Converting JSON data to Java objects: A How-to Guide
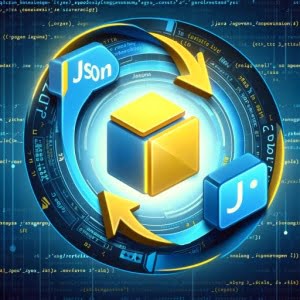
Are you finding it challenging to convert JSON to a Java object? You’re not alone. Many developers find themselves in a similar situation, but there’s a method to the madness.
Think of JSON as a universal language that Java can understand with the right translator. This translator comes in the form of libraries like Jackson or Gson, which can seamlessly convert JSON to Java objects.
This guide will walk you through the process of converting JSON to Java object, from the basic use to advanced techniques. We’ll cover everything from using libraries like Jackson and Gson, handling complex scenarios like nested JSON objects and arrays, to troubleshooting common issues.
So, let’s dive in and start mastering JSON to Java object conversion!
TL;DR: How Do I Convert JSON to a Java Object?
Converting JSON to a Java object can be done using libraries like Jackson:
objectMapper.readValue(jsonString, User.class)
.
Here’s a basic example using the Jackson library:
ObjectMapper objectMapper = new ObjectMapper();
User user = objectMapper.readValue(jsonString, User.class);
# Output:
# User object populated with data from jsonString
In this example, we’re using the ObjectMapper
class from the Jackson library to convert a JSON string (jsonString
) into a Java object (User
). The readValue
method is used to map the JSON data to the User
class.
This is just a basic example. Continue reading for a deeper understanding and more advanced usage scenarios of converting JSON to Java object.
Table of Contents
- Converting JSON to Java Object: Basic Use with Jackson
- Handling Complex Scenarios: Nested JSON and Arrays
- Exploring Alternatives: Gson and JSON-B
- Troubleshooting JSON to Java Conversion: Common Issues
- Understanding JSON and Java Objects
- Role of Jackson and Gson in JSON to Java Conversion
- Real-World Applications and Beyond
- Wrapping Up: Mastering JSON to Java Object Conversion
Converting JSON to Java Object: Basic Use with Jackson
To get started with converting JSON to a Java object, we’ll use the Jackson library. Jackson is a popular library in Java that provides tools for processing JSON data, including a feature to convert JSON to a Java object.
Here’s a simple step-by-step guide on how to use Jackson for this purpose:
- Add the Jackson library to your Java project. You can do this by adding the following dependency to your
pom.xml
file if you’re using Maven, or to yourbuild.gradle
file if you’re using Gradle.
<!-- Maven -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.5</version>
</dependency>
# Gradle
implementation 'com.fasterxml.jackson.core:jackson-databind:2.12.5'
- Create a Java class that matches the structure of your JSON data. For example, if your JSON data represents a user with a name and email, you might have a
User
class like this:
public class User {
private String name;
private String email;
// getters and setters
}
- Use the
ObjectMapper
class from Jackson to convert the JSON string to a Java object. Here’s how you can do it:
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = "{"name":"John Doe","email":"[email protected]"}";
User user = objectMapper.readValue(jsonString, User.class);
# Output:
# User object with name 'John Doe' and email '[email protected]'
In this example, we’re creating an instance of the ObjectMapper
class, which is the main class in Jackson for converting between JSON and Java objects. We’re then using the readValue
method to convert our JSON string to a User
object.
This is a straightforward way to convert JSON to a Java object using Jackson. However, it’s important to note that this approach assumes that your JSON data matches the structure of your Java object. If this isn’t the case, you might encounter errors or unexpected behavior. In the next section, we’ll cover more complex scenarios and how to handle them.
Handling Complex Scenarios: Nested JSON and Arrays
As you dive deeper into JSON to Java object conversion, you’ll encounter more complex scenarios. Let’s discuss a few of them: nested JSON objects, handling arrays, and dealing with null values.
Converting Nested JSON Objects
Nested JSON objects are quite common. These are JSON objects with other JSON objects as their properties. Here’s how you can handle them:
public class User {
private String name;
private ContactInfo contactInfo;
// getters and setters
}
public class ContactInfo {
private String email;
private String phone;
// getters and setters
}
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = "{"name":"John Doe","contactInfo":{"email":"[email protected]","phone":"1234567890"}}";
User user = objectMapper.readValue(jsonString, User.class);
# Output:
# User object with name 'John Doe' and contactInfo object with email '[email protected]' and phone '1234567890'
In this example, we have a User
class with a nested ContactInfo
object. The ContactInfo
class has two properties: email
and phone
. We’re using the readValue
method as before, but this time it’s capable of handling the nested JSON object and converting it to a nested Java object.
Handling JSON Arrays
JSON arrays are also common. These are represented as lists in Java. Here’s how you can convert a JSON array to a Java list:
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = "[{"name":"John Doe"},{"name":"Jane Doe"}]";
List<User> users = objectMapper.readValue(jsonString, new TypeReference<List<User>>() {});
# Output:
# List of User objects with names 'John Doe' and 'Jane Doe'
In this example, we’re using the readValue
method again, but this time with a TypeReference
to specify that we’re converting the JSON array to a list of User
objects.
Dealing with Null Values
Sometimes, your JSON data might contain null values. By default, Jackson will map these to null
in your Java object. If you want to change this behavior, you can use the @JsonInclude
annotation in your Java class:
@JsonInclude(JsonInclude.Include.NON_NULL)
public class User {
private String name;
private String email;
// getters and setters
}
With this annotation, any null values in the JSON data will be ignored during the conversion process, and the corresponding properties in the Java object will not be set.
These are just a few examples of the complex scenarios you might encounter when converting JSON to a Java object. By understanding these, you’ll be better equipped to handle whatever JSON data comes your way.
Exploring Alternatives: Gson and JSON-B
While Jackson is a powerful tool for converting JSON to Java objects, it’s not the only library available. Let’s explore two alternative libraries: Gson and JSON-B.
Converting JSON to Java Object with Gson
Gson is a popular JSON processing library developed by Google. It provides simple methods for converting JSON to Java objects and vice versa.
Here’s how you can perform the conversion with Gson:
Gson gson = new Gson();
String jsonString = "{"name":"John Doe","email":"[email protected]"}";
User user = gson.fromJson(jsonString, User.class);
# Output:
# User object with name 'John Doe' and email '[email protected]'
In this example, we’re using the fromJson
method of the Gson
class to convert the JSON string to a User
object. It’s quite similar to how we used Jackson’s readValue
method.
JSON-B: The Java EE Way
JSON-B (JSON Binding) is a part of Java EE and provides a standard way to bind JSON documents to Java objects. If you’re working in a Java EE environment, you might prefer using JSON-B.
Here’s how you can convert JSON to a Java object with JSON-B:
Jsonb jsonb = JsonbBuilder.create();
String jsonString = "{"name":"John Doe","email":"[email protected]"}";
User user = jsonb.fromJson(jsonString, User.class);
# Output:
# User object with name 'John Doe' and email '[email protected]'
In this example, we’re using the fromJson
method of the Jsonb
class to perform the conversion. Again, the process is quite similar to the other libraries.
Making the Right Choice
Choosing between Jackson, Gson, and JSON-B largely depends on your specific needs and environment. Jackson is incredibly versatile and handles complex scenarios well, but Gson might be more appealing if you prefer a simpler API or are already using other Google libraries. JSON-B, on the other hand, is a good choice if you’re working in a Java EE environment.
Regardless of the library you choose, the fundamental process of converting JSON to a Java object remains the same: map the JSON data to a Java class and use a method provided by the library to perform the conversion.
Troubleshooting JSON to Java Conversion: Common Issues
While converting JSON to Java objects, you might encounter a few common issues. Let’s discuss how to handle incompatible data types, exceptions, and large JSON files.
Dealing with Incompatible Data Types
Incompatibility between JSON data types and Java data types can cause issues during conversion. For instance, if your JSON data contains a number but your Java class expects a string, you’ll get an error.
To handle this, you can use custom deserializers in Jackson or Gson. Here’s an example using Jackson:
public class StringToNumberDeserializer extends JsonDeserializer<String> {
@Override
public String deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
return String.valueOf(p.getValueAsLong());
}
}
@JsonDeserialize(using = StringToNumberDeserializer.class)
public class User {
private String id;
// other fields
}
In this example, we’re using a custom deserializer (StringToNumberDeserializer
) that converts a number to a string during the JSON to Java object conversion.
Handling Exceptions
Exceptions can occur during the conversion process for various reasons, such as malformed JSON or unexpected data types. It’s important to handle these exceptions to prevent your application from crashing.
Here’s how you can handle exceptions with Jackson:
try {
User user = objectMapper.readValue(jsonString, User.class);
} catch (JsonProcessingException e) {
// handle exception
}
In this example, we’re using a try-catch block to handle any JsonProcessingException
that might occur during the conversion process.
Dealing with Large JSON Files
Large JSON files can be memory-intensive to process. To handle large JSON files, you can use the streaming API provided by Jackson or Gson. This allows you to process the JSON data in chunks, reducing memory usage.
Here’s an example using Jackson’s streaming API:
JsonFactory factory = new JsonFactory();
JsonParser parser = factory.createParser(new File("large.json"));
while (!parser.isClosed()) {
JsonToken jsonToken = parser.nextToken();
// process jsonToken
}
In this example, we’re using Jackson’s JsonFactory
and JsonParser
classes to process a large JSON file in chunks.
These are just a few of the common issues you might encounter when converting JSON to Java objects. By understanding these, you’ll be better prepared to handle any challenges that come your way.
Understanding JSON and Java Objects
Before we dive deeper into the conversion process, it’s essential to understand what JSON and Java objects are and why we need to convert between the two.
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It’s a text format that’s completely language independent but uses conventions familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
Here’s an example of a simple JSON object:
{
"name": "John Doe",
"email": "[email protected]"
}
What is a Java Object?
A Java object is an instance of a class where the class is a blueprint or prototype that defines the variables and methods common to all objects of a certain kind.
Here’s an example of a simple Java object:
public class User {
private String name;
private String email;
// getters and setters
}
Why Convert Between JSON and Java Objects?
In modern web development, data is often exchanged between a browser and a server in JSON format. However, Java is a statically-typed language, which means you can’t use JSON directly in Java code. You need to convert the JSON data to a Java object before you can use it.
Role of Jackson and Gson in JSON to Java Conversion
This is where libraries like Jackson and Gson come in. They provide tools to convert JSON to Java objects and vice versa. These libraries handle all the details of the conversion process, allowing you to focus on using the data in your application.
Real-World Applications and Beyond
The conversion of JSON to Java objects is not just an academic exercise. It has profound implications in real-world applications, particularly in areas such as web development and data analysis.
Web Development and JSON
In web development, data is often exchanged between the client (browser) and the server in the form of JSON. This data needs to be converted into Java objects on the server-side for processing.
Whether you’re developing a simple web application or working on a complex system, understanding how to convert JSON to Java objects is a crucial skill.
Data Analysis with JSON
In data analysis, JSON is a common format for data interchange. Data analysts often need to convert JSON data into Java objects to perform various operations, such as filtering, sorting, and aggregation.
Mastering JSON to Java object conversion can significantly streamline your data analysis workflow.
Handling JSON in Other Languages
While this guide focuses on converting JSON to Java objects, it’s worth noting that similar processes exist in other programming languages. For example, in Python, you would use the json
module to convert JSON to Python objects (dictionaries), and in JavaScript, you would use JSON.parse()
to convert JSON to JavaScript objects.
Working with APIs
Many web APIs return data in JSON format. When you’re working with these APIs in a Java environment, you’ll often need to convert the returned JSON data into Java objects. This makes JSON to Java object conversion a key skill in API development and integration.
Further Resources for JSON to Java Object Conversion Mastery
If you’re interested in diving deeper into JSON to Java object conversion, here are some resources you might find helpful:
- Fundamentals Covered: Java Casting – Understand how to use casting to access superclass and subclass members in Java.
Java ParseInt Method – Master parsing integer values from strings for accurate data conversion in Java.
Understanding ToString in Java – Learn about overriding toString() to provide customized string representations for objects.
Baeldung’s Guide on Jackson covers using the Jackson library for JSON processing in Java.
Gson User Guide – The official user guide for the Gson library.
Java EE JSON Processing Tutorial – An in-depth tutorial on JSON processing in Java EE, including JSON-B.
With these resources and the knowledge you’ve gained from this guide, you’re well on your way to becoming a master of JSON to Java object conversion.
Wrapping Up: Mastering JSON to Java Object Conversion
In this comprehensive guide, we’ve delved deep into the process of converting JSON to Java objects, a fundamental skill in web development and data analysis.
We began with the basics, learning how to use the Jackson library to perform the conversion. We then ventured into more advanced territory, handling complex scenarios like nested JSON objects, arrays, and null values. Along the way, we tackled common challenges you might face during the conversion process, such as dealing with incompatible data types, handling exceptions, and processing large JSON files.
We also explored alternative libraries for JSON to Java object conversion, including Gson and JSON-B. These alternatives, while similar in functionality, offer unique features that might make them more suitable for certain use cases.
Here’s a quick comparison of the libraries we’ve discussed:
Library | Versatility | Ease of Use | Environment Compatibility |
---|---|---|---|
Jackson | High | Moderate | Any Java environment |
Gson | Moderate | High | Any Java environment, Google libraries |
JSON-B | Moderate | High | Java EE environment |
Whether you’re just starting out with JSON to Java object conversion or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the process and its real-world applications.
With the knowledge you’ve gained from this guide, you’re well-equipped to handle any JSON data that comes your way. Happy coding!