Writing Comments in Bash | Script Documentation Guide
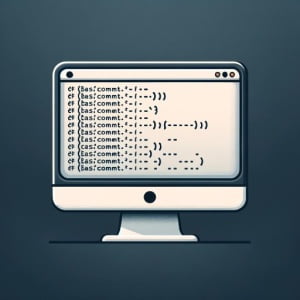
Have you ever found yourself lost in your own Bash scripts, struggling to understand what each line does? You’re not alone. Many developers find it challenging to navigate through their code, especially as it grows more complex. Think of Bash comments as your personal guide, helping you and others understand your code better.
Comments in Bash are like breadcrumbs in a forest. They leave a trail for you and others to follow, making your scripts more readable and maintainable. They’re an essential tool in any Bash programmer’s toolkit, regardless of their skill level.
In this guide, we’ll walk you through the process of using comments in Bash, from the basics to more advanced techniques. We’ll cover everything from adding simple one-line comments to handling multi-line comments and even using comments for debugging.
So, let’s dive in and start mastering Bash comments!
TL;DR: How Do I Comment in Bash?
To comment in Bash, you simply start the line with a
'#'
. For instance,# This is a comment
is a valid comment in Bash.
Here’s a simple example:
# This is a top-level comment
echo 'Hello, World!' # This is an inline comment
# Output:
# 'Hello, World!'
In this example, we’ve used a top-level comment to describe the overall purpose of the script, and an inline comment to provide additional context for a specific line of code. The echo
command prints ‘Hello, World!’, and the inline comment explains what this line does.
This is just a basic way to use comments in Bash, but there’s much more to learn about making your scripts more readable and maintainable. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- The Basics of Bash Comments
- Multi-Line Bash Comments and Debugging
- Exploring Alternative Commenting Techniques in Bash
- Troubleshooting Bash Comments: Common Issues and Solutions
- The Role of Comments in Scripting and Programming
- The Power of Comments in Large Bash Scripts and Projects
- Wrapping Up: Mastering Bash Comments
The Basics of Bash Comments
Bash comments are an integral part of writing clean and understandable code. They provide important context and explanation for what your code is doing, which is invaluable when you or others are revisiting your scripts.
The Importance of Comments in Code
Comments are not just for you. They’re for anyone who might need to read your code, including your future self. Good commenting practice can save hours of deciphering code, making it easier to maintain and debug.
How to Use Comments in Bash
In Bash, a comment starts with the hash symbol (#
). Anything after #
on that line is considered a comment and is ignored by the Bash interpreter. Here’s an example:
# This is a comment in Bash
# Output:
# (No output, as comments are not executed)
In this example, the line starting with #
is a comment. It’s not executed when you run the script, so it has no impact on your code’s functionality—it’s purely for human readers.
Where to Use Comments
You can use comments anywhere in your script. It’s common to put a comment at the top of a file to explain the script’s purpose, and then use inline comments throughout the script to explain specific lines of code. Here’s an example:
# This script prints a greeting
echo 'Hello, World!' # Prints 'Hello, World!'
# Output:
# 'Hello, World!'
The Pitfalls of Not Using Comments
Without comments, your code can become difficult to understand, especially as it grows more complex. This can lead to errors and make your code harder to maintain and debug. So, it’s always a good idea to comment your code, even if it seems simple at the time.
Multi-Line Bash Comments and Debugging
As your Bash scripts become more complex, you might find yourself needing to comment out multiple lines of code or use comments for debugging. Here’s how you can do that.
Handling Multi-Line Comments
Bash doesn’t have a specific syntax for multi-line comments like some other languages, but you can use a trick with the :
command and a ‘here document’ to achieve the same effect. Here’s how:
: << 'END_COMMENT'
This is a
multi-line comment
in Bash
END_COMMENT
# Output:
# (No output, as comments are not executed)
In this example, :
is a no-op (no operation) command, and ‘END_COMMENT’ is an arbitrary string that marks the end of the comment. Everything between << 'END_COMMENT'
and END_COMMENT
is treated as a comment.
Using Comments for Debugging
Comments can also be useful for debugging your scripts. For example, you can comment out a section of your code to isolate a problem. Here’s an example:
# This is the original script
# echo 'This line is causing problems'
echo 'This line is fine'
# Output:
# 'This line is fine'
In this example, we’ve commented out the first echo
command because it’s causing problems. This allows us to run the rest of the script without executing the problematic line.
Exploring Alternative Commenting Techniques in Bash
While the traditional method of using the #
symbol is the most common way to create comments in Bash, there are alternative approaches that can offer more flexibility, especially for complex scripts.
Using ‘Here Documents’ for Comments
‘Here Documents’ is a type of redirection in Bash that allows you to pass multiple lines of input to a command. You can use this feature to create multi-line comments. Here’s an example:
: << 'COMMENT'
This is a
multi-line comment
using a here document
COMMENT
# Output:
# (No output, as comments are not executed)
In this code block, :
is a no-op (no operation) command, and ‘COMMENT’ is an arbitrary string that marks the end of the comment. Everything between << 'COMMENT'
and COMMENT
is treated as a comment.
Advantages and Disadvantages of Using ‘Here Documents’
The main advantage of using ‘here documents’ for comments is that they allow you to easily comment out large blocks of code, which can be very useful for debugging. They also allow you to include special characters in your comments without needing to escape them.
On the downside, ‘here documents’ can make your scripts more complex and harder to read if used excessively. They’re also not as widely recognized as traditional comments, so they may confuse other developers who are not familiar with them.
Recommendations
While ‘here documents’ can be a powerful tool for creating comments in Bash, they’re best used sparingly and for specific purposes, such as commenting out large blocks of code or including special characters in your comments. For most cases, traditional comments using the #
symbol will be the most readable and maintainable option.
Troubleshooting Bash Comments: Common Issues and Solutions
While using comments in Bash is straightforward, there are a few potential pitfalls that you might encounter. Let’s explore some of these common issues and how to resolve them.
Syntax Errors
While comments are ignored by the Bash interpreter, incorrect syntax can still cause errors. For example, if you forget to close a ‘here document’, you’ll get a syntax error.
: << 'COMMENT'
This is a
multi-line comment
# Forgot to close the comment
# Output:
# bash: warning: here-document at line 1 delimited by end-of-file (wanted `COMMENT')
In this example, we forgot to include the closing ‘COMMENT’, which caused a syntax error. To fix this, we simply need to add the closing ‘COMMENT’.
: << 'COMMENT'
This is a
multi-line comment
COMMENT
# Output:
# (No output, as comments are not executed)
Inline Comments and Commands
When using inline comments, be careful not to accidentally comment out part of your command. For example:
echo 'Hello, World!' # This comment is fine
echo 'Hello, # World!' # This comment causes a problem
# Output:
# 'Hello, World!'
# 'Hello, '
In the second echo
command, the #
symbol starts a comment, which causes the ‘World!’ part to be ignored. To fix this, we can either move the comment to a new line or use quotes to ensure the #
is treated as part of the string.
echo 'Hello, World!' # This comment is fine
echo 'Hello, # World!'
# Output:
# 'Hello, World!'
# 'Hello, # World!'
Remember, comments in Bash can be a powerful tool for making your scripts more readable and maintainable, but like any tool, they need to be used correctly. By understanding these common issues and how to avoid them, you can use comments effectively and avoid unnecessary bugs in your scripts.
The Role of Comments in Scripting and Programming
Comments are an essential part of any programming language, and Bash is no exception. They serve a critical role in making your code understandable to others and your future self.
Enhancing Code Readability
Imagine trying to understand a complex mathematical equation without any explanation. You’d likely get lost in the myriad of symbols and operations. The same applies to code. Without comments, your code can become a labyrinth that’s hard to navigate and understand.
Here’s an example of a Bash script without comments:
for i in {1..10}; do
if (( i % 2 == 0 )); then
echo $i
fi
done
# Output:
# 2
# 4
# 6
# 8
# 10
This script prints the even numbers between 1 and 10. But without any comments, it’s not immediately clear what this script does.
Now, let’s add some comments:
# This script prints the even numbers between 1 and 10
for i in {1..10}; do
# If i is divisible by 2
if (( i % 2 == 0 )); then
echo $i # Print i
fi
done
# Output:
# 2
# 4
# 6
# 8
# 10
With the added comments, the purpose of the script and the logic behind it become much clearer.
Facilitating Code Maintenance
Comments are also crucial for maintaining your code. They provide context for why certain decisions were made, which can be invaluable when you’re revisiting your scripts after some time or when someone else is trying to understand your code. A well-commented script can save hours of debugging and deciphering, making your code much easier to maintain and update.
The Power of Comments in Large Bash Scripts and Projects
As your Bash scripts grow and evolve into larger projects, comments become even more crucial. They serve as a roadmap, guiding you and others through your code, making it easier to understand, maintain, and debug.
Relevance of Comments in Larger Scripts
In larger scripts or projects, the complexity of the code increases. Here, comments act as crucial signposts that explain the flow and logic of your code. They can help break down complex procedures into understandable chunks, making your script accessible not only to you but also to other developers who might work on your project.
Exploring Related Concepts
Mastering comments is just one part of becoming proficient in Bash scripting. There are other related concepts that you might find useful, such as Bash scripting best practices, script debugging, and understanding the importance of code readability and maintainability.
Further Resources for Mastering Bash Comments
If you’re interested in diving deeper into Bash comments and related topics, here are some resources that you might find helpful:
- Advanced Bash-Scripting Guide: This is an in-depth guide to Bash scripting, including a detailed section on comments.
Bash Guide for Beginners: This guide covers the basics of Bash scripting, including how to use comments effectively.
Google’s Shell Style Guide: This style guide by Google provides best practices for writing clean and maintainable Bash scripts, including the use of comments.
Remember, the key to mastering Bash comments—or any coding concept, for that matter—is practice. So, don’t be afraid to get your hands dirty and write some code. Happy scripting!
Wrapping Up: Mastering Bash Comments
In this comprehensive guide, we’ve delved into the world of Bash comments, an essential tool for making your Bash scripts more readable and maintainable.
We began with the basics, learning how to add simple one-line comments to our scripts. We then explored more advanced techniques, such as handling multi-line comments and using comments for debugging. Along the way, we tackled common issues that you might face when using comments in Bash, such as syntax errors, and provided solutions and workarounds for each issue.
We also looked at alternative approaches to commenting in Bash, such as using ‘here documents’. We discussed the advantages and disadvantages of these methods, and provided recommendations on when to use them.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
# for single-line comments | Simple and easy to use | Can be tedious for multi-line comments |
: << 'END_COMMENT' for multi-line comments | Useful for commenting out large blocks of code | Can make scripts more complex if used excessively |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of how to use comments effectively in Bash.
With a good grasp of Bash comments, you’re now equipped to write cleaner, more maintainable scripts. Happy scripting!