Express Framework for Node.js | Setup Guide with npm
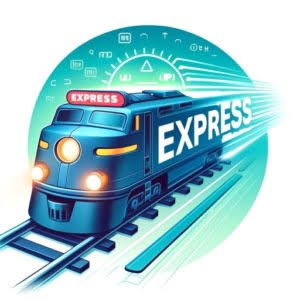
While setting up robust server-side applications for IOFlood, one of the key tools we rely on is Express.js, a popular web framework for Node.js. To help make others familiar with this tool, we have made this guide a beginner-friendly approach to kickstarting your Express projects. Whether you’re new to backend development or looking to enhance your existing Node.js applications, our step-by-step instructions and practical examples will help you harness the power of Express.js.
This guide will walk you through using npm to manage your Express dependencies efficiently. Whether you’re a beginner just starting out, or an experienced developer looking to refine your workflow, this article is designed to provide you with a comprehensive understanding of npm and Express. From basic installation to advanced features, we’ll cover everything you need to know to make the most out of Express in your Node.js projects.
Let’s dive into the world of server-side development together and unlock the potential of Express with npm.
TL;DR: How Do I Start Using Express with npm?
To use Express in your Node.js project, you need to install it using npm and the command,
npm install express
. Then, use Express to create a new application or use,npm install -g express-generator
ot generate a basic Express application structure.
Here’s a quick command to get you started:
npm install express --save
# Output:
# 'express': '^4.17.1' added to your package.json dependencies
This command installs Express and adds it to your project’s dependencies. It’s a straightforward way to begin building web applications with Express in Node.js. The --save
flag ensures that Express is added to your project’s package.json
file, making it easier to manage your project’s dependencies.
This is just the beginning of what you can do with Express and npm. There’s a whole world of possibilities for building efficient, scalable web applications. > Keep reading for more detailed instructions, advanced configurations, and best practices.
Table of Contents
Kickstarting Your Journey with Express
Embarking on the path of web development with Node.js brings you to the doorstep of one of its most powerful and popular frameworks: Express. Leveraging Express in your Node.js projects can significantly streamline the development process, making it an essential skill for beginners. This section will guide you through the initial steps of installing Express with npm and crafting a simple yet functional Express application.
Installing Express
The first step in utilizing Express is to install it into your project using npm, Node.js’s package manager. This process is straightforward and sets the foundation for your project.
npm init -y
npm install express
# Output:
# 'express' dependency added to your package.json file
The npm init -y
command creates a package.json
file in your project directory, a crucial file that tracks your project’s dependencies. Following this, npm install express
adds Express as a dependency, making it available for use in your project.
Creating a ‘Hello, World!’ Application
With Express installed, you’re ready to create your first application. A ‘Hello, World!’ app is a traditional starting point, demonstrating the basic structure of an Express application.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Application running on port 3000');
});
# Output:
# Application running on port 3000
In this example, you create an Express application that listens on port 3000. When a user navigates to the root URL (/
), the application responds with ‘Hello, World!’. This simple application demonstrates the core concepts of Express: application creation, routing, and listening on a port. It’s a solid foundation upon which more complex applications can be built.
By following these steps, you’ve successfully set up your project with npm, installed Express, and created a basic application. This process showcases the simplicity and power of Express, making it an excellent starting point for beginners diving into web development with Node.js.
Elevating Your Express Applications
Once you’ve grasped the basics of Express by creating a simple application, it’s time to explore its more advanced capabilities. This section delves into intermediate-level features of Express, such as middleware, routing, and serving static files, which are essential for developing more complex and scalable web applications.
Implementing Middleware
Middleware functions are at the heart of Express applications, offering a way to execute code, make modifications to the request and response objects, and end the request-response cycle. Here’s how you can implement a simple logging middleware that prints the current path and request method to the console.
const express = require('express');
const app = express();
app.use((req, res, next) => {
console.log(`Received ${req.method} request for '${req.path}'`);
next();
});
app.get('/', (req, res) => {
res.send('Middleware in action!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
# Output:
# Server running on port 3000
# Received GET request for '/'
This code snippet demonstrates the power of middleware for logging purposes. By calling next()
, it passes control to the next middleware function in the stack, or to the route handler if no more middleware exists. This example illustrates how middleware can be utilized for a variety of tasks, including logging, authentication, and more.
Mastering Routing
Routing is another powerful feature of Express that allows you to manage different HTTP requests at different URLs. Express provides a robust set of features to handle routing effectively.
app.get('/about', (req, res) => {
res.send('About Page');
});
app.post('/submit', (req, res) => {
res.send('Form Submitted');
});
In this simple example, we define two routes: /about
for GET requests and /submit
for POST requests. This demonstrates how Express can handle different types of requests and paths, making it incredibly flexible for web application development.
Serving Static Files
A common requirement for web applications is to serve static files such as images, CSS, and JavaScript files. Express simplifies this process with its built-in middleware.
app.use(express.static('public'));
By using the express.static
middleware and specifying a directory (in this case, ‘public’), Express will automatically serve the files located in that directory. This functionality is crucial for delivering assets that are part of your application’s user interface.
Through the exploration of middleware, routing, and static files, we’ve seen how Express can be used to build more complex and feature-rich web applications. These intermediate-level features are essential for developers looking to leverage the full power of Express in their projects.
Expanding Express with NPM Scripts
When you’ve reached a level of comfort with npm and Express, it’s time to explore the landscape of alternative approaches that can further enhance your applications. NPM scripts stand out as a powerful ally, automating tasks and streamlining your development process. Moreover, integrating Express with other Node.js frameworks can introduce a new level of complexity and capability to your projects.
Automating with NPM Scripts
NPM scripts can transform your workflow by automating repetitive tasks such as testing, linting, and building your application. Here’s an example of how to set up a simple npm script for starting your Express server.
"scripts": {
"start": "node app.js"
}
# Output:
# Server running on port 3000
In your package.json
file, under the scripts
section, you can define a start
command that executes node app.js
. Running npm start
from your terminal will now start your Express application, as defined in app.js
. This example illustrates the simplicity and power of npm scripts for managing and executing common tasks.
Leveraging Other Node.js Frameworks
While Express is a robust solution for many applications, sometimes the requirements of your project might lead you to consider other Node.js frameworks. Integrating Express with frameworks like Koa or Fastify can offer different advantages, such as improved performance or a more flexible middleware system.
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = 'Hello from Koa';
});
app.listen(3000);
# Output:
# Application running on port 3000
This Koa application example demonstrates an alternative to Express for handling HTTP requests. Koa provides a more modern and flexible approach to middleware, allowing you to use async functions for more control over the request/response lifecycle. By exploring other frameworks like Koa, you can tailor your stack to best fit the needs of your application.
Exploring these alternative approaches and tools can significantly enhance the capabilities of your Express applications. Whether through the automation of npm scripts or the integration with other Node.js frameworks, there are numerous paths to elevate your web development projects. This expert-level exploration not only broadens your technical toolkit but also opens up new possibilities for innovation and efficiency in your applications.
When diving into the world of Node.js and Express, it’s not uncommon to encounter a few bumps along the way. This section aims to shed light on some of the typical issues developers face when using Express with npm, offering practical solutions and tips to navigate these challenges effectively.
Dependency Conflicts
One of the first hurdles you might encounter involves dependency conflicts. As your project grows, so does the list of dependencies, increasing the risk of incompatible versions.
To identify and resolve dependency conflicts, you can use the npm list
command to see the installed versions of all packages and their dependencies.
npm list
# Output:
# [email protected]
# [email protected]
In this output, npm list
reveals the versions of all installed packages. If you notice any conflicts, consider updating the conflicting packages or your project’s dependencies to compatible versions.
Versioning Issues
Another common challenge involves managing package versions. It’s crucial to keep your project’s dependencies up to date to avoid security vulnerabilities and ensure compatibility.
To update a specific package, such as Express, you can use the npm update
command:
npm update express
# Output:
# express: 4.17.1 updated to 4.18.0
This command updates Express to the latest compatible version, addressing potential security issues and adding new features. Regularly updating your dependencies can help mitigate versioning issues.
Deployment Challenges
Deploying an Express application can also present its own set of challenges, from environment configuration to server compatibility.
One common issue is ensuring that your application listens on the correct port when deployed. This can be managed by setting an environment variable for the port number:
const port = process.env.PORT || 3000;
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
# Output:
# Server running on port 3000
In this code snippet, the application listens on the port number provided by the process.env.PORT
environment variable, defaulting to 3000 if it’s not set. This approach allows for flexible deployment across different environments.
Encountering issues when using Express with npm is a natural part of the development process. By understanding common pitfalls and how to address them, you can ensure a smoother experience as you build and deploy your Express applications. Remember, the key to successful troubleshooting is regular maintenance, thorough testing, and staying updated on best practices.
Understanding Node.js and npm
Before diving into the world of Express, it’s crucial to grasp the basics of Node.js and npm (Node Package Manager), as they form the backbone of your development environment when working with Express. Node.js is a runtime environment that allows you to execute JavaScript on the server side, while npm is its package manager, facilitating the management of packages or modules that your project depends on.
Node.js: The JavaScript Runtime
Node.js revolutionized how JavaScript is used, taking it from a browser-based language to one that can run on servers. This shift opened up new avenues for developing web applications.
console.log('Hello from Node.js');
# Output:
# Hello from Node.js
This simple example demonstrates how Node.js executes JavaScript code outside of a browser. Running this code in a Node.js environment will print ‘Hello from Node.js’ to the console, showcasing the ability to run JavaScript server-side.
npm: Managing Dependencies
npm plays a pivotal role in managing the libraries and packages your project needs. It interacts with the npm registry, a vast collection of open-source JavaScript tools, to install, update, and manage packages.
npm init -y
# Output:
# Creates a package.json file with default values
The npm init -y
command creates a package.json
file in your project directory, which tracks your project’s dependencies. This file is essential for managing the packages your application requires, ensuring that anyone working on the project can install the same dependencies.
Express: Simplifying Web Development
Express is a web application framework for Node.js, designed to simplify the process of building web applications and APIs. It provides a thin layer of fundamental web application features, without obscuring Node.js features.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to Express!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
# Output:
# App listening on port 3000
In this example, we create a basic Express application that listens on port 3000. When a user navigates to the root URL (/
), the application responds with ‘Welcome to Express!’. This demonstrates the simplicity and efficiency of setting up a web server with Express, highlighting its role as a powerful tool for web development.
By understanding the fundamentals of Node.js, npm, and how Express leverages these technologies, developers can more effectively utilize Express in their projects. This foundational knowledge is crucial for navigating the npm ecosystem and maximizing the capabilities of Express in your web applications.
Best Practices for Scaling Express
As your proficiency with Express and npm grows, the horizon of possibilities expands. The journey from developing simple web applications to architecting robust, scalable systems beckons. This section explores leveraging Express and npm in larger-scale applications, delving into microservices, security best practices, and performance optimization. It’s a roadmap for taking your Express applications beyond the basics, preparing them to meet the demands of modern web development.
Embracing Microservices Architecture
Microservices architecture represents a method of developing software systems that are highly maintainable, testable, and loosely coupled. Express, with its minimalistic and unopinionated framework, serves as an excellent foundation for building microservices.
const express = require('express');
const app = express();
app.get('/service', (req, res) => {
res.json({ service: 'Microservice Example', status: 'Running' });
});
app.listen(3000, () => {
console.log('Microservice running on port 3000');
});
# Output:
# Microservice running on port 3000
This code snippet demonstrates a basic microservice that returns the status of a service. By breaking down applications into smaller, manageable pieces, you can improve scalability and flexibility, making it easier to develop and deploy new features.
Prioritizing Security
Security is paramount, especially as your applications grow in complexity and scale. Implementing security best practices from the start can safeguard your application against common vulnerabilities.
const helmet = require('helmet');
app.use(helmet());
# Output:
# Application now has improved security headers
Utilizing middleware like helmet
can enhance your application’s security by setting various HTTP headers. It’s a simple yet effective step towards securing your Express applications.
Optimizing Performance
Performance optimization is crucial for maintaining a smooth user experience as your application scales. Techniques such as caching and load balancing are vital.
const redis = require('redis');
const client = redis.createClient();
app.get('/cache', (req, res) => {
client.get('data', (error, data) => {
if (error) throw error;
if (data != null) {
res.send(data);
} else {
// Fetch data and set to cache
}
});
});
# Output:
# Efficient data retrieval with caching
This example shows how integrating a caching mechanism with Redis can significantly reduce data retrieval times, enhancing the overall performance of your application.
Further Resources for Mastering Express and npm
To continue your journey in mastering Express and npm, here are three handpicked resources:
- Express.js Official Documentation – The best place to start, offering comprehensive guides and API references.
Node.js and npm Documentation – Essential reading for understanding the npm ecosystem.
Building Scalable Web Applications with Node.js and Express – A resource for diving deeper into building scalable applications with Express.
These resources will provide you with a wealth of information and practical examples to further your understanding and skills in working with Express and npm, setting you on a path towards developing sophisticated and scalable web applications.
Wrapping Up: Using Express with NPM
In this comprehensive guide, we’ve navigated through the essentials of using Express with npm, laying the foundation for robust web application development with Node.js. From setting up your first Express application to exploring advanced features and tackling common issues, this guide has aimed to equip you with the knowledge needed to confidently use Express in your projects.
We began with the basics, demonstrating how to install Express using npm and create a simple ‘Hello, World!’ application. This initial step is crucial for understanding how Express integrates into the Node.js ecosystem and serves as the starting point for web application development.
Moving forward, we delved into more advanced topics, such as implementing middleware, handling routing, and serving static files. These features are vital for building scalable and maintainable web applications, showcasing Express’s flexibility and power.
We also explored alternative approaches and tools that can complement Express, such as npm scripts for automation and integrating with other Node.js frameworks. These expert-level insights aim to broaden your development horizons, encouraging you to experiment with new techniques and tools.
Feature | Importance | Use Case |
---|---|---|
Middleware | High | Custom functionality between request and response |
Routing | Critical | Managing different HTTP requests |
Static Files | Essential | Serving images, CSS, and JavaScript |
Whether you’re just starting out with Express and npm or looking to deepen your understanding, we hope this guide has provided you with valuable insights and practical examples. Express, combined with npm, offers a powerful and efficient approach to web application development, enabling you to build everything from small projects to large-scale applications.
With the fundamentals covered and advanced techniques explored, you’re well-equipped to take your Express applications to the next level. Remember, continuous learning and experimentation are key to mastering web development. Happy coding!