Learn Python: For Loops With Index (With Examples)
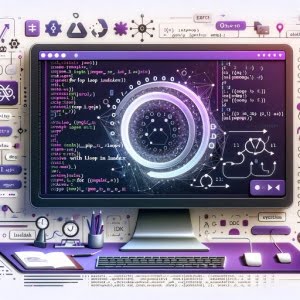
Ever stumbled upon a situation where you needed to iterate over a sequence in Python, but also wanted to keep track of the index? It’s akin to having a bookmark in a book, where the index in a for loop helps you know your position.
This is a common scenario, especially when dealing with complex data structures or when you need to perform certain operations based on the position of the item in the sequence.
In this guide, we’ll walk you through the process of using a for loop with an index in Python. We’ll start from the basics and gradually move towards more advanced techniques. So, whether you’re a beginner or looking to brush up your skills, this guide has got you covered! Let’s dive in and explore the power of ‘for loops with index’ in Python.
TL;DR: How Do I Use a For Loop with an Index in Python?
You can use the
enumerate()
function in a for loop to get both the index and the value. Here’s a simple example:
for index, value in enumerate(['apple', 'banana', 'cherry']):
print(index, value)
# Output:
# 0 apple
# 1 banana
# 2 cherry
In this example, we use the enumerate()
function in a for loop to iterate over a list of fruits. The enumerate()
function adds a counter to the list (or any other iterable), and returns it in a form of enumerate object. This object can then be used in a for loop to get both the index and the value of each item in the sequence.
Intrigued? Stick around for a more detailed explanation and advanced usage scenarios of for loops with an index in Python.
Table of Contents
Using enumerate()
in Python For Loops
The enumerate()
function is a built-in function of Python. Its usefulness comes into play when you need to have a counter to an iterable while iterating over it.
Let’s consider a simple list of fruits:
fruits = ['apple', 'banana', 'cherry']
If we want to print out each fruit along with its index, we can use the enumerate()
function in a for loop like so:
for index, fruit in enumerate(fruits):
print(f'Index: {index}, Fruit: {fruit}')
# Output:
# Index: 0, Fruit: apple
# Index: 1, Fruit: banana
# Index: 2, Fruit: cherry
In this code block, enumerate(fruits)
returns an enumerate object that produces tuples containing an index and a value (the element from the list). The for
loop then iterates over these tuples, and the variables index
and fruit
are set to the index and value of each tuple respectively.
It’s important to note that the index it provides is zero-based, which means it starts counting from 0. If you’re not careful, this can lead to off-by-one errors. We’ll cover how to handle these potential pitfalls in the troubleshooting section.
Advanced Techniques
As you get more comfortable with the basic usage of for loops with an index in Python, you might find yourself needing to use them in more complex scenarios. Let’s delve into some of these advanced uses.
Nested Loops and Indexing
There might be situations where you need to iterate over nested lists or other complex data structures. Here’s how you can use enumerate()
function in nested loops:
nested_list = [['apple', 'banana'], ['carrot', 'beans'], ['duck', 'chicken']]
for outer_index, inner_list in enumerate(nested_list):
for inner_index, item in enumerate(inner_list):
print(f'Outer Index: {outer_index}, Inner Index: {inner_index}, Item: {item}')
# Output:
# Outer Index: 0, Inner Index: 0, Item: apple
# Outer Index: 0, Inner Index: 1, Item: banana
# Outer Index: 1, Inner Index: 0, Item: carrot
# Outer Index: 1, Inner Index: 1, Item: beans
# Outer Index: 2, Inner Index: 0, Item: duck
# Outer Index: 2, Inner Index: 1, Item: chicken
In this example, we have a nested list and we’re using two enumerate()
functions in nested for loops to get the indices and items from the outer and inner lists.
Looping Over Dictionaries with Index
The enumerate()
function can also be used with dictionaries, which can be particularly useful when you need to keep track of the order in which keys or values are iterated over:
fruits_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
for index, (key, value) in enumerate(fruits_dict.items()):
print(f'Index: {index}, Key: {key}, Value: {value}')
# Output:
# Index: 0, Key: apple, Value: 1
# Index: 1, Key: banana, Value: 2
# Index: 2, Key: cherry, Value: 3
In this code block, we’re using enumerate()
on fruits_dict.items()
, which returns a list of dictionary’s items (key-value pairs). The for
loop then iterates over these items, and the variables index
, key
, and value
are set to the index and key-value pair of each item respectively.
Using Index for Conditional Logic
There might be cases where you want to perform different operations depending on the index. Here’s an example:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
if index % 2 == 0:
print(f'Index: {index}, Fruit: {fruit} (even index)')
else:
print(f'Index: {index}, Fruit: {fruit} (odd index)')
# Output:
# Index: 0, Fruit: apple (even index)
# Index: 1, Fruit: banana (odd index)
# Index: 2, Fruit: cherry (even index)
In this example, we’re using the index in a conditional statement to perform different operations for even and odd indices.
These are just a few examples of how you can use for loops with an index in Python for more advanced scenarios. The possibilities are endless, and the more you practice, the more you’ll find how useful this technique can be!
Alternatives: zip()
and List Comprehension
While the enumerate()
function is a powerful tool for using a for loop with an index in Python, there are alternative approaches that can be useful in certain scenarios. Let’s explore two of these: the zip()
function and list comprehension.
Using zip()
to Iterate with Index
The zip()
function can be used to iterate over two or more lists in parallel. If you have a separate list of indices, you can use zip()
to iterate over the indices and items simultaneously:
indices = [0, 1, 2]
fruits = ['apple', 'banana', 'cherry']
for index, fruit in zip(indices, fruits):
print(f'Index: {index}, Fruit: {fruit}')
# Output:
# Index: 0, Fruit: apple
# Index: 1, Fruit: banana
# Index: 2, Fruit: cherry
In this example, zip(indices, fruits)
returns a list of tuples, where each tuple contains an index and a fruit. The for
loop then iterates over these tuples, and the variables index
and fruit
are set to the index and fruit of each tuple respectively.
List Comprehension with enumerate()
List comprehension is a concise way to create lists in Python. You can use enumerate()
in a list comprehension to create a list of tuples, where each tuple contains an index and a value:
fruits = ['apple', 'banana', 'cherry']
index_fruit_pairs = [(index, fruit) for index, fruit in enumerate(fruits)]
print(index_fruit_pairs)
# Output:
# [(0, 'apple'), (1, 'banana'), (2, 'cherry')]
In this code block, the list comprehension [(index, fruit) for index, fruit in enumerate(fruits)]
creates a list of tuples, where each tuple contains an index and a fruit. This can be useful when you need to store the index-fruit pairs for later use.
Both of these alternative approaches have their own advantages. Let’s compare these two approaches in the table below:
Technique | Advantages | Limitations |
---|---|---|
zip() Function | Useful when there are separate lists of indices and items. | Requires separate lists of indices and items. |
List Comprehension with enumerate() | Can be a concise way to create a list of index-item pairs. | Can be less readable than a for loop for complex operations. |
Both methods, the
zip()
function and list comprehension withenumerate()
, have their own strengths and weaknesses. The choice between them depends largely on the specific requirements of your code and the data you’re working with. Always choose the one that makes your code cleaner and easier to understand.
Troubleshooting Python For Loops and Index
For loops with an index in Python can be incredibly useful, especially using the enumerate()
function. However, they are not without their potential pitfalls. Here, we’ll discuss some common issues you may encounter, and provide solutions and workarounds to help you avoid them.
Off-By-One Errors
One of the most common mistakes when using a for loop with an index in Python is the off-by-one error. This occurs when you expect the index to start at 1, but in Python, indices start at 0.
For example, consider the following code:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits, start=1):
print(f'Fruit {index}: {fruit}')
# Output:
# Fruit 1: apple
# Fruit 2: banana
# Fruit 3: cherry
In this example, we’re using the start
parameter of the enumerate()
function to start counting from 1, instead of the default 0. This can be useful when you want the index to represent a position or rank that starts from 1.
Iterating Over None
Another common issue is trying to iterate over None
with a for loop. If the iterable is None
, the enumerate()
function will raise a TypeError
:
for index, value in enumerate(None):
print(index, value)
# Output:
# TypeError: 'NoneType' object is not iterable
To avoid this error, you can check if the iterable is None
before using it in a for loop:
iterable = None
if iterable is not None:
for index, value in enumerate(iterable):
print(index, value)
In this code block, we’re checking if iterable
is None
before using it in a for loop. If iterable
is None
, the for loop is skipped, and no error is raised.
These are just a few examples of the issues you may encounter when using a for loop with an index in Python. By being aware of these potential pitfalls, and knowing how to avoid them, you can write more robust and error-free code.
Understanding Python’s For Loop and Indexing
Before we delve further into the usage of for loops with an index in Python, it’s important to understand the fundamentals of Python’s for loop and the concept of indexing.
Python’s For Loop: A Quick Recap
In Python, a for
loop is used to iterate over a sequence such as a list, tuple, dictionary, string, etc. It’s a control flow statement, which allows code to be executed repeatedly.
Here’s a simple for loop that iterates over a list:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, fruit
is a variable that takes the value of each item in the fruits
list in each iteration of the loop.
Indexing in Python: Zero-Based and More
Indexing in Python is zero-based, which means that the first element has an index of 0, the second element has an index of 1, and so on. You can access an item in a list by its index using the syntax list[index]
.
Here’s an example:
fruits = ['apple', 'banana', 'cherry']
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
print(fruits[2]) # Output: cherry
In this code block, we’re accessing each item in the fruits
list by its index.
When you’re using a for loop with an index in Python, you’re essentially combining these two concepts: you’re iterating over a sequence with a for loop, and you’re keeping track of the index of each item. This can be incredibly useful in many scenarios, as we’ve seen in the previous sections. With a solid understanding of these fundamentals, you’ll be able to use for loops with an index in Python more effectively and efficiently.
Exploring Related Concepts
Once you’ve mastered the use of for loops with an index in Python, there are plenty of related concepts to explore. For example, you might want to learn more about list comprehension, a powerful feature in Python that allows you to create new lists based on existing ones in a concise and readable way.
Or, you might be interested in generator expressions, a high-performance, memory-efficient alternative to list comprehension. These are just a few of the many powerful features Python has to offer for handling sequences and iterating over data.
To deepen your understanding of these and other related concepts, we recommend checking out the following resources:
- Python Loop Techniques: Quick Insights – Dive into Python’s looping best practices for clean and efficient code.
Python Program Termination: Using “exit()” – Discover the role of “exit” in abrupt program termination and its use cases.
Python “while” Loop – Learn the basics of Python’s “while” loop and its continuous execution.
Constructing For Loops in Python – DigitalOcean provides a tutorial on how to construct ‘for’ loops in Python 3.
Python Generators Exercises – W3resource offers exercises related to Python generators for practice and learning.
How to Use Python Conditional Statements – FreeCodeCamp’s straightforward guide on using conditional statements (if, else, elif) in Python.
official Python documentation, as well as resources like Python.org’s tutorial on control flow tools, which includes a section on the for
statement.
Recap: For Loops with Index in Python
In this comprehensive guide, we’ve explored how to use a for loop with an index in Python, starting from the basics and moving on to more advanced techniques. We’ve seen how the enumerate()
function can be a powerful tool for this task, providing a counter to an iterable and making your code cleaner and more efficient.
We also discussed some common issues you may encounter, such as off-by-one errors, and provided solutions and workarounds to help you avoid these pitfalls.
Beyond the enumerate()
function, we explored alternative approaches like using the zip()
function or list comprehension. Each of these methods has its own advantages and can be useful in certain scenarios.
In the end, the best approach depends on your specific needs and the structure of your data. By understanding these different methods and knowing when to use each one, you can write more robust and efficient Python code.