Python Ordereddict Usage Guide (With Examples)
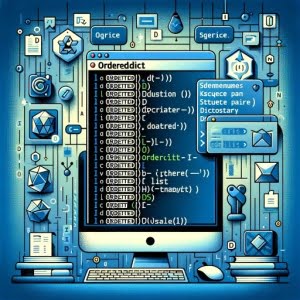
Are you grappling with Python’s OrderedDict? Think of an OrderedDict like a meticulously arranged bookshelf, where each book (or item) has its specific place.
In this comprehensive guide, we’ll delve deep into Python’s OrderedDict. Whether you’re a beginner or an intermediate Python programmer, this guide aims to enhance your understanding and efficient use of Python’s OrderedDict.
So, let’s embark on this journey to demystify OrderedDict and make it a valuable tool in your Python programming toolkit. Remember, the key to mastering Python’s OrderedDict is practice, so we’ve included plenty of code examples for you to try out as you read along.
TL;DR: How Do I Use Python’s OrderedDict?
To use Python’s OrderedDict, you initiate it from the collections module and use it like a standard dictionary. However, the major difference is that it maintains the order of items.
Here’s a simple example illustrating this concept:
from collections import OrderedDict
d = OrderedDict()
d['a'] = 1
d['b'] = 2
print(d)
# Output:
# OrderedDict([('a', 1), ('b', 2)])
In the above code, we first import OrderedDict from the collections module. Then, we create an instance of it. We add two items to it (‘a’ and ‘b’), and finally, we print the dictionary. The output shows that the items are in the same order we added them, unlike a regular Python dictionary, which doesn’t guarantee order. It’s as simple as that!
But wait, there’s more! If you’re curious about more detailed explanations, advanced usage scenarios, or even alternative approaches, keep reading. We’ve got a lot more to share about Python’s OrderedDict.
Table of Contents
Getting Started with Python’s OrderedDict
In the world of Python, an OrderedDict is a dictionary subclass that remembers the order in which its contents—keys and values—are added. Let’s dive into the details of how to create, add items to, and retrieve items from an OrderedDict.
Creating an OrderedDict
First, let’s create an OrderedDict. You can do this by importing the OrderedDict class from the collections module. Here’s how:
from collections import OrderedDict
# Create an instance of OrderedDict
d = OrderedDict()
print(d)
# Output:
# OrderedDict()
In the above code, we created an empty OrderedDict. Simple, right?
Adding Items to an OrderedDict
Next, let’s add some items to our OrderedDict. The process is similar to adding items to a regular dictionary.
# Add items to the OrderedDict
d['apple'] = 'fruit'
d['carrot'] = 'vegetable'
print(d)
# Output:
# OrderedDict([('apple', 'fruit'), ('carrot', 'vegetable')])
In the above code, we added two items to our OrderedDict: ‘apple’ and ‘carrot’. The output demonstrates that the items are stored in the order they were added.
Retrieving Items from an OrderedDict
You can retrieve items from an OrderedDict just like you would from a regular dictionary. Here’s how:
# Retrieve items from the OrderedDict
print(d['apple'])
# Output:
# 'fruit'
In the above code, we retrieved the value of ‘apple’ from our OrderedDict, which returned ‘fruit’.
Remember: Unlike a regular dictionary, an OrderedDict maintains the order of items, which can be incredibly useful in certain situations. However, keep in mind that an OrderedDict uses more memory than a regular dictionary, which might be a potential pitfall if you’re dealing with a large amount of data.
Advanced Techniques with Python’s OrderedDict
Now that we’ve covered the basics, let’s explore some more complex uses of OrderedDict. This includes reordering items, deleting items, and even combining OrderedDicts.
Reordering Items in an OrderedDict
One of the unique features of an OrderedDict is the ability to reorder its items. Let’s see how it works:
# Reorder items in the OrderedDict
d.move_to_end('apple')
print(d)
# Output:
# OrderedDict([('carrot', 'vegetable'), ('apple', 'fruit')])
In the above code, we used the move_to_end
method to move ‘apple’ to the end of our OrderedDict. The output shows that ‘apple’ is now the last item in the dictionary.
Deleting Items from an OrderedDict
Deleting items from an OrderedDict is as straightforward as from a regular dictionary. Here’s an example:
# Delete an item from the OrderedDict
del d['apple']
print(d)
# Output:
# OrderedDict([('carrot', 'vegetable')])
In the above code, we deleted ‘apple’ from our OrderedDict using the del
keyword. The output shows that ‘apple’ is no longer in the dictionary.
Combining OrderedDicts
You can also merge two OrderedDicts while maintaining the order of items. Let’s see how:
# Create a second OrderedDict
d2 = OrderedDict()
d2['orange'] = 'fruit'
d2['spinach'] = 'vegetable'
# Merge the two OrderedDicts
d.update(d2)
print(d)
# Output:
# OrderedDict([('carrot', 'vegetable'), ('orange', 'fruit'), ('spinach', 'vegetable')])
In the above code, we first created a second OrderedDict, d2
, and then merged it with our original OrderedDict, d
, using the update
method. The output shows that the items from d2
were added to the end of d
, maintaining their original order.
These advanced techniques can make your Python programming more efficient, especially when dealing with complex data structures.
Exploring Alternatives to Python’s OrderedDict
While OrderedDict is a powerful tool, it’s not the only way to maintain order in a dictionary in Python. In this section, we’ll explore some alternative methods, including using regular dictionaries in Python 3.7 and above, and leveraging third-party libraries.
Using Regular Dictionaries in Python 3.7+
Starting with Python 3.7, regular dictionaries maintain the insertion order, making them behave much like an OrderedDict. Here’s an example:
# Using a regular dictionary in Python 3.7+
d3 = {}
d3['banana'] = 'fruit'
d3['broccoli'] = 'vegetable'
print(d3)
# Output:
# {'banana': 'fruit', 'broccoli': 'vegetable'}
In the above code, we created a regular dictionary and added two items. The output shows that the items are in the same order we added them.
This feature provides the functionality of an OrderedDict without the extra memory overhead. However, it lacks some of the methods provided by OrderedDict, such as move_to_end
.
Using Third-Party Libraries
There are also third-party libraries, such as sortedcontainers
, that provide sorted dictionary types. Here’s an example of how to use a SortedDict:
# Using SortedDict from sortedcontainers
from sortedcontainers import SortedDict
d4 = SortedDict()
d4['banana'] = 'fruit'
d4['broccoli'] = 'vegetable'
print(d4)
# Output:
# SortedDict({'banana': 'fruit', 'broccoli': 'vegetable'})
In the above code, we imported SortedDict from the sortedcontainers
library and used it to create a sorted dictionary. The output shows that the items are sorted by their keys. While this is a powerful tool, it does require installing an additional library, which may not be ideal in all situations.
While Python’s OrderedDict is a powerful tool for maintaining order in a dictionary, there are alternatives that may better suit your needs, depending on your specific use case and the version of Python you’re using.
Addressing Common Issues with Python’s OrderedDict
Like any tool, OrderedDict comes with its own set of considerations. In this section, we’ll discuss common issues you might encounter when using OrderedDict, such as memory usage and performance considerations. We’ll also provide solutions and workarounds for these issues.
Memory Usage
One of the main considerations when using OrderedDict is its memory usage. An OrderedDict uses more memory than a regular dictionary due to the additional data it stores to maintain order. Here’s a simple comparison:
import sys
# Create a regular dictionary and an OrderedDict with the same items
dict_regular = {'a': 1, 'b': 2}
dict_ordered = OrderedDict(a=1, b=2)
# Compare their memory usage
print('Regular dict:', sys.getsizeof(dict_regular))
print('OrderedDict:', sys.getsizeof(dict_ordered))
# Output:
# Regular dict: 240
# OrderedDict: 400
In the above code, we created a regular dictionary and an OrderedDict with the same items, and then compared their memory usage using the sys.getsizeof
function. The output shows that the OrderedDict uses significantly more memory.
Performance Considerations
In addition to memory usage, you should also consider the performance implications of using an OrderedDict. While the difference is usually negligible for small data sets, it can become noticeable when working with large data sets.
This is especially true for operations that involve reordering or deleting items, which are more computationally expensive for an OrderedDict than for a regular dictionary.
While Python’s OrderedDict is a powerful tool, it’s important to be aware of these considerations. Depending on your specific needs and the size of your data, a regular dictionary or an alternative approach might be more efficient.
Python Dictionaries vs OrderedDict
To truly appreciate Python’s OrderedDict, it’s important to understand its predecessor, the standard Python dictionary. Let’s delve into the fundamental differences between these two data types and why maintaining order in a dictionary can be crucial.
Understanding Python Dictionaries
A Python dictionary is a built-in data type that stores data in key-value pairs. Here’s a simple example of a Python dictionary:
# Create a regular dictionary
dict_regular = {'cat': 'animal', 'rose': 'flower'}
print(dict_regular)
# Output:
# {'cat': 'animal', 'rose': 'flower'}
In the above code, we created a regular dictionary with two items: ‘cat’ and ‘rose’. The output shows the items in the order we added them. However, in versions of Python before 3.7, this order is not guaranteed to be preserved.
The Importance of Order
While the order of items might not matter in many situations, there are cases where it can be critical. For example, if you’re working with time-series data, the order of entries is essential to maintain the chronological sequence of events. Similarly, in a task queue, the order of tasks determines their execution sequence. In these cases, using an OrderedDict can be invaluable.
In conclusion, while Python’s standard dictionary is a versatile tool for storing key-value pairs, the OrderedDict provides an additional layer of functionality by maintaining the order of items. This makes it a powerful tool in your Python programming toolkit, especially when the order of items is important.
Further Resources
For more in-depth information on OrderedDict and other Python data structures, check out these resources:
- IOFlood’s Blog Post on Python Dictionaries, with syntax and examples. Dive deep into the world of Python dictionaries and become a proficient user of this essential data structure.
Combining Data Sources: Python Merge Dictionaries – Master the process of merging dictionaries in Python.
Pretty Printing Python Dictionaries: Improving Readability – Learn how to format and print Python dictionaries for readability.
Python Documentation on OrderedDict – The official Python documentation provides an explanation of OrderedDict in the collections module.
Questions on OrderedDict – This is a compilation of discussions, questions, and answers related to the usage of OrderedDict in Python on the Stack Overflow platform.
Guide on OrderedDict in Python – This guide from Geeks for Geeks provides an in-depth understanding of the OrderedDict data structure in Python, demonstrating its functionalities with examples.
To deepen your understanding of Python, consider exploring related concepts like lists, tuples, sets, and other dictionary types.
Each of these structures has its own strengths and use-cases, and a well-rounded knowledge of them will make you a more versatile programmer.
Wrapping Up Python’s OrderedDict
In this comprehensive guide, we’ve traversed the ins and outs of Python’s OrderedDict, a powerful tool that maintains the order of items in a dictionary. We’ve explored everything from basic usage to advanced techniques, and even delved into alternative approaches.
Key Takeaways
- OrderedDict is a dictionary subclass in Python that remembers the order of items, unlike regular dictionaries in Python versions prior to 3.7.
- It provides additional methods like
move_to_end
for reordering items, which regular dictionaries lack. - While useful, OrderedDict uses more memory than regular dictionaries, which could be a potential pitfall for large data sets.
Alternative Approaches
- Regular dictionaries in Python 3.7 and above maintain the order of items, providing similar functionality to OrderedDict without the memory overhead.
Third-party libraries like
sortedcontainers
offer sorted dictionary types, providing additional functionality at the cost of requiring an extra library.
In conclusion, while OrderedDict is a powerful tool, it’s important to consider your specific needs and the trade-offs involved. Whether you choose to use an OrderedDict, a regular dictionary, or a third-party library, understanding these concepts will make you a more effective Python programmer.