Python Matplotlib Scatter Plot: Mastering plt.scatter
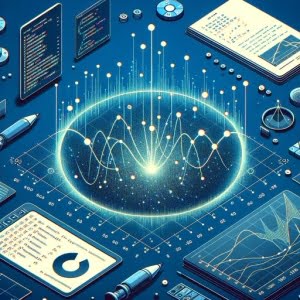
Are you finding it challenging to create scatter plots in Python using matplotlib’s plt.scatter function? Don’t worry, you’re not alone. Many data enthusiasts and Python learners often find themselves in a similar situation when they first start out.
Think of plt.scatter as your personal artist, ready to create beautiful scatter plots from your data. It’s a powerful tool that, once mastered, can help you make sense of complex data sets and uncover hidden patterns.
In this guide, we will walk you through the process of creating scatter plots using plt.scatter, from the basics to more advanced techniques. Whether you’re a beginner just starting out with data visualization in Python, or an intermediate user looking to refine your skills, this guide has something for you.
So, let’s dive in and start plotting!
TL;DR: How Do I Create a Scatter Plot in Python with Matplotlib’s plt.scatter?
You can create a scatter plot in Python with matplotlib’s plt.scatter function as follows:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
plt.scatter(x, y)
plt.show()
# Output:
# Displays a scatter plot with x and y data points.
This simple block of code will create a scatter plot of the x and y data. The plt.scatter(x, y)
function plots the x and y data points as a scatter plot, and plt.show()
displays the plot. It’s as simple as that!
But don’t stop here. This is just the tip of the iceberg. Read on for more detailed instructions, advanced usage, and to truly master creating scatter plots with matplotlib’s plt.scatter in Python.
Table of Contents
- Creating Scatter Plots: The Basics
- Customizing Scatter Plots: Size, Color, and Shape
- Alternative Methods for Scatter Plots in Matplotlib
- Troubleshooting plt.scatter: Common Issues and Solutions
- Unraveling Scatter Plots and Matplotlib
- plt.scatter: A Key Player in Data Analysis and Machine Learning
- Expanding Your Horizons: Other Plots and Libraries
- Wrapping Up: Mastering Scatter Plots with plt.scatter
Creating Scatter Plots: The Basics
Let’s start with the basics of creating scatter plots using matplotlib’s plt.scatter function. The plt.scatter function is a versatile function that allows you to create scatter plots in Python quickly. The basic syntax of plt.scatter is as follows:
plt.scatter(x, y)
In this syntax, x
and y
are arrays or lists of numerical data. These represent the x and y coordinates of the data points in the scatter plot.
Here’s a simple example of how to create a scatter plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
plt.scatter(x, y)
plt.show()
# Output:
# Displays a scatter plot with x and y data points.
In this example, we first import the matplotlib.pyplot module as plt. Then, we define two lists of numbers for x and y. These numbers represent the coordinates of the data points in the scatter plot. The plt.scatter(x, y)
function plots these points as a scatter plot, and plt.show()
displays the plot.
This is the most basic use of plt.scatter to create a scatter plot. However, plt.scatter comes with several parameters that allow you to customize the appearance and behavior of your scatter plots. We’ll explore these in the next section.
Customizing Scatter Plots: Size, Color, and Shape
Once you’ve mastered the basics of creating scatter plots using plt.scatter, it’s time to explore the advanced features that make this function so powerful.
One of the key advantages of plt.scatter is its ability to customize the size, color, and shape of the markers in your scatter plot. This can be extremely helpful when you’re trying to differentiate between different data points or highlight certain aspects of your data.
Changing the Size of Markers
You can change the size of the markers in your scatter plot using the ‘s’ parameter in the plt.scatter function. The ‘s’ parameter accepts a scalar or an array, with each value defining the size of each marker. Here’s an example:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
sizes = [20, 50, 100, 200, 500]
plt.scatter(x, y, s=sizes)
plt.show()
# Output:
# Displays a scatter plot with varying marker sizes.
In this example, the sizes of the markers vary according to the values in the ‘sizes’ list.
Changing the Color of Markers
To change the color of the markers, you can use the ‘c’ parameter. The ‘c’ parameter can take a single color format string, or a sequence of color specifications of length N. Here’s how you can do it:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
colors = ['red', 'green', 'blue', 'yellow', 'purple']
plt.scatter(x, y, c=colors)
plt.show()
# Output:
# Displays a scatter plot with different colored markers.
In this example, each marker has a different color specified by the ‘colors’ list.
Changing the Shape of Markers
Finally, you can change the shape of the markers using the ‘marker’ parameter. The ‘marker’ parameter accepts a variety of string values representing different marker shapes. Here’s an example of how to use it:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
plt.scatter(x, y, marker='^')
plt.show()
# Output:
# Displays a scatter plot with triangle-shaped markers.
In this example, the ‘^’ string value makes the markers appear as triangles.
By customizing the size, color, and shape of the markers, you can create more informative and visually appealing scatter plots. This is just a glimpse of what you can do with plt.scatter. In the next section, we’ll explore alternative methods for creating scatter plots in matplotlib.
Alternative Methods for Scatter Plots in Matplotlib
While plt.scatter is a powerful tool for creating scatter plots in matplotlib, it’s not the only method available. Another popular approach is using the plt.plot function with an ‘o’ marker. This method can be a handy alternative when you want to create quick and straightforward scatter plots.
Using plt.plot for Scatter Plots
Here’s how you can create a scatter plot using plt.plot:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
plt.plot(x, y, 'o')
plt.show()
# Output:
# Displays a scatter plot similar to plt.scatter.
In this example, the ‘o’ character in the plt.plot function creates a scatter plot. The resulting plot is similar to what you would get with plt.scatter.
Comparing plt.scatter and plt.plot
While both plt.scatter and plt.plot can create scatter plots, there are some differences between the two methods. plt.scatter allows for more customization, such as changing the size, color, and shape of the markers. On the other hand, plt.plot is simpler and can be faster for large datasets, but it doesn’t offer the same level of customization.
In conclusion, the choice between plt.scatter and plt.plot depends on your specific needs. If you want to create a simple scatter plot quickly, plt.plot might be the way to go. But if you need more control over the appearance of your scatter plot, plt.scatter is the better option.
Troubleshooting plt.scatter: Common Issues and Solutions
While plt.scatter is a powerful function for creating scatter plots in matplotlib, it’s not without its quirks. Here we’ll discuss some common issues you may encounter when using plt.scatter and how to resolve them.
Mismatched Array Sizes
One of the most common errors when using plt.scatter is having mismatched array sizes for the x and y data. The x and y arrays must have the same length. If they don’t, plt.scatter will throw a ValueError. Here’s an example:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16]
try:
plt.scatter(x, y)
plt.show()
except ValueError as e:
print(f'Error: {e}')
# Output:
# Error: x and y must be the same size
In this example, the x array has 5 elements while the y array has only 4. This mismatch in size causes plt.scatter to throw a ValueError. The solution is to ensure that your x and y arrays have the same length.
Invalid Values in Data
Another common issue is having invalid values (like NaN or inf) in your data. plt.scatter can handle NaN values by not plotting them, but inf values will cause an OverflowError. Here’s how you can handle this:
import matplotlib.pyplot as plt
import numpy as np
x = [1, 2, 3, 4, np.inf]
y = [1, 4, 9, 16, 25]
try:
plt.scatter(x, y)
plt.show()
except OverflowError as e:
print(f'Error: {e}')
# Output:
# Error: Range of x-axis values is too large to be rendered.
In this example, the x array contains an inf value, which causes plt.scatter to throw an OverflowError. The solution is to clean your data and replace or remove inf values before plotting.
These are just a few examples of the issues you might encounter when using plt.scatter. However, with a good understanding of the function and careful preparation of your data, you can avoid these pitfalls and create beautiful, informative scatter plots.
Unraveling Scatter Plots and Matplotlib
Before we delve deeper into plt.scatter, it’s important to understand the fundamentals of scatter plots and the matplotlib library. These two elements form the bedrock of data visualization in Python.
Understanding Scatter Plots
A scatter plot is a type of data visualization that uses dots to represent the values obtained for two different variables – one plotted along the x-axis and the other plotted along the y-axis. Scatter plots are particularly useful when you want to explore relationships between two numerical variables, and they can be instrumental in spotting trends, correlations, and outliers in your data.
Exploring Matplotlib
Matplotlib is a plotting library for the Python programming language. It provides an object-oriented API for embedding plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK. Matplotlib is also a popular choice for creating static, animated, and interactive visualizations in Python.
The Role of Scatter Plots in Data Visualization
Data visualization is a critical component of data analysis. It allows us to visually access complex data sets and find patterns, trends, and insights that might not be evident from raw data. Scatter plots, with their ability to illustrate the relationship between two variables, play a pivotal role in this process.
For example, in the context of machine learning, scatter plots can help visualize the distribution of data points across different classes, which can be crucial in tasks like classification or clustering. Similarly, in exploratory data analysis, scatter plots can help identify correlations between variables, detect outliers, or suggest potential transformations of variables.
In the next section, we’ll discuss how plt.scatter can be used beyond basic scatter plots and its applications in data analysis and machine learning.
plt.scatter: A Key Player in Data Analysis and Machine Learning
The plt.scatter function is more than just a tool for creating scatter plots. It’s a powerful ally in the world of data analysis and machine learning. By providing a visual representation of data, scatter plots can reveal patterns and trends that might be missed in tabular data. This can be incredibly valuable when exploring a new dataset or presenting your findings to others.
For instance, in machine learning, plt.scatter can be used to visualize the distribution of data points across different classes in a classification problem. This can help identify imbalances in the dataset and inform strategies for addressing them. Similarly, in exploratory data analysis, scatter plots can help identify correlations between variables, which can be instrumental in feature selection.
import matplotlib.pyplot as plt
from sklearn.datasets import make_blobs
# create a sample data
X, y = make_blobs(n_samples=300, centers=4, random_state=0, cluster_std=1.0)
# create a scatter plot
plt.scatter(X[:, 0], X[:, 1], c=y, s=50, cmap='viridis')
plt.show()
# Output:
# Displays a scatter plot with data points colored by their class.
In this example, we’re using plt.scatter to visualize a synthetic dataset created with sklearn’s make_blobs function. The scatter plot provides a clear picture of the distribution of data points across the four classes.
Expanding Your Horizons: Other Plots and Libraries
While plt.scatter is a powerful tool, it’s just one of many in the matplotlib library. Depending on your specific needs, you might find other types of plots, such as line plots (plt.plot), bar plots (plt.bar), or histograms (plt.hist), more suitable. Each type of plot has its strengths and is best suited to certain kinds of data and specific tasks.
Beyond matplotlib, there are also other data visualization libraries in Python that you might find useful. Libraries like Seaborn, Plotly, and Bokeh offer a wealth of additional features and plot types, along with more modern aesthetics. Exploring these libraries can open up new possibilities for your data visualizations.
Further Resources for Plotting
To continue your data exploration and visualization journey, we suggest you Click Here, for a complete guide on Matplotlib insights like color mapping and custom color schemes.
We would also like to present some valuable resources to aid you in mastering the art of graphical data representation using Python’s plotting libraries:
- Visualizing Data with Python: A Guide to Python Visualizers – Dive into the world of interactive data visualization in Python.
Matplotlib Histograms Made Easy – Learn how to create histograms with Matplotlib for data distribution analysis.
Exploring Big Data Using Python – Discover techniques for visualising and exploring big data through Python.
Seaborn Python Library – Learn to create engaging visualisations with Seaborn, a Python data visualization library.
Python Data Visualization Libraries – A comprehensive guide to various Python libraries for data visualization.
Wrapping Up: Mastering Scatter Plots with plt.scatter
In this comprehensive guide, we’ve explored how to create scatter plots in Python with matplotlib’s plt.scatter
function.
We started with the basics, showing how to create a simple scatter plot, and then delved into more advanced techniques, such as customizing the size, color, and shape of the markers.
We also discussed alternative approaches to creating scatter plots, particularly using plt.plot
with an ‘o’ marker. While plt.scatter
offers more customization options, plt.plot
can be a faster and simpler alternative for large datasets.
Along the way, we addressed common issues you might encounter when using plt.scatter
, such as mismatched array sizes and invalid values in data, and provided solutions to these problems.
Finally, we went beyond scatter plots, discussing the role of plt.scatter
in data analysis and machine learning, and suggesting related topics for further exploration, such as other types of plots in matplotlib and other data visualization libraries in Python.
Method | Customization | Speed | Complexity |
---|---|---|---|
plt.scatter | High | Moderate | Moderate |
plt.plot | Low | High | Low |
In conclusion, mastering plt.scatter
is an essential skill for anyone interested in data visualization in Python. Whether you’re a beginner just starting out with Python, an intermediate user looking to refine your skills, or a seasoned data professional, we hope this guide has been a valuable resource on your journey.