Python While Loop | Complete Guide (With Examples)
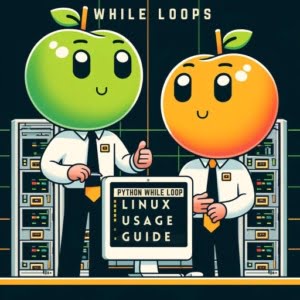
Utilizing a while loop in Python is crucial while programming on Linux servers at IOFLOOD, allowing iterative execution of code blocks based on specified conditions. In our experience, while loops enhance code control and efficiency, particularly in tasks requiring dynamic loop conditions. Today’s article explores the usage of a while loop in Python, offering practical examples and insights to empower our customers with effective coding practices on their bare metal cloud server.
In this comprehensive guide, we’ll start from the basics and gradually venture into more advanced techniques of using while loops in Python. Whether you’re a newbie just dipping your toes into the Python waters or a seasoned coder looking to refresh your knowledge, this guide is designed for you.
Buckle up for a deep dive into the world of Python while loops!
TL;DR: How do I use a while loop in Python?
In Python, a while loop is declared using the
'while'
keyword followed by a condition,while {condition to evaluate}: #code block to execute
. The loop will continue to execute as long as the condition remains true.
Here’s a simple example:
i = 0
while i < 5:
print(i)
i += 1
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the condition is ‘i < 5’. The loop starts with ‘i’ as 0 and continues to print ‘i’ and increment it by 1 until ‘i’ is no longer less than 5. This results in the numbers 0 through 4 being printed.
Intrigued? Keep reading for a more detailed exploration of while loops in Python, including advanced usage and troubleshooting tips.
Table of Contents
Python While Loop: The Basics
At its core, a while loop in Python is pretty straightforward. It starts with the ‘while’ keyword, followed by a condition, and then a colon. The statements inside the loop are indented and executed as long as the condition remains true. Let’s break down a simple example:
i = 0
while i < 5:
print(i)
i += 1
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, ‘i < 5’ is the condition. The loop starts with ‘i’ as 0 and continues to print ‘i’ and increment it by 1 until ‘i’ is no longer less than 5. This results in the numbers 0 through 4 being printed.
The primary advantage of a while loop is its flexibility. Unlike a for loop, which operates on a fixed range or sequence, a while loop continues to operate as long as a condition is met. This makes it ideal for situations where you don’t know in advance how many times you need the loop to run.
However, this flexibility can also be a pitfall. If the condition for a while loop is always true, it can result in an infinite loop, which can cause your program to hang or crash. Therefore, it’s crucial to ensure that the condition for your while loop will eventually become false.
Exploring Nested While Loops and While-Else Constructs
As you become more comfortable with Python’s while loop, you can begin to explore more complex uses. Let’s delve into two advanced concepts: nested while loops and while-else constructs.
Nested While Loops
Just like you can nest if statements and for loops, you can also nest while loops. This means you can have a while loop inside another while loop. Here’s an example:
i = 0
while i < 3:
j = 0
while j < 3:
print(f'i: {i}, j: {j}')
j += 1
i += 1
# Output:
# i: 0, j: 0
# i: 0, j: 1
# i: 0, j: 2
# i: 1, j: 0
# i: 1, j: 1
# i: 1, j: 2
# i: 2, j: 0
# i: 2, j: 1
# i: 2, j: 2
In this example, for each iteration of the outer loop (i), the inner loop (j) runs completely, creating a sort of matrix of possible i, j combinations.
While-Else Constructs
Python also allows for an ‘else’ clause with its while loop, which is executed when the while loop’s condition becomes false. This can be particularly useful when you want to perform a certain action once your loop has completed its iterations. Let’s see it in action:
i = 0
while i < 5:
print(i)
i += 1
else:
print('Loop has ended')
# Output:
# 0
# 1
# 2
# 3
# 4
# Loop has ended
In this example, the message ‘Loop has ended’ is printed once ‘i’ is no longer less than 5, indicating the end of the loop.
These advanced techniques can add another layer of functionality to your Python programming, enabling you to handle more complex tasks and logic.
Exploring Alternatives to While Loops
While loops are powerful, Python offers other looping constructs that might be better suited depending on your specific scenario. In this section, we’ll explore for loops and the use of break, continue, and pass statements within loops.
For Loops: A Different Kind of Loop
For loops in Python are used for iterating over a sequence such as a list, tuple, dictionary, string, or a set. They are different from while loops in that they run for a pre-defined number of times. Here’s a simple for loop example:
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the for loop iterates over each item in the range from 0 to 4, printing each number. Unlike the while loop, the for loop doesn’t require a condition to stop. It stops automatically after it has iterated over all items in the sequence.
Break, Continue, and Pass: Controlling Your Loop
Python provides the break, continue, and pass statements to give you more control over your loops:
- The
break
statement allows you to stop the loop even if the while condition is true:
i = 0
while i < 5:
if i == 3:
break
print(i)
i += 1
# Output:
# 0
# 1
# 2
In this example, the break statement stops the loop before ‘i’ equals 3, even though the while condition (‘i < 5’) is still true.
- The
continue
statement allows you to skip the rest of the loop and immediately continue with the next iteration:
i = 0
while i < 5:
i += 1
if i == 3:
continue
print(i)
# Output:
# 1
# 2
# 4
# 5
Here, when ‘i’ equals 3, the continue statement causes the loop to skip printing ‘i’ and immediately continue with the next iteration.
- The
pass
statement is used when you need a statement for syntax reasons, but you don’t want any command or code to be executed:
i = 0
while i < 5:
if i == 3:
pass
print(i)
i += 1
# Output:
# 0
# 1
# 2
# 3
# 4
In this case, the pass statement does nothing when ‘i’ equals 3, and the loop continues to print ‘i’ as usual.
Choosing between while loops, for loops, and controlling your loop with break, continue, and pass depends on your specific use case. Understanding the differences and how to use each can greatly enhance your Python programming.
Troubleshooting Common Issues with Python While Loops
While loops are a powerful tool in Python, they can sometimes lead to unexpected issues. In this section, we’ll discuss some common problems you might encounter when using while loops and how to solve them.
The Dreaded Infinite Loop
One common issue when using while loops is the infinite loop. This happens when the condition for the while loop never becomes false. Here’s an example:
i = 0
while i < 5:
print(i)
# i += 1
# Output:
# 0
# 0
# 0
# ...
In this code, the increment operation is commented out, so ‘i’ remains 0, and the condition ‘i < 5’ is always true. This causes the while loop to run indefinitely, printing ‘0’ over and over again.
To avoid infinite loops, always ensure that the condition for your while loop is updated inside the loop and will eventually become false.
Handling Errors in Your Loop
Another common issue is errors occurring within your while loop. If an error occurs, it can cause your loop to stop prematurely. To handle this, you can use a try-except block inside your while loop:
i = 0
while i < 5:
try:
print(10 / i)
except ZeroDivisionError:
print('Cannot divide by zero')
i += 1
# Output:
# Cannot divide by zero
# 10.0
# 5.0
# 3.3333333333333335
# 2.5
In this code, when ‘i’ is 0, a ZeroDivisionError occurs. The error is caught by the except block, and ‘Cannot divide by zero’ is printed instead. The loop then continues with the next iteration.
By understanding these common issues and how to handle them, you can use while loops more effectively in your Python programming.
Understanding Loops and Control Flow in Programming
Before we delve deeper into the Python while loop, it’s important to understand the broader concept of loops and control flow in programming.
The Concept of Loops
In programming, a loop is a sequence of instructions that is continually repeated until a certain condition is met. It’s like a conveyor belt that keeps moving until it’s turned off. In Python, while loops and for loops are the two main types of loops.
Loops are a fundamental part of programming because they automate repetitive tasks. Without loops, if you wanted to print numbers 1 to 5, you would have to write the print statement five times. With a loop, you can accomplish this with just a few lines of code:
for i in range(1, 6):
print(i)
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the for loop iterates over the range from 1 to 5, printing each number. This saves time and makes your code more efficient.
Control Flow: The Traffic Cop of Programming
Control flow refers to the order in which the instructions in your code are executed. Control structures, such as loops and conditional statements (like if, elif, else), direct the flow of your program. They are like the traffic cop at an intersection, directing the flow of traffic.
Understanding loops and control flow is crucial to mastering Python while loops. With this knowledge, you can write more efficient code and solve complex problems more easily.
The Power of While Loops in Large Projects
While loops are not just for simple tasks or standalone scripts. Their real power shines through when they are used in larger projects or applications. Whether it’s handling user input in a software application, reading data from a file until the end, or running a server loop that waits for client requests, while loops play an integral role in many programming scenarios.
For instance, consider a script that needs to read and process data from a large file. A while loop could be used to read the data line by line, processing each line and then moving on to the next one, until it reaches the end of the file. This would not only be more efficient than reading the entire file into memory, but it would also allow the script to handle files of any size.
with open('large_file.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break # End of file
process(line) # Some function to process the line
# No output to display as it depends on the file and process function
In this code block, the while loop reads and processes each line of the file until it reaches the end. This is a simple yet powerful demonstration of how while loops can be used in larger scripts or projects.
Diving Deeper: Conditional Statements and Recursion
To further enhance your Python skills, consider exploring related concepts such as conditional statements (if, elif, else) and recursion. Conditional statements allow your code to make decisions and perform different actions depending on various conditions. Recursion, on the other hand, is a method where the solution to a problem depends on solutions to smaller instances of the same problem.
There are many resources available online for learning more about these topics. Consider the following:
- Quick Guide on Python Loop Essentials can help you master while loops in Python.
Looping with Index in Python: Using “for” Loops – Explores how to use loops for iterating over sequences with index values.
Python’s “yield”: Streaming Data Like a Pro – Learn how to implement lazy evaluation and data streaming with “yield.”
Python.org’s Official Documentation hosts a variety of resources, including understanding loops and control flow in Python.
Codecademy’s Python Course includes modules on Python’s loops and control structures.
Coursera’s Python Courses covers various topics, including control flow and loops in Python.
With a solid understanding of while loops and related concepts, you’ll be well on your way to mastering Python programming.
Python While Loops: A Recap
In this comprehensive guide, we’ve journeyed from the basics of Python while loops to more advanced techniques, providing practical examples along the way. We’ve touched on the fundamental syntax of a while loop, its use in Python programming, and how it compares with other looping constructs like the for loop.
Key Takeaways
- While loops execute a set of statements as long as a condition is true. They offer flexibility, especially when the number of iterations is unknown in advance.
Nested while loops and while-else constructs provide more control and complexity, allowing for more sophisticated programming logic.
Python also offers alternative looping constructs like the for loop, and control statements like break, continue, and pass, to handle different scenarios and enhance your programming.
Common issues with while loops include infinite loops and handling errors. Using try-except blocks within your loops can help manage these issues.
While loops play an integral role in larger scripts or projects, handling tasks like user input or reading data from a file.
Looping Constructs: A Comparison
Loop Type | Use Case |
---|---|
While Loop | When you want to repeat a block of code an unknown number of times until a condition is met. |
For Loop | When you want to iterate over a sequence (like a list, tuple, or string) or when you know the number of times you want to repeat a block of code. |
In conclusion, while loops are a powerful tool in Python programming. Understanding how to use them effectively can greatly enhance your coding skills and efficiency. As you continue your Python journey, don’t forget to explore related concepts like conditional statements and recursion to further expand your programming toolkit.