Python Guide: How to Write JSON to a File
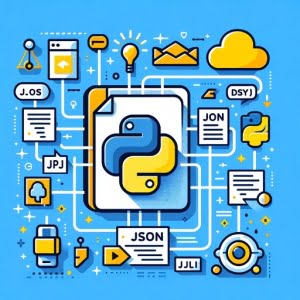
Are you finding it challenging to write JSON data to a file in Python? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can make this process a breeze.
Think of Python’s ability to write JSON to a file as a skilled scribe – it can effortlessly inscribe JSON data into a file. This capability is a powerful tool for tasks such as data serialization, configuration files, and more.
In this guide, we’ll walk you through the process of writing JSON data to a file in Python, from the basics to more advanced techniques. We’ll cover everything from using Python’s built-in json.dump()
function to handling more complex scenarios and even alternative approaches.
So, let’s dive in and start mastering how to write JSON to a file in Python!
TL;DR: How Do I Write JSON to a File in Python?
To write JSON data to a file in Python, you can use the
json.dump()
function. This function allows you to serialize your JSON data and write it into a file.
Here’s a simple example:
import json
data = {'name': 'John', 'age': 30}
with open('data.json', 'w') as f:
json.dump(data, f)
# Output:
# This will create a file named 'data.json' and write the JSON data into it.
In this example, we first import the json module. We then create a dictionary with some data. Using the json.dump()
function, we open a file named ‘data.json’ in write mode and write the JSON data into it.
This is a basic way to write JSON to a file in Python, but there’s much more to learn about handling more complex scenarios and alternative approaches. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics: Python’s json.dump() Function
- Tackling Complex Scenarios: Nested JSON and Large Files
- Exploring Alternative Methods
- Overcoming Hurdles: Common Issues and Their Solutions
- Understanding JSON and File Handling in Python
- Exploring Practical Applications and Further Learning
- Wrapping Up: Mastering the Art of Writing JSON to a File in Python
Understanding the Basics: Python’s json.dump()
Function
Python’s built-in json
module provides a simple and intuitive method to write JSON data to a file – the json.dump()
function. This function serializes Python objects as a JSON formatted stream to a file. Let’s break it down step by step.
Step 1: Import the JSON module
Start by importing the json
module. This module provides the necessary functions to work with JSON data.
import json
Step 2: Prepare Your Data
Next, prepare the data you want to write to the file. This could be a dictionary, a list, or any other Python data type that is serializable to JSON.
data = {'name': 'John', 'age': 30}
In this example, we have a dictionary with two keys: ‘name’ and ‘age’.
Step 3: Write JSON Data to a File
Finally, use the json.dump()
function to write the JSON data to a file. Open the file in write mode ('w'
) and call the json.dump()
function with two arguments: the data and the file object.
with open('data.json', 'w') as f:
json.dump(data, f)
# Output:
# This will create a file named 'data.json' and write the JSON data into it.
In this example, the json.dump()
function serializes the data
dictionary as a JSON formatted stream and writes it to ‘data.json’. The with
statement ensures that the file is properly closed after it is no longer needed.
And there you have it – a basic guide to writing JSON data to a file in Python using the json.dump()
function. While this covers simple use cases, more complex scenarios may require additional steps and considerations. Let’s explore those next.
Tackling Complex Scenarios: Nested JSON and Large Files
While the basic use of the json.dump()
function can handle most scenarios, there are instances where you might need to deal with more complex situations like nested JSON data or large JSON files. Let’s delve into these cases.
Writing Nested JSON Data
Nested JSON data refers to JSON data that contains other JSON data in a hierarchical manner. Python handles this with ease. Let’s take a look at how to write nested JSON data to a file.
import json
# Nested JSON data
nested_data = {
'name': 'John',
'age': 30,
'children': [
{
'name': 'Alice',
'age': 5
},
{
'name': 'Bob',
'age': 7
}
]
}
# Write nested JSON data to a file
with open('nested_data.json', 'w') as f:
json.dump(nested_data, f)
# Output:
# This will create a file named 'nested_data.json' and write the nested JSON data into it.
In the above example, we have a dictionary with a key ‘children’ that contains a list of dictionaries. When we write this dictionary to a file using the json.dump()
function, Python handles the nested data without any issues.
Handling Large JSON Files
For large JSON files, it’s more efficient to write the file in chunks rather than loading the entire file into memory. Python’s json.dump()
function doesn’t directly support this, but we can achieve the same result by writing each chunk of data separately. Here’s an example:
import json
# Large data
large_data = [{'name': 'John', 'age': i} for i in range(1000000)]
# Write large data to a file in chunks
with open('large_data.json', 'w') as f:
for chunk in large_data:
json.dump(chunk, f)
f.write('
')
# Output:
# This will create a file named 'large_data.json' and write the large JSON data into it.
In this example, we have a large list of dictionaries. Instead of writing the entire list to the file at once, we write each dictionary to the file separately. This way, we never have to load the entire list into memory, making the process more memory-efficient.
By understanding how to handle these advanced scenarios, you’ll be better prepared to write JSON data to a file in Python, no matter the complexity of the scenario.
Exploring Alternative Methods
While Python’s built-in json.dump()
function is a powerful tool for writing JSON data to a file, there are other methods you can use, especially when dealing with more complex scenarios or specific requirements. Let’s explore some of these alternatives.
Using Third-Party Libraries: jsonlines
The jsonlines
library is a simple Python library for working with JSONLines data. It’s particularly useful when dealing with large JSON files that need to be read or written line by line.
Here’s an example of how to use jsonlines
to write JSON data to a file:
import jsonlines
# Data to write
data = [{'name': 'John', 'age': i} for i in range(10)]
# Write data to a file using jsonlines
with jsonlines.open('data.jsonl', mode='w') as writer:
for item in data:
writer.write(item)
# Output:
# This will create a file named 'data.jsonl' and write the JSON data into it line by line.
In this example, we use the jsonlines.open()
function to open a file in write mode. We then iterate over our data and write each item to the file line by line using the writer.write()
method.
Advantages:
- Efficient for large files as it writes data line by line, reducing memory usage.
Disadvantages:
- Requires installing an additional third-party library.
Recommendations:
Use jsonlines
when dealing with large files or when you need to write JSON data to a file line by line.
By understanding these alternative approaches, you can choose the best method for your specific needs when writing JSON data to a file in Python.
Overcoming Hurdles: Common Issues and Their Solutions
While Python makes it easy to write JSON data to a file, you may encounter some common issues. Let’s look at a few of these potential problems and how to solve them.
Handling Special Characters
JSON data often includes special characters, which can cause issues if not handled properly. Python’s json.dump()
function automatically escapes special characters, which means it adds a backslash (\
) before special characters. Here’s an example:
import json
# JSON data with special characters
data = {'message': 'Hello, World!
'}
# Write data to a file
with open('data.json', 'w') as f:
json.dump(data, f)
# Output:
# This will create a file named 'data.json' and write the JSON data into it, properly escaping the special characters.
In this example, the json.dump()
function automatically escapes the newline character ().
Dealing with File Permissions
When writing to a file, you may encounter permission errors if Python doesn’t have the necessary write permissions for the file or directory. Here’s how to handle this issue:
import json
# JSON data
data = {'name': 'John', 'age': 30}
# Try to write data to a file
try:
with open('data.json', 'w') as f:
json.dump(data, f)
except PermissionError:
print('Permission denied. Please check your file permissions.')
# Output:
# If Python doesn't have the necessary write permissions, it will print 'Permission denied. Please check your file permissions.'
In this example, we use a try/except block to catch the PermissionError
. If Python doesn’t have the necessary write permissions, it will print a helpful error message.
By understanding and addressing these common issues, you can ensure a smoother experience when writing JSON data to a file in Python.
Understanding JSON and File Handling in Python
To fully grasp how Python writes JSON data to a file, it’s essential to understand the fundamentals of JSON and file handling in Python.
What is JSON?
JSON (JavaScript Object Notation) is a popular data format with a diverse range of applications. It’s human-readable, lightweight, and can represent data structures like objects and arrays. Here’s an example of JSON data:
{
'name': 'John',
'age': 30,
'city': 'New York'
}
In this example, the JSON data is a dictionary with three keys: ‘name’, ‘age’, and ‘city’.
File Handling in Python
Python provides several functions to create, read, update, and delete files. The open()
function is one of the most commonly used functions for file handling. Here’s an example of how to use it:
# Open a file
f = open('data.txt', 'w')
# Write data to the file
f.write('Hello, World!')
# Close the file
f.close()
# Output:
# This will create a file named 'data.txt', write 'Hello, World!' to it, and then close the file.
In this example, we use the open()
function to open a file named ‘data.txt’ in write mode (‘w’). We then write ‘Hello, World!’ to the file using the write()
method and finally close the file with the close()
method.
By understanding the fundamentals of JSON and file handling in Python, you’ll have a solid foundation for writing JSON data to a file.
Exploring Practical Applications and Further Learning
Writing JSON data to a file in Python is a useful skill that has practical applications in various areas. Let’s delve into some of these applications and suggest related topics for further exploration.
Data Serialization for Web APIs
One of the primary uses of writing JSON data to a file is for data serialization in web APIs. Web APIs often use JSON data format for sending and receiving data because it’s lightweight and easy to work with. Here’s an example of how you might write JSON data to a file when working with a web API:
import json
import requests
# Make a request to a web API
response = requests.get('https://api.example.com/data')
# Write the JSON data to a file
with open('api_data.json', 'w') as f:
json.dump(response.json(), f)
# Output:
# This will create a file named 'api_data.json' and write the JSON data from the web API into it.
In this example, we make a GET request to a web API using the requests
library. We then write the JSON response data to a file using the json.dump()
function.
Configuration Files
Another common use of writing JSON data to a file is for creating configuration files. Configuration files are often written in JSON format because it’s easy to read and write. Here’s an example of how you might create a configuration file:
import json
# Configuration data
data = {'setting1': 'value1', 'setting2': 'value2'}
# Write the configuration data to a file
with open('config.json', 'w') as f:
json.dump(data, f)
# Output:
# This will create a file named 'config.json' and write the configuration data into it.
In this example, we create a dictionary with some configuration settings and write it to a file named ‘config.json’.
Further Resources for Mastering Python JSON Handling
If you’re interested in diving deeper into handling JSON data in Python, here are some resources that you might find helpful:
- Python JSON Explained – Learn about the “json” module in Python for efficient JSON data handling.
Python urllib.parse.quote() Explained – Learn how to prepare URLs for HTTP requests with URL encoding techniques.
Writing CSV Files in Python A quick guide on Python’s capabilities for writing data to CSV files.
Python’s Official JSON Documentation provides a comprehensive overview of the
json
module’s capabilities.Real Python’s Guide to JSON Data in Python provides a practical approach to working with JSON data in Python.
Python for Beginners’ JSON Tutorial covers the basics of working with JSON data in Python and is perfect for beginners.
By understanding the practical applications of writing JSON data to a file in Python and exploring further resources, you can continue to expand your Python skills and knowledge.
Wrapping Up: Mastering the Art of Writing JSON to a File in Python
In this comprehensive guide, we’ve delved into the process of writing JSON data to a file in Python, exploring the basics, tackling more advanced scenarios, and even diving into alternative approaches.
We started with the basics, learning how to use Python’s built-in json.dump()
function to write JSON data to a file. We then moved onto more complex scenarios, such as writing nested JSON data and handling large JSON files, providing you with the knowledge to tackle a wide range of situations.
We also explored alternative approaches, such as using the jsonlines
library, giving you a sense of the broader landscape of tools for writing JSON data to a file.
Along the way, we addressed common issues you might encounter, such as handling special characters and dealing with file permissions, equipping you with the solutions to overcome these hurdles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
json.dump() | Simple and direct | Can be memory-intensive for large files |
jsonlines | Efficient for large files | Requires installing a third-party library |
Whether you’re just starting out with writing JSON data to a file in Python or you’re looking to enhance your skills, we hope this guide has provided you with a deeper understanding and practical knowledge of the process.
Writing JSON data to a file in Python is a valuable skill, opening up possibilities for data serialization, creating configuration files, and more. You’re now well equipped to leverage this skill in your Python projects. Happy coding!