advanced techniques. We’ll cover everything from the simple toArray()
method to handling more complex scenarios, such as dealing with null values and converting a List of custom objects to an Array.
So, let’s dive in and start mastering the conversion of a List to an Array in Java!
TL;DR: How Do I Convert a List to an Array in Java?
Converting a List to an Array in Java is a common task that can be accomplished using the
toArray()
method with the syntax,String[] newArray = listToConvert.toArray(new String[0]);
. This method transforms the elements of a List into an Array.
Here’s a simple example:
List<String> list = new ArrayList<String>();
list.add('Hello');
String[] array = list.toArray(new String[0]);
# Output:
# array: ['Hello']
In this example, we create a List and add a single element ‘Hello’. We then use the toArray()
method to convert this List into an Array. The parameter we pass to toArray(new String[0])
is a new String array with zero elements. This is used as a type hint to Java, telling it what type of array we want to get back.
This is a basic way to convert a List to an Array in Java, but there’s much more to learn about handling more complex scenarios and alternative approaches. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding the Basics: toArray() Method in Java
- Advanced Techniques: Converting Complex Lists to Arrays
- Exploring Alternative Approaches for List to Array Conversion
- Troubleshooting Java List to Array Conversion
- Java’s Core Data Structures: List and Array
- Real-World Applications of Java List to Array Conversion
- Exploring Related Java Concepts
- Wrapping Up: List to Array Conversion
Understanding the Basics: toArray()
Method in Java
The toArray()
method is a built-in method in Java, specifically designed to convert a List to an Array. It’s the most straightforward way to perform this conversion, and is the foundation upon which more advanced techniques are built.
Here’s a basic example of how it works:
List<String> list = new ArrayList<String>();
list.add('Hello');
list.add('World');
String[] array = list.toArray(new String[0]);
# Output:
# array: ['Hello', 'World']
In this example, we first create a List and add two elements: ‘Hello’ and ‘World’. We then use the toArray()
method where we pass a new String array with zero elements as a parameter. This is a type hint to Java, telling it that we want to get back an array of Strings.
The Advantages of toArray()
The toArray()
method is simple and easy to use. It’s a built-in method, which means you don’t need to import or install any additional libraries to use it.
Potential Pitfalls of toArray()
While toArray()
is a powerful tool, it’s not without its limitations. For instance, you need to ensure that the type of the array you’re trying to create matches the type of elements in your List. If they don’t match, Java will throw an ArrayStoreException
. Additionally, if your List contains null
values, these will be transferred to the array, which might not be the desired outcome in some scenarios.
Advanced Techniques: Converting Complex Lists to Arrays
As you gain more experience with Java, you’ll likely encounter scenarios that require more advanced techniques. This might include converting a List of custom objects to an Array, or handling null
values in the List.
Converting a List of Custom Objects to an Array
Let’s imagine you have a List of custom objects, like Book
, and you want to convert this List into an Array. Here’s how you can do it:
List<Book> bookList = new ArrayList<Book>();
bookList.add(new Book('To Kill a Mockingbird', 'Harper Lee'));
bookList.add(new Book('1984', 'George Orwell'));
Book[] bookArray = bookList.toArray(new Book[0]);
# Output:
# bookArray: [Book@1b6d3586, Book@4554617c]
In this example, we first create a List of Book
objects and add two books to it. We then use the toArray()
method to convert this List into an Array of Book
objects. The output represents the memory addresses of the Book
objects in the array.
Handling Null Values in the List
If your List contains null
values, these will be transferred to the array when using the toArray()
method. Here’s an example:
List<String> listWithNulls = new ArrayList<String>();
listWithNulls.add('Hello');
listWithNulls.add(null);
listWithNulls.add('World');
String[] arrayWithNulls = listWithNulls.toArray(new String[0]);
# Output:
# arrayWithNulls: ['Hello', null, 'World']
In this example, the second element in the List is null
, and this null
value is included in the resulting array. Depending on your specific use case, you might need to handle these null
values in a different way, such as filtering them out before the conversion, or replacing them with a default value.
Exploring Alternative Approaches for List to Array Conversion
While the toArray()
method is a great tool for converting a List to an Array in Java, there are other methods you can use, especially if you’re dealing with more complex scenarios or looking for more efficient solutions. Let’s explore some of these alternatives.
Using Java 8 Streams
Java 8 introduced the concept of Streams, which can be used to perform a variety of operations on data sequences, including converting a List to an Array. Here’s an example:
List<String> list = Arrays.asList('Hello', 'World');
String[] array = list.stream().toArray(String[]::new);
# Output:
# array: ['Hello', 'World']
In this code snippet, we first create a List with two elements. We then use a Stream to convert this List into an Array. The toArray()
method from the Stream API is more flexible and powerful than the one from the Collection API, as it can handle more complex data transformations.
Using Third-Party Libraries
There are also several third-party libraries available that provide methods to convert a List to an Array in Java, such as Apache Commons Lang and Guava. These libraries can offer more advanced features and better performance in some cases.
Here’s an example using Apache Commons Lang:
List<String> list = Arrays.asList('Hello', 'World');
String[] array = list.toArray(ArrayUtils.EMPTY_STRING_ARRAY);
# Output:
# array: ['Hello', 'World']
In this example, we use the toArray()
method from Apache Commons Lang, which works similarly to the built-in toArray()
method but can offer better performance for large Lists.
While these alternative approaches can be more powerful or efficient, they also come with their own trade-offs. For instance, using Streams or third-party libraries might make your code harder to understand for beginners, or introduce additional dependencies to your project. As always, you should choose the method that best fits your specific needs and constraints.
Troubleshooting Java List to Array Conversion
During the process of converting a List to an Array in Java, you might encounter some common issues. Understanding these issues and knowing how to solve them is an important part of mastering this task. Let’s take a look at some of these potential problems and their solutions.
Dealing with NullPointerException
A NullPointerException
can occur if you try to convert a null
List to an Array. Here’s an example:
List<String> nullList = null;
String[] array = nullList.toArray(new String[0]);
# Output:
# Exception in thread 'main' java.lang.NullPointerException
In this case, the List nullList
is null
, so when we try to call the toArray()
method on it, Java throws a NullPointerException
. To avoid this, you should always check if your List is null
before trying to convert it to an Array.
Handling ArrayStoreException
An ArrayStoreException
can occur if the type of the elements in the List doesn’t match the type of the Array you’re trying to create. Here’s an example:
List<Integer> integerList = Arrays.asList(1, 2, 3);
String[] array = integerList.toArray(new String[0]);
# Output:
# Exception in thread 'main' java.lang.ArrayStoreException: java.lang.Integer
In this case, the List contains Integer
elements, but we’re trying to create a String
Array. Java can’t convert Integer
to String
automatically, so it throws an ArrayStoreException
. To avoid this, you should ensure that the type of the Array matches the type of the elements in your List.
Remember, understanding common issues and their solutions can help you write more robust and error-free code. Always keep these considerations in mind when converting a List to an Array in Java.
Java’s Core Data Structures: List and Array
To fully grasp the process of converting a Java List to an Array, it’s crucial to understand the basic characteristics and functionalities of these two core data structures.
Understanding Java Lists
In Java, a List is part of the Java Collections Framework and represents an ordered collection (also known as a sequence). Lists can contain duplicate elements, and they also allow random access, which means you can get any element from the list by its index number.
Here’s an example of creating and using a List in Java:
List<String> list = new ArrayList<String>();
list.add('Hello');
list.add('World');
# Output:
# list: ['Hello', 'World']
In this example, we create a List and add two elements to it. We can access these elements by their index, with ‘Hello’ at index 0 and ‘World’ at index 1.
Understanding Java Arrays
An Array in Java is a basic data structure that holds a fixed number of values of a single type. The length of an Array is established when the Array is created, and it can’t be changed.
Here’s an example of creating and using an Array in Java:
String[] array = new String[2];
array[0] = 'Hello';
array[1] = 'World';
# Output:
# array: ['Hello', 'World']
In this example, we create an Array with a length of 2 and add two elements to it. Similar to a List, we can access these elements by their index.
With a solid understanding of Lists and Arrays in Java, you’ll be better equipped to understand the process of converting a List to an Array, and how to handle the various scenarios that can arise during this conversion.
Real-World Applications of Java List to Array Conversion
The process of converting a List to an Array in Java is not just an academic exercise. It has real-world applications in various domains, such as data processing and file handling.
Data Processing Applications
In data processing, you often need to transform and manipulate data. Converting a List to an Array can be a fundamental part of this process. Arrays can be more efficient than Lists in terms of memory usage and performance, which can be crucial when dealing with large datasets.
File Handling Scenarios
When working with files in Java, you often read data into a List because it’s a dynamic data structure that can easily accommodate an unknown number of data items. However, once the data is loaded, you might need to convert this List to an Array for further processing or to use APIs that require an Array as input.
Exploring Related Java Concepts
Mastering the conversion of a List to an Array in Java can also be a stepping stone to understanding related concepts, such as the Java Collections Framework and Java Streams.
The Java Collections Framework
The Java Collections Framework is a set of classes and interfaces that implement commonly reusable collection data structures. This includes the List interface we’ve been discussing, but also other data structures like Set, Map, Queue, and more.
Java Streams
Java Streams, introduced in Java 8, is a powerful tool for processing collections of objects. It provides a simple, declarative API for performing complex data transformations, such as filtering, mapping, reducing, and more.
Further Resources for Mastering Java List and Array
To deepen your understanding of these topics, here are some recommended resources:
- IOFlood’s Article on Java Casting discusses implicit and explicit casting in Java and when to use each.
Array to List in Java – Convert arrays to lists seamlessly in Java for versatile data handling.
Converting Int to String – Explore methods to transform int data into string representations.
Oracle’s Java Tutorials cover all aspects of Java programming, including the Collections Framework and Streams.
Baeldung’s Guide to Java Arrays covers everything you need to know about Arrays in Java.
JavaCodeGeeks’ Java List Conversion Tutorial provides a deep dive into converting lists to arrays, and vice-versa.
Wrapping Up: List to Array Conversion
In this comprehensive guide, we’ve delved into the process of converting a List to an Array in Java, a common task that is fundamental to many applications in Java programming.
We began with the basics, exploring how to use the toArray()
method to perform a simple conversion. We then dove deeper, discussing more advanced techniques, such as converting a List of custom objects to an Array and handling null
values in the List.
Along the way, we tackled common challenges you might encounter during the conversion process, such as NullPointerException
and ArrayStoreException
, providing you with practical solutions and workarounds for each issue.
We also explored alternative approaches to List to Array conversion, such as using Java 8 Streams or third-party libraries. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity |
---|---|---|
toArray() Method | Moderate | Low |
Java 8 Streams | High | Moderate |
Third-Party Libraries | High | High |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to convert a List to an Array in Java, and the various scenarios and challenges you might encounter along the way.
The ability to convert a List to an Array is a fundamental skill in Java programming, opening up a world of possibilities for data manipulation and processing. Now, you’re well equipped to tackle this task with confidence. Happy coding!