parseInt() Java Method: From Strings to Integers
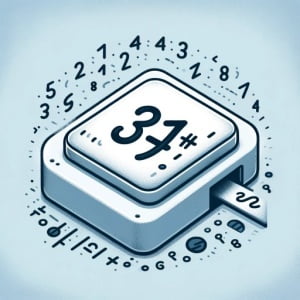
Are you finding it challenging to convert strings to integers in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled mathematician, Java’s parseInt method can transform a string of digits into a usable integer. These integers can then be used in a variety of ways in your Java programs, from calculations to control structures.
This guide will explain how to use the parseInt method in Java, from basic usage to more advanced techniques. We’ll explore parseInt’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering parseInt in Java!
TL;DR: How Do I Use parseInt in Java?
The
Integer.parseInt()
method is used to convert a string to an integer in Java, with the syntaxint num = Integer.parseInt(str);
. This function takes a string of digits and transforms it into a usable integer.
Here’s a simple example:
String str = "123";
int num = Integer.parseInt(str);
System.out.println(num);
// Output:
// 123
In this example, we’ve used the Integer.parseInt()
method to convert the string ‘123’ into an integer. The string is passed as an argument to the method, which then returns the integer value. The result is then printed to the console, outputting ‘123’.
This is a basic way to use parseInt in Java, but there’s much more to learn about string to integer conversion. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Mastering Basic Use of ParseInt in Java
- Handling Exceptions with ParseInt in Java
- Exploring Alternative Conversion Methods in Java
- Troubleshooting Common Issues with ParseInt in Java
- Digging Deeper: Java’s String and Integer Classes
- Exploring Broader Applications of ParseInt in Java
- Wrapping Up: ParseInt in Java
Mastering Basic Use of ParseInt in Java
The Integer.parseInt()
method is a powerful tool in Java’s arsenal when it comes to converting strings into integers. Let’s delve deeper into how it works.
The Integer.parseInt()
method is a static method of the Integer class in Java. It takes a string as an argument and returns an integer. The string provided must only contain digits; otherwise, a NumberFormatException
will be thrown.
Let’s look at a simple example:
String str = "456";
int num = Integer.parseInt(str);
System.out.println(num);
// Output:
// 456
In this code block, we’ve declared a string str
with the value ‘456’. The Integer.parseInt()
method is then called with str
as the argument, converting the string ‘456’ into the integer 456. This integer is stored in the variable num
, which is then printed to the console.
This method is incredibly useful when you need to perform mathematical operations on numeric data that has been inputted or received as a string. However, it’s crucial to ensure that the string being passed to Integer.parseInt()
only contains digits. If the string contains any non-digit characters, a NumberFormatException
will be thrown, and your program will crash.
In the next section, we’ll delve into how to handle such exceptions and explore more advanced uses of the Integer.parseInt()
method.
Handling Exceptions with ParseInt in Java
As you become more comfortable with using Integer.parseInt()
, it’s important to understand how to handle exceptions that may arise during string to integer conversion. One of the most common exceptions you’ll encounter is NumberFormatException
.
A NumberFormatException
is thrown when you try to parse a string that contains non-digit characters. This includes spaces, punctuation, and letters. To prevent your program from crashing, you can use a try-catch block to handle this exception.
Let’s look at an example:
String str = "123abc";
try {
int num = Integer.parseInt(str);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println("Invalid number format");
}
// Output:
// Invalid number format
In this code block, Integer.parseInt()
tries to parse the string ‘123abc’, which contains non-digit characters. This results in a NumberFormatException
. However, because the code is wrapped in a try-catch block, the exception is caught, and ‘Invalid number format’ is printed to the console instead of the program crashing.
Using try-catch blocks to handle exceptions is a best practice in Java programming. It allows you to control the flow of your program and provide meaningful feedback to the user, even when unexpected input is encountered.
Exploring Alternative Conversion Methods in Java
While Integer.parseInt()
is a popular method for converting strings to integers in Java, it’s not the only one. Another method you can use is Integer.valueOf()
.
The Integer.valueOf()
method is similar to Integer.parseInt()
, but there’s a key difference: Integer.valueOf()
returns an instance of Integer, while Integer.parseInt()
returns an int.
Let’s look at an example:
String str = "789";
Integer num = Integer.valueOf(str);
System.out.println(num);
// Output:
// 789
In this code block, Integer.valueOf()
is used to convert the string ‘789’ into an Integer. The result is then printed to the console.
So, when should you use Integer.parseInt()
and when should you use Integer.valueOf()
?
- Use
Integer.parseInt()
when you need a primitive int. This method is also slightly more efficient in terms of speed. - Use
Integer.valueOf()
when you need an Integer object. This method uses caching and can be more memory-efficient when dealing with a large number of integers.
Here’s a comparison table for quick reference:
Method | Returns | Best Used For |
---|---|---|
Integer.parseInt() | int | Speed, when you need a primitive |
Integer.valueOf() | Integer | Memory efficiency, when you need an object |
By understanding these different methods and their advantages, you can choose the best tool for your specific needs in any given scenario.
Troubleshooting Common Issues with ParseInt in Java
String to integer conversion in Java using Integer.parseInt()
or Integer.valueOf()
is typically straightforward. However, certain issues may arise that could disrupt this process. Let’s discuss some of these common problems and how to tackle them.
Dealing with NumberFormatException
As we’ve previously discussed, a NumberFormatException
is thrown when a string containing non-digit characters is passed to Integer.parseInt()
or Integer.valueOf()
. This can be mitigated by using a try-catch block.
String str = "123abc";
try {
int num = Integer.parseInt(str);
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println("Invalid number format");
}
// Output:
// Invalid number format
In this example, the string ‘123abc’ contains non-digit characters. When this string is passed to Integer.parseInt()
, a NumberFormatException
is thrown. However, the try-catch block catches this exception and prints ‘Invalid number format’ instead of crashing the program.
Handling Non-Numeric Strings
If you’re dealing with a string that may contain non-numeric characters and you want to extract the digits, you could use a regular expression to remove the non-digit characters before parsing.
String str = "123abc456";
str = str.replaceAll("\\D", "");
int num = Integer.parseInt(str);
System.out.println(num);
// Output:
// 123456
In this code block, the replaceAll()
method is used to remove all non-digit characters from the string ‘123abc456’, resulting in the string ‘123456’. This string is then passed to Integer.parseInt()
, which successfully converts it to the integer 123456.
By understanding these common issues and their solutions, you can ensure that your string to integer conversions in Java are robust and error-free.
Digging Deeper: Java’s String and Integer Classes
To fully grasp the process of converting strings to integers in Java using Integer.parseInt()
, it’s important to understand the fundamental concepts behind Java’s String and Integer classes.
The String Class
In Java, strings are objects that are backed internally by a char array. When you create a string in Java, you’re actually creating an object of the String class. This class comes with numerous methods for manipulating and dealing with strings.
String str = "Hello, World!";
System.out.println(str.length());
// Output:
// 13
In this example, we’ve created a string str
and used the length()
method of the String class to print the length of the string. The output is ’13’, which is the number of characters in the string.
The Integer Class
The Integer class in Java is a wrapper class for the int primitive type. It provides a number of useful class (i.e., static) methods to convert an int to a String and a String to an int, among other utilities.
int i = 10;
String str = Integer.toString(i);
System.out.println(str);
// Output:
// 10
In this code block, we’ve used the Integer.toString()
method to convert the integer ’10’ into a string. The result is the string ’10’.
Understanding these fundamental classes in Java is crucial for comprehending the process of string to integer conversion. In the next section, we’ll explore how these concepts tie into real-world applications of data processing and user input handling.
Exploring Broader Applications of ParseInt in Java
The ability to convert strings to integers in Java using Integer.parseInt()
is not just an isolated skill—it plays a vital role in many aspects of programming, from data processing to user input handling.
Data Processing
In data processing, you often need to convert strings into integers. For example, you might be reading data from a file where numbers are stored as strings. In such cases, Integer.parseInt()
becomes an essential tool for data manipulation and analysis.
User Input Handling
When dealing with user input in applications, you often receive numerical data as strings. Integer.parseInt()
allows you to convert this input into a usable form for calculations, comparisons, and other operations.
Exploring Related Concepts
Once you’ve mastered string to integer conversion in Java, there are plenty of related concepts to explore. Handling other data types, mastering exception handling, and delving into Java’s other built-in methods are all excellent ways to deepen your Java expertise.
Further Resources for Java String Conversion
To help you continue your learning journey, here are some additional resources:
- Best Practices for Java Casting – Discover how to cast arrays and collections in Java for data manipulation.
Java String to Int Conversion – Master converting strings to integers for robust data processing in Java applications.
Converting JSON to Java Object – Learn techniques to map JSON properties to Java object attributes.
Oracle’s Java Documentation – A comprehensive resource covering all aspects of Java programming.
Java Tutorials by TutorialsPoint offers a wide range of Java tutorials, including string conversion.
Java Programming Course by Codecademy provides hands-on experience with Java string and integer manipulation.
Wrapping Up: ParseInt in Java
In this comprehensive guide, we’ve journeyed through the world of parseInt in Java, a powerful tool for converting strings to integers.
We began with the basics, learning how to use the Integer.parseInt()
method to convert a string of digits into a usable integer. We then ventured into more advanced territory, exploring how to handle exceptions like NumberFormatException
using try-catch blocks.
We also delved into alternative approaches to string to integer conversion, such as using the Integer.valueOf()
method, and provided a comparison of these methods to help you choose the best tool for your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Returns | Best Used For |
---|---|---|
Integer.parseInt() | int | Speed, when you need a primitive |
Integer.valueOf() | Integer | Memory efficiency, when you need an object |
Whether you’re just starting out with parseInt in Java or you’re looking to level up your string to integer conversion skills, we hope this guide has given you a deeper understanding of parseInt and its capabilities.
With its balance of speed and versatility, parseInt is a powerful tool for string to integer conversion in Java. Happy coding!